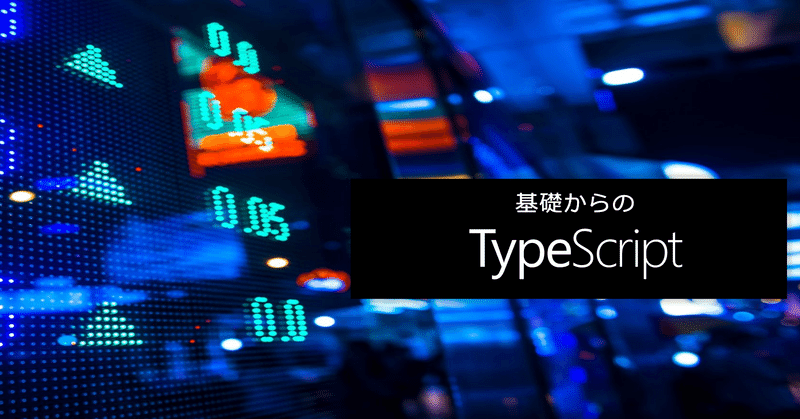
基礎からのTypeScript[オブジェクト指向]
外資系企業でソフトウェアエンジニアをしております、タロイモと言います。今日もよろしくお願いします。
前々回からTypeScriptの文法について解説しています。
今回は第三回としてTypeScriptのオブジェクト指向を説明していきます。
1. class
class構文
クラスの文法は以下の通りです。
class クラス名 {
プロパティ名: プロパティのデータ型
constructor(コンストラクタの引数: コンストラクタの引数のデータ型){
...
}
関数(引数: 引数のデータ型): 返り値のデータ型 {
...
}
}
具体的には以下のように書くことができます。
class Student {
name: string;
classNumber: number;
teacher: string;
constructor(name: string, classNumber: number, teacher: string) {
this.name = name;
this.className = className;
this.teacher = teacher;
}
introduce(): string {
return `私の名前は${this.name}です。${this.className}組で、担任は${this.teacher}です。`
}
}
let taroimo = new Student("タロイモ", 1, "じゃがいも");
console.log(taroimo.introduce()); //私の名前はタロイモです。1組で、担任はじゃがいもです。
アクセス修飾子
javaなどで当たり前になるclassのメンバへのアクセスを制御できるアクセス修飾子も対応しています。
public:クラスの外からアクセス可(デフォルト)
protected:同じクラスのメンバー、派生クラス(継承先のクラス)のメンバーからのみアクセス可
private:同じクラスからのみアクセス可
→プロパティ名の前に#をつけてもprivate修飾子になる。
getter, setter
getterとsetterはプロパティ名の先頭にgetかsetを付けるだけなので簡単です。
//getter
get プロパティ名(): 返り値のデータ型 {
return this.プライベートプロパティ名
}
//setter
set プロパティ名(引数: プロパティのデータ型){
this.プライベートプロパティ名 = 引数;
}
class Student {
private name: string;
classNumber: number;
teacher: string;
set name(value: string) {
this.name = value;
}
get name(): name {
return this.name;
}
}
let taroimo = new Student();
//setter
taroimo.name = "タロイモ";
//getter
console.log(taroimo.name); //タロイモ
static(静的メンバ)
staticは
・インスタンスを生成しなくても静的メソッドを実行できる。
・親クラスから派生したインスタンスがプロパティを共有できる。
という特徴があります。
class Student {
static name: string = "タロイモ";
static getName(): name {
return this.name;
}
}
console.log(Student.name); //タロイモ
console.log(Student.getName()); //タロイモ
let student1 = new Student();
let student2 = new Student();
console.log(student1.name); //タロイモ
console.log(student2.name); //タロイモ
2. 継承
継承はシンプルにできます。
class クラス名 extends 継承元クラス名 {
...
}
class School {
name: string;
constructor(name: string) {
this.name = name;
}
set name(value: string){
this.name = value;
}
get name() {
return this.name;
}
}
class HighSchool extends School{
...
}
let exSchool = new HighSchool("タロイモ高校");
exSchool.name(); //タロイモ高校
オーバーライド
typescriptではabstractクラスのオーバーライドも可能です。
class クラス名 extends 継承元クラス名 {
constructor(コンストラクタの引数: コンストラクタの引数のデータ型) {
super(コンストラクタの引数);
}
継承元クラスの関数名(): 関数の返り値のデータ型 {
return super.継承元クラスの関数名();
}
}
class School {
name: string;
constructor(name: string) {
this.name = name;
}
set name(value: string){
this.name = value;
}
introduceName(): string{
return this.name;
}
}
class HighSchool extends School{
founder: string;
constructor(name: string, founder: string) {
super(name);
this.founder = founder;
}
introduceName(): string{
return super.introduceName() + `、創立者は${this.founder}です。`;
}
}
let exSchool = new HighSchool("タロイモ高校", "タロイモ");
exSchool.introduceName(); //タロイモ高校、創立者はタロイモです。
3. インターフェース
少し長くなったので、インターフェースについては次の記事にて紹介します。
4. まとめ
お疲れ様でした。
つくづく感じるのはJavaとJavaScriptを理解していると、TypeScriptで新たに覚えることはほとんどないということです。
今回もご精読ありがとうございました。
よろしければサポートお願いします! サポートは、サービスの開発・改良や、記事を書く際の素材費とさせていただきます。 少しでも有益な情報発信をしていけるよう努めてまいります。 是非とも応援よろしくお願いします!!!🙇♂️