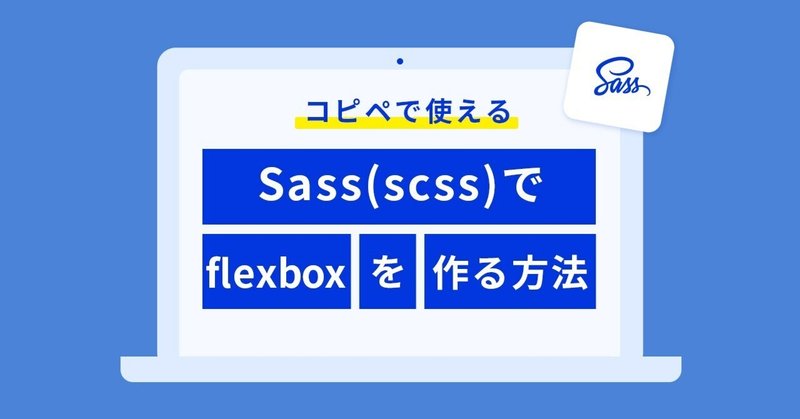
[コピペで使える]Sass(scss)でflexboxを作る方法2パターン
Sassでflexboxを使う時の方法を2つまとめました。
備忘録としてのメモです。
ちなみに、ベンダープレフィックスについてはコンパイルの時につける設定にしてくださいね!
方法1:classとして使う方法
Bootstrapとかみたいにcssフレームワーク的な感じでクラス名でflexboxを使いたい方向け。
scss
.flex {
display: flex;
&._fxd {
&_c {
flex-direction: column;
}
&_cr {
flex-direction: column-reverse;
}
&_r {
flex-direction: row;
}
&_rr {
flex-direction: row-reverse;
}
}
&._fxw {
&_n {
flex-wrap: nowrap;
}
&_w {
flex-wrap: wrap;
}
&_wr {
flex-wrap: wrap-reverse;
}
}
&._jc {
&_c {
justify-content: center;
}
&_fe {
justify-content: flex-end;
}
&_fs {
justify-content: flex-start;
}
&_sa {
justify-content: space-around;
}
&_sb {
justify-content: space-between;
}
}
&._ai {
&_b {
align-items: baseline;
}
&_c {
align-items: center;
}
&_fe {
align-items: flex-end;
}
&_fs {
align-items: flex-start;
}
&_s {
align-items: stretch;
}
}
&._ac {
&_c {
align-content: center;
}
&_fe {
align-content: flex-end;
}
&_fs {
align-content: flex-start;
}
&_s {
align-content: stretch;
}
&_sa {
align-content: space-around;
}
&_sb {
align-content: space-between;
}
}
}
.flex_item {
&._ord {
@for $i from 1 through 10 {
&_#{$i} {
order: $i;
}
}
}
}
実際コーディングで使う時はこんな感じ。
html
<div class="flex _jc_sb _ac_c">
<div>...</div>
<div>...</div>
<div>...</div>
</div>
コンパイル後のcssはこんな感じ。
css
.flex {
display: flex;
}
.flex._jc_sb {
justify-content: space-between;
}
.flex._ai_c {
align-items: center;
}
こちらを参照にしています
引用元:Sass(SCSS)でflexboxのComponentをつくる
方法2:mixinにして使いたい方
方法1のコンポーネント化して同じクラス名を使い回すのに対し、こちらはincludeで呼び出して使うパターンですね。
scss
@mixin flexbox() {
display: flex;
}
@mixin flex($values) {
flex: $values;
}
@mixin flex-direction($direction) {
flex-direction: $direction;
}
@mixin flex-wrap($wrap) {
flex-wrap: $wrap;
}
@mixin flex-flow($flow) {
flex-flow: $flow;
}
@mixin order($val) {
order: $val;
}
@mixin flex-grow($grow) {
flex-grow: $grow;
}
@mixin flex-shrink($shrink) {
flex-shrink: $shrink;
}
@mixin flex-basis($width) {
flex-basis: $width;
}
@mixin justify-content($justify) {
justify-content: $justify;
}
@mixin align-content($align) {
align-content: $align;
}
@mixin align-items($align) {
align-items: $align;
}
@mixin align-self($align) {
align-self: $align;
}
実際コーディングで使う時はこんな感じ。
html
<div class="hoge">
<div>...</div>
<div>...</div>
<div>...</div>
</div>
scss
.hoge{
@include flexbox;
@include justify-content(space-between);
}
コンパイル後のcssはこんな感じ。
css
.hoge {
display: flex;
align-items: center;
justify-content: space-between;
}
こちらを参照にしています
引用元:flexbox.scss
https://gist.github.com/richardtorres314/26b18e12958ba418bb37993fdcbfc1bd
方法2の方が一般的かなという気がしますが、個人的には方法1の方がscssの記述量も少ないし、cssがバッティングしづらいのかな〜という気がします。
みなさんはどちらがお好きですか?(^^)
この記事が気に入ったらサポートをしてみませんか?