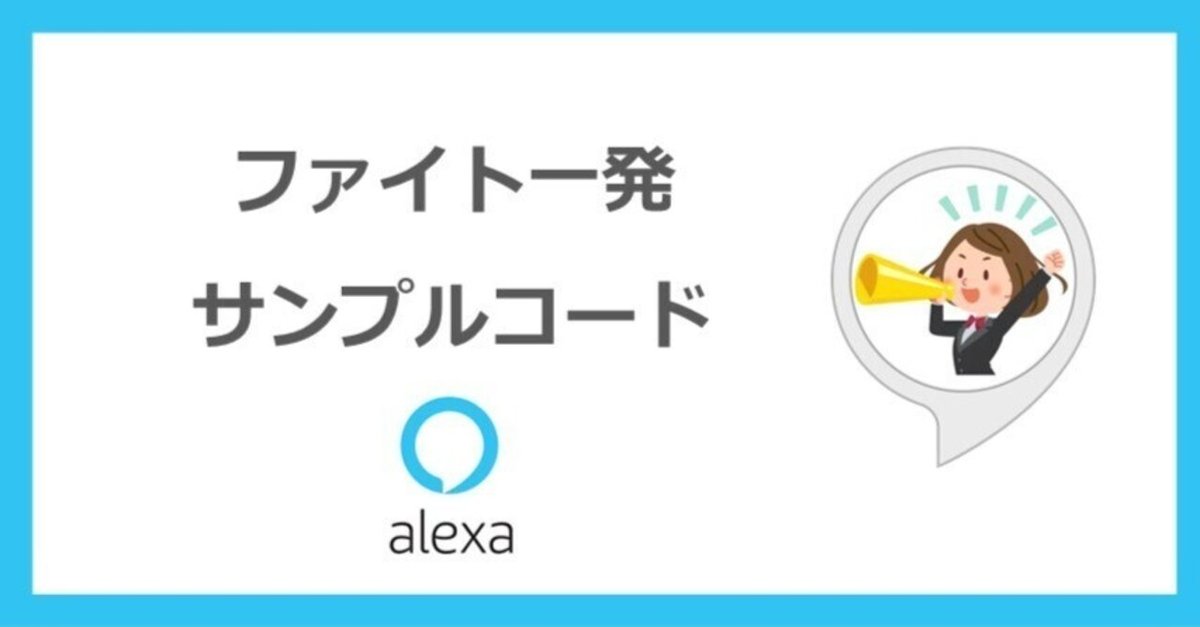
アレクサスキル サンプルコード ファイト一発
What's ファイト一発
アレクサが「(ファイト!一発!」と応援してくれるスキルです。
工夫ポイント
効果音 5種類 (ホイッスル / 拍手)
2行目 3種類 (ファイトの行)
3行目 12種類(今日も~の行)
のランダムで組合せて対話文を作るので、5x3x12 = 72 種類の言い方を生成します。
サンプルコード
200行ほどのコードです。
コードエディタにコピペした後、セリフを編集する等してオリジナルスキルをつくってみて下さい。
/* *
* This sample demonstrates handling intents from an Alexa skill using the Alexa Skills Kit SDK (v2).
* Please visit https://alexa.design/cookbook for additional examples on implementing slots, dialog management,
* session persistence, api calls, and more.
* */
//効果音
const range_se = 5;
const op1 ='<audio src="soundbank://soundlibrary/sports/whistles/sports_whistles_02"/>';
const op2 ='<audio src="soundbank://soundlibrary/sports/whistles/sports_whistles_03"/>';
const op3 ='<audio src="soundbank://soundlibrary/sports/whistles/sports_whistles_04"/>';
const op4 ='<audio src="soundbank://soundlibrary/sports/whistles/sports_whistles_05"/>';
const op5 ='<audio src="soundbank://soundlibrary/sports/whistles/sports_whistles_08"/>';
const ops = [op1, op2, op3, op4, op5];
const end1 ='<audio src="soundbank://soundlibrary/human/amzn_sfx_crowd_applause_01"/>';
const end2 ='<audio src="soundbank://soundlibrary/human/amzn_sfx_crowd_applause_02"/>';
const end3 ='<audio src="soundbank://soundlibrary/human/amzn_sfx_crowd_applause_03"/>';
const end4 ='<audio src="soundbank://soundlibrary/human/amzn_sfx_crowd_applause_04"/>';
const end5 ='<audio src="soundbank://soundlibrary/human/amzn_sfx_crowd_applause_05"/>';
const ends = [end1, end2, end3, end4, end5];
const break05 = '<break time="0.5s"/>';
// フレーズ集
const range_f = 3;
const f1 = '<amazon:emotion name="excited" intensity="low"> ファイトー。<break time="0.5s"/><prosody volume="x-loud"> <prosody rate="65%"> 一発 </prosody></prosody> </amazon:emotion><break time="1s"/>';
const f2 = '<amazon:emotion name="excited" intensity="medium"> ファイトー。<break time="0.5s"/><prosody volume="x-loud"> <prosody rate="65%"> 一発 </prosody></prosody> </amazon:emotion><break time="1s"/>';
const f3 = '<amazon:emotion name="excited" intensity="high"> ファイトー。<break time="0.5s"/><prosody volume="x-loud"> <prosody rate="65%"> 一発 </prosody></prosody> </amazon:emotion><break time="1s"/>';
const fs = [f1, f2, f3];
// フレーズ集
const range_c = 12;
const c1 = '<amazon:emotion name="excited" intensity="low"> 今日もいちにち。ガンバレ! </amazon:emotion><break time="0.5s"/>';
const c2 = '<amazon:emotion name="excited" intensity="low"> いっつも応援してるよ! </amazon:emotion><break time="0.5s"/>。';
const c3 = '<amazon:emotion name="excited" intensity="low"> あなたの頑張り、いつも見てるね! </amazon:emotion><break time="0.5s"/>。';
const c4 = '<amazon:emotion name="excited" intensity="low"> みんなのために。立派だね! </amazon:emotion><break time="0.5s"/>。';
const c5 = '<amazon:emotion name="excited" intensity="low"> カッコイイ! </amazon:emotion><break time="0.5s"/>。';
const c6 = '<amazon:emotion name="excited" intensity="low"> いっつも、ステキだね! </amazon:emotion><break time="0.5s"/>。';
const c7 = '<amazon:emotion name="excited" intensity="low"> あなたらしく、いてくださいね?</amazon:emotion><break time="0.5s"/>。';
const c8 = '<amazon:emotion name="excited" intensity="low"> わたしは、あなたの味方だよ? </amazon:emotion><break time="0.5s"/>。';
const c9 = '<amazon:emotion name="excited" intensity="low"> 良いいち日を! </amazon:emotion><break time="0.5s"/>。';
const c10 = '<amazon:emotion name="excited" intensity="low"> 無理しちゃダメだよ?たまには休んじゃえ! </amazon:emotion><break time="0.5s"/>。';
const c11 = '<amazon:emotion name="excited" intensity="low"> あなたなら、大丈夫! </amazon:emotion><break time="0.5s"/>。';
const c12 = '<amazon:emotion name="excited" intensity="low"> いっつも、そばにいるよ?</amazon:emotion><break time="0.5s"/>。';
const cs = [c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12];
const Alexa = require('ask-sdk-core');
const LaunchRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'LaunchRequest';
},
handle(handlerInput) {
//効果音
function getRandomInt_se(range_se) {
return Math.floor(Math.random() * range_se);
}
let count_se = getRandomInt_se(range_se);
let op = (ops [ count_se ]);
let end = (ends [ count_se ]);
//ファイト
function getRandomInt_f(range_f) {
return Math.floor(Math.random() * range_f);
}
let count_f = getRandomInt_f(range_f);
let f = (fs [ count_f ]);
//cheers
function getRandomInt_c(range_c) {
return Math.floor(Math.random() * range_c);
}
let count_c = getRandomInt_c(range_c);
let c = (cs [ count_c ]);
//最終発話
const speakOutput = op + f + c + break05 + end;
return handlerInput.responseBuilder
.speak(speakOutput)
//.reprompt(speakOutput)
.getResponse();
}
};
const HelloWorldIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'HelloWorldIntent';
},
handle(handlerInput) {
const speakOutput = '<amazon:emotion name="excited" intensity="low">こんにちは。</amazon:emotion>' +
op1 + f2 + c1 + break05 + end1 ;
return handlerInput.responseBuilder
.speak(speakOutput)
//.reprompt('add a reprompt if you want to keep the session open for the user to respond')
.getResponse();
}
};
const HelpIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.HelpIntent';
},
handle(handlerInput) {
const speakOutput = '<amazon:emotion name="excited" intensity="low"> こんにちは。って言ってみて?全力で応援するよ。 </amazon:emotion>';
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
const CancelAndStopIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& (Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.CancelIntent'
|| Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.StopIntent');
},
handle(handlerInput) {
const speakOutput = '<amazon:emotion name="excited" intensity="low">じゃあね。また、あした! </amazon:emotion>';
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
/* *
* FallbackIntent triggers when a customer says something that doesn’t map to any intents in your skill
* It must also be defined in the language model (if the locale supports it)
* This handler can be safely added but will be ingnored in locales that do not support it yet
* */
const FallbackIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.FallbackIntent';
},
handle(handlerInput) {
const speakOutput = 'Sorry, I don\'t know about that. Please try again.';
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
/* *
* SessionEndedRequest notifies that a session was ended. This handler will be triggered when a currently open
* session is closed for one of the following reasons: 1) The user says "exit" or "quit". 2) The user does not
* respond or says something that does not match an intent defined in your voice model. 3) An error occurs
* */
const SessionEndedRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'SessionEndedRequest';
},
handle(handlerInput) {
console.log(`~~~~ Session ended: ${JSON.stringify(handlerInput.requestEnvelope)}`);
// Any cleanup logic goes here.
return handlerInput.responseBuilder.getResponse(); // notice we send an empty response
}
};
/* *
* The intent reflector is used for interaction model testing and debugging.
* It will simply repeat the intent the user said. You can create custom handlers for your intents
* by defining them above, then also adding them to the request handler chain below
* */
const IntentReflectorHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest';
},
handle(handlerInput) {
const intentName = Alexa.getIntentName(handlerInput.requestEnvelope);
const speakOutput = `You just triggered ${intentName}`;
return handlerInput.responseBuilder
.speak(speakOutput)
//.reprompt('add a reprompt if you want to keep the session open for the user to respond')
.getResponse();
}
};
/**
* Generic error handling to capture any syntax or routing errors. If you receive an error
* stating the request handler chain is not found, you have not implemented a handler for
* the intent being invoked or included it in the skill builder below
* */
const ErrorHandler = {
canHandle() {
return true;
},
handle(handlerInput, error) {
const speakOutput = 'Sorry, I had trouble doing what you asked. Please try again.';
console.log(`~~~~ Error handled: ${JSON.stringify(error)}`);
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
/**
* This handler acts as the entry point for your skill, routing all request and response
* payloads to the handlers above. Make sure any new handlers or interceptors you've
* defined are included below. The order matters - they're processed top to bottom
* */
exports.handler = Alexa.SkillBuilders.custom()
.addRequestHandlers(
LaunchRequestHandler,
HelloWorldIntentHandler,
HelpIntentHandler,
CancelAndStopIntentHandler,
FallbackIntentHandler,
SessionEndedRequestHandler,
IntentReflectorHandler)
.addErrorHandlers(
ErrorHandler)
.withCustomUserAgent('sample/hello-world/v1.2')
.lambda();
インテント
スキルの呼び出しと共に発話して終了するスキルなので実質使用しませんが、ALEXA Skillの仕様上カスタムインテントを最低1つは登録する必要があるため、HelloWorldIntentで適当に登録します。
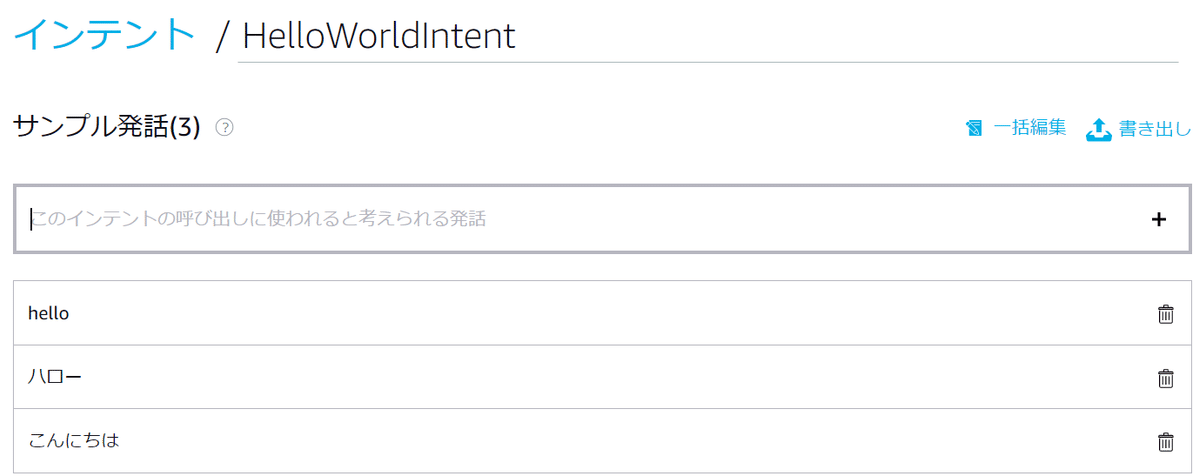
この記事が気に入ったらサポートをしてみませんか?