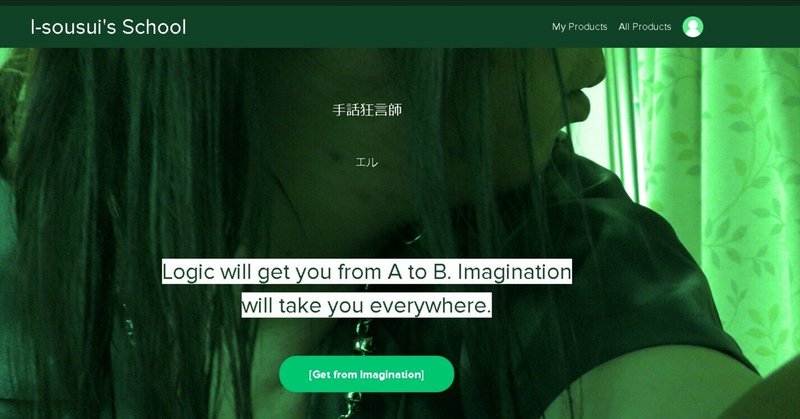
Make some games with html and javascript.適当に遊んでみた。
Guessing Game:
htmlCopy code
<!DOCTYPE html> <html> <head> <title>Guessing Game</title> </head> <body> <h1>Guessing Game</h1> <p>Try to guess the number between 1 and 100:</p> <input type="text" id="guessInput"> <button onclick="checkGuess()">Submit</button> <p id="result"></p> <script> var secretNumber = Math.floor(Math.random() * 100) + 1; function checkGuess() { var guess = parseInt(document.getElementById('guessInput').value); if (isNaN(guess)) { document.getElementById('result').textContent = 'Please enter a valid number.'; } else if (guess < secretNumber) { document.getElementById('result').textContent = 'Too low! Try again.'; } else if (guess > secretNumber) { document.getElementById('result').textContent = 'Too high! Try again.'; } else { document.getElementById('result').textContent = 'Congratulations! You guessed it!'; } document.getElementById('guessInput').value = ''; } </script> </body> </html>
Tic-Tac-Toe Game:
htmlCopy code
<!DOCTYPE html> <html> <head> <title>Tic-Tac-Toe</title> <style> .cell { width: 100px; height: 100px; border: 1px solid black; text-align: center; font-size: 50px; cursor: pointer; } </style> </head> <body> <h1>Tic-Tac-Toe</h1> <div id="board"> <div class="cell" onclick="makeMove(0)"></div> <div class="cell" onclick="makeMove(1)"></div> <div class="cell" onclick="makeMove(2)"></div> <div class="cell" onclick="makeMove(3)"></div> <div class="cell" onclick="makeMove(4)"></div> <div class="cell" onclick="makeMove(5)"></div> <div class="cell" onclick="makeMove(6)"></div> <div class="cell" onclick="makeMove(7)"></div> <div class="cell" onclick="makeMove(8)"></div> </div> <button onclick="resetBoard()">Reset</button> <script> var currentPlayer = 'X'; var board = ['', '', '', '', '', '', '', '', '']; function makeMove(index) { if (board[index] === '') { board[index] = currentPlayer; document.getElementById('board').children[index].textContent = currentPlayer; currentPlayer = currentPlayer === 'X' ? 'O' : 'X'; } } function resetBoard() { currentPlayer = 'X'; board = ['', '', '', '', '', '', '', '', '']; var cells = document.getElementById('board').children; for (var i = 0; i < cells.length; i++) { cells[i].textContent = ''; } } </script> </body> </html>
<!DOCTYPE html> <html> <head> <title>Memory Game</title> <style> .card { width: 100px; height: 100px; background-color: #ccc ;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Memory Game</h1>
<div id="gameBoard">
<div class="card" onclick="flipCard(0)"></div>
<div class="card" onclick="flipCard(1)"></div>
<div class="card" onclick="flipCard(2)"></div>
<div class="card" onclick="flipCard(3)"></div>
<div class="card" onclick="flipCard(4)"></div>
<div class="card" onclick="flipCard(5)"></div>
<div class="card" onclick="flipCard(6)"></div>
<div class="card" onclick="flipCard(7)"></div>
<div class="card" onclick="flipCard(8)"></div>
<div class="card" onclick="flipCard(9)"></div>
<div class="card" onclick="flipCard(10)"></div>
<div class="card" onclick="flipCard(11)"></div>
</div>
<button onclick="resetGame()">Reset</button>
<script>
var cards = ['A', 'A', 'B', 'B', 'C', 'C', 'D', 'D', 'E', 'E', 'F', 'F'];
var flippedCards = [];
var matchedCards = [];
function flipCard(index) {
if (matchedCards.includes(index) || flippedCards.length === 2) {
return;
}
if (flippedCards.length === 0) {
flippedCards.push(index);
document.getElementById('gameBoard').children[index].textContent = cards[index];
} else if (flippedCards.length === 1 && !flippedCards.includes(index)) {
flippedCards.push(index);
document.getElementById('gameBoard').children[index].textContent = cards[index];
if (cards[flippedCards[0]] === cards[flippedCards[1]]) {
matchedCards.push(flippedCards[0]);
matchedCards.push(flippedCards[1]);
}
setTimeout(function() {
hideCards();
}, 1000);
}
}
function hideCards() {
if (matchedCards.includes(flippedCards[0]) || matchedCards.includes(flippedCards[1])) {
return;
}
document.getElementById('gameBoard').children[flippedCards[0]].textContent = '';
document.getElementById('gameBoard').children[flippedCards[1]].textContent = '';
flippedCards = [];
}
function resetGame() {
flippedCards = [];
matchedCards = [];
shuffleArray(cards);
var cardsHTML = document.getElementById('gameBoard').children;
for (var i = 0; i < cardsHTML.length; i++) {
cardsHTML[i].textContent = '';
}
}
// Helper function to shuffle an array
function shuffleArray(array) {
for (var i = array.length - 1; i > 0; i--) {
var j = Math.floor(Math.random() * (i + 1));
var temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
</script>
</body>
</html>
この記事が気に入ったらサポートをしてみませんか?