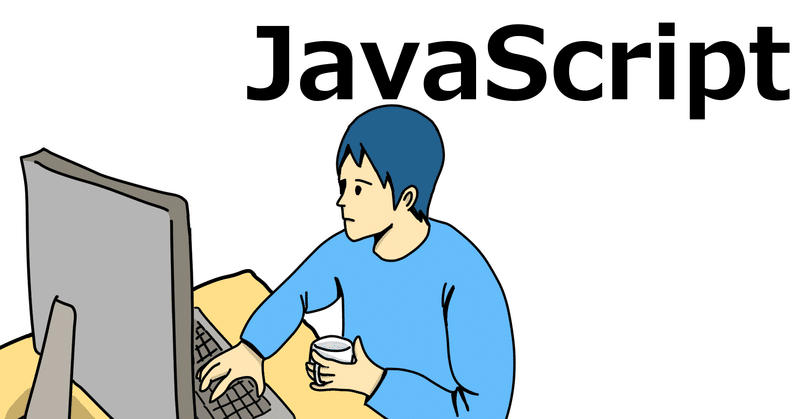
【JavaScript】画像操作
画像オブジェクト
Image 画像オブジェクト
画像を扱うオブジェクトです
例
mImg = new Image()
mImageを画像オブジェクトとする
サンプル
<html>
<head>
<title>サンプル</title>
<script language="JavaScript">
<!--
mImage = new Image();
mImage.src = "000.jpg";
//-->
</script>
</head>
<body>
<h2>画像オブジェクト</h2>
<img src="111.jpg" name="img" width="32" height="32"><br />
<a href="#" onmouseover="img.src='222.jpg'" onmouseout="img.src='444.jpg'">マウスを乗せると表示する</a>
</body>
</html>
画像の枠幅
border 枠幅
画像の枠を返します
例
alert("枠幅は" + document.mPhoto.border + "です")
mPhotoという名前の画像の枠幅をアラートダイアログに表示する
alert("枠幅は" + document.image[2].border + "です")
3番目の画像の枠幅をアラートダイアログに表示する
サンプル
<html>
<head>
<title>サンプル</title>
</head>
<body>
<h2>画像の枠幅</h2>
<a href=""><img src="000.jpg" name="img" border="1"></a><br />
<a href="#" onmouseover="alert(img.border)">枠幅表示</a><br />
</body>
</html>
画像の読み込み状態
complete 読み込み完了状態画像が完全に読み込まれたかどうかを返します
例
alert(document.mPhoto.complete)
mPhotoという名前の画像の読み込み完了状態を表示する
alert(document.images[4].complete)
5番目の画像の読み込み完了状態を表示する
サンプル
<html>
<head>
<title>サンプル</title>
</head>
<body>
<h2>画像の読み込み状態</h2>
<img src="000.jpg" name="img">----
<img src="111.jpg" name="img2"><br />
<a href="#" onmouseover="alert(img.complete)">左の画像状態表示</a><br />
<a href="#" onmouseover="alert(img2.complete)">右の画像状態表示</a><br />
</body>
</html>
画像の縦横幅
height 縦幅
width 横幅
画像の縦、横の幅を返します
例
alert(document.mPhoto.width)
mPhotoという名前の画像の横幅アラートダイアログに表示する
wd = document.Images[0].height
一番最初の画像の横幅を変数wdに代入する
document.myChar.width = 256
画像名mCharの横幅256ピクセルにする
サンプル
<html>
<head>
<title>サンプル</title>
</head>
<body>
<h2>画像の縦横幅</h2>
<img src="000.jpg" name="img" width="48" height="32"><br />
<a href="#" onmouseover="alert(img.width)">横幅表示</a><br />
<a href="#" onmouseover="alert(img.height)">縦幅表示</a><br />
</body>
</html>
画像と文字の間隔
hspace 文字との縦の問題
vspace 文字との横の問題
画像と文字との間隔の縦、横を返します。
例
alert(document.mPhoto.vspace)
mPhotoという名前の画像と文字との横幅を表示する
alert(document.mPhoto.hspace)
mPhotoという名前の画像と文字との縦幅を表示する
サンプル
<html>
<head>
<title>サンプル</title>
</head>
<body>
<h2>画像と文字の間隔</h2>
<img src="000.jpg" name="img" hspace="12" vspace="8"><br />
<a href="#" onmouseover="alert(img.hspace)">横間幅表示</a><br />
<a href="#" onmouseover="alert(img.vspace)">縦間幅表示</a><br />
</body>
</html>
画像名/画像数
length 画像数
name 画像名
画像の総数、名前を返します
例
num = document.images.length
画像総数を変数numに代入する
fName = document.images[0].name
最初の画像の名前を変数fNameに代入する
サンプル
<html>
<head>
<title>サンプル</title>
</head>
<body>
<h2>画像名/画像数</h2>
<img src="000.jpg">
<img src="001.jpg">
<img src="002.jpg">
<img src="003.jpg">
<br />
<a href="#" onmouseover="alert(document.images.length)">画像総数</a>
</body>
</html>
画像アドレス
lowsrc 低解像度画像URL
src 画像URL
画像のアドレス(URL)を読み出し/書き込みます
例
mImg = new Image()
mImg.src = "000.jpg"
"000.jpg"画像プレロードを行う
tURL = document.mPhoto.src
mPhotoという名前の画像アドレス(URL)を変数tURLに代入
サンプル
<html>
<head>
<title>サンプル</title>
</head>
<body>
<h2>画像アドレス</h2>
<img src="000.jpg" lowsrc="001.jpg" name="img" width="32" height="12"><br />
<a href="#" onmouseover="alert(img.lowsrc)">lowsrcアドレス表示</a><br />
<a href="#" onmouseover="alert(img.src)">srcアドレス表示</a>
</body>
</html>
イベント設定
onabort 読み込み中断時
onerror 読み込みエラー発生時
onload 読み込み完了時
画像に対して発生したイベントを設定します
例
document.mImg.onbort = abortFunc
mImgという名前の画像の読み古中断したときの処理先をaboutFuncにする
dcoument.mImg.onerror = errFunc
mImgという名前の画像の読み込みエラー時の処理先をerrFuncにする
document.mImg.onload = oadFunc
mImgという名前の画像の読み込み完了時の処理先をloadFuncにする
サンプル
<html>
<head>
<title>サンプル</title>
<script language="JavaScript">
<!--
function check() {
alert("画像が正常に読み込みませんでした");
}
//-->
</script>
</head>
<body>
<h2>イベント設定</h2>
<img src="000.jpg" name="img"><br />
<script language="JavaScript">
<!--
document.img.onerror = check;
//-->
</script>
</body>
</html>
キーイベント設定
onkeydown キーダウン
onkeypress キープレス
onkeyup キーアップ
画像に対して発生したイベントを設定します
例
document.mImg.onkeydown = keyFunc
mImgという名前の画像上でキーが押されたときの処理先をkeyFuncにする
document.mImg.onkeypress = keyFunc
mImgという名前の画像上でキーがリピート時の処理先をkeyFuncにする
document.mImg.onkeyup = keyFunc
mImgという名前の画像上でキーが離されたときの処理先をkeyFuncにする
サンプル
<html>
<head>
<title>サンプル</title>
<script language="JavaScript">
<!--
function Keycheck() {
alert("キーが押されました");
}
//-->
</script>
</head>
<body>
<h2>キーイベント設定</h2>
<img src="000.jpg" name="img"><br />
<script language="JavaScript">
<!--
document.img.onkeydown = Keycheck;
//-->
</script>
</body>
</html>
この記事が気に入ったらサポートをしてみませんか?