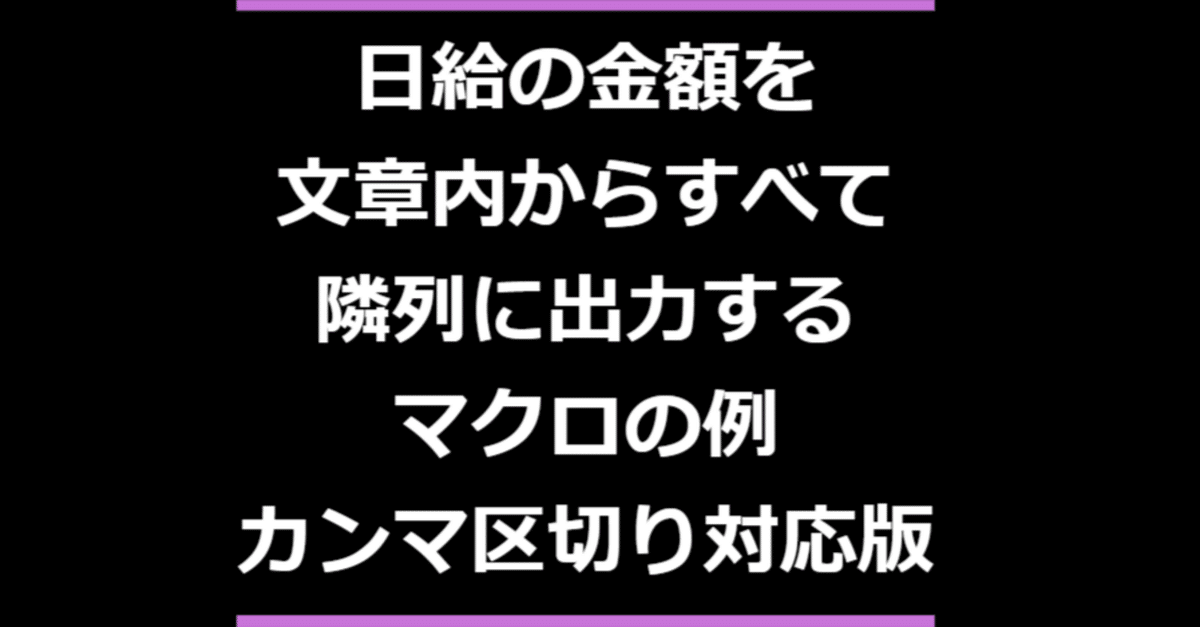
文章内にある日給をすべて隣列にだすよカンマ区切り3種対応
この説明は、ChatGPTで作成しています。
概要
このプロシージャは、Excelのセル内に記載された「日給〇〇円」という金額を自動で見つけ出し、その金額部分を隣のセルに数値として出力します。日給の表記に使われている「,」(カンマ)や「、」(日本語の読点)、そして「.」(ピリオド)にも対応しているため、様々な書き方の「日給」を正しく抽出することができます。
プロシージャの仕組み
画面更新の停止
最初に、`Application.ScreenUpdating = False` というコードで、画面更新を停止します。これにより、処理中の画面のチラつきを防ぎ、動作をスムーズにします。正規表現オブジェクトの作成
`CreateObject("VBScript.RegExp")` を使って、2つの正規表現オブジェクトを作成しています。`reg`: 「日給〇〇円」というパターンにマッチするためのオブジェクト
`regClean`: 数字以外の文字(「日給」「円」や「,」「、」「.」)を取り除くためのオブジェクト
正規表現パターンの設定
`reg` のパターン
`日給\d{4,5}円|日給(\d{1,3}([,、.]\d{3})*)円` という設定で、「日給10000円」や「日給12,000円」「日給12、000円」などの様々な日給表記に対応しています。`regClean` のパターン
`[^\d]` により数字以外の文字を取り除くよう設定しています。
セルの値を一つずつ確認
`For Each myRng In Selection` により、選択範囲のセルを一つずつ確認します。`reg.Execute(txt)` によって、そのセル内の「日給〇〇円」の文字列をすべて見つけ出します。
`regClean.Replace()` により、「日給」や「円」、カンマなどを取り除いて、純粋な数字だけを隣のセルに出力します。
画面更新の再開
最後に `Application.ScreenUpdating = True` で画面の更新を再開します。
ポイント
正規表現を使って文字列を見つけ出し、必要な部分だけを抽出しています。
選択範囲内の複数のセルに対応しているので、一度にたくさんのデータを処理できます。
こんな時に使える
Excel上で大量のデータから「日給」を探して、金額をまとめたいとき
日給がカンマやピリオド、読点を含む場合でも正しく数値として扱いたいとき
参考リンク
Sub 文章内にある日給をすべて隣列にだすよカンマ区切り3種対応()
Application.ScreenUpdating = False
Dim reg As Object, regClean As Object
Set reg = CreateObject("VBScript.RegExp") 'オブジェクト作成
Set regClean = CreateObject("VBScript.RegExp") ' クリーニング用のオブジェクト作成
Dim myRng As Range
Dim txt As String
Dim match As Object
Dim i As Long
' 時給パターンの正規表現
With reg
.Pattern = "日給\d{4,5}円|日給(\d{1,3}([,、.]\d{3})*)円"
.IgnoreCase = True
.Global = True
End With
' クリーニング用の正規表現パターン
With regClean
.Pattern = "[^0-9]" ' 数字以外の文字をすべて取り除く
.IgnoreCase = True
.Global = True
End With
On Error Resume Next
For Each myRng In Selection
txt = myRng.Value
Set match = reg.Execute(txt)
For i = 1 To match.count
' matchの結果をクリーニングして隣のセルに出力
myRng.Offset(0, i).Value = regClean.Replace(match(i - 1).Value, "")
Next i
Next myRng
Application.ScreenUpdating = True
End Sub
関連キーワード
#excel #できること #vba #正規表現 #日給抽出 #データクレンジング #セル操作 #スクリーンアップデート #自動化 #カンマ対応 #テキスト操作 #データ抽出 #隣セル出力 #金額抽出 #VBScript #クリーニング #選択範囲 #データ処理 #効率化 #プログラミング
English Translation
Extract Daily Wages from Text and Output Them in Adjacent Columns (Supports 3 Comma Types)
This explanation is created by ChatGPT.
Overview
This procedure scans Excel cells for any text containing "日給〇〇円" (meaning daily wage in Japanese yen) and extracts the numeric values to the adjacent cell. It can handle different notations using "," (comma), "、" (Japanese comma), and "." (period) within the wage amount.
How the Procedure Works
Stopping Screen Updates
The code `Application.ScreenUpdating = False` stops screen updates, which prevents flickering and speeds up the process.Creating Regular Expression Objects
Using `CreateObject("VBScript.RegExp")`, two regular expression objects are created:`reg`: Finds patterns matching "日給〇〇円"
`regClean`: Removes non-numeric characters, such as "日給", "円", "," (comma), "、", and "." (period).
Setting Regular Expression Patterns
For `reg`: The pattern `日給\d{4,5}円|日給(\d{1,3}([,、.]\d{3})*)円` matches variations like "日給10000円", "日給12,000円", "日給12、000円", etc.
For `regClean`: The pattern `[^\d]` removes all non-numeric characters.
Processing Each Cell
The loop `For Each myRng In Selection` iterates over the selected cells.`reg.Execute(txt)` finds all occurrences of "日給〇〇円" within the cell.
`regClean.Replace()` removes unnecessary characters, leaving only the pure numeric value, which is outputted to the adjacent cell.
Restarting Screen Updates
Finally, `Application.ScreenUpdating = True` restarts the screen updates.
Key Points
The use of regular expressions enables flexible text matching and extraction.
The code can handle multiple cells at once, making it efficient for processing large datasets.
When to Use This
When you need to extract daily wage amounts from Excel data quickly
When daily wage amounts include commas, periods, or different punctuation marks
Reference Links
Related Keywords
#excel #capabilities #vba #regex #dailywageextraction #datacleaning #celloperations #screenupdate #automation #commasupport #textprocessing #dataextraction #adjacentcelloutput #wageextraction #VBScript #datacleaning #dataselection #dataprocessing #efficiency #programming
この記事が気に入ったらサポートをしてみませんか?