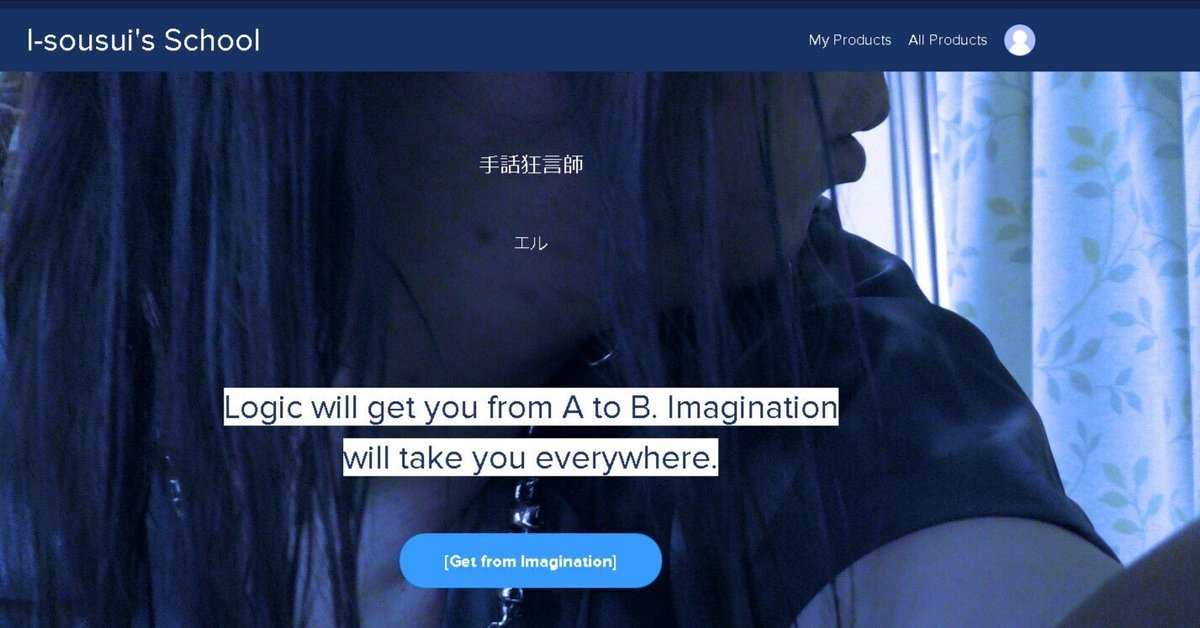
Djangoを使ったサイトをプログラミングをし、サンプルを作って。
Certainly! Django is a powerful web framework that allows you to build robust and scalable websites. Here's a basic example of how you can create a sample site using Django:
Step 1: Set up the Django Project
Install Django by running the following command in your command prompt or terminal:Copy codepip install django
Create a new Django project by running:Copy codedjango-admin startproject sample_site
Step 2: Create a Django App
Change directory into the project folder:bashCopy codecd sample_site
Create a new Django app within the project:Copy codepython manage.py startapp sample_app
Step 3: Configure the Database
Open the settings.py file inside the sample_site folder.
Update the DATABASES section with your database details, such as the database engine, name, user, and password.
Step 4: Define Models
Open the models.py file inside the sample_app folder.
Define your models using Django's model syntax, representing the data you want to store. For example:pythonCopy codefrom django.db import models class SampleModel(models.Model): name = models.CharField(max_length=100) description = models.TextField() created_at = models.DateTimeField(auto_now_add=True)
Step 5: Create Database Tables
Apply the migrations to create the necessary database tables:Copy codepython manage.py makemigrations python manage.py migrate
Step 6: Create Views
Open the views.py file inside the sample_app folder.
Define your views, which handle HTTP requests and return responses. For example:pythonCopy codefrom django.shortcuts import render from .models import SampleModel def sample_view(request): samples = SampleModel.objects.all() return render(request, 'sample.html', {'samples': samples})
Step 7: Create Templates
Create a folder named templates inside the sample_app folder.
Inside the templates folder, create a file named sample.html and define the HTML template for displaying the samples. For example:htmlCopy code<html> <head> <title>Sample Site</title> </head> <body> <h1>Samples:</h1> <ul> {% for sample in samples %} <li>{{ sample.name }} - {{ sample.description }}</li> {% endfor %} </ul> </body> </html>
Step 8: Configure URLs
Open the urls.py file inside the sample_site folder.
Add the URL pattern to map to your view. For example:pythonCopy codefrom django.urls import path from sample_app.views import sample_view urlpatterns = [ path('samples/', sample_view, name='sample-view'), ]
Step 9: Run the Development Server
Start the development server by running the following command:Copy codepython manage.py runserver
Now, you can access your sample site by navigating to http://localhost:8000/samples/ in your web browser. It will display a list of samples fetched from the database.
This is just a basic example to get you started. You can further customize and enhance your site by adding forms, authentication, additional views, and more, depending on your requirements.
この記事が気に入ったらサポートをしてみませんか?