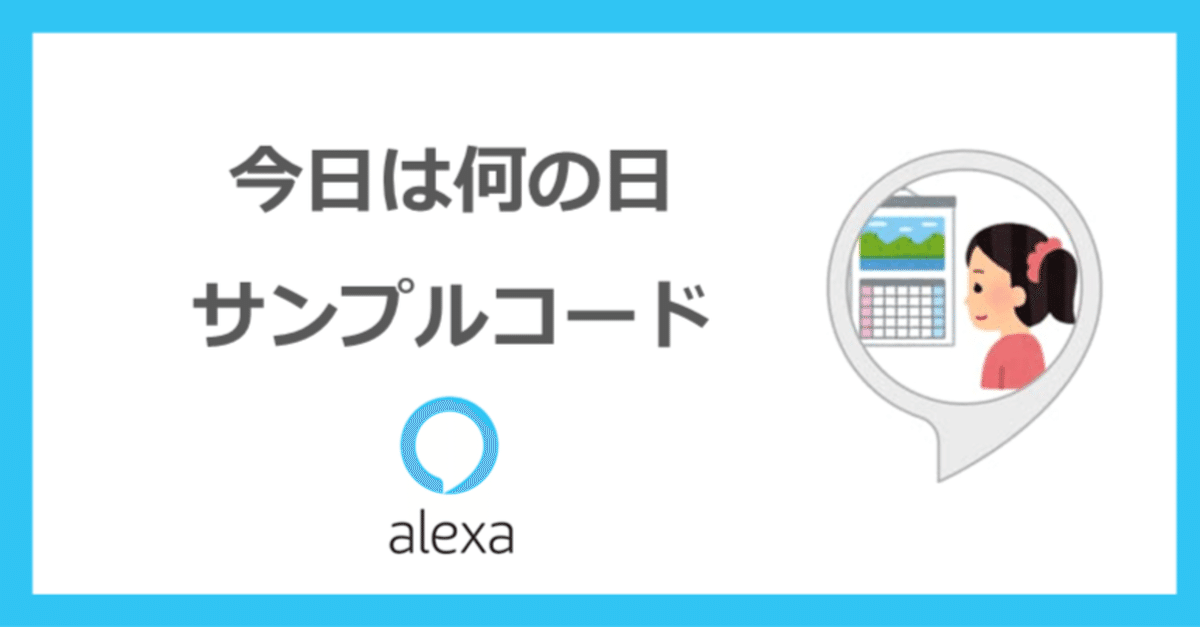
アレクサスキル サンプルコード 今日は何の日?
What's 今日は何の日
今日の日付に関する記念日と由来を教えてくれるスキルです。
サンプルコード
200行ほどのコードです。
コードエディタにコピペした後、以下の値を変えた派生のスキルをつくってみてください。
/* *
* This sample demonstrates handling intents from an Alexa skill using the Alexa Skills Kit SDK (v2).
* Please visit https://alexa.design/cookbook for additional examples on implementing slots, dialog management,
* session persistence, api calls, and more.
今日は何の日を開いて
* */
/*
毎日記念日;https://netwadai.com/blog/post-2363#1%E6%9C%88%E3%81%AE%E8%A8%98%E5%BF%B5%E6%97%A5%E4%B8%80%E8%A6%A7
http://hukumusume.com/366/kinenbi/pc/itiran/01gatu.htm
エクセル=>json変換サイト;https://tableconvert.com/ja/excel-to-json
サンプルコード 引用元:https://www.servernote.net/article.cgi?id=javascript-find-filter-in-jsonarray
*/
const Alexa = require('ask-sdk-core');
const LaunchRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'LaunchRequest';
},
handle(handlerInput) {
//日付取得 ex)12-24
let today = new Date();
let month = today.getMonth() + 1;
let date = today.getDate();
today = month + '-' + date;
//today = '06-13'; //テスト用
//曜日取得
const dayList = ['日', '月', '火', '水', '木', '金', '土'];
let day = dayList[new Date().getDay()];
//今日の名前を取得 xxの日
let allDate = require("./date.json");
let dateData = allDate.dateData.find((v) => v.date == today);
let dateName = dateData.name;
let dateMeans = dateData.means;
//発話生成
const speakOutput = '今日は' + month + '月' + date + '日。'
+ day + '曜日、'
+ '<break time="1s"/>'
+ dateName + 'です。' + dateMeans
+ '<break time="1.5s"/>'
+'さて、明日は「何の日」でしょうか。' + '<break time="1s"/>' + 'また明日も聞いて下さい。'
+ '<say-as interpret-as="interjection">それでは</say-as>.';
return handlerInput.responseBuilder
.speak(speakOutput)
//.reprompt(speakOutput)
.getResponse();
}
};
const HelloWorldIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'HelloWorldIntent';
},
handle(handlerInput) {
const speakOutput = '';
return handlerInput.responseBuilder
.speak(speakOutput)
//.reprompt('add a reprompt if you want to keep the session open for the user to respond')
.getResponse();
}
};
const HelpIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.HelpIntent';
},
handle(handlerInput) {
const speakOutput = 'You can say hello to me! How can I help?';
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
const CancelAndStopIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& (Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.CancelIntent'
|| Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.StopIntent');
},
handle(handlerInput) {
const speakOutput = 'Goodbye!';
return handlerInput.responseBuilder
.speak(speakOutput)
.getResponse();
}
};
/* *
* FallbackIntent triggers when a customer says something that doesn’t map to any intents in your skill
* It must also be defined in the language model (if the locale supports it)
* This handler can be safely added but will be ingnored in locales that do not support it yet
* */
const FallbackIntentHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest'
&& Alexa.getIntentName(handlerInput.requestEnvelope) === 'AMAZON.FallbackIntent';
},
handle(handlerInput) {
const speakOutput = 'Sorry, I don\'t know about that. Please try again.';
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
/* *
* SessionEndedRequest notifies that a session was ended. This handler will be triggered when a currently open
* session is closed for one of the following reasons: 1) The user says "exit" or "quit". 2) The user does not
* respond or says something that does not match an intent defined in your voice model. 3) An error occurs
* */
const SessionEndedRequestHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'SessionEndedRequest';
},
handle(handlerInput) {
console.log(`~~~~ Session ended: ${JSON.stringify(handlerInput.requestEnvelope)}`);
// Any cleanup logic goes here.
return handlerInput.responseBuilder.getResponse(); // notice we send an empty response
}
};
/* *
* The intent reflector is used for interaction model testing and debugging.
* It will simply repeat the intent the user said. You can create custom handlers for your intents
* by defining them above, then also adding them to the request handler chain below
* */
const IntentReflectorHandler = {
canHandle(handlerInput) {
return Alexa.getRequestType(handlerInput.requestEnvelope) === 'IntentRequest';
},
handle(handlerInput) {
const intentName = Alexa.getIntentName(handlerInput.requestEnvelope);
const speakOutput = `You just triggered ${intentName}`;
return handlerInput.responseBuilder
.speak(speakOutput)
//.reprompt('add a reprompt if you want to keep the session open for the user to respond')
.getResponse();
}
};
/**
* Generic error handling to capture any syntax or routing errors. If you receive an error
* stating the request handler chain is not found, you have not implemented a handler for
* the intent being invoked or included it in the skill builder below
* */
const ErrorHandler = {
canHandle() {
return true;
},
handle(handlerInput, error) {
const speakOutput = 'Sorry, I had trouble doing what you asked. Please try again.';
console.log(`~~~~ Error handled: ${JSON.stringify(error)}`);
return handlerInput.responseBuilder
.speak(speakOutput)
.reprompt(speakOutput)
.getResponse();
}
};
/**
* This handler acts as the entry point for your skill, routing all request and response
* payloads to the handlers above. Make sure any new handlers or interceptors you've
* defined are included below. The order matters - they're processed top to bottom
* */
exports.handler = Alexa.SkillBuilders.custom()
.addRequestHandlers(
LaunchRequestHandler,
HelloWorldIntentHandler,
HelpIntentHandler,
CancelAndStopIntentHandler,
FallbackIntentHandler,
SessionEndedRequestHandler,
IntentReflectorHandler)
.addErrorHandlers(
ErrorHandler)
.withCustomUserAgent('sample/hello-world/v1.2')
.lambda();
工夫ポイント
JSONで作成したリストから今日の日付を検索するようにしました
//今日の名前を取得 xxの日
let allDate = require("./date.json");
let dateData = allDate.dateData.find((v) => v.date == today);
let dateName = dateData.name;
let dateMeans = dateData.means;
JSON
{
"dateData":
[
{
"date": "1-1",
"name": "鉄腕アトムの日",
"means": "鉄腕アトムの日は、1963年、(昭和38年)1月1日にテレビアニメ、「鉄腕アトム」の放映が開始された日を記念して制定された記念日です。"
},
{
"date": "1-2",
"name": "ロケットの日",
"means": "この記念日は、1959年、(昭和34年)1月2日に当時のソビエト連邦が世界初の月ロケット、「ルナ、(ルーニク)1号」の打ち上げに成功したことを記念して制定された記念日です。、「ルナ1号」は月に衝突させる予定でしたが、月に衝突することなく太陽の周回軌道に入り、期せずして世界初の人工衛星となりました。"
},
・
・ (割愛)
・
{
"date": "12-31",
"name": "大晦日",
"means": "大晦日とは、長かった一年が終わり、一年の締めくくりとして年越しそばを食べる日です。年越しそばは江戸中期からの習慣で、金箔職人が飛び散った金箔を練ったそば粉の固まりに引付けて集めていたため、年越しそばを残すと翌年は金運に恵まれないといいます。この日のそばは、来る年の金運がかかっているというわけです。また、金は鉄のように錆びたりせず、永遠に不変の物であることから、長寿への願いも込められているのです。"
}
]
}
この記事が参加している募集
この記事が気に入ったらサポートをしてみませんか?