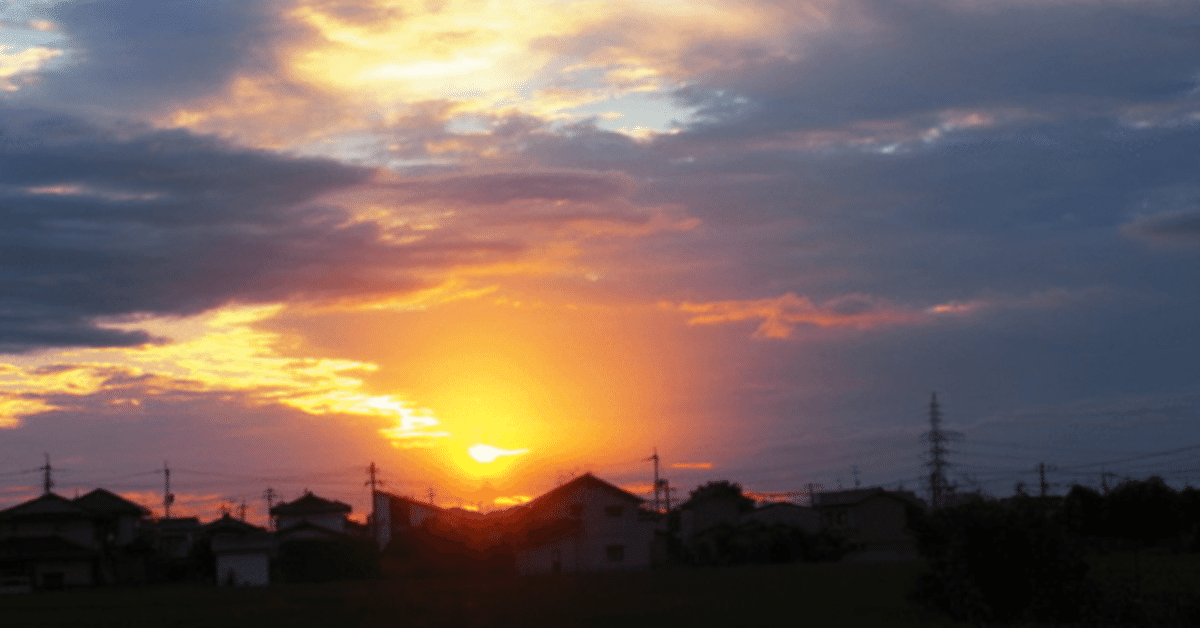
Photo by
hanasfather
基本情報技術者試験の科目BをJavaとPythonのプログラムで考える No.31
基本情報技術者試験で出題されるプログラム言語が疑似言語に変更されましたね。合格に向けて学習を進めるにおいては、やはり普段から使っているプログラム言語に置き換えて考えてみたいですよね。
このサイトでは、IPA情報処理推進機構で公開されている科目Bのプログラミングの問題を中心に、疑似言語をJavaとPythonのコードに置き換えて解説していきます。
1日1問の解説を発信していく予定です。基本情報技術者試験の合格を目指されている方のお役に立てれば幸いです。
基本情報技術者試験 科目 B サンプル問題(2022年4月公開)
https://www.ipa.go.jp/shiken/syllabus/ps6vr7000000oett-att/fe_kamoku_b_sample.pdf
問5 文字列の扱い
まずは問題の意味を読解しましょう。
下図のように4つの英単語があるとする。
任意の異なる 2 文字 “n” と “f” に着目して、次の割合をもとめる。
“n” の次に “f” が続く箇所の数 ÷ 末尾以外の “n” の出現回数
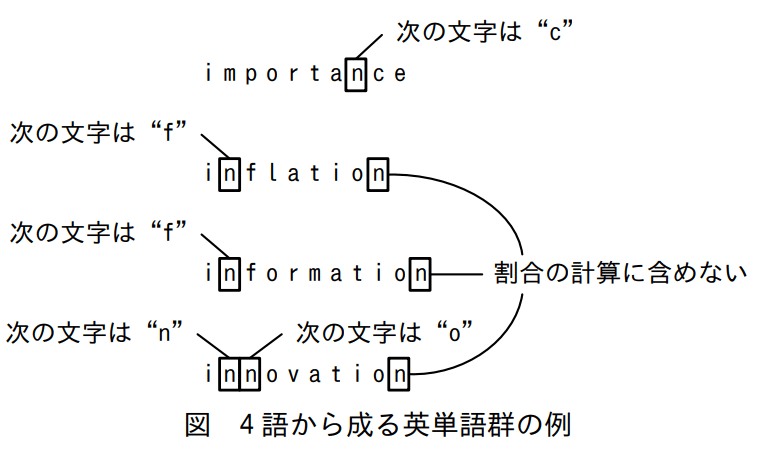
“n” の次に “f” が続く箇所の数 2か所
末尾以外の “n” の出現回数 5
よって、2 ÷ 5 =0.4 となる。
1.Javaのコードで考えてみよう
package kamokuB;
class Words{
private String[] englishWords;
Words(String[] englishWords){
this.englishWords = englishWords;
}
// 配列englishWordsの中で、各要素に含まれるstrの総出現回数をカウントするメソッド
public int freq(String str) {
int count = 0;
for (String word : englishWords) {
int index = 0;
while ((index = word.indexOf(str, index)) != -1) {
count++;
index += str.length();
}
}
return count;
}
// 配列englishWordsの各要素がstrで終わる場合、その数を返すメソッド
public int freqE(String str) {
int count = 0;
for (String word : englishWords) {
if (word.endsWith(str)) {
count++;
}
}
return count;
}
}
public class R3_Sample05 {
static Words words;
// c1の次にc2が出現する割合を返す
static double prob(char c1, char c2){
String s1 = Character.toString(c1);
String s2 = Character.toString(c2);
if (words.freq(s1 + s2) > 0)
// 実数で計算するため、double型にキャスト
return (double)words.freq(s1 + s2) / (words.freq(s1) - words.freqE(s1));
else
return 0;
}
public static void main(String[] args) {
words = new Words(new String[]{"importance","inflation","information","innovation"});
System.out.println(prob('n','f'));
}
}
2.Pythonのコードで考えてみよう
# 2022年4月公開問題5
class Words:
def __init__(self, english_words):
self.english_words = english_words
# 各要素に含まれるstrの総出現回数をカウントするメソッド
def freq(self, string):
count = 0
for word in self.english_words:
count += word.count(string)
return count
# 各要素がstrで終わる場合、その数を返すメソッド
def freqE(self, string):
count = 0
for word in self.english_words:
if word.endswith(string):
count += 1
return count
def prob(c1, c2):
s1 = str(c1)
s2 = str(c2)
if words.freq(s1 + s2) > 0:
return words.freq(s1 + s2) / (words.freq(s1) - words.freqE(s1))
else:
return 0.0
words = Words(["importance", "inflation", "information", "innovation"])
print(prob('n', 'f'))
いかがでしょうか。
用意されているクラス Words の2つのメソッド(freq()、freqE()) の
機能、引数、戻り値を正確に把握することが大事ですね。
この記事が気に入ったらサポートをしてみませんか?