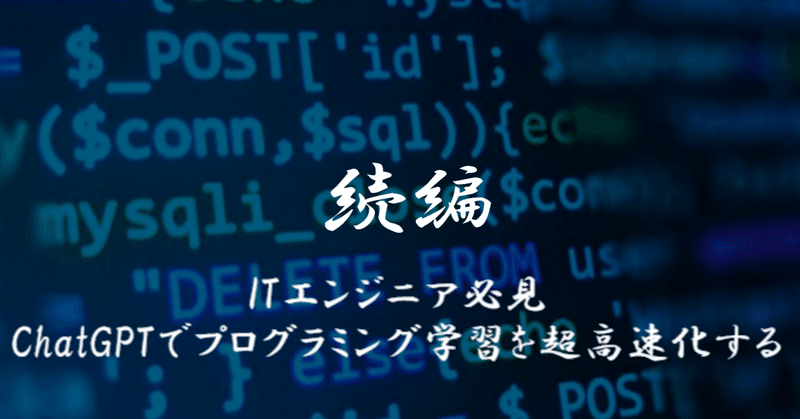
【続編】ITエンジニア必見 - ChatGPTでプログラミングを超高速化する
前回、ChatGPTがプログラミングにおいて強力な相棒になってくれることを紹介した。他にも便利な使い方が多数あるのでここで紹介しておく。
自分が書いたコードのUnit Test を作ってくれる
ITエンジニアにとってUnit Testを書くのは比較的面倒で、楽しくない作業ではないだろうか。ChatGPTを使うことでUnit Testの作成を大幅に効率化できる可能性がある。
具体的にはChatGPTにUnit Testを作って欲しい”ソースコードを張り付ける”、もしくは”コードが存在するurlを張り付ける”。そのうえで、 "Please create unit test"と書いてみて頂きたい。(2023/03/38時点では日本語でお願いするとUnit Testの一部しか作ってくれなかったので英語でChatGPTにお願いした方がよいかもしれない)
すると、自分が作成したコードのUnit Test を以下のように自動的に作ってくれるのだ。ものによってエラー系もちゃんと作ってくれる。逆にパッケージの依存関係などで、うまくUnit Testを作成できなこともあったが、面倒なUnit Testを作る工数を大幅に削減できる可能性があるのは間違いない。
Open AIのコードを使ってUnit Testの自動作成を試してみた結果
https://github.com/openai/openai-python/blob/main/openai/util.py
import unittest
from openai.util import *
class TestOpenAIUtil(unittest.TestCase):def test_parse_duration(self):
self.assertEqual(parse_duration("1d"), 86400)
self.assertEqual(parse_duration("2h"), 7200)
self.assertEqual(parse_duration("30m"), 1800)
self.assertEqual(parse_duration("60s"), 60)
self.assertEqual(parse_duration("1y"), 31536000)
self.assertEqual(parse_duration("6mo"), 15552000)
self.assertEqual(parse_duration("1w"), 604800)
self.assertRaises(ValueError, parse_duration, "2x")
def test_find_first_match(self):
self.assertEqual(find_first_match(['a', 'b', 'c'], ['d', 'e', 'a', 'f']), 2)
self.assertEqual(find_first_match(['a', 'b', 'c'], ['d', 'e', 'f']), None)
self.assertEqual(find_first_match(['a', 'b', 'c'], ['d', 'e', 'c', 'f']), 2)
self.assertEqual(find_first_match([], ['a', 'b', 'c']), None)
self.assertEqual(find_first_match(['a', 'b', 'c'], []), None)
def test_levenshtein_distance(self):
self.assertEqual(levenshtein_distance("kitten", "sitting"), 3)
self.assertEqual(levenshtein_distance("rosettacode", "raisethysword"), 8)
self.assertEqual(levenshtein_distance("abcdef", "abcdef"), 0)
self.assertEqual(levenshtein_distance("", "hello"), 5)
self.assertEqual(levenshtein_distance("world", ""), 5)
def test_limit_threads(self):
self.assertEqual(limit_threads(5), 5)
self.assertEqual(limit_threads(None), None)
self.assertEqual(limit_threads(-1), None)
if name == 'main':
unittest.main()
These tests cover the three functions in openai/util.py: parse_duration(), find_first_match(), levenshtein_distance(), and limit_threads(). The tests should give you confidence that the functions work correctly and as intended.
コードレビューをやってくれる
おそらくGitでMain/Masterレポジトリにマージする際、レビューを必須にしている方も多いだろう。なんと、あなたが書いたコードをChatGPTがレビューしてくれる。しかも適切な回答をくれるので是非とも導入したい機能だ。
例えば以下のようにChatGPTにレビューをお願いしてみる。
以下のコードのレビューをしてください。
string = input()
try:
num = int(input())
print(string+num)
except (TypeError, ValueError) as e:
print(e)
レビュー結果 by Chat GPT
このコードのレビューを行います。
1行目のinput()で、ユーザーに入力を促すメッセージが表示されていないため、ユーザーが何を入力すべきか分かりません。適切なメッセージを表示するようにしましょう。
3行目でinput()を呼び出していますが、これは数字の入力を促しているので、変数名をnum_inputなど、適切なものに変更するとよいでしょう。
4行目でstring+numという演算を行っていますが、stringが文字列型で、numが整数型なので、型エラーが発生します。ここでの目的が、stringとnumを連結させることである場合、numを文字列型に変換する必要があります。str(num)などを使って、適切に変換しましょう。
最後に、例外処理でTypeErrorとValueErrorの両方を捕捉していますが、どちらか一方で十分である場合、不要な例外処理を行わないようにすると、コードの効率性が向上します。
修正したコードは以下のようになります。
string = input("文字列を入力してください: ")
try:
num_input = int(input("整数を入力してください: "))
num_str = str(num_input)
print(string + num_str)
except ValueError as e:
print(e)
以上の点を考慮し、コードを修正するとより安全で効率的なコードとなるでしょう。
IaC(infrastructure as code)のコードも自動生成 - Teraform
クラウド開発では所謂、インフラ構築の自動化IaC(infrastructure as code)を導入しているプロジェクトも多いのではないだろうか?IaCはビジネスロジックの入らない比較的シンプルなコードになるが、細かいオプションなどが多いので書き方などを調べるのはなかなか骨が折れる。なんとChatGPTはオプション込みでIaCを自動生成してくれるのだ。
例えば以下のようにお願いしてみるとセットアップ方法も含めてコードを出力してくれる。便利!
TeraformでAWS S3を作りたい
出力結果 by Chat GPT
Terraformを使用してAWS S3を作成する手順を以下に示します。
AWSアカウントのセットアップ Terraformを使用する前に、AWSアカウントを作成し、認証情報を取得する必要があります。
Terraformのインストール Terraformをインストールするには、公式ウェブサイトからダウンロードしてインストールするか、パッケージ管理システムを使用することができます。
AWSプロバイダの設定 Terraformを使用してAWSリソースを管理するためには、AWSプロバイダを設定する必要があります。AWSプロバイダを設定するには、AWSの認証情報を提供する必要があります。
S3バケットの作成 AWS S3バケットを作成するために、Terraformのコードを記述します。以下は、S3バケットを作成するためのサンプルコードです。
provider "aws" {
region = "us-west-2"
}
resource "aws_s3_bucket" "my_bucket" {
bucket = "my-bucket"
acl = "private"
tags = {
Name = "my-bucket"
Environment = "dev"
}
}
IaCのコードも自動生成 - Bicep
TeraformだけでなくMicrosoft Azureで提供されているBicepなども、もちろん利用できる。もちろん前述した通り、リージョンなどのオプションも指定可能である。
Bicepで西日本にAzure Storageを作りたい
出力結果 by Chat GPT
以下は、Bicep を使用して Azure Storage を西日本に作成する方法の例です。
1.最初に、Azure CLI をインストールし、ログインします。
2.Bicep ファイルを作成します。例えば、"storageaccount.bicep"という名前のファイルを作成します。
3.以下のように、Bicep ファイルに Storage Account の定義を追加します。
resource storageAccount '<resource-group-name>/Microsoft.Storage/storageAccounts@2021-04-01' = {
name: '<storage-account-name>'
location: 'japaneast'
kind: 'StorageV2'
sku: {
name: 'Standard_LRS'
}
tags: {
environment: 'dev'
}
}
4.上記の定義で使用されるパラメータを説明します。
resource-group-name: ストレージ アカウントを作成するリソース グループの名前を指定します。
storage-account-name: ストレージ アカウントの名前を指定します。
location: ストレージ アカウントを作成する地域を指定します。この例では、japaneast を指定しています。
kind: ストレージ アカウントの種類を指定します。StorageV2 を指定することをお勧めします。
sku: ストレージ アカウントの SKU を指定します。この例では、Standard_LRS を指定しています。
tags: ストレージ アカウントに適用するタグを指定します。
5.以下のコマンドを使用して、Bicep ファイルをデプロイします。
az deployment group create --resource-group <resource-group-name> --template-file storageaccount.bicep
これで、Bicep を使用して Azure Storage Account を西日本に作成できます。
Stack Overflowで調べる必要がない。
実装していると何かしらのエラーが出る。そして原因、解決策を特定するのにすごく時間がかかった、1日潰した、なんて経験のあるエンジニアも多いのではないだろうか。その時、Stackover flowで同様の問題がないのか、解決策がないのか調べたことがあるITエンジニアも多いだろう。それでも求めてる回答がなかなかみつからず時間を消費してしまう、なんてこともあるだろう。こんなときChatGPTが便利だ。例えばStack Overflowの以下のQ&Aをサンプルとして使ってみる。(ちなみに、私は英語で試してみたが、みなさんには日本語なども試して結果をコメント欄に共有して頂きたい。)
ChatGPTへの質問(Stack Overflowの質問をそのまま張り付けただけ)
What are Null Pointer Exceptions (java.lang.NullPointerException) and what causes them?
What methods/tools can be used to determine the cause so that you stop the exception from causing the program to terminate prematurely?
出力結果 by ChatGPT
"A Null Pointer Exception (NPE) is a runtime error in Java that occurs when a program tries to use a null object reference. In other words, the program is trying to access an object, but the object doesn't exist. This can happen when a variable is not initialized or when it is assigned a null value. For example, consider the following Java code:
csharpCopy codeString name = null;
System.out.println(name.length());
In this code, the variable name is assigned a null value, so when we try to access its length() method, a Null Pointer Exception will be thrown. To prevent these exceptions from causing a program to terminate prematurely, you can use several methods and tools. One approach is to use defensive programming techniques such as checking if an object is null before trying to use it. For example:
csharpCopy codeif (name != null) {
System.out.println(name.length());
}
Another approach is to use an IDE (Integrated Development Environment) like Eclipse or IntelliJ IDEA that has built-in tools for detecting and preventing Null Pointer Exceptions. These tools can analyze your code and highlight potential NPEs before you run the program. Additionally, Java provides several tools for debugging and troubleshooting runtime errors, including the use of a debugger to step through the code and identify where the NPE is occurring. Stack traces can also be helpful in pinpointing the exact line of code that is causing the exception. Finally, logging tools like log4j or java.util.logging can be used to log error messages and provide information about the state of the program at the time of the exception."
注意点
ChatGPTはITエンジニアの良き相棒になれることを紹介したが、パブリックに公開されているChatGPTに機密情報を入れるのは避けた方がよい。なぜならそのデータはOpen AIの学習に使われたり、最悪のケースでは他のユーザーへの回答に使われる可能性もあるからだ。企業内で使うのであれば有償のAzure OpenAI Serviceなどを検討してみると良いだろう。
この記事が気に入ったらサポートをしてみませんか?