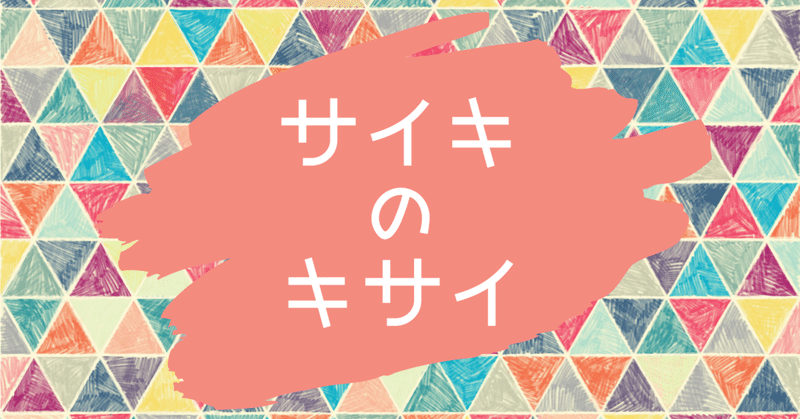
生き物感じる、サイキのキサイ。 《鳥の羽根編》
こちら に下のコードを貼り付けて実行しよう!
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
void func1(){
for (int i = 0; i < 200; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
stroke(255,255,255);
point( width*0.5+(x1=_x1)*40, height*0.5-(y1=_y1)*40 );
}
}
実行は画面上の『▶︎』ですが,実行前に,
右側の『Mode』を「Pjs」に変更してください.
なお,一見,再帰になっていないように見えますが,
次のように記載すると再帰であることが分かります.
(変更したのは draw と recursive だけです.同じ形になります)
void setup() {
size(720, 720);
background(0);
}
void draw() {
recursive(millis()/(1000*frameRate()));
}
void recursive(int n) {
func1();
if( n > 0 ){
recursive(n-1);
}
}
float x1=1.0, y1=0.0;
void func1(){
for (int i = 0; i < 200; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
stroke(255,255,255);
point( width*0.5+(x1=_x1)*40, height*0.5-(y1=_y1)*40 );
}
}
これにもっとアート要素を追加してみようと思います.
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
void func1(){
for (int i = 0; i < 3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
fill(random(0,255),random(0,255),random(0,255));
ellipse( width*0.5+(x1=_x1)*40, height*0.5-(y1=_y1)*40, 8, 8);
}
}
色を揃えてみると...
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
void func1(){
if( random(0,30) < 1 ){
fill(random(0,255),random(0,255),random(0,255));
}
for (int i = 0; i < 3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
ellipse( width*0.5+(x1=_x1)*40, height*0.5-(y1=_y1)*40, 8, 8);
}
}
円をもっと大量に出すと...
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
void func1(){
if( random(0,30) < 1 ){
fill(random(0,255),random(0,255),random(0,255));
}
for (int i = 0; i < 100; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
ellipse( width*0.5+(x1=_x1)*40, height*0.5-(y1=_y1)*40, 16, 16);
}
}
線に変えてみると...
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
void func1(){
if( random(0,30) < 1 ){
stroke(random(0,255),random(0,255),random(0,255));
}
for (int i = 0; i < 3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
line( width *0.5 + x1 *40, height*0.5 - y1 *40,
width *0.5 + (x1=_x1)*40, height*0.5 - (y1=_y1)*40 );
}
if( random(0,100) <= 1 ){
setup();
}
}
線と円を混ぜてみると...
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
void func1(){
if( random(0,30) < 1 ){
stroke(random(0,255),random(0,255),random(0,255));
}
for (int i = 0; i < 3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
line ( width *0.5 + x1*40, height*0.5 - y1*40,
width *0.5 +_x1*40, height*0.5 -_y1*40 );
ellipse( width *0.5 +_x1*40, height*0.5 -_y1*40, 10, 10);
x1=_x1;
y1=_y1;
}
if( random(0,100) <= 1 ){
setup();
}
}
3つの点のうち,2つだけを結ぶと...
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
float r = random(0,255),g = random(0,255),b = random(0,255);
void func1(){
if( random(0,30) < 1 ){
stroke(r = random(0,255),g = random(0,255),b = random(0,255));
}else{
stroke(r,g,b);
}
for (int i = 0; i <3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
line( width*0.5 + x1*40, height*0.5 - y1*40,
width*0.5 +_x1*40, height*0.5 -_y1*40 );
noStroke();
if( i == 2 ){
fill(r,g,b);
ellipse( width*0.5 + x1*40, height*0.5 - y1*40, 8, 8);
}
x1=_x1;
y1=_y1;
}
if( random(0,100) <= 1 ){
setup();
}
}
単純に線だけでも...
void setup() {
size(720, 720);
background(0);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
void func1(){
stroke(random(0,255),random(0,255),random(0,255));
for (int i = 0; i <3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
line( width*0.5 + x1 *40, height*0.5 - y1 *40,
width*0.5 +(x1=_x1)*40, height*0.5 -(y1=_y1)*40 );
noStroke();
}
if( random(0,100) <= 1 ){
setup();
}
}
グラデーションをつけて...
void setup() {
size(720, 720);
background(0);
frameRate(30);
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
int r = 200;
void func1(){
r = (2*abs((millis()/10)%200-100));
stroke(r,r,r);
for (int i = 0; i <3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
line( width*0.5 + x1 *40, height*0.5 - y1 *40,
width*0.5 +(x1=_x1)*40, height*0.5 -(y1=_y1)*40 );
noStroke();
}
if( random(0,150) <= 1 ){
setup();
}
}
少しだけ色を加えると...
void setup() {
size(720, 720);
background(0);
frameRate(30);
maxl = 0;
}
void draw() {
func1();
}
float x1=1.0, y1=0.0;
float l, maxl;
int r = 200;
void func1(){
r = (2*abs((millis()/10)%200-100));
stroke(r,r,r);
for (int i = 0; i <3; i++) {
float _x1 = y1 - 0.97*x1 + 4.0/(1+x1*x1) - 3.0;
float _y1 = -0.98*x1;
l = sqrt((x1-_x1)*(x1-_x1)+(y1-_y1)*(y1-_y1));
if( ( i==0 ) && ( maxl < l ) ){
stroke(random(0,255),random(0,255),random(0,255));
maxl = l;
}
line( width*0.5 + x1 *40, height*0.5 - y1 *40,
width*0.5 +(x1=_x1)*40, height*0.5 -(y1=_y1)*40 );
noStroke();
}
if( random(0,150) <= 1 ){
setup();
}
}
ぜひオリジナルのアートを作って遊びましょう!
この記事が気に入ったらサポートをしてみませんか?