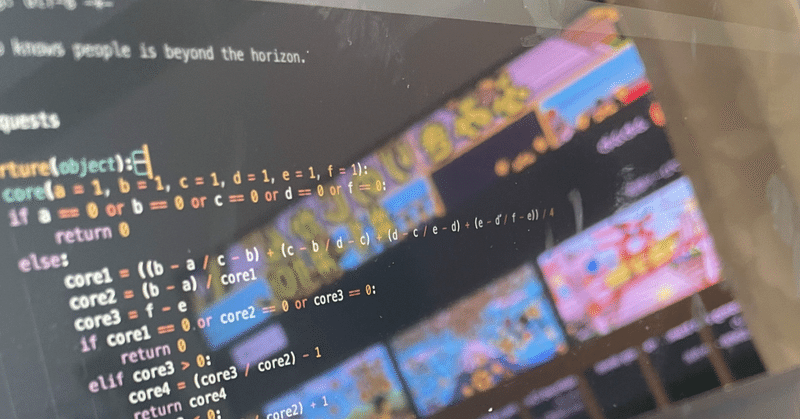
Photo by
mctmy339
Python Pro Bootcamp、27日目 Tkinterの基礎 + アーギュメントの使い方
今日はGUIで動くプログラムを作りたいと考え、Tkinterを使ったプログラムの基礎、およびアーギュメントの効率的な使い方を学習しました。Tkinterを使ったプログラムの作成は全3篇になっていますので、この調子で頑張りたいと思います。
Tkinterの基礎
#TkinterでGUIを用いたプログラムを作成する
import tkinter as tk
from tkinter import *
from PIL import Image, ImageTk
window = tk.Tk()
window.title("GUI Program")
window.minsize(width=500, height=300)
#GUIにラベルを表示する
text = "I am a Label"
my_label = tk.Label(window, text=text, font = ("Arial", 24, "bold"))
#my_label.pack(side="right")
my_label.pack()
#指定したラベルの内容を後から変更する
my_label["text"] = "New text"
my_label.config(text = "New text")
#ボタンをクリック、等々の際のイベントを記述する
def button_clicked():
#print("I got clicked!")
#ラベルに表示するテキストを変更する
#my_label.config(text="Button Got Clicked!")
#ラベルに表示する項目をエントリーからとってくる
new_text = input.get()
my_label.config(text = new_text)
#GUIにボタンを表示する
button = Button(text="Click Here:", command=button_clicked)
#button.pack(side="right")
button.pack()
#GUIにエントリー(文字を入力できる枠)を表示する
input = Entry(width = 10)
input.pack()
#GUIに画像を表示する
size_wh = 320
img = Image.open("FaceFahren.png")
img = img.resize((size_wh,size_wh))
my_icon = ImageTk.PhotoImage(img)
my_label02 = tk.Label(window, image = my_icon, bg="white", width=size_wh, height=size_wh)
my_label02.pack()
#メインループを実行しないとtkinterのウィンドウは表示されない
window.mainloop()
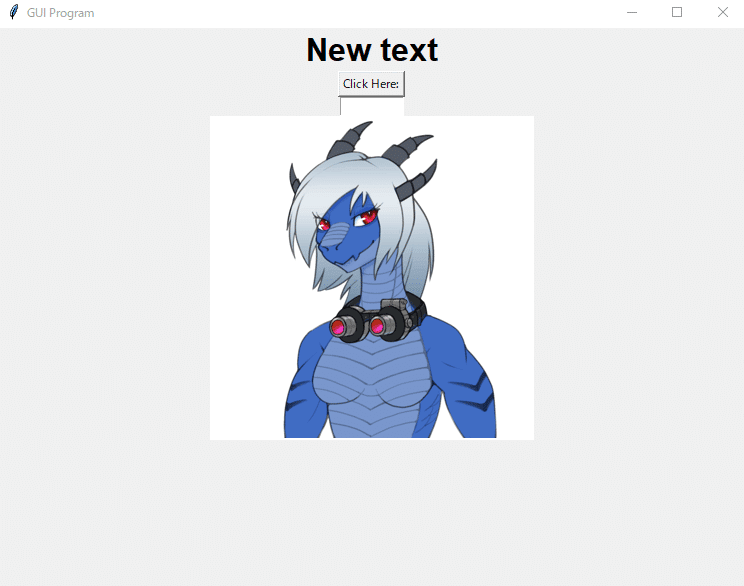
Tkinterチートシート集
from tkinter import *
#Creating a new window and configurations
window = Tk()
window.title("Widget Examples")
window.minsize(width=500, height=500)
#Labels
label = Label(text="This is old text")
label.config(text="This is new text")
label.pack()
#Buttons
def action():
print("Do something")
#calls action() when pressed
button = Button(text="Click Me", command=action)
button.pack()
#Entries
entry = Entry(width=30)
#Add some text to begin with
entry.insert(END, string="Some text to begin with.")
#Gets text in entry
print(entry.get())
entry.pack()
#Text
text = Text(height=5, width=30)
#Puts cursor in textbox.
text.focus()
#Adds some text to begin with.
text.insert(END, "Example of multi-line text entry.")
#Get's current value in textbox at line 1, character 0
print(text.get("1.0", END))
text.pack()
#Spinbox
def spinbox_used():
#gets the current value in spinbox.
print(spinbox.get())
spinbox = Spinbox(from_=0, to=10, width=5, command=spinbox_used)
spinbox.pack()
#Scale
#Called with current scale value.
def scale_used(value):
print(value)
scale = Scale(from_=0, to=100, command=scale_used)
scale.pack()
#Checkbutton
def checkbutton_used():
#Prints 1 if On button checked, otherwise 0.
print(checked_state.get())
#variable to hold on to checked state, 0 is off, 1 is on.
checked_state = IntVar()
checkbutton = Checkbutton(text="Is On?", variable=checked_state, command=checkbutton_used)
checked_state.get()
checkbutton.pack()
#Radiobutton
def radio_used():
print(radio_state.get())
#Variable to hold on to which radio button value is checked.
radio_state = IntVar()
radiobutton1 = Radiobutton(text="Option1", value=1, variable=radio_state, command=radio_used)
radiobutton2 = Radiobutton(text="Option2", value=2, variable=radio_state, command=radio_used)
radiobutton1.pack()
radiobutton2.pack()
#Listbox
def listbox_used(event):
# Gets current selection from listbox
print(listbox.get(listbox.curselection()))
listbox = Listbox(height=4)
fruits = ["Apple", "Pear", "Orange", "Banana"]
for item in fruits:
listbox.insert(fruits.index(item), item)
listbox.bind("<<ListboxSelect>>", listbox_used)
listbox.pack()
window.mainloop()
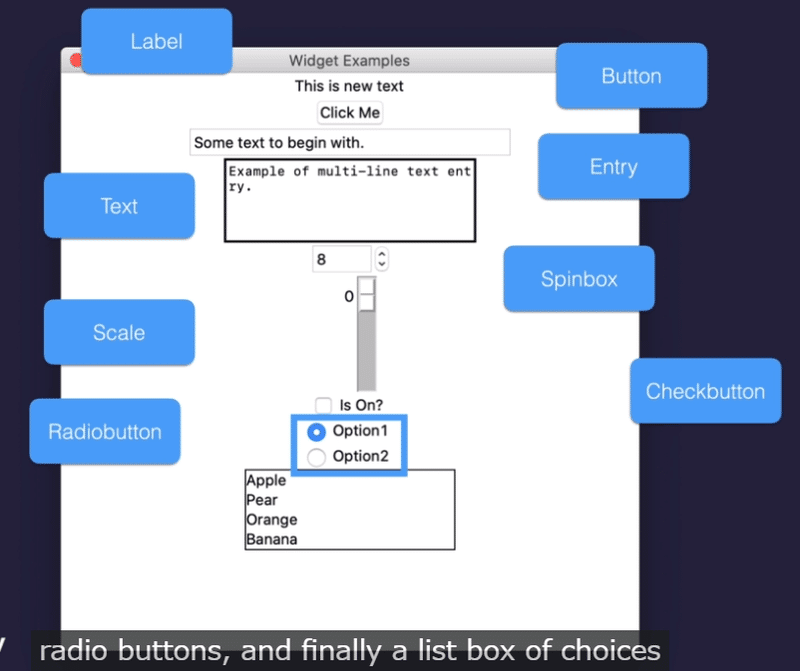
アーギュメントの使い方
#*argという引数を指定すれば、引数を無限に増やすことができる(引数をタプルとして扱う)
def add(*arg):
sum = 0
for n in args:
sum += n
return sum
print(add(3,5,6))
#**kwargsは引数をキーワードアーグメント=辞書の形で引数とする
def calculate(**kwargs):
print(kwargs)
#for key, value in kwargs.items():
# print(key)
# print(value)
#kwargがaddであれば加算を、multiplyであれば乗算を行う。ここがキーワードアーギュメントの便利な点
n += kwargs["add"]
n *= kwargs["multiply"]
calculate(add=3, multiply=5)
class Car:
def __init__(self, **kw):
#辞書型を直接参照するのではなく、Noneを返すことができるメソッドを使ってバグを回避する
#self.make = kw["make"]
#self.model = kw["model"]
self.make = kw.get("make")
self.model = kw.get("model")
my_car = Car(make="Nissan", model="GT-6")
print(my_car.model)
Tkinterを使ったプログラムについては、例のPythonでお題メーカーを作成してみたをGUI化したりなどもできたらいいなと思ってます。この調子で頑張りますね。
この記事が気に入ったらサポートをしてみませんか?