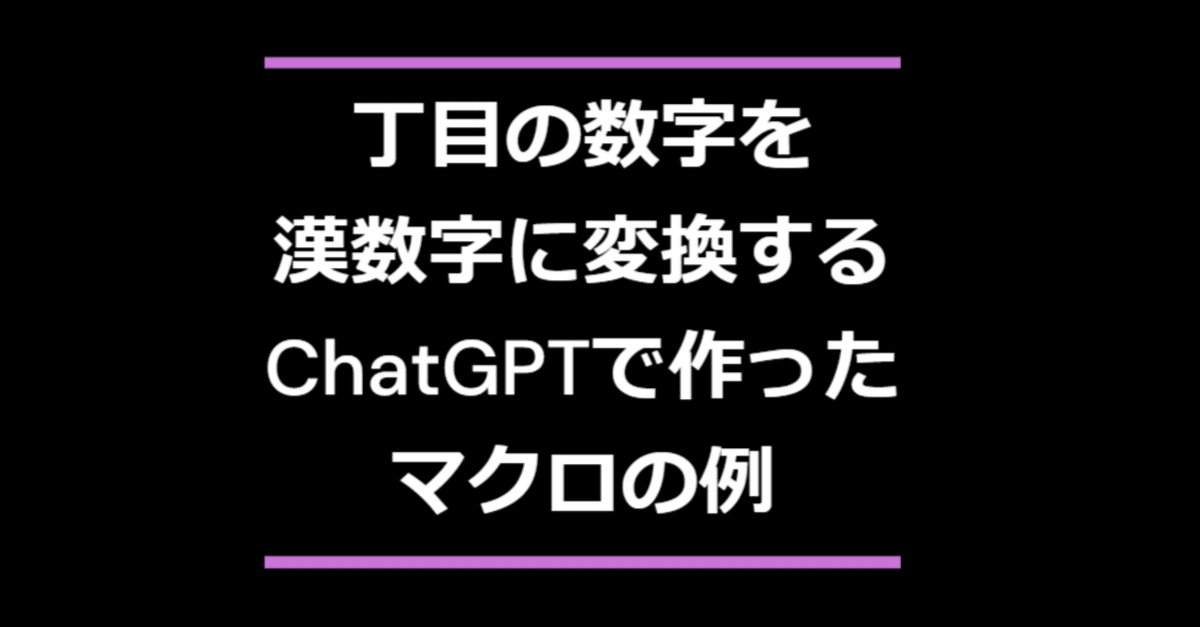
丁目の数値を漢数字にするよChatGPTで作ったマクロ
この説明は、ChatGPTで作成しています。
このマクロは、Excelのセル内にある「○丁目」という文字列を見つけて、その数値部分を漢数字に変換します。
仕組みの説明
変数の宣言:
rng、cell、regex、matches、match、text などの変数が宣言されています。これらはそれぞれセルの範囲や正規表現のマッチ結果などを保持します。
kanjiDigits と kanjiUnits という配列が宣言されています。これらは漢数字のディジット(例:一、二、三)と単位(例:十、百、千)を保持しています。
正規表現オブジェクトの作成:
regex オブジェクトが作成され、「\d+丁目」というパターンが設定されます。これは「1丁目」、「2丁目」などの形式を見つけるためのものです。
選択範囲の取得:
rng には、ユーザーが選択したセル範囲が設定されます。
選択範囲内の各セルをループ:
各セルの値を文字列として取得し、正規表現を使って「○丁目」の形式を探します。
数値を漢数字に変換:
マッチした部分(例:「1丁目」の「1」)を取り出し、数値に変換します。
その数値を漢数字に変換します。具体的には、各桁を対応する漢数字に変換し、十、百、千などの単位を適用します。
特殊な漢数字の規則(例:「一十」を「十」に変える)も適用します。
セルの値を更新:
漢数字に変換された「○丁目」を元のセルの値と置き換えます。
このマクロを実行すると、選択したセル範囲内の「○丁目」の数値部分が漢数字に変換されます。
Sub 丁目の数値を漢数字にするよChatGPTで作ったマクロ()
Dim rng As Range
Dim cell As Range
Dim regex As Object
Dim matches As Object
Dim match As Object
Dim text As String
' 漢数字のディジットと単位
Dim kanjiDigits As Variant
kanjiDigits = Array("", "一", "二", "三", "四", "五", "六", "七", "八", "九")
Dim kanjiUnits As Variant
kanjiUnits = Array("", "十", "百", "千")
' 正規表現オブジェクトを作成
Set regex = CreateObject("VBScript.RegExp")
regex.Global = True
regex.IgnoreCase = False
regex.Pattern = "\d+丁目"
' 選択範囲を取得
Set rng = Selection
' 選択範囲内の各セルをループ
For Each cell In rng
' セルの値を文字列として取得
text = cell.Value
' 正規表現でマッチを検索
Set matches = regex.Execute(text)
' マッチがある場合
If matches.Count > 0 Then
For Each match In matches
' 数値部分を抽出
Dim numStr As String
numStr = Left(match.Value, Len(match.Value) - 2) ' "丁目"を除去
' 数値を漢数字に変換
Dim num As Long
num = CLng(numStr)
Dim kanjiNum As String
kanjiNum = ""
Dim unitPos As Integer
unitPos = 0
Do While num > 0
Dim digit As Integer
digit = num Mod 10
If digit > 0 Then
If unitPos > 0 Then
kanjiNum = kanjiDigits(digit) & kanjiUnits(unitPos) & kanjiNum
Else
kanjiNum = kanjiDigits(digit) & kanjiNum
End If
ElseIf unitPos > 0 And kanjiNum <> "" Then
kanjiNum = kanjiUnits(unitPos) & kanjiNum
End If
num = num \ 10
unitPos = unitPos + 1
Loop
' 特殊な漢数字の規則を適用
If InStr(kanjiNum, "一十") = 1 Then
kanjiNum = Mid(kanjiNum, 2)
End If
' 漢数字に変換された文字列を置き換え
text = Replace(text, match.Value, kanjiNum & "丁目")
Next match
' セルの値を更新
cell.Value = text
End If
Next cell
End Sub
ハッシュタグ
#excel #できること #vba #丁目 #漢数字 #数値変換 #正規表現 #セル操作 #文字列処理 #プログラミング初心者 #VBA入門 #自動化 #オフィスワーク #マクロ作成 #ExcelTips #スプレッドシート #効率化 #データ処理 #Excelマクロ #業務改善
Translation to English
丁目の数値を漢数字にするよChatGPTで作ったマクロ
This explanation is created by ChatGPT.
This macro finds strings like "1丁目" (where "丁目" means district or block in Japanese addresses) in the cells of an Excel sheet and converts the numeric part to Kanji characters.
Explanation of the Mechanism
Variable Declaration:
Variables such as rng, cell, regex, matches, match, and text are declared. These hold the cell range, regex match results, and other necessary values.
Arrays kanjiDigits and kanjiUnits are declared to hold Kanji digits (e.g., 一, 二, 三) and units (e.g., 十, 百, 千).
Creating the Regular Expression Object:
A regex object is created with the pattern "\d+丁目". This pattern finds strings like "1丁目", "2丁目", etc.
Getting the Selected Range:
rng is set to the user-selected cell range.
Looping Through Each Cell in the Selected Range:
The value of each cell is obtained as a string and the regex is used to find matches for the "○丁目" format.
Converting Numbers to Kanji:
The numeric part of the match (e.g., the "1" in "1丁目") is extracted and converted to a number.
The number is then converted to Kanji characters by mapping each digit to the corresponding Kanji character and applying units like ten, hundred, thousand.
Special Kanji rules (e.g., converting "一十" to "十") are also applied.
Updating Cell Values:
The original "○丁目" in the cell is replaced with the Kanji version.
When this macro is run, the numeric parts of "○丁目" in the selected cell range are converted to Kanji characters.
Sub ConvertNumberToKanjiInChomeCreatedByChatGPT()
Dim rng As Range
Dim cell As Range
Dim regex As Object
Dim matches As Object
Dim match As Object
Dim text As String
' Kanji digits and units
Dim kanjiDigits As Variant
kanjiDigits = Array("", "一", "二", "三", "四", "五", "六", "七", "八", "九")
Dim kanjiUnits As Variant
kanjiUnits = Array("", "十", "百", "千")
' Create regex object
Set regex = CreateObject("VBScript.RegExp")
regex.Global = True
regex.IgnoreCase = False
regex.Pattern = "\d+丁目"
' Get selected range
Set rng = Selection
' Loop through each cell in the selected range
For Each cell In rng
' Get the cell value as a string
text = cell.Value
' Search for matches with regex
Set matches = regex.Execute(text)
' If there are matches
If matches.Count > 0 Then
For Each match In matches
' Extract the numeric part
Dim numStr As String
numStr = Left(match.Value, Len(match.Value) - 2) ' Remove "丁目"
' Convert the numeric part to Kanji
Dim num As Long
num = CLng(numStr)
Dim kanjiNum As String
kanjiNum = ""
Dim unitPos As Integer
unitPos = 0
Do While num > 0
Dim digit As Integer
digit = num Mod 10
If digit > 0 Then
If unitPos > 0 Then
kanjiNum = kanjiDigits(digit) & kanjiUnits(unitPos) & kanjiNum
Else
kanjiNum = kanjiDigits(digit) & kanjiNum
End If
ElseIf unitPos > 0 And kanjiNum <> "" Then
kanjiNum = kanjiUnits(unitPos) & kanjiNum
End If
num = num \ 10
unitPos = unitPos + 1
Loop
' Apply special Kanji rules
If InStr(kanjiNum, "一十") = 1 Then
kanjiNum = Mid(kanjiNum, 2)
End If
' Replace with the converted Kanji string
text = Replace(text, match.Value, kanjiNum & "丁目")
Next match
' Update the cell value
cell.Value = text
End If
Next cell
End Sub
Hashtags
#excel #whatyoucando #vba #chome #kanjinumbers #numberconversion #regex #celloperations #stringprocessing #beginnersprogramming #VBAintro #automation #officework #macroscreation #ExcelTips #spreadsheets #efficiency #dataprocessing #ExcelMacros #workimprovement