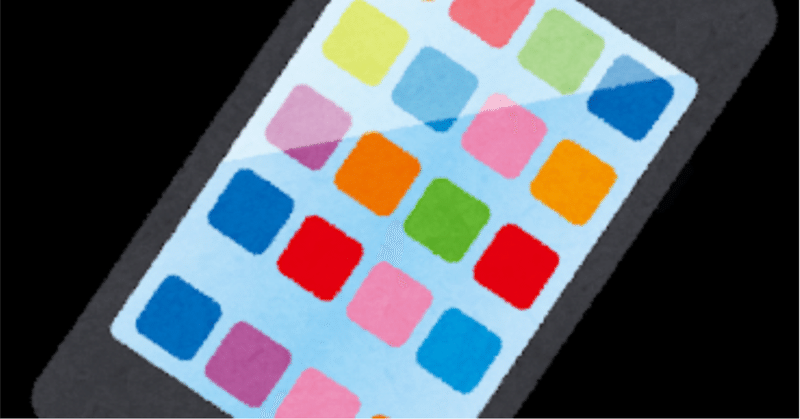
【徒然iOS】気ままにUIKit55〜Map Kit View 長押しした位置にピンを刺す〜
概要
このマガジンは四十を過ぎたおっさんが、
を参考にStoryboardでiOSアプリを完全に趣味で楽しんでいるだけな記事を気ままに上げてます。
今回
をハイ、レッツゴ🕺
前準備
念の為、
バックアップ
新しいクラス
ビューコントローラの追加
イニシャルビューの変更
をいつも通りやってから本題へ💃
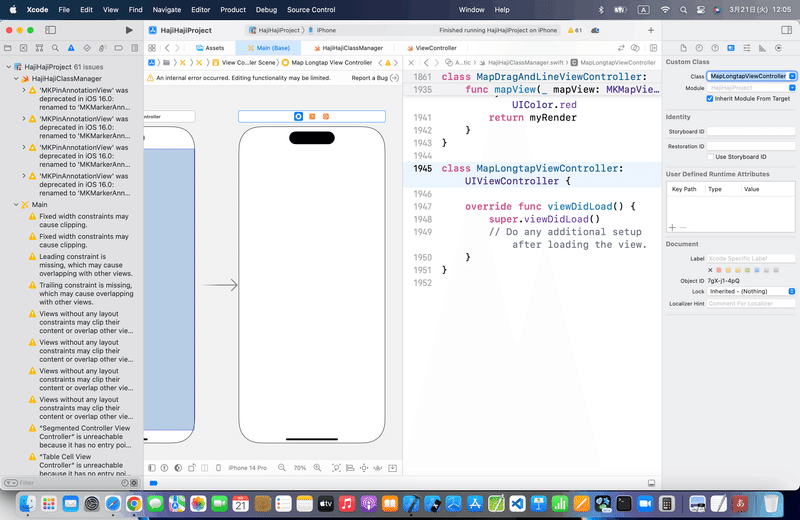
本題
⒈前回までと同様に、MAPKitを組み込んでアウトレット接続しとく
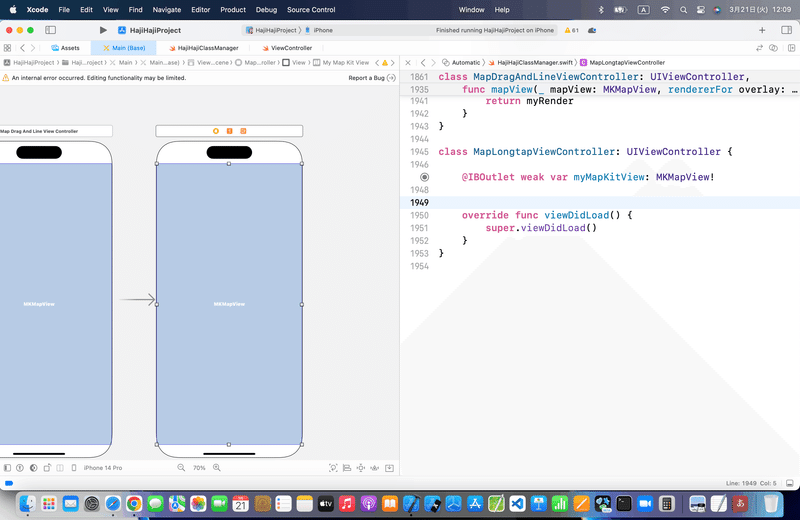
⒉ロングタップジェスチャを配置して、アクション接続
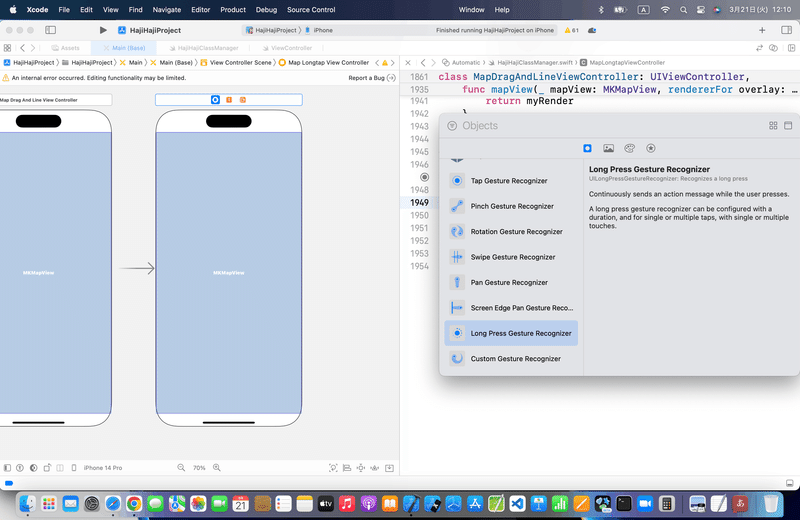
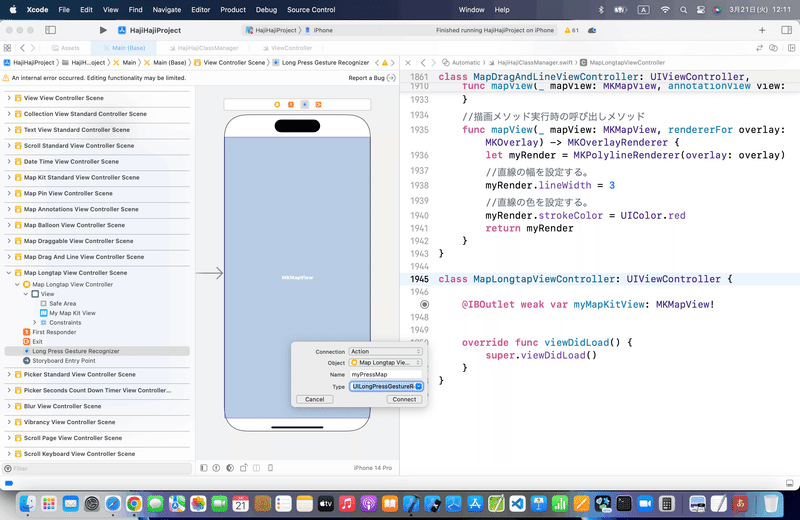
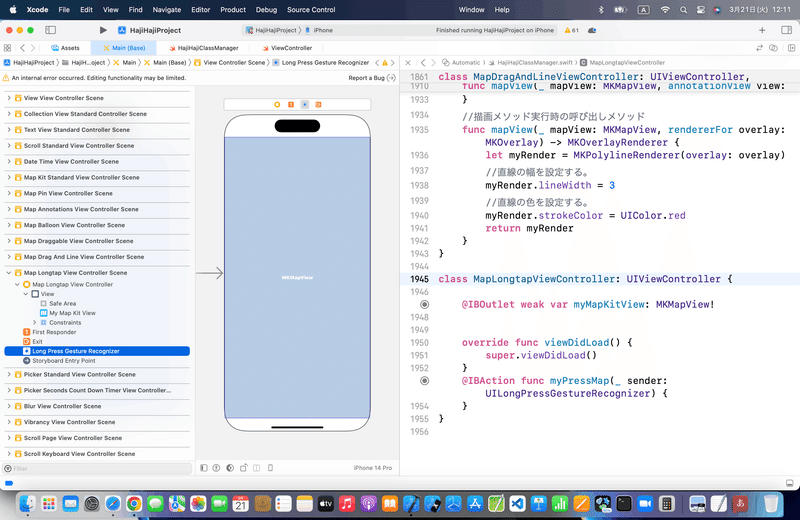
⒊以下のコードを組み込む〜〜〜
//
// ViewController.swift
//
import UIKit
import MapKit
class ViewController: UIViewController,MKMapViewDelegate {
@IBOutlet weak var testMapView: MKMapView!
//最初からあるメソッド
override func viewDidLoad() {
super.viewDidLoad()
//中心座標
let center = CLLocationCoordinate2DMake(35.0, 140.0)
//表示範囲
let span = MKCoordinateSpanMake(1.0, 1.0)
//中心座標と表示範囲をマップに登録する。
let region = MKCoordinateRegionMake(center, span)
testMapView.setRegion(region, animated:true)
//デリゲート先に自分を設定する。
testMapView.delegate = self
}
//マップビュー長押し時の呼び出しメソッド
@IBAction func pressMap(sender: UILongPressGestureRecognizer) {
//マップビュー内のタップした位置を取得する。
let location:CGPoint = sender.locationInView(testMapView)
if (sender.state == UIGestureRecognizerState.Ended){
//タップした位置を緯度、経度の座標に変換する。
let mapPoint:CLLocationCoordinate2D = testMapView.convertPoint(location, toCoordinateFromView: testMapView)
//ピンを作成してマップビューに登録する。
let annotation = MKPointAnnotation()
annotation.coordinate = CLLocationCoordinate2DMake(mapPoint.latitude, mapPoint.longitude)
annotation.title = "ピン"
annotation.subtitle = "\(annotation.coordinate.latitude), \(annotation.coordinate.longitude)"
testMapView.addAnnotation(annotation)
}
}
//アノテーションビューを返すメソッド
func mapView(mapView: MKMapView, viewForAnnotation annotation: MKAnnotation) -> MKAnnotationView? {
let testView = MKPinAnnotationView(annotation: annotation, reuseIdentifier: nil)
//吹き出しを表示可能にする。
testView.canShowCallout = true
return testView
}
}
を参考に〜〜〜
今回のコード(長押しのみ)
class MapLongtapViewController: UIViewController,MKMapViewDelegate {
@IBOutlet weak var myMapKitView: MKMapView!
let x = 139.692101
let y = 35.689634
let latitudeDelta = 10.0
let longitudeDelta = 10.0
//最初からあるメソッド
override func viewDidLoad() {
super.viewDidLoad()
//中心座標
let center = CLLocationCoordinate2DMake(y, x)
//表示範囲
let span = MKCoordinateSpan(latitudeDelta: latitudeDelta, longitudeDelta: longitudeDelta)
//中心座標と表示範囲をマップに登録する。
let region = MKCoordinateRegion(center: center, span: span)
myMapKitView.setRegion(region, animated:true)
//デリゲート先に自分を設定する。
myMapKitView.delegate = self
}
//マップビュー長押し時の呼び出しメソッド
@IBAction func myPressMap(_ sender: UILongPressGestureRecognizer) {
//マップビュー内のタップした位置を取得する。
let location:CGPoint = sender.location(in: myMapKitView)
if (sender.state == UIGestureRecognizer.State.ended){
//タップした位置を緯度、経度の座標に変換する。
let mapPoint:CLLocationCoordinate2D = myMapKitView.convert(location, toCoordinateFrom: myMapKitView)
//ピンを作成してマップビューに登録する。
let annotation = MKPointAnnotation()
annotation.coordinate = CLLocationCoordinate2DMake(mapPoint.latitude, mapPoint.longitude)
annotation.title = "ピン"
annotation.subtitle = "\(annotation.coordinate.latitude), \(annotation.coordinate.longitude)"
myMapKitView.addAnnotation(annotation)
}
}
//アノテーションビューを返すメソッド
func mapView(_ mapView: MKMapView, viewFor annotation: MKAnnotation) -> MKAnnotationView? {
let myView = MKPinAnnotationView(annotation: annotation, reuseIdentifier: nil)
//吹き出しを表示可能にする。
myView.canShowCallout = true
return myView
}
}
⒋シミュレータで実行
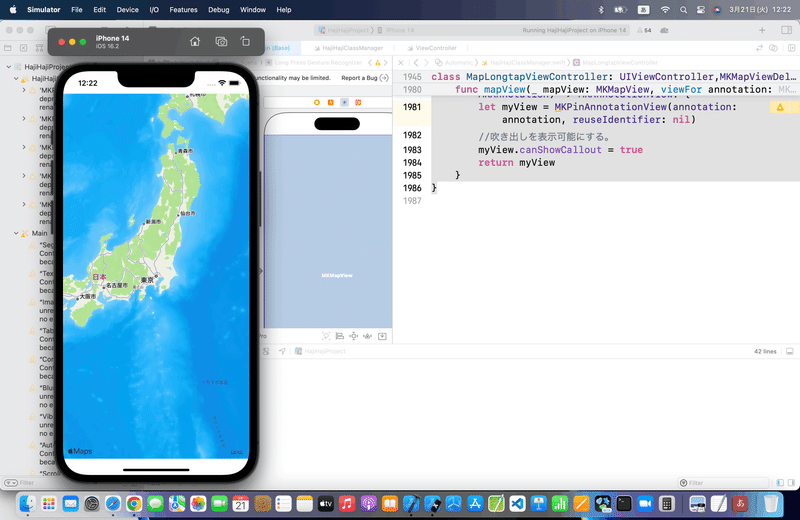
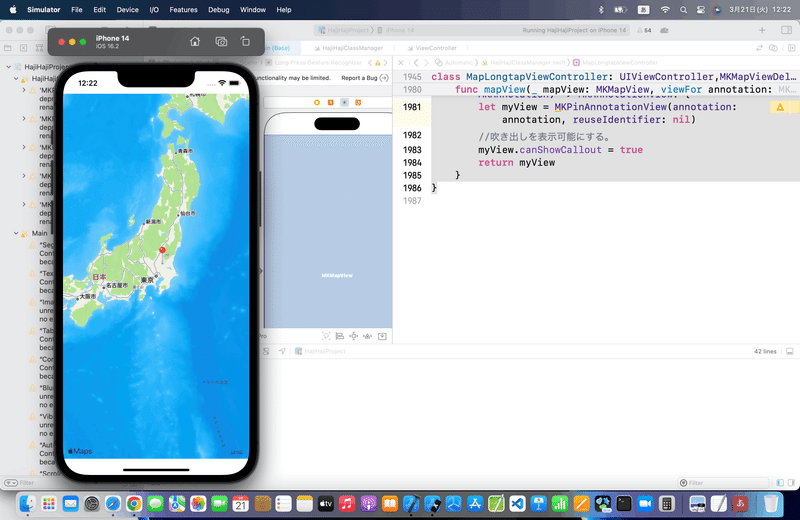
ハイ、出来上がり🕺
⒌ピンの場所を移動する
以下のコードを参考に〜〜〜
//
// ViewController.swift
//
import UIKit
import MapKit
class ViewController: UIViewController,MKMapViewDelegate {
@IBOutlet weak var testMapView: MKMapView!
let annotation = MKPointAnnotation()
//最初からあるメソッド
override func viewDidLoad() {
super.viewDidLoad()
//中心座標
let center = CLLocationCoordinate2DMake(35.0, 140.0)
//表示範囲
let span = MKCoordinateSpanMake(1.0, 1.0)
//中心座標と表示範囲をマップに登録する。
let region = MKCoordinateRegionMake(center, span)
testMapView.setRegion(region, animated:true)
//ピンを作成してマップビューに登録する。
annotation.coordinate = CLLocationCoordinate2DMake(35.0, 140.0)
annotation.title = "ピン"
annotation.subtitle = "\(annotation.coordinate.latitude), \(annotation.coordinate.longitude)"
testMapView.addAnnotation(annotation)
//デリゲート先に自分を設定する。
testMapView.delegate = self
}
//マップビュー長押し時の呼び出しメソッド
@IBAction func pressMap(sender: UILongPressGestureRecognizer) {
//マップビュー内のタップした位置を取得する。
let location:CGPoint = sender.locationInView(testMapView)
if (sender.state == UIGestureRecognizerState.Ended){
//タップした位置を緯度、経度の座標に変換する。
let mapPoint:CLLocationCoordinate2D = testMapView.convertPoint(location, toCoordinateFromView: testMapView)
//ピンの座標とサブタイトルを更新する。
annotation.coordinate = CLLocationCoordinate2DMake(mapPoint.latitude, mapPoint.longitude)
annotation.subtitle = "\(annotation.coordinate.latitude), \(annotation.coordinate.longitude)"
}
}
//アノテーションビューを返すメソッド
func mapView(mapView: MKMapView, viewForAnnotation annotation: MKAnnotation) -> MKAnnotationView? {
let testView = MKPinAnnotationView(annotation: annotation, reuseIdentifier: nil)
//吹き出しを表示可能にする。
testView.canShowCallout = true
return testView
}
}
コードを書き換え〜〜〜
今回のコード(長押し移動)
class MapLongtapViewController: UIViewController,MKMapViewDelegate {
@IBOutlet weak var myMapKitView: MKMapView!
let x = 139.692101
let y = 35.689634
let latitudeDelta = 10.0
let longitudeDelta = 10.0
let annotation = MKPointAnnotation()
//最初からあるメソッド
override func viewDidLoad() {
super.viewDidLoad()
//中心座標
let center = CLLocationCoordinate2DMake(y, x)
//表示範囲
let span = MKCoordinateSpan(latitudeDelta: latitudeDelta, longitudeDelta: longitudeDelta)
//中心座標と表示範囲をマップに登録する。
let region = MKCoordinateRegion(center: center, span: span)
myMapKitView.setRegion(region, animated:true)
//ピンを作成してマップビューに登録する。
annotation.coordinate = CLLocationCoordinate2DMake(y, x)
annotation.title = "ピン"
annotation.subtitle = "\(annotation.coordinate.latitude), \(annotation.coordinate.longitude)"
myMapKitView.addAnnotation(annotation)
//デリゲート先に自分を設定する。
myMapKitView.delegate = self
}
//マップビュー長押し時の呼び出しメソッド
@IBAction func myPressMap(_ sender: UILongPressGestureRecognizer) {
//マップビュー内のタップした位置を取得する。
let location:CGPoint = sender.location(in: myMapKitView)
if (sender.state == UIGestureRecognizer.State.ended){
//タップした位置を緯度、経度の座標に変換する。
let mapPoint:CLLocationCoordinate2D = myMapKitView.convert(location, toCoordinateFrom: myMapKitView)
//ピンの座標とサブタイトルを更新する。
annotation.coordinate = CLLocationCoordinate2DMake(mapPoint.latitude, mapPoint.longitude)
annotation.subtitle = "\(annotation.coordinate.latitude), \(annotation.coordinate.longitude)"
}
}
//アノテーションビューを返すメソッド
func mapView(_ mapView: MKMapView, viewFor annotation: MKAnnotation) -> MKAnnotationView? {
let myView = MKPinAnnotationView(annotation: annotation, reuseIdentifier: nil)
//吹き出しを表示可能にする。
myView.canShowCallout = true
return myView
}
}
実行結果
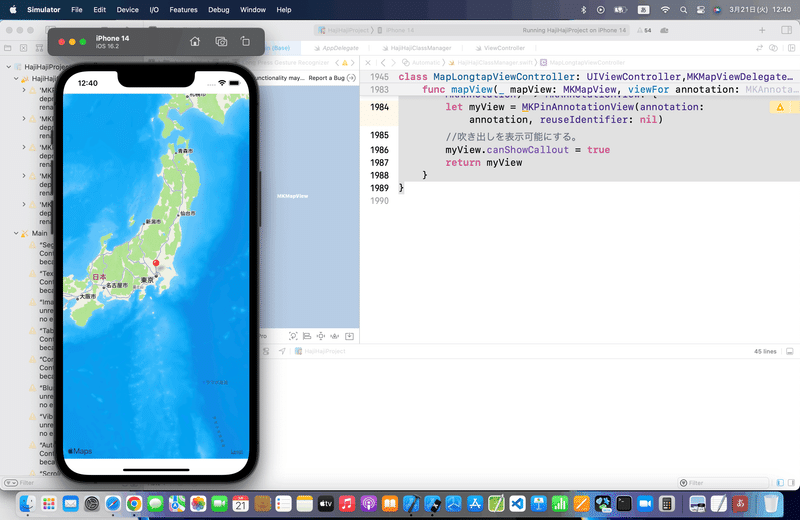
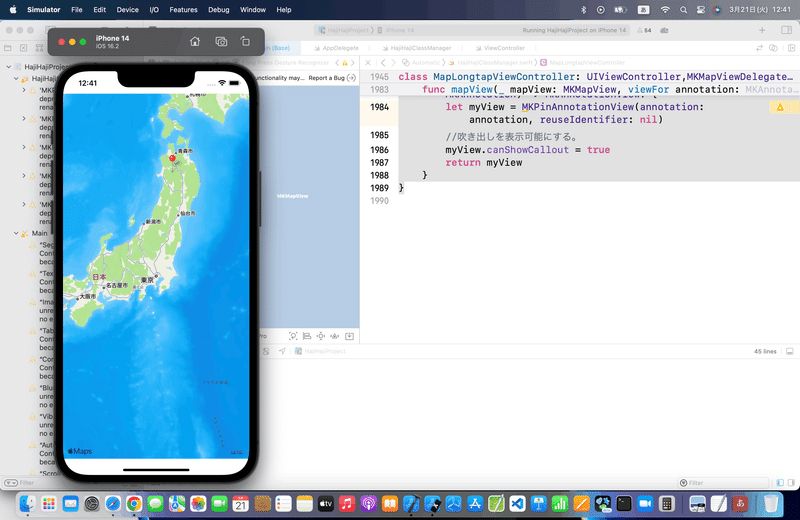
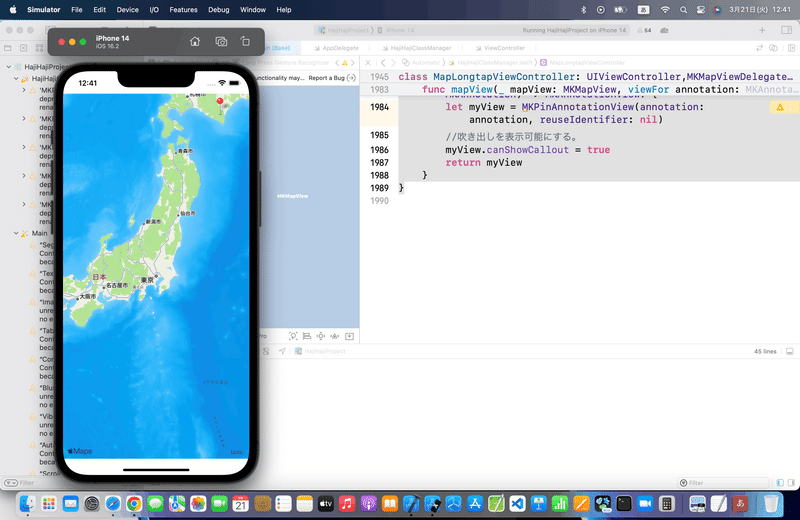
ハイ、出来た🕺
今回のポイント
これ実は、、、
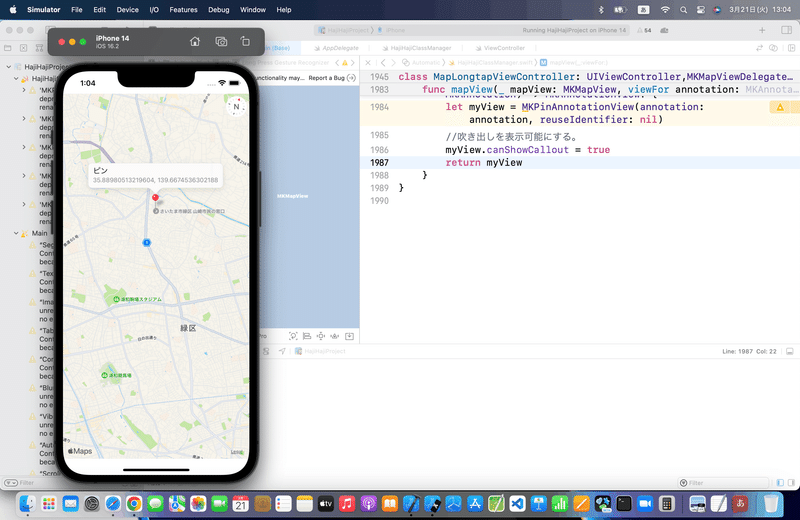
だからまあ、
「これでどんなアプリが作れるだろう」
を考えながらやると吸収も早いし。
標準機能を知らない人に、
「まず、何を作りたいかを決めるのがプログラミングの上達のコツ」
って言ってるのってナンセンスだって、他の記事で書いたんだよね〜〜〜〜🕺
まずは、たくさんの標準機能を知ること
Apple公式
さて、次回は
をレッツゴする🕺
この記事が気に入ったらサポートをしてみませんか?