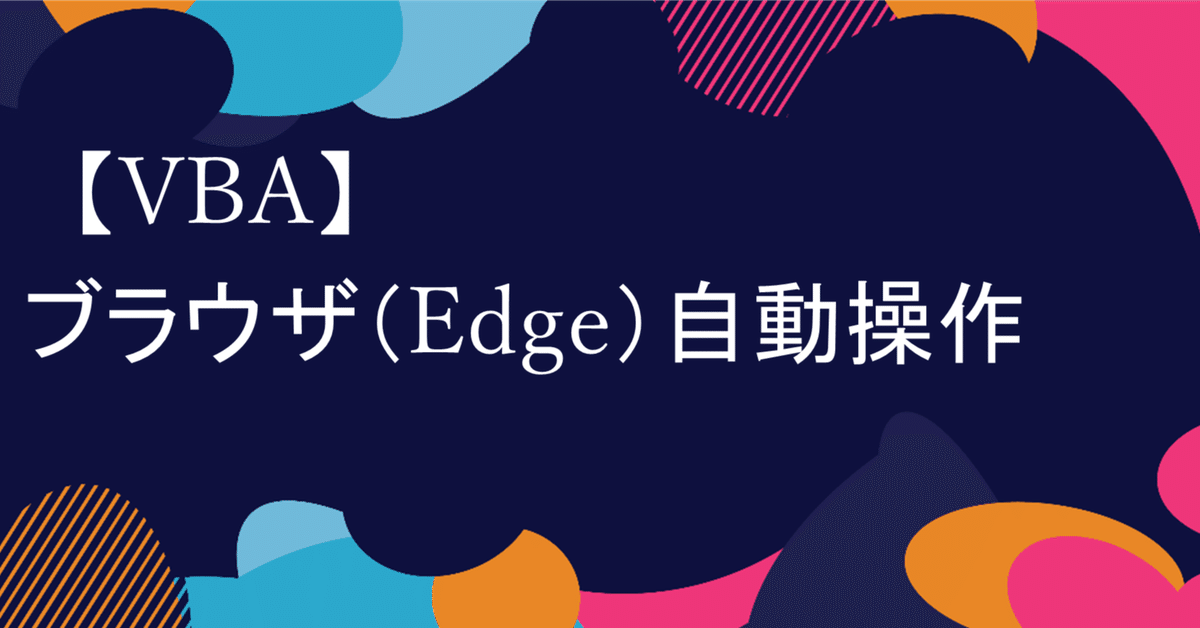
【VBA】Edge IEモードを自動操作(インストール不要)
Excel VBAで、ブラウザ(Microsott Edge)のIEモードを操作するコードのサンプルです。
追加インストールやダウンロード不要なので、何かとセキュリティポリシーが厳しい会社PC環境でも使えます。
※IEモードではなく標準モードを操作したいときはこちらの記事を参照。
全体像はこちら。(よく分からない人は読み飛ばしてOK)
【概要】
・WebDriverインストール不要(Seleniumを使わない)
・Win APIを使用。(Windows 64bit版)
・自動操作の流れ
①操作対象画面をブラウザのIEモードで開く。
②プロセス監視して、ブラウザが起動するまで処理待機。
③ウィンドウキャプション名を監視して、ページが読み込まれるまで処理待機。
④操作対象画面をDOM化(IHTMLDocument2オブジェクトを生成)
⑤操作対象画面を自動操作(DOM操作)
【動作確認済み環境】
・Windows Server 2016 Standard (64bit)、Excel® for Microsoft 365 (64bit)
・Windows 10 Pro (64bit)、Excel® for Microsoft 365 (64bit)
・Windows 10 Home (64bit)、Excel® 2021 (32bit)
コード(前半)はこちら。
'プロセス監視用API
Private Declare PtrSafe Function OpenProcess Lib "kernel32" (ByVal dwDesiredAccess As Long, ByVal bInheritHandle As Long, ByVal dwProcessID As LongPtr) As LongPtr
Private Declare PtrSafe Function CloseHandle Lib "kernel32" (ByVal hObject As LongPtr) As Long
Private Declare PtrSafe Function WaitForInputIdle Lib "user32" (ByVal hProcess As LongPtr, ByVal dwMilliseconds As Long) As LongPtr
Private Const SYNCHRONIZE As Long = &H100000
Private Const INFINITE As Long = &HFFFF
'------------------------
'ウィンドウ情報(ハンドル・キャプション名・クラス名)取得用API
Private Declare PtrSafe Function EnumWindows Lib "user32" (ByVal lpEnumFunc As LongPtr, ByVal lParam As Long) As Long
Private Declare PtrSafe Function EnumChildWindows Lib "user32.dll" (ByVal hWndParent As LongPtr, ByVal lpEnumFunc As LongPtr, lParam As Long) As Long
Private Declare PtrSafe Function IsWindowVisible Lib "user32" (ByVal hWnd As LongPtr) As LongPtr
Private Declare PtrSafe Function GetWindowText Lib "user32" Alias "GetWindowTextA" (ByVal hWnd As LongPtr, ByVal lpString As String, ByVal cch As LongPtr) As LongPtr
Private Declare PtrSafe Function GetParent Lib "user32" (ByVal hWnd As LongPtr) As LongPtr
Private Declare PtrSafe Function GetNextWindow Lib "user32" Alias "GetWindow" (ByVal hWnd As LongPtr, ByVal wFlag As LongPtr) As LongPtr
Private Declare PtrSafe Function GetClassName Lib "user32" Alias "GetClassNameA" (ByVal hWnd As LongPtr, ByVal lpClassName As String, ByVal nMaxCount As LongPtr) As LongPtr
Private Const GW_HWNDNEXT = &H2
'------------------------
'Edge画面DOM取得用API
Private Declare PtrSafe Function RegisterWindowMessage Lib "user32" Alias "RegisterWindowMessageA" (ByVal lpString As String) As LongPtr
Private Declare PtrSafe Function SendMessageTimeout Lib "user32" Alias "SendMessageTimeoutA" (ByVal hWnd As LongPtr, ByVal msg As LongPtr, _
ByVal wParam As LongPtr, ByVal lParam As LongPtr, ByVal fuFlags As LongPtr, ByVal uTimeout As LongPtr, lpdwResult As LongPtr) As LongPtr
Private Declare PtrSafe Function ObjectFromLresult Lib "oleacc" (ByVal lResult As LongPtr, riid As Any, ByVal wParam As LongPtr, ppvObject As Object) As LongPtr
Private Type GUID
Data1 As Long
Data2 As Integer
Data3 As Integer
Data4(0 To 7) As Byte
End Type
Private Const SMTO_ABORTIFHUNG = &H2
'------------------------
'Edge画面操作用API
Private Declare PtrSafe Sub Sleep Lib "kernel32" (ByVal ms As LongPtr)
Private Declare PtrSafe Function SetForegroundWindow Lib "user32" (ByVal hWnd As LongPtr) As LongPtr
'------------------------
'モジュール変数
Private Title As String
Private hWnd As LongPtr
Private IES_hWnd As LongPtr 'クラス名「Internet_Explorer_Server」のハンドル
Private Num As Integer 'ウィンドウハンドル要素数
Private hWnds() As LongPtr 'ウィンドウハンドル配列
Private objEdge As Object 'mshtml.IHTMLDocument2 'IES_hWndウィンドウをDOM化(HTMLDocument化)したオブジェクト
'-----------メイン処理-----------
Private Sub Sample()
'ブラウザ起動&プロセス監視(入力受付状態になるまで待つ)
Dim URL As String
Dim rc As LongPtr
Dim ProchWnd As LongPtr
URL = "https://www.google.co.jp/" '←任意のURLに変えて
rc = Shell(Environ("ProgramFiles(x86)") & "\Microsoft\Edge\Application\msedge.exe " & URL, vbNormalFocus)
'rc = Shell(Environ("ProgramFiles") & "\Microsoft\Edge\Application\msedge.exe " & URL, vbNormalFocus)
'rc = Shell(Environ("LOCALAPPDATA") & "\Microsoft\msedge.exe " & URL, vbNormalFocus)
Sleep 500
ProchWnd = OpenProcess(SYNCHRONZE, 0&, rc)
If ProchWnd <> 0 Then
Call WaitForInputIdle(ProchWnd, INFINITE)
End If
Call CloseHandle(ProchWnd)
Debug.Print "Edge立上げ完了"
'ページ読み込み待ち
Dim TimeOutCnt As Integer
TimeOutCnt = 0
Do
Sleep 2000
Title = "Google"
Call Get_IES_hWnd(Title) 'Sub①
TimeOutCnt = TimeOutCnt + 1
If TimeOutCnt > 5 Then
MsgBox "タイムアウト"
End
End If
Loop While IES_hWnd = 0
Debug.Print "ページ読み込み完了"
'HTMLDocument2オブジェクト生成
Call Get_HTMLDocument(IES_hWnd) 'Sub②
'対象ページ操作
objEdge.getElementsByName("q")(0).Value = "地獄の油揚げ"
For Each button In objEdge.getElementsByTagName("input")
If button.Type = "submit" Then
button.Click
Exit For
End If
Next
Debug.Print "ブラウザ操作完了"
End Sub
'-----------メイン処理(おわり)-----------
'-----------サブルーチン処理----------
Sub Get_IES_hWnd(ByVal Title As String) 'Sus①
’本記事の後半で紹介。
End Sub
Private Sub Get_HTMLDocument(IES_hWnd As LongPtr) 'Sub②
’本記事の後半で紹介。
End Sub
'-----------サブルーチン処理(おわり)----------
簡略化のため、エラー処理は抜いてあります。
このサンプルでは、「Googleを開く→検索ボックスに文字入力→検索ボタンを押す」の操作を自動化してみました。
あとはサブルーチン処理(後半)を埋めれば、動作します。
サブルーチン処理のコード(後半)はこちら。
ここから先は
¥ 1,000
この記事が気に入ったらサポートをしてみませんか?