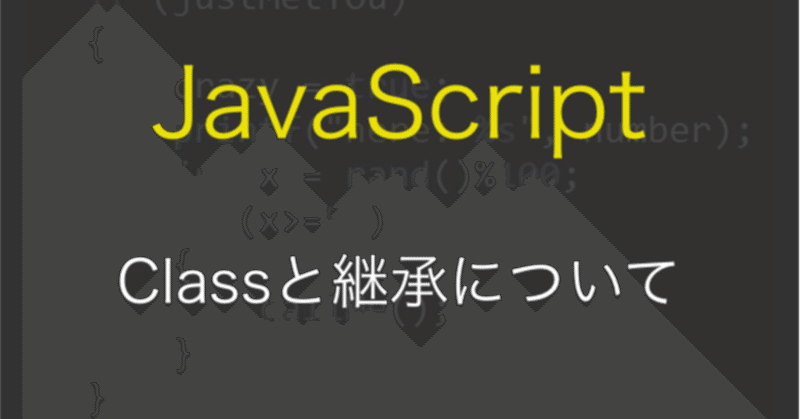
JS学習記録第14回 Classと継承について
こんばんは!
前回はプロトタイプの継承についてまとめてみました!
今回はclassの継承についてまとめてみたいと思います!
classについて
classはcssなどを理解している人には分かるかもしれませんが、classを使用することによって使い回しができるようになります。
前回まとめたプロトタイプと同じ意味になりますが、簡単にいうと新しい記述方法といった感じなんでしょうか?(T ^ T)多分
classで記述する方がまとまって見えます!
例として前回のプロトタイプのソースを持ってきます。
function Person(name,age){
this.name = name;
this.age = age;
this.a = function(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
}
function Person2(name,age){
Person.call(this,name,age);
}
Person2.prototype = Object.create(Person.prototype);
Person2.prototype = Object.create(Person.prototype);
でPersonという関数のデータをPerson2という関数に継承するという意味になりましたよね。
ではまず、継承の前に、
プロトタイプの記述をclassを用いて書いてみると、以下のようになります。
class Person{
constructor(name,age){
this.name = name;
this.age = age;
}
a(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
}
const b = new Person('yuta', 28);
console.log(b);
b.a();
実際にコンソールを出力してみても、値が正しく表示されていることがわかります。
class 関数名{
constructor(引数);
}
この記述方法が基準になってきます。
では次に継承をしてみましょう。
class Person{
constructor(name,age){
this.name = name;
this.age = age;
}
a(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
}
class Person2 extends Person{
constructor(name,age){
super(name,age)
}
a(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
}
継承は以上のようにすることができます!
class Person2 extends Person←PersonのデータをPerson2に持たせる。
このextendsが鍵ですね!
そしてsuperというメソッド?でPersonのデータを保持するようにしてます。
この流れのようですね。
class Person{
constructor(name,age){
this.name = name;
this.age = age;
}
a(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
}
class Person2 extends Person{
constructor(name,age){
super(name,age)
}
a(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
}
const b = new Person2('yuta', 28);
console.log(b);
試しにPerson2の中身を確認すると、
Person2に値が入っていることがわかります!
因みに新たにPerson2に値を追加することも可能です。
class Person{
constructor(name,age){
this.name = name;
this.age = age;
}
a(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
}
class Person2 extends Person{
constructor(name,age){
super(name,age)
}
a(){
console.log('私の名前は' + this.name + 'です。年齢は' + this.age + 'です。' )
}
c(){
console.log('こんにちは' + this.name);
}
}
const b = new Person2('yuta', 28);
console.log(b);
b.c();
以上でclassの継承についてまとめてみました!
プロトタイプで記述するのとclassで記述する方法はやっていることは同じことですが、理解を含める上では両方理解するのが大事そうですね。。
大阪在住の29歳。web制作会社勤務。noteを通してたくさんの出会いを作っていきたいです。台湾が大好きで中国語勉強中。日台夫婦。日々の挑戦の記録や、社会の生きづらさ、台湾のことなどを書いていこうと思います。2023年台湾に移住予定です。