JS 入力フォーム#1 [見た目・デザイン]
流れ
HTMLとCSSで入力フォームの見た目を整える。 ←今回
JavaScriptで入力内容の確認を行う。
送信ボタンがクリックされたときの処理を実装する。
確認画面の見た目を作成する。
確認画面に入力されたデータを表示する処理を追加する。
全体のまとめ
画面
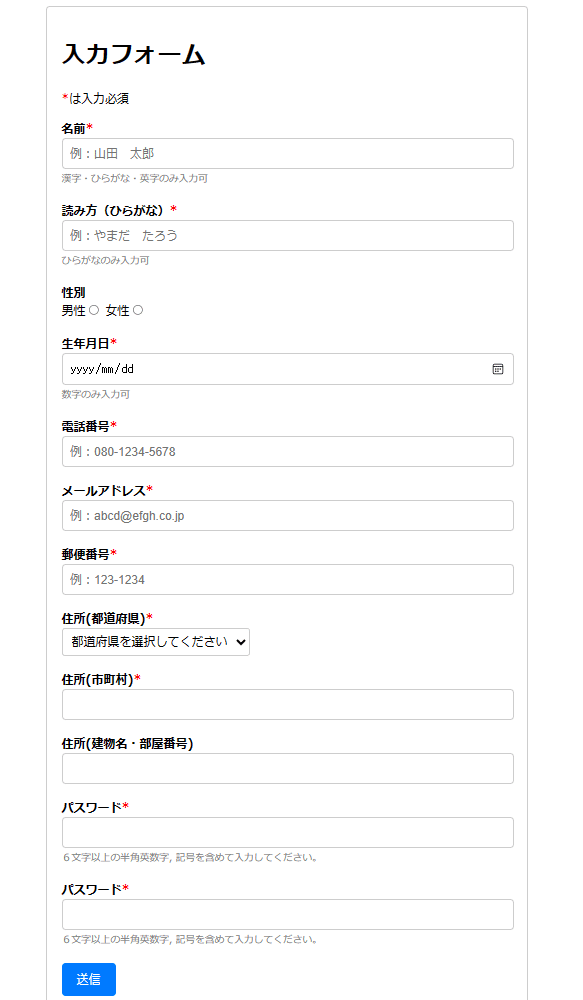
ソースコード
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>入力フォームの例</title>
<link rel="stylesheet" href="index.css">
</head>
<body>
<div class="container">
<h1>入力フォーム</h1>
<p><span class="required">*</span>は入力必須</p>
<form id="inputForm" action="../ComfirmForm/comfirm.html">
<div class="form-group">
<label for="name">名前<span class="required">*</span></label>
<input type="text" id="nameKan" name="nameKan" placeholder="例:山田 太郎" name="nameKab" required>
<span class="attention">漢字・ひらがな・英字のみ入力可</span>
</div>
<div class="form-group">
<label for="name">読み方(ひらがな)<span class="required">*</span></label>
<input type="text" id="nameHira" name="nameHira" placeholder="例:やまだ たろう" name="nameHira" required>
<span class="attention">ひらがなのみ入力可</span>
<div id="nameHiraMessage"></div>
</div>
<div class="form-group">
<label>性別</label>
男性<input type="radio" id="male" name="gender" value="male" required>
女性<input type="radio" id="female" name="gender" value="female" required>
<div id="genderMessage"></div>
</div>
<div class="form-group">
<label for="dob">生年月日<span class="required">*</span></label>
<input type="date" id="YMD" name="YMD" name="YMD" required>
<span class="attention">数字のみ入力可</span>
</div>
<div class="form-group">
<label for="phone">電話番号<span class="required">*</span></label>
<input type="tel" id="phone" name="phone" placeholder="例:080-1234-5678" name="phone" required>
</div>
<div class="form-group">
<label for="email">メールアドレス<span class="required">*</span></label>
<input type="email" id="email" name="email" placeholder="例:abcd@efgh.co.jp" name="email" required>
</div>
<div class="form-group">
<label for="zipcode">郵便番号<span class="required">*</span></label>
<input type="text" id="zipcode" name="zipcode" maxlength="8" placeholder="例:123-1234" name="zipcode" required>
<div id="addressMessage"></div>
</div>
<div class="form-group">
<label for="prefecture">住所(都道府県)<span class="required">*</span></label>
<select id="prefecture" name="prefecture" required>
<option value="" disabled selected>都道府県を選択してください</option>
</select>
</div>
<div class="form-group">
<label for="city">住所(市町村)<span class="required">*</span></label>
<input type="text" id="city" name="city" required>
</div>
<div class="form-group">
<label for="address">住所(建物名・部屋番号)</label>
<input type="text" id="building" name="building" required>
</div>
<div class="form-group">
<label for="password">パスワード<span class="required">*</span></label>
<input type="password" id="password" name="password" required>
<span class="attention">6文字以上の半角英数字, 記号を含めて入力してください。</span>
</div>
<div class="form-group">
<label for="password">パスワード<span class="required">*</span></label>
<input type="password" id="samePassword" name="samePassword" required>
<span class="attention">6文字以上の半角英数字, 記号を含めて入力してください。</span>
</div>
<h2><div id="errorMessage" class="error-message"></div></h2>
<button type="button" id="submitButton" onclick="submitForm()">送信</button>
</form>
</div>
<script src="index.js"></script>
</body>
</html>
CSS
.container {
max-width: 600px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc ;
border-radius: 5px;
}
.form-group {
margin-bottom: 20px;
margin-right: 20px;
}
label {
display: block;
font-weight: bold;
}
input[type="text"],
input[type="email"],
input[type="tel"],
input[type="password"],
input[type="date"] {
width: 100%;
padding: 10px;
font-size: 16px;
border-radius: 5px;
border: 1px solid #ccc ;
}
button {
padding: 10px 20px;
font-size: 16px;
border-radius: 5px;
background-color: #007bff ;
color: #fff ;
border: none;
cursor: pointer;
}
button:hover {
background-color: #0056b3 ;
}
.required {
color: red;
}
.error-message {
color: red;
margin-top: 10px;
}
.attention{
color: grey;
font-size: small;
}
select {
font-size: 16px;
background-color: #fff ;
color: #000 ;
padding: 8px;
border: 1px solid #ccc ;
border-radius: 4px;
}
この記事が気に入ったらサポートをしてみませんか?