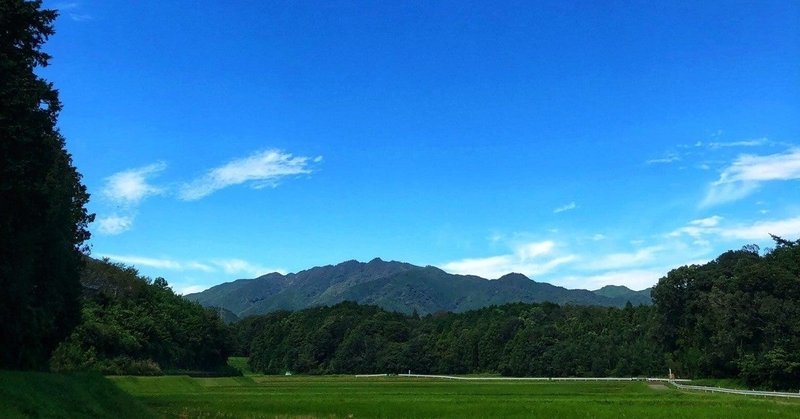
Railsまとめ③
ログイン機能付きのブログアプリ作成
・ルートパス
アプリケーションの大元のアクセス先。英語でrootと書き、
根っこという意味。ルートパスから様々なページに分岐していく。
・root メソッド
root to: 'コントローラー名#アクション名'
①ルーティングを設定
config/routes.rb を編集
Rails.application.routes.draw do
root to: 'posts#index'
end
②コントローラーを設定
ターミナル
# コントローラ作成
$ rails g controller posts
app/controllers/posts_controller.rb を編集
class PostsController < ApplicationController
def index
end
end
③モデルを作成
ターミナル
# モデルの作成
$ rails g model post
④マイグレーションファイル編集
db/migrate/2020~~~~~_create_posts.rb を編集
def change
create_table :posts do |t|
t.text :title
t.text :content
t.timestamps
end
end
end
# マイグレーション実行。Sequel Proでテーブル確認。
$ rails db:migrate
〜マイグレーションファイルの修正方法〜
データベース反映済みのマイグレーションファイルは修正しない。
・rails db:rollback コマンド
ロールバック→修正→マイグレートで修復。
・rails db:migrate:status コマンド
マイグレーションファイルがデータベースに適用されているか調べる。
適用時はupと表示、非適用時は、downと表示され修正や削除が可能。
⑤コントローラーに追記
app/controllers/posts_controller.rb を編集。
# indexに追記
@posts = Post.all
⑥ビューファイルを作成
index.html.erb を作成、編集。
<% @posts.each do |post| %>
<div class="content">
<div class="content__left">
<div class="content__left--image"></div>
</div>
<div class="content__right">
<div class="content__right__top">
<%= post.title %>
</div>
<div class="content__right__bottom">
<div class="content__right__bottom--date">
<%= post.created_at %>
</div>
</div>
</div>
</div>
<% end %>
・レイアウトテンプレート
アプリケーションのビューファイルの共通部分をまとめたもの。app/views/layouts/application.html.erbがそれにあたります。
・yield メソッド
ここに全てのビューファイルが集約されるという仕組み。
ヘッダーを集約
app/views/layouts/application.html.erb を編集
# 全ページのヘッダーを一緒にできる。
<!DOCTYPE html>
<html>
<head>
<title>Blog</title>
<%= csrf_meta_tags %>
<%= csp_meta_tag %>
<%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %>
<%= javascript_include_tag 'application', 'data-turbolinks-track': 'reload' %>
</head>
<body>
<header class="header">
<div class="header__title">
<%= link_to "Blog", '#', class: "header__title--text" %>
</div>
<div class="header__right">
<%= link_to "新規投稿", '#', class: "header__right--btn" %>
</div>
</header>
<div class="contents">
<%= yield %>
</div>
</body>
</html>
・stylesheet_link_tag メソッド
呼び出すCSSのファイルを指定することができるヘルパーメソッド。
CSSファイルは app/assets/stylesheets/ というディレクトリに配置する。
stylesheet_link_tagのapplicationは、app/assets/stylesheets/application.cssからファイルを読み込むことを示しています。
・require_tree
引数として与えられたディレクトリ以下のCSSファイルをアルファベット順に全て読み込むという意味がある。require_treeの引数は.(ドット)。
引数.(ドット)はカレントディレクトリを表す。app/assets/stylesheets というディレクトリにあるファイルは全て読み込まれる。
app/assets/stylesheets/posts.scss を編集
body{
background-color: #f6f6f6;
min-width: 650px;
.header{
height: 56px;
width: 100%;
padding: 0 150px;
background-color: #55c500;
color: #fff;
display: flex;
justify-content: space-between;
&__title{
font-size: 30px;
line-height: 56px;
&--text{
text-decoration: none;
color: #fff;
}
}
&__right{
&--btn{
text-decoration: none;
line-height: 56px;
color: #fff;
margin-left:15px;
}
}
}
.contents{
margin: 30px auto 0;
background-color: #fff;
height: 600px;
width: 70%;
overflow: scroll;
.content{
padding: 10px;
border-bottom: 1px solid #f6f6f6;
display: flex;
&__left{
padding-right: 20px;
&--image{
height: 55px;
width: 55px;
border: solid 1px;
}
}
&__right{
width: calc(100%-100px);
&__top{
font-size: 20px;
font-weight: bold;
&--title{
text-decoration: none;
color: #000000;
}
}
&__bottom{
font-size: 15px;
color: #999;
display: flex;
&--userName{
&--btn{
text-decoration: none;
color: #999;
}
}
&--date{
margin-left: 20px;
}
}
}
&__title{
font-size: 30px;
font-weight: bold;
padding-top: 30px;
text-align: center;
}
}
.postTitle{
padding-top: 20px;
text-align: center;
font-size: 20px;
font-weight: bold;
}
.postDate{
font-size: 15px;
color: #999;
text-align: center;
}
.postAuthor{
font-size: 15px;
color: #999;
text-align: center;
}
.postManage{
display: flex;
justify-content: center;
margin-top: 10px;
&__edit{
display: block;
border-radius: 5px;
color: #ffffff;
background-color:#55c500;
padding: 0 10px;
text-decoration: none;
margin-right: 10px;
}
&__delete{
display: block;
border-radius: 5px;
color: #ffffff;
background-color:#55c500;
padding: 0 10px;
text-decoration: none;
margin-left: 10px;
}
}
.postText{
margin: 20px 30px;
}
}
.form{
width: 85%;
height: 100%;
margin: 0 auto;
padding-top: 30px;
&__title{
display: block;
width: 100%;
height: 50px;
}
&__text{
display: block;
width: 100%;
height: 50%;
margin-top: 15px;
}
&__btn{
display: block;
width: 30%;
margin: 15px auto 0;
}
}
.user{
padding: 15px 30px;
}
}
・resources メソッド
Railsで定義される7つのアクションをひとまとめにしたメソッド。
・only オプション
resourcesメソッドはオプションを指定しないと、全アクションが生成される。onlyで、指定アクションのルーティングのみ設定できる。
投稿ページ実装
①ルーティングを設定
config/routes.rb を編集
# newアクションのルーティングを生成
Rails.application.routes.draw do
root to: 'posts#index'
resources :posts, only: [:new]
end
②コントローラー編集
app/controllers/posts_controller.rb を編集
# index下に追記
def new
end
③ビューファイル作成
app/views/posts/new.html.erb を作成、編集。
<div class="content__title">
新規記事の作成
</div>
④ブラウザで確認
http://localhost:3000/posts/new
⑤リンクを設定
app/views/layouts/application.html.erb を編集
# 遷移先の記述方法を変更
<div class="header__title">
<%= link_to "Blog", root_path, class: "header__title--text" %>
</div>
<div class="header__right">
<%= link_to "新規投稿", 'new_post_path', class: "header__right--btn" %>
</div>
・Prefix
URI Patternを変数化したもの。rails routesで確認できる。
【例】
# Prefix未使用
<%= link_to "Blog", '/', class: "header__title--text" %>
# Prefix使用
<%= link_to "Blog", root_path, class: "header__title--text" %>
・form_with メソッド
Rails 5.1バージョンから推奨されているフォーム実装のヘルパーメソッド。
【例】
<!-- form_tag使用例 -->
<%= form_tag('/posts', method: :post) do %>
<input type="text" name="content">
<input type="submit" value="投稿">
<% end %>
<!-- form_with使用例 -->
<%= form_with model: @post, local: true do |form| %>
<%= form.text_field :content %>
<%= form.submit '投稿' %>
<% end %>
・form_withで自動でパスを選択し、HTTPメソッドを指定する必要が無い。
・ActiveRecordを継承するモデルのインスタンスが利用可(@postが該当)
・form_withではinputタグは用いない。
⑥インスタンスを生成
app/controllers/posts_controller.rb を編集
# new内に記述
@post = Post.new
⑦フォームを記載
app/views/posts/new.html.erb を編集
<div class="content__title">
新規記事の作成
</div>
<%= form_with model: @post, class: :form, local: true do |form| %>
<%= form.text_field :title, placeholder: :タイトル, class: :form__title %>
<%= form.text_area :content, placeholder: :本文, class: :form__text %>
<%= form.submit '投稿する', class: :form__btn %>
<% end %>
投稿機能の実装
①ルーティングを設定
config/routes.rb を編集。
# createアクション追加。rails routesで確認。
resources :posts, only: [:new, :create]
②コントローラー編集
app/controllers/posts_controller.rb を編集。
# new以下に追記
def create
Post.create(post_params)
end
# form_withはrequire(モデル名)を追記する。
private
def post_params
params.require(:post).permit(:title, :content)
end
・リダイレクト
本来受け取ったパスとは別のパスへ転送する機能
・redirect_to メソッド
【例】
# パスで直接リダイレクト先を指定する場合
redirect_to "リダイレクト先のパス"
③リダイレクト処理
app/controllers/posts_controller.rb を編集。
def create
Post.create(post_params)
redirect_to root_path
end
・バリデーション
データを登録する際に、一定の制約をかけること。モデルに記載する。
空のデータが登録できないようにする。すでに登録されている文字列を登録できないようにする(メールアドレスの登録など)。
文字数制限をかける(パスワードなど)。
④バリデーション設定
app/models/post.rb を編集
class Post < ApplicationRecord
validates :title, :content, presence: true
end
個別記事にアクセス
①ルーティングを設定
config/routes.rb を編集。
# showアクションのルーティングを生成。rails routesで確認。
resources :posts, only: [:new, :create, :show]
/posts/:id(.:format)が生成される。ブラウザからのリクエストにメッセージのIDを含み、この:idに入るとコントローラーにparams[:id]として送られる。
データベース内のIDカラム。
②コントローラー編集
app/controllers/posts_controller.rb を編集。
# create下に追記
def show
@post = Post.find(params[:id])
end
③ビューファイル作成
app/views/posts/show.html.erb を作成、編集。
<div class="postTitle">
<%= @post.title %>
</div>
<div class="postDate">
<%= @post.created_at %>
</div>
<div class="postAuthor">
</div>
<div class="postText">
<%= simple_format @post.content %>
</div>
・simple_format メソッド
投稿時に改行を含むデータの送信時、その改行を反映する。
④詳細ページに遷移する設定
app/views/posts/index.html.erb
<div class="content__right__top">
<%= link_to post.title, post_path(post.id), class: "content__right__top--title" %>
</div>
⑤ブラウザで確認
データを編集する
①ルーティングを設定
config/routes.rb を編集。
# editアクションのルーティングを生成。rails routesで確認。
resources :posts, only: [:new, :create, :show, :edit]
②コントローラー編集
app/controllers/posts_controller.rb を編集。
# show下に追記
def edit
@post = Post.find(params[:id])
end
③ビューファイル作成
app/views/posts/edit.html.erb を作成、編集。
<div class="content__title">
投稿の編集
</div>
<%= form_with model: @post, class: :form, local: true do |form| %>
<%= form.text_field :title, placeholder: :タイトル, class: :form__title %>
<%= form.text_area :content, placeholder: :本文, class: :form__text %>
<%= form.submit '投稿する', class: :form__btn %>
<% end %>
④編集ページに遷移する。
app/views/posts/show.html.erb
<div class="postTitle">
<%= @post.title %>
</div>
<div class="postDate">
<%= @post.created_at %>
</div>
<div class="postAuthor">
</div>
<div class="postManage">
<%= link_to "編集", edit_post_path(@post.id), class: "postManage__edit" %>
</div>
<div class="postText">
<%= simple_format @post.content %>
</div>
⑤ブラウザで確認
⑥ルーティングを設定
config/routes.rb を編集
# updateアクションのルーティングを生成。rails routesで確認。
resources :posts, only: [:new, :create, :show, :edit, :update]
⑦コントローラー編集
・updateメソッド
ActiveRecordのメソッド。すでにレコードに含まれている情報を更新する
app/controllers/posts_controller.rb を編集
# edit下に追記
def update
post = Post.find(params[:id])
post.update(post_params)
redirect_to post_path(post.id)
end
⑧ビューファイル作成
app/views/posts/edit.html.erb を作成、編集。
<div class="content__title">
投稿の編集
</div>
<%= form_with model: @post, class: :form, local: true do |form| %>
<%= form.text_field :title, placeholder: :タイトル, class: :form__title %>
<%= form.text_area :content, placeholder: :本文, class: :form__text %>
<%= form.submit '投稿する', class: :form__btn %>
<% end %>
⑨編集ページに遷移する設定
app/views/posts/show.html.erb
<div class="postTitle">
<%= @post.title %>
</div>
<div class="postDate">
<%= @post.created_at %>
</div>
<div class="postAuthor">
</div>
<div class="postManage">
<%= link_to "編集", edit_post_path(@post.id), class: "postManage__edit" %>
</div>
<div class="postText">
<%= simple_format @post.content %>
</div>
⑩ブラウザで確認
データ削除
①ルーティングを設定
config/routes.rb を編集
# destroyアクションのルーティングを生成。rails routesで確認。
resources :posts, only: [:new, :create, :show, :edit, :update, :destroy]
②コントローラー編集
app/controllers/posts_controller.rb を編集
# update下に追記。2行目で削除するレコードを指定。3行目で削除。
def destroy
post = Post.find(params[:id])
post.destroy
redirect_to root_path
end
③ビューファイル作成
app/views/posts/show.html.erb を作成、編集。
<div class="postTitle">
<%= @post.title %>
</div>
<div class="postDate">
<%= @post.created_at %>
</div>
<div class="postAuthor">
</div>
<div class="postManage">
<%= link_to "編集", edit_post_path(@post.id), class: "postManage__edit" %>
<%= link_to "削除", post_path(@post.id), method: :delete, class: "postManage__delete" %>
</div>
<div class="postText">
<%= simple_format @post.content %>
</div>
・except オプション
resourcesメソッドのオプション。除外するという意味。
この後に指定したアクションに対するルーティングは生成されません。
config/routes.rb を編集
Rails.application.routes.draw do
root to: 'posts#index'
resources :posts, except: :index
end
この記事が気に入ったらサポートをしてみませんか?