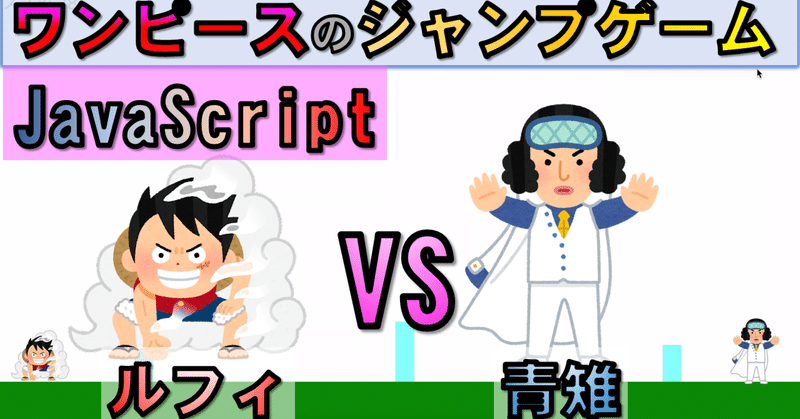
【JavaScript】ジャンプゲームをプログラミングで作成(ルフィVS青雉)
JavaScriptでジャンプゲームを作成しました。
青雉が氷のブロックで攻撃します。
それをジャンプで回避し続けるゲームとなります。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>ジャンプゲーム</title>
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="stylesheet" media="screen" href="style.css">
</head>
<body>
<div id="ground"></div>
<div id="character"></div>
<div id="enemy"></div>
<div class="obstacles"></div>
<h2>スコア: <span id="score">0</span></h2>
<script src="index.js"></script>
</body>
</html>
* {
margin: 0;
padding: 0;
}
body {
width: 100%;
height: 100vh;
position: relative;
overflow: hidden;
}
#ground{
width: 100%;
height: 100px;
background-color: green;
position: absolute;
bottom: 0;
}
#character{
width: 100px;
height: 100px;
background-image: url("img/luffy.png");
background-size: contain;
position: absolute;
bottom: 90px;
right: calc(100% - 120px);
}
#enemy{
width: 120px;
height: 120px;
background-image: url("img/aokiji.png");
background-size: contain;
background-repeat: no-repeat;
background-position: center;
position: absolute;
bottom: 90px;
left: calc(100% - 140px);
}
.obstacle{
width: 30px;
background-color: rgb(132, 245, 255);
position: absolute;
bottom: 100px;
right: 100px;
}
h2 {
margin-top: 10px;
margin-left: 10px;
}
let character = document.getElementById("character");
let characterBottom = parseInt(window.getComputedStyle(character).getPropertyValue("bottom"));
let characterRight = parseInt(window.getComputedStyle(character).getPropertyValue("right"));
let characterWidth = parseInt(window.getComputedStyle(character).getPropertyValue("width"));
//window.getComputedStyle(character) CSSプロパティの値を含むオブジェクトを返す
let ground = document.getElementById("ground");
let groundBottom = parseInt(window.getComputedStyle(ground).getPropertyValue("bottom"));
let groundHeight = parseInt(window.getComputedStyle(ground).getPropertyValue("height"));
let isJumping = false;
let upTime; //ジャンプしている時間(上昇中)を管理
let downTime;//ジャンプしている時間(落下中)を管理
const JUMP_SPEED = 10;
const JUMP_HEIGHT = 250;
const OBSTACLE_SPEED = 5;
let displayScore = document.getElementById("score");
let score = 0;
const CLEAR_SCORE = 100;
function showScore() {
score++;
displayScore.innerText = score;
}
setInterval(showScore, 100);
function Jump() {
if(isJumping) return;
upTime = setInterval(() => {
if (characterBottom >= groundHeight + JUMP_HEIGHT) {
clearInterval(upTime);
downTime = setInterval(() => {
if (characterBottom <= groundHeight) {
clearInterval(downTime);
isJumping = false;
}
characterBottom -= JUMP_SPEED;
character.style.bottom = characterBottom + "px";
}, 20);
}
characterBottom += JUMP_SPEED;
character.style.bottom = characterBottom + "px";
isJumping = true;
}, 20);
}
function generateObstacle() {
let obstacles = document.querySelector(".obstacles");
let obstacle = document.createElement("div");
obstacle.setAttribute("class", "obstacle");
obstacles.appendChild(obstacle);
let randomTimeout = Math.floor(Math.random() * 1000) + 1000;
let obstacleRight = parseInt(window.getComputedStyle(obstacle).getPropertyValue("right"));
let obstacleBottom = parseInt(window.getComputedStyle(obstacle).getPropertyValue("bottom"));
let obstacleWidth = parseInt(window.getComputedStyle(obstacle).getPropertyValue("width"));
let obstacleHeight = Math.floor(Math.random() * JUMP_HEIGHT/4) + JUMP_HEIGHT/5;
function moveObstacle() {
obstacleRight += OBSTACLE_SPEED;
obstacle.style.right = obstacleRight + "px";
obstacle.style.bottom = obstacleBottom + "px";
obstacle.style.width = obstacleWidth + "px";
obstacle.style.height = obstacleHeight + "px";
if (characterRight >= obstacleRight - characterWidth && characterRight <= obstacleRight + obstacleWidth && characterBottom <= obstacleBottom + obstacleHeight) {
alert(`ゲームオーバー`);
clearInterval(obstacleInterval);
clearTimeout(obstacleTimeout);
location.reload();
}
if (score > CLEAR_SCORE) {
alert("ゲームクリア");
clearInterval(obstacleInterval);
clearTimeout(obstacleTimeout);
location.reload();
}
}
let obstacleInterval = setInterval(moveObstacle, 20);
let obstacleTimeout = setTimeout(generateObstacle, randomTimeout);
}
generateObstacle();
function control(e) {
if (e.key == "ArrowUp") {
Jump();
}
}
document.addEventListener("keydown", control);
この記事が気に入ったらサポートをしてみませんか?