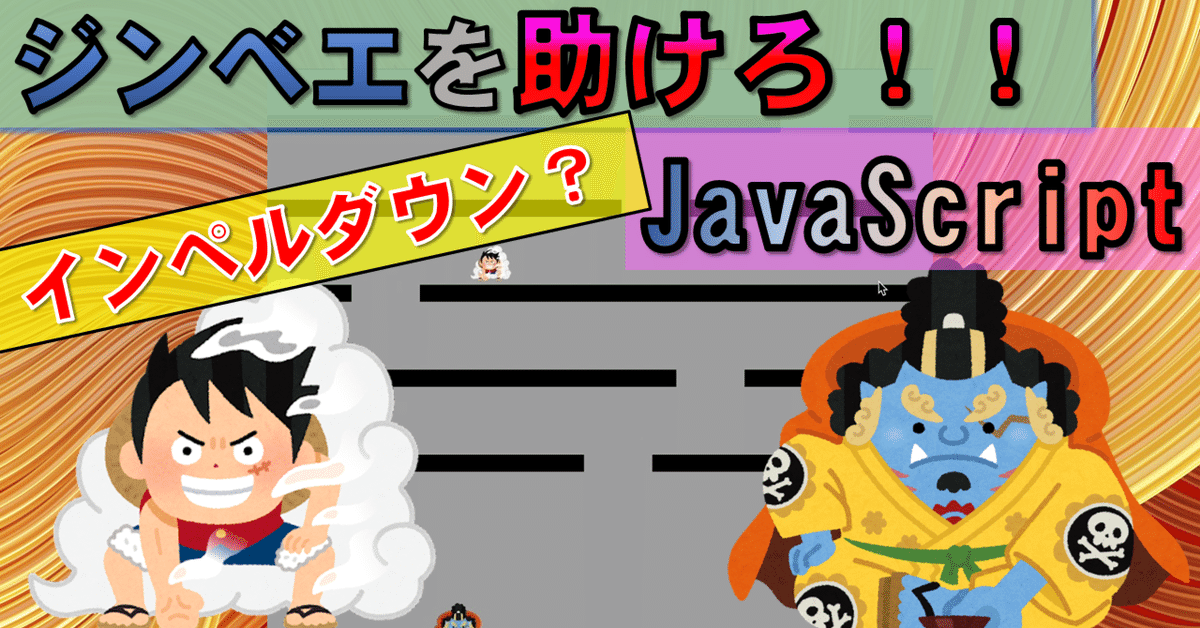
【JavaScript】ワンピースのインペルダウン?(地下へ進むゲーム)をプログラミングで作成
JavaScriptで地下へ突き進むゲームを作成しました。
インペルダウンでルフィがエースを助けに行く状況をイメージしてみました。
ただ、今回はジンベエのところまで辿り着ければクリアです。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>地下迷宮ゲーム</title>
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="stylesheet" media="screen" href="style.css">
</head>
<body>
<div id="game">
<div id="character"></div>
</div>
<script src="index.js"></script>
</body>
</html>
* {
margin: 0;
padding: 0;
}
#game {
width: 800px;
height: 700px;
border: 1px solid black;
margin: auto;
background-color: darkgrey;
}
#character {
width: 50px;
height: 50px;
background-image: url("img/luffy.png");
background-size: contain;
position: relative;
top: 200px;
left: 190px;
z-index: 100;
}
.block {
width: 800px;
height: 20px;
background-color: black;
position: relative;
top: 500px;
margin-top: -20px;
}
.hole {
width: 80px;
height: 20px;
background-color: darkgrey;
position: relative;
top: 500px;
margin-top: -20px;
}
#jinbe {
width: 80px;
height: 80px;
background-image: url("img/jinbe.png");
background-size: contain;
position: relative;
left: 190px;
z-index: 100;
}
let character = document.getElementById("character");
const characterWidth = parseInt(window.getComputedStyle(character).getPropertyValue("width"));
const characterHeight = parseInt(window.getComputedStyle(character).getPropertyValue("height"));
const moveSpeed = 2;//キャラの動くスピード
let game = document.getElementById("game");
const gameWidth = parseInt(window.getComputedStyle(game).getPropertyValue("width"));
const gameHeight = parseInt(window.getComputedStyle(game).getPropertyValue("height"));
let interval;//キャラの動きのインターバル
let moving = true;
let counter = 0;
let blockLastTop;
let holeLastTop;
let currentBlocks = []; //枠内にあるブロック
const blockDistance = 100;
const blockHeight = 20;
const blockTop = 500;
const holeWidth = 80;
const moveBlockSpeed = 0.5;
const clearBlockCount = 10;
function moveLeft() {
let left = parseInt(window.getComputedStyle(character).getPropertyValue("left"));
if (left > 0) {
character.style.left = left - moveSpeed + "px";
}
}
function moveRight() {
let left = parseInt(window.getComputedStyle(character).getPropertyValue("left"));
if (left < gameWidth - characterWidth) {
character.style.left = left + moveSpeed + "px";
}
}
document.addEventListener("keydown", (e) => {
if (moving) {
moving = false;
if (e.key === "ArrowLeft") {
interval = setInterval(moveLeft, 1);
} else if (e.key === "ArrowRight") {
interval = setInterval(moveRight, 1);
}
}
});
document.addEventListener("keyup", () => {
clearInterval(interval);
moving = true;
});
let blocks = setInterval(function () {
let blockLast = document.getElementById("block" + (counter - 1));
let holeLast = document.getElementById("hole" + (counter - 1));
if (counter > 0 && counter < clearBlockCount) {
blockLastTop = parseInt(window.getComputedStyle(blockLast).getPropertyValue("top"));
holeLastTop = parseInt(window.getComputedStyle(holeLast).getPropertyValue("top"));
}
if (counter < clearBlockCount && (blockLastTop < blockTop - blockHeight || counter === 0)) {
let block = document.createElement("div");
let hole = document.createElement("div");
block.setAttribute("class", "block");
hole.setAttribute("class", "hole");
block.setAttribute("id", "block" + counter);
hole.setAttribute("id", "hole" + counter);
block.style.top = blockLastTop + blockDistance + "px";
hole.style.top = holeLastTop + blockDistance + "px";
let random = Math.floor(Math.random() * (gameWidth - holeWidth));
hole.style.left = random + "px";
game.appendChild(block);
game.appendChild(hole);
currentBlocks.push(counter);
counter++;
}
let characterTop = parseFloat(getComputedStyle(character).getPropertyValue("top"));
let characterLeft = parseFloat(getComputedStyle(character).getPropertyValue("left"));
let drop = true;
if (characterTop <= 0) {
alert(`ゲームオーバー`);
clearInterval(blocks);
location.reload();
}
if (counter == clearBlockCount) {
counter++;
let jinbe = document.createElement("div");
jinbe.setAttribute("id", "jinbe");
jinbe.style.top = (gameHeight - 130) + "px";
game.appendChild(jinbe);
}
if (counter > clearBlockCount && characterTop >= (gameHeight - characterHeight)) {
alert("ゲームクリア");
clearInterval(blocks);
location.reload();
}
for (let i = 0; i < currentBlocks.length; i++) {
let current = currentBlocks[i];
let iblock = document.getElementById("block" + current);
let ihole = document.getElementById("hole" + current);
let iblockTop = parseFloat(window.getComputedStyle(iblock).getPropertyValue("top"));
let iholeLeft = parseFloat(window.getComputedStyle(ihole).getPropertyValue("left"));
iblock.style.top = iblockTop - moveBlockSpeed + "px";
ihole.style.top = iblockTop - moveBlockSpeed + "px";
if (iblockTop < - blockHeight) {
currentBlocks.shift();
iblock.remove();
ihole.remove();
}
if (iblockTop - blockHeight < characterTop && iblockTop > characterTop) {
drop = false;
if (iholeLeft <= characterLeft && iholeLeft + holeWidth >= characterLeft + characterWidth) {
drop = true;
}
}
}
if (drop) {
if (characterTop < gameHeight - characterHeight) {
character.style.top = characterTop + moveSpeed + "px";
}
} else {
character.style.top = characterTop - moveBlockSpeed + "px";
}
}, 1);
この記事が気に入ったらサポートをしてみませんか?