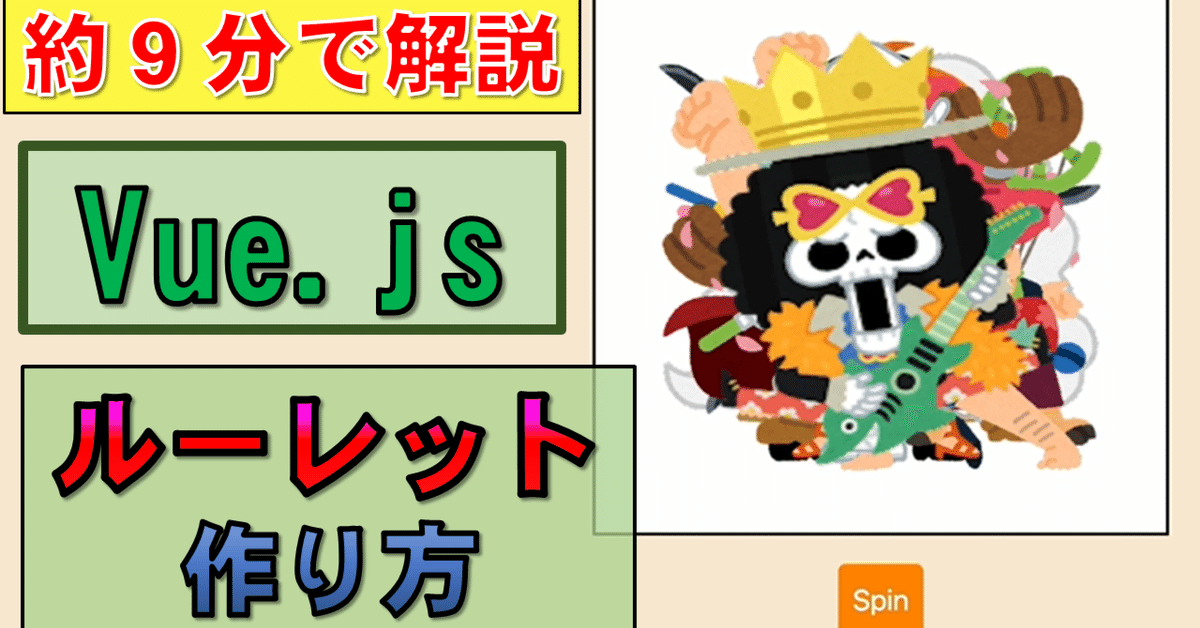
【Vue.js】ワンピースのルーレットを作成
今回はVue.jsを利用して、ルーレットゲームを作成しました。
v-bindやv-on、v-forなど基本的なものを利用していますので、理解しやすいかと思います。
ぜひ、作成してみてください。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>ルーレット</title>
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"
integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
<link rel="stylesheet" media="screen" href="style.css">
</head>
<body>
<div id="app">
<div id="roulette">
<img v-for="(character, index) in characters" :key="character" :src="'img/'+ character +'.png'"
:class="{'active': index === activeIndex}"
>
</div>
<button id="spin-button" @click="spin()">Spin</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js"></script>
<script src="index.js"></script>
</body>
</html>
const app = new Vue({
el: '#app',
data() {
return {
characters: ['luffy', 'zoro', 'sanji', 'nami', 'usopp', 'chopper', 'robin', 'franky', 'brook'],
activeIndex: 0,
spinning: false,
}
},
methods: {
spin() {
if (this.spinning) {
return;
}
this.spinning = true;
const numCharacters = this.characters.length;//9
const targetIndex = Math.floor(Math.random() * numCharacters); //0~8
const numRotations = 10 + targetIndex; //10~18回 最低回す
let currentIndex = this.activeIndex;
let rotationCount = 0;
const rotateInterval = setInterval(() => {
currentIndex = (currentIndex + 1) % numCharacters;
//index番号を1つ増やす
this.activeIndex = currentIndex;
rotationCount++;
if (rotationCount >= numRotations && currentIndex === targetIndex) {
clearInterval(rotateInterval);
this.spinning = false;
}
}, 300);
}
}
});
body {
text-align: center;
background-color: antiquewhite;
}
#roulette {
width: 400px;
height: 400px;
position: relative;
margin: 0 auto;
border: 2px solid black;
background-color: white;
}
#roulette img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 80%;
height: 80%;
opacity: 0;
transition: all 1s ease-in-out;
}
#roulette img.active {
opacity: 1;
}
#spin-button {
display: block;
margin: 20px auto;
padding: 10px;
font-size: 20px;
background-color: #ff8c00;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
この記事が気に入ったらサポートをしてみませんか?