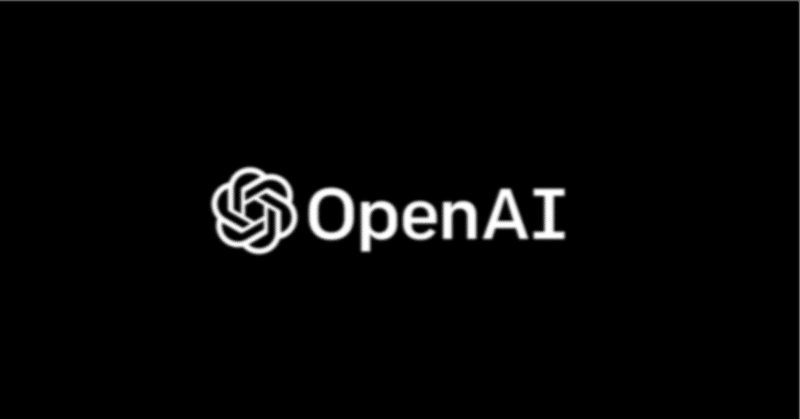
LangChain で ChatGPTプラグイン を使う機能を試す
「LangChain」で「ChatGPTプラグイン」を使う機能を試したので、まとめました。
1. AIPluginTool
「AIPluginTool」は、「ChatGPTプラグイン」などのAI用のプラグインを、LangChainのツールとして利用できるようにするクラスです。
現在、認証のないプラグインでのみ機能します。
2. Colabでの準備
Google Colabでの準備手順は、次のとおりです。
(1) パッケージのインストール。
# パッケージのインストール
!pip install langchain
!pip install openai
(2) 環境変数の準備。
以下のコードの <OpenAI_APIのトークン> にはOpenAI APIのトークンを指定します。(有料)
import os
os.environ["OPENAI_API_KEY"] = "<OpenAI_APIのトークン>"
(3) ChatGPTプラグインをツールとして読み込む。
今回は、「Klarna」のプラグイン (数千のオンラインショップから価格を検索・比較で) を読み込みます。
from langchain.tools import AIPluginTool
# ChatGPTプラグインをツールとして読み込む
tool = AIPluginTool.from_plugin_url(
"https://www.klarna.com/.well-known/ai-plugin.json")
(4) エージェントの準備
from langchain.chat_models import ChatOpenAI
from langchain.agents import load_tools, initialize_agent
# エージェントの準備
llm = ChatOpenAI(temperature=0)
tools = load_tools(["requests"] )s
tools += [tool]
agent_chain = initialize_agent(
tools,
llm,
agent="zero-shot-react-description",
verbose=True
)
(5) 質問応答。
klanaのプラグインに商品の情報を聞いてみました。
# 質問応答
agent_chain.run("what t shirts are available in klarna?")
> Entering new AgentExecutor chain...
I need to check the Klarna Shopping API to see if it has information on available t shirts.
Action: KlarnaProducts
Action Input: None
Observation: Usage Guide: Use the Klarna plugin to get relevant product suggestions for any shopping or researching purpose. The query to be sent should not include stopwords like articles, prepositions and determinants. The api works best when searching for words that are related to products, like their name, brand, model or category. Links will always be returned and should be shown to the user.
OpenAPI Spec: {'openapi': '3.0.1', 'info': {'version': 'v0', 'title': 'Open AI Klarna product Api'}, 'servers': [{'url': 'https://www.klarna.com/us/shopping'}], 'tags': [{'name': 'open-ai-product-endpoint', 'description': 'Open AI Product Endpoint. Query for products.'}], 'paths': {'/public/openai/v0/products': {'get': {'tags': ['open-ai-product-endpoint'], 'summary': 'API for fetching Klarna product information', 'operationId': 'productsUsingGET', 'parameters': [{'name': 'q', 'in': 'query', 'description': 'query, must be between 2 and 100 characters', 'required': True, 'schema': {'type': 'string'}}, {'name': 'size', 'in': 'query', 'description': 'number of products returned', 'required': False, 'schema': {'type': 'integer'}}, {'name': 'budget', 'in': 'query', 'description': 'maximum price of the matching product in local currency, filters results', 'required': False, 'schema': {'type': 'integer'}}], 'responses': {'200': {'description': 'Products found', 'content': {'application/json': {'schema': {'$ref': '#/components/schemas/ProductResponse'}}}}, '503': {'description': 'one or more services are unavailable'}}, 'deprecated': False}}}, 'components': {'schemas': {'Product': {'type': 'object', 'properties': {'attributes': {'type': 'array', 'items': {'type': 'string'}}, 'name': {'type': 'string'}, 'price': {'type': 'string'}, 'url': {'type': 'string'}}, 'title': 'Product'}, 'ProductResponse': {'type': 'object', 'properties': {'products': {'type': 'array', 'items': {'$ref': '#/components/schemas/Product'}}}, 'title': 'ProductResponse'}}}}
Thought:I need to use the Klarna Shopping API to search for t shirts.
Action: requests_get
Action Input: https://www.klarna.com/us/shopping/public/openai/v0/products?q=t%20shirts
Observation: {"products":[{"name":"Lacoste Men's Pack of Plain T-Shirts","url":"https://www.klarna.com/us/shopping/pl/cl10001/3202043025/Clothing/Lacoste-Men-s-Pack-of-Plain-T-Shirts/?source=openai","price":"$28.99","attributes":["Material:Cotton","Target Group:Man","Color:White,Black"]},{"name":"Hanes Men's Ultimate 6pk. Crewneck T-Shirts","url":"https://www.klarna.com/us/shopping/pl/cl10001/3201808270/Clothing/Hanes-Men-s-Ultimate-6pk.-Crewneck-T-Shirts/?source=openai","price":"$13.82","attributes":["Material:Cotton","Target Group:Man","Color:White"]},{"name":"Nike Boy's Jordan Stretch T-shirts","url":"https://www.klarna.com/us/shopping/pl/cl359/3201863202/Children-s-Clothing/Nike-Boy-s-Jordan-Stretch-T-shirts/?source=openai","price":"$14.99","attributes":["Color:White,Green","Model:Boy","Pattern:Solid Color","Size (Small-Large):S,XL,L,M"]},{"name":"Polo Classic Fit Cotton V-Neck T-Shirts 3-Pack","url":"https://www.klarna.com/us/shopping/pl/cl10001/3203028500/Clothing/Polo-Classic-Fit-Cotton-V-Neck-T-Shirts-3-Pack/?source=openai","price":"$29.95","attributes":["Material:Cotton","Target Group:Man","Color:White,Blue,Black"]},{"name":"adidas Comfort T-shirts Men's 3-pack","url":"https://www.klarna.com/us/shopping/pl/cl10001/3202640533/Clothing/adidas-Comfort-T-shirts-Men-s-3-pack/?source=openai","price":"$14.99","attributes":["Material:Cotton","Target Group:Man","Color:White,Black","Pattern:Solid Color"]}]}
Thought:These are the t shirts available on Klarna:
- Lacoste Men's Pack of Plain T-Shirts
- Hanes Men's Ultimate 6pk. Crewneck T-Shirts
- Nike Boy's Jordan Stretch T-shirts
- Polo Classic Fit Cotton V-Neck T-Shirts 3-Pack
- adidas Comfort T-shirts Men's 3-pack
Final Answer: The t shirts available on Klarna are Lacoste Men's Pack of Plain T-Shirts, Hanes Men's Ultimate 6pk. Crewneck T-Shirts, Nike Boy's Jordan Stretch T-shirts, Polo Classic Fit Cotton V-Neck T-Shirts 3-Pack, and adidas Comfort T-shirts Men's 3-pack.
> Finished chain.
'The t shirts available on Klarna are Lacoste Men's Pack of Plain T-Shirts, Hanes Men's Ultimate 6pk. Crewneck T-Shirts, Nike Boy's Jordan Stretch T-shirts, Polo Classic Fit Cotton V-Neck T-Shirts 3-Pack, and adidas Comfort T-shirts Men's 3-pack.
質問 : klarnaで入手できるTシャツは何ですか?
応答 : klanaで販売しているTシャツは、Lacoste Men's Pack of Plain T-Shirts、Hanes Men's Ultimate 6pk. Crewneck T-Shirts、Nike Boy's Jordan Stretch T-Shirts、Polo Classic Fit Cotton V-Neck T-Shirts 3-Pack、adidas Comfort T-shirts Men's 3-Packです。
関連
この記事が気に入ったらサポートをしてみませんか?