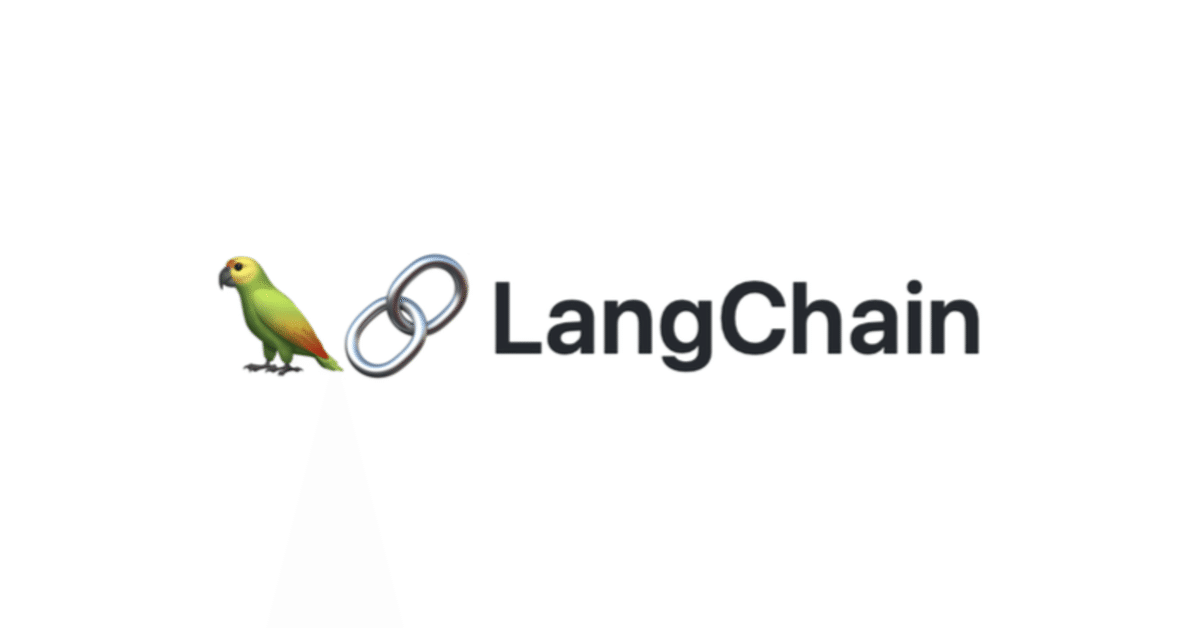
LangChain クイックスタートガイド - Python版
Python版の「LangChain」のクイックスタートガイドをまとめました。
・LangChain v0.0.89
【最新版の情報は以下で紹介】
1. LangChain
「LangChain」は、「大規模言語モデル」 (LLM : Large language models) と連携するアプリの開発を支援するライブラリです。
「LLM」という革新的テクノロジーによって、開発者は今まで不可能だったことが可能になりました。しかし、「LLM」を単独で使用するだけでは、真に強力なアプリケーションを作成するのに不十分です。本当の力は、それを他の計算や知識と組み合わせた時にもたらされます。「LangChain」は、そのようなアプリケーションの開発をサポートします。
主な用途は、次の3つになります。
2. インストール
Google Colabでのインストール手順は、次のとおりです。
(1) パッケージのインストール。
# パッケージのインストール
!pip install langchain
!pip install openai
(2) 環境変数の準備。
以下のコードの <OpenAI_APIのトークン> にはOpenAI APIのトークンを指定します。(有料)
# 環境変数の準備
import os
os.environ["OPENAI_API_KEY"] = "<OpenAI_APIのトークン>"
3. LangChain のモジュール
「LangChain」は、言語モデル アプリケーションの構築に使用できる多くのモジュールを提供します。モジュールを組み合わせて複雑なアプリケーションを作成したり、個別に使用したりできます。
主なモジュールは、次のとおりです。
・LLM : 言語モデルによる推論の実行。
・プロンプトテンプレート : ユーザー入力からのプロンプトの生成。
・チェーン : 複数のLLMやプロンプトの入出力を繋げる。
・エージェント : ユーザー入力を元にしたチェーンの動的呼び出し。
・メモリ : チェーンやエージェントで状態を保持。
4. LLM
「LangChain」の最も基本的な機能は、LLMを呼び出すことです。言語モデルによる推論を実行します。
今回は例として、ユーザー入力「コンピュータゲームを作る日本語の新会社名をを1つ提案してください」でLLMを呼び出します。
from langchain.llms import OpenAI
# LLMの準備
llm = OpenAI(temperature=0.9)
# LLMの呼び出し
print(llm("コンピュータゲームを作る日本語の新会社名をを1つ提案してください"))
ツクルゲームス
「LLM」の使い方について詳しくは、「LLM入門ガイド」を参照してください。
5. プロンプトテンプレート
「プロンプトテンプレート」は、ユーザー入力からプロンプトを生成するためのテンプレートです。アプリケーションで LLM を使用する場合、通常、ユーザー入力を直接LLM に渡すことはありません。ユーザー入力を基に「プロンプトテンプレート」でプロンプトを作成して、それをLLMに渡します。
今回は例として、ユーザー入力「作るもの」を基に「○○を作る日本語の新会社名をを1つ提案してください」というプロンプトを生成します。
from langchain.prompts import PromptTemplate
from langchain.chains import LLMChain
# プロンプトテンプレートの準備
prompt = PromptTemplate(
input_variables=["product"],
template="{product}を作る日本語の新会社名をを1つ提案してください",
)
# プロンプトの生成
print(prompt.format(product="家庭用ロボット"))
家庭用ロボットを作る日本語の新会社名をを1つ提案してください
「プロンプトテンプレート」の使い方について詳しくは、「プロンプトテンプレート入門ガイド」を参照してください。
6. チェーン
これまで、「LLM」や「プロンプトテンプレート」を単独で使用してきましたが、実際のアプリケーションでは、それらの組み合わせて使います。「チェーン」を使うことで、複数のLLMやプロンプトの入出力も繋げることができます。
「チェーン」は、LLMなどの「プリミティブ」または他の「チェーン」で構成され、最も基本的な「LLMChain」は「LLM」と「プロンプトテンプレート」で構成されます。
今回は例として、ユーザー入力「作るもの」を基に「○○を作る日本語の新会社名」を生成します。
from langchain.chains import LLMChain
from langchain.llms import OpenAI
from langchain.prompts import PromptTemplate
# LLMの準備
llm = OpenAI(temperature=0.9)
# プロンプトテンプレートの準備
prompt = PromptTemplate(
input_variables=["product"],
template="{product}を作る日本語の新会社名をを1つ提案してください",
)
# チェーンの準備
chain = LLMChain(llm=llm, prompt=prompt)
# チェーンの実行
chain.run("家庭用ロボット")
ポケットロボットカンパニー
「チェーン」の使い方について詳しくは、「チェーン入門ガイド」を参照してください。
7. エージェント
「チェーン」はあらかじめ決められた順序で実行しますが、「エージェント」はLLMによって「実行するアクション」とその「順序」を決定します。
アクションは、「ツールを実行してその出力を観察」または「ユーザーに戻る」のいずれか、「ツール」は、Google検索などの特定の機能のことになります。
・エージェント : 実行するアクションと順番を決定
・アクション : ツールを実行してその出力を観察 or ユーザーに戻る
・ツール : 特定の機能
今回は例として、次の2つのツールを使うエージェントを作成します。
・serpapi : Google検索
・llm-math : 数学の計算
(1) パッケージのインストール。
「serpapi」を使うには「google-search-results」が必要になります。
# パッケージのインストール
!pip install google-search-results
(2) 環境変数の準備。
以下のコードの <SerpAPIのトークン> にはSerpAPIのトークン、を指定します。
# 環境変数の準備
import os
os.environ["SERPAPI_API_KEY"] = "<SerpAPIのトークン>"
(3) エージェントの準備。
from langchain.agents import load_tools
from langchain.agents import initialize_agent
from langchain.llms import OpenAI
# LLMの準備
llm = OpenAI(temperature=0)
# ツールの準備
tools = load_tools(["serpapi", "llm-math"], llm=llm)
# エージェントの準備
agent = initialize_agent(
tools,
llm,
agent="zero-shot-react-description",
verbose=True
)
(4) エージェントの実行。
「llm-math」が役立ちそうな質問をします。
# エージェントの実行
agent.run("富士山の高さは?それに2を掛けると?")
> Entering new AgentExecutor chain...
I need to find the height of Mt. Fuji and then multiply it by two.
Action: Search
Action Input: "Mt. Fuji height"
Observation: Japan’s Mt. Fuji is an active volcano about 100 kilometers southwest of Tokyo. Commonly called “Fuji-san,” it’s the country’s tallest peak, at 3,776 meters. A pilgrimage site for centuries, it’s considered one of Japan’s 3 sacred mountains, and summit hikes remain a popular activity. Its iconic profile is the subject of numerous works of art, notably Edo Period prints by Hokusai and Hiroshige. ― Google
Thought: I now know the height of Mt. Fuji
Action: Calculator
Action Input: 3776 * 2
Observation: Answer: 7552
Thought: I now know the final answer
Final Answer: Mt. Fuji's height is 3776 meters, and when multiplied by two, it is 7552 meters.
> Finished chain.
'Mt. Fuji's height is 3776 meters, and when multiplied by two, it is 7552 meters.
英語ではありますが、正解です。
(4) エージェントの実行。
「serpapi」が役立ちそうな質問をします。
# エージェントの実行
agent.run("ぼっち・ざ・ろっく!の作者の名前は?")
> Entering new AgentExecutor chain...
I need to find out who the author of this manga is.
Action: Search
Action Input: "ぼっち・ざ・ろっく!" author
Observation: Aki Hamaji
Thought: I now know the final answer
Final Answer: Aki Hamaji
> Finished chain.
'Aki Hamaji
英語ではありますが、正解です。
「エージェント」の使い方について詳しくは、「エージェント入門ガイド」を参照してください。
8. メモリ
これまでの「チェーン」や「エージェント」はステートレスでしたが、「メモリ」を使うことで、「チェーン」や「エージェント」で過去の会話のやり取りを記憶することができます。過去の会話の記憶を使って、会話することができます。
(1) ConversationChainの準備。
「ConversationChain」は会話用のチェーンで、メモリの機能を持ちます。
from langchain import OpenAI, ConversationChain
# ConversationChainの準備
chain = ConversationChain(
llm=OpenAI(temperature=0),
verbose=True
)
# 会話の実行
chain.predict(input="こんにちは!")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
Human: こんにちは!
AI:
> Finished ConversationChain chain.
' こんにちは!私はAIです。どうぞよろしくお願いします!'
(2) 会話の実行。
# 会話の実行
chain.predict(input="AIさんの好きな料理は?")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
Human: こんにちは!
AI: こんにちは!私はAIです。どうぞよろしくお願いします!
Human: AIさんの好きな料理は?
AI:
> Finished chain.
' 私の好きな料理は、和食です。特に、お寿司が大好きです!
(3) 会話の実行。
# 会話の実行
chain.predict(input="その料理の作り方は?")
> Entering new ConversationChain chain...
Prompt after formatting:
The following is a friendly conversation between a human and an AI. The AI is talkative and provides lots of specific details from its context. If the AI does not know the answer to a question, it truthfully says it does not know.
Current conversation:
Human: こんにちは!
AI: こんにちは!私はAIです。どうぞよろしくお願いします!
Human: AIさんの好きな料理は?
AI: 私の好きな料理は、和食です。特に、お寿司が大好きです!
Human: その料理の作り方は?
AI:
> Finished chain.
' お寿司の作り方は、お米を炊いて、お醤油とお砂糖を混ぜて、お米にかけて、お魚や野菜などをのせて、巻いて完成です!
「メモリ」の使い方について詳しくは、「メモリ入門ガイド」を参照してください。
関連
・LangChain Integrations
・LangChain Hub
・Text Splitter Playground
この記事が気に入ったらサポートをしてみませんか?