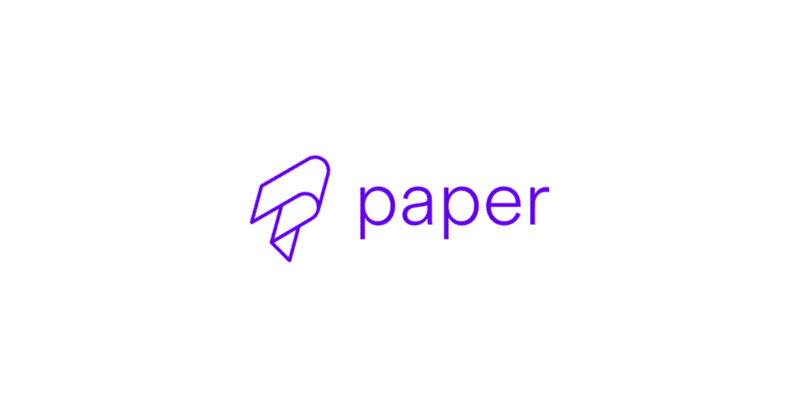
React Native Paper の使い方
「React Native Paper」の使い方をまとめました。
前回
1. React Native Paper
「React Native Paper」は、「React Native」のUIライブラリのひとつです。デフォルトでGoogleのマテリアルデザインガイドラインに準拠します。
2. React Native Paper の使い方
「React Native Paper」の使い方の手順は次のとおりです。
2-1. セットアップ
(1) React Nativeプロジェクトの生成。
npx react-native init my_app
cd my_app
(2) パッケージのインストール。
npm install react-native-paper
npm install react-native-safe-area-context
npm install react-native-vector-icons
npm install @react-native-community/masked-view
2-2. Androidのセットアップ
(1) android/app/build.gradle(NOT android/build.gradle)に以下の設定を追加。
apply from: file("../../node_modules/react-native-vector-icons/fonts.gradle")
詳しくは、こちらを参照。
2-3. iOSのセットアップ
(1) podのインストール。
cd ios
pod install
cd ..
(2) workspaceを開き、署名し、iPhoneにインストールできることを確認。
(3) 「Info.plist」に以下の項目を追加。
<key>UIAppFonts</key>
<array>
<string>AntDesign.ttf</string>
<string>Entypo.ttf</string>
<string>EvilIcons.ttf</string>
<string>Feather.ttf</string>
<string>FontAwesome.ttf</string>
<string>FontAwesome5_Brands.ttf</string>
<string>FontAwesome5_Regular.ttf</string>
<string>FontAwesome5_Solid.ttf</string>
<string>FontAwesome6_Brands.ttf</string>
<string>FontAwesome6_Regular.ttf</string>
<string>FontAwesome6_Solid.ttf</string>
<string>Foundation.ttf</string>
<string>Ionicons.ttf</string>
<string>MaterialIcons.ttf</string>
<string>MaterialCommunityIcons.ttf</string>
<string>SimpleLineIcons.ttf</string>
<string>Octicons.ttf</string>
<string>Zocial.ttf</string>
<string>Fontisto.ttf</string>
</array>
詳しくは、こちらを参照。
2-4. コードの編集と実行
(1) PaperProviderの追加。
「PaperProvider」でルートコンポーネントをラップします。React Nativeプロジェクトがある場合は、AppRegistry.registerComponent に渡されるコンポーネントに追加することが推奨されています。
・index.js
import { AppRegistry } from 'react-native';
import { name as appName } from './app.json';
import { Provider as PaperProvider } from 'react-native-paper';
import App from './App';
// ホームスクリーン
const Main = () => (
<PaperProvider>
<App />
</PaperProvider>
);
AppRegistry.registerComponent(appName, () => Main);
(2) コードの編集と実行。
・App.tsx
import * as React from 'react';
import { Button, Text } from 'react-native-paper';
import { SafeAreaView, StyleSheet } from 'react-native';
// ホームスクリーン
const App: React.FC = () => (
<SafeAreaView style={styles.container}>
<Text style={styles.text}>Hello!</Text>
<Button icon="camera" mode="contained" onPress={() => console.log('Pressed')}>
Press me
</Button>
</SafeAreaView>
);
// スタイルシート
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 24,
marginBottom: 20,
},
});
export default App;
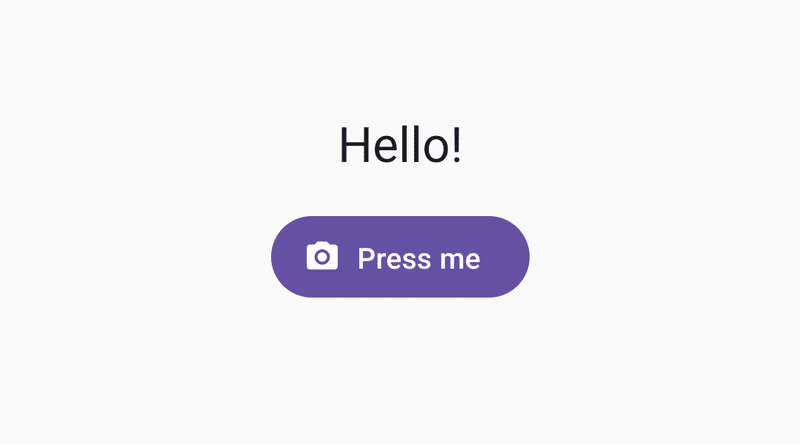
3. ナビゲーションの実装
(1) パッケージのインストール。
npm install @react-navigation/native
npm install @react-navigation/stack
npm install react-native-screens
npm install react-native-gesture-handler
・@react-navigation/native : React Navigationのコアパッケージ
・@react-navigation/stack : Stack Navigationのパッケージ
・react-native-screens : React Navigationのパフォーマンス向上
・react-native-gesture-handler : タッチイベント
(2) コードの編集と実行。
import * as React from 'react';
import { Button, Text } from 'react-native-paper';
import { SafeAreaView, StyleSheet } from 'react-native';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator, CardStyleInterpolators, StackNavigationProp } from '@react-navigation/stack';
// Stack Natigationのパラメータ一覧
type RootStackParamList = {
Home: undefined;
Details: undefined;
};
// ホームスクリーンのナビゲーションプロパティ
type HomeScreenNavigationProp = StackNavigationProp<RootStackParamList, 'Home'>;
// ホームスクリーンのプロパティ
type HomeScreenProps = {
navigation: HomeScreenNavigationProp;
};
// ホームスクリーン
const HomeScreen: React.FC<HomeScreenProps> = ({ navigation }) => (
<SafeAreaView style={styles.container}>
<Text style={styles.text}>Hello!</Text>
<Button
icon="camera"
mode="contained"
onPress={() => navigation.navigate('Details')}
>
Next
</Button>
</SafeAreaView>
);
// 詳細スクリーン
const DetailsScreen: React.FC = () => (
<SafeAreaView style={styles.container}>
<Text style={styles.text}>Details Screen</Text>
</SafeAreaView>
);
// スタック
const Stack = createStackNavigator();
const App: React.FC = () => (
<NavigationContainer>
<Stack.Navigator
initialRouteName="Home"
screenOptions={{
gestureEnabled: true,
gestureDirection: 'horizontal',
cardStyleInterpolator: CardStyleInterpolators.forHorizontalIOS,
}}>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
// スタイルシート
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 24,
marginBottom: 20,
},
});
export default App;
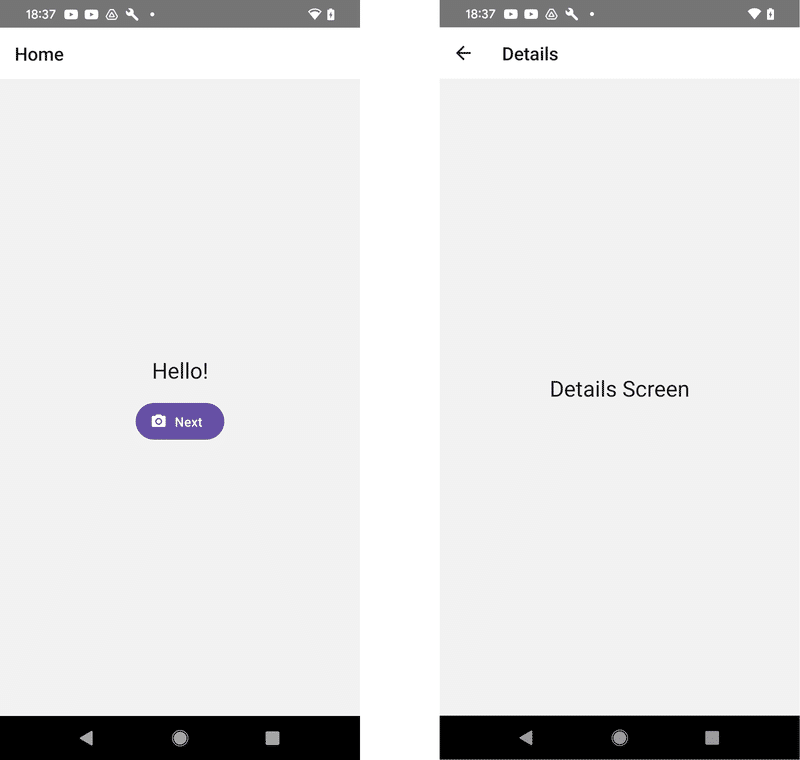
【おまけ】 テーマのプロパティ一覧
「react-native-paper」のテーマのプロパティ一覧は、以下で確認できます。
・dark (boolean): darkテーマかlightテーマか
・version: アプリ内でどのデザインシステムコンポーネントに従うか
・3 - new Material You(MD3)
・2 - previous Material Design (MD2)
・mode ('adaptive' | 'exact'): darkテーマのカラーモード
・roundness (number): ボタンなどの共通要素の丸み
・colors (object): さまざまな要素で使用されるさまざまな色
・primary key color: UI全体の主要コンポーネント
・primary
・onPrimary
・primaryContainer
・onPrimaryContainer
・secondary key color: UI内の目立たないコンポーネント
・secondary
・onSecondary
・secondaryContainer
・onSecondaryContainer
・teriary key color: バランス・アクセント
・tertiary
・onTertiary
・tertiaryContainer
・onTertiaryContainer
・neutral key color: 表面・背景
・background
・onBackground
・surface
・onSurface
・neutral variant key color: 中程度の強調テキストとアイコンなど
・surfaceVariant
・onSurfaceVariant
・outline
・error: エラー
・error
・onError
・errorContainer
・onErrorContainer
・elevation (object): 基本色に基づいたカラーオーバーレイ
・level0- 透明
・level1- 不透明度 5%
・level2- 不透明度 8%
・level3- 不透明度 11%
・level4- 不透明度 12%
・level5- 不透明度 14%
・disabled state: 無効
・surfaceDisabled
・onSurfaceDisabled
・additional role mappings: 追加ロールマッピング
・shadow
・inverseOnSurface
・inverseSurface
・inversePrimary
・backdrop
・fonts (object): フォントスタイル
・[variant例labelMedium] (object):
・fontFamily
・letterSpacing
・fontWeight
・lineHeight
・fontSize
・animation (object):
・scale
【おまけ】 コンポーネント一覧
「react-native-paper」のコンポーネント一覧は、以下で確認できます。
・ActivityIndicator
・Appbar
・Avatar
・Badge
・Banner
・BottomNavigation
・Button
・Card
・Checkbox
・Chip
・DataTable
・Dialog
・Divider
・Drawer
・FAB
・HelperText
・Icon
・IconButton
・List
・Menu
・Modal
・Portal
・ProgressBar
・RadioButton
・Searchbar
・SegmentedButtons
・Snackbar
・Surface
・Switch
・Text
・TextInput
・ToggleButton
・Tooltip
・TouchableRipple
【おまけ】 アイコン一覧
「react-native-vector-icons」のアイコン一覧は、以下で確認できます。
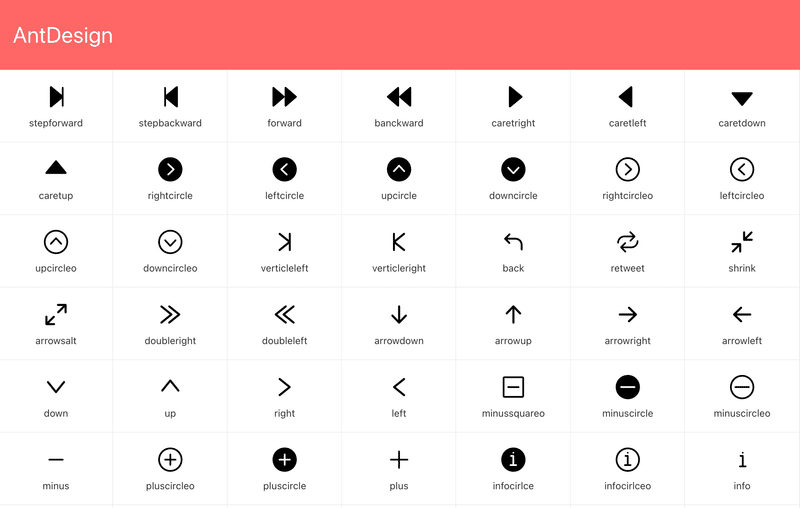
【おまけ】 推奨ライブラリ
公式で推奨されているライブラリは、次のとおりです。
・Tabs
・react-native-community/react-native-tab-view
・web-ridge/react-native-paper-tabs
・Bottom sheet
・osdnk/reanimated-bottom-sheet
・gorhom/react-native-bottom-sheet
・Date Picker
・web-ridge/react-native-paper-dates
・react-native-community/react-native-datetimepicker
・Time Picker
・System Colors
この記事が気に入ったらサポートをしてみませんか?