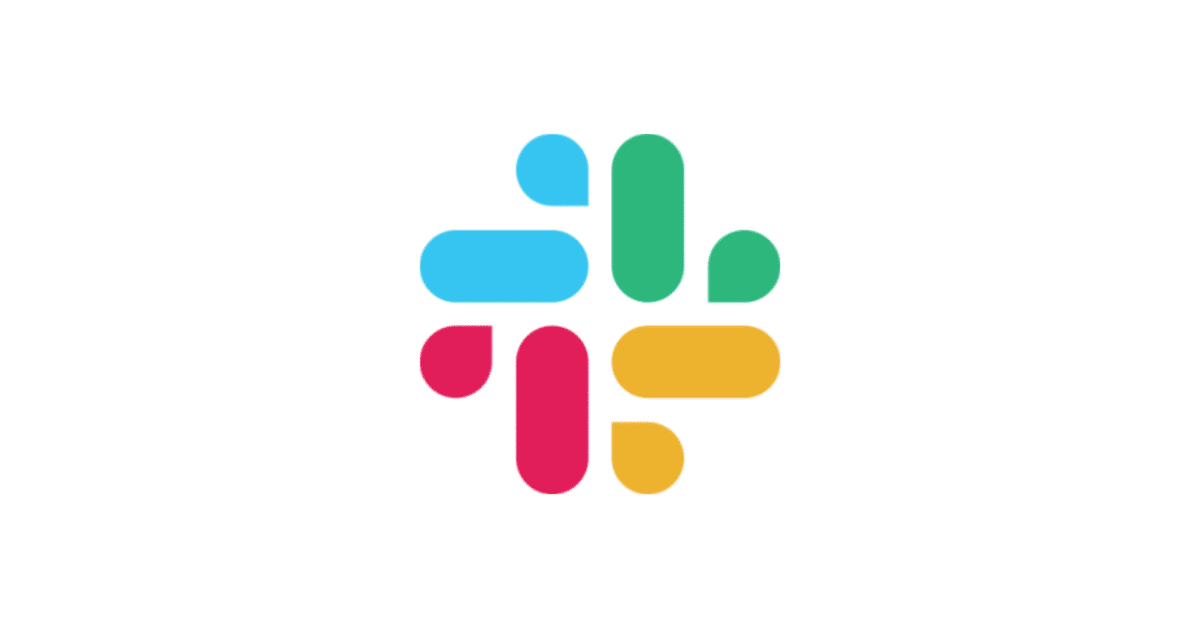
Photo by
eisukeai
【Google Colab】Slackで特定の絵文字がどのくらい使われているかカウントしてみた!!
社内で使用されているMissionやValueを示すカスタム絵文字がどのくらい使われているかを調べたいと思ったことがあり、Pythonを使って調べました。
has::eyes: みたいな感じでhas:オプションを使うと全ての投稿を取得し、if文などで制御しなくて済むことが分かりました。
セルの1個目 SDKのインストール
"""
ファイルを開いたら必ず実行が必要
実行前に!pip install slack_sdkを実行してください。
セッションが切れると無効になってしまいます。
"""
!pip install slack_sdk
!pip install gspread
セルの2個目 Google Spreadsheetとの連携
# Google Spreadsheetにアクセスするための認証を付与する
from google.colab import auth
auth.authenticate_user()
import gspread
from google.auth import default
creds, _ = default()
gc = gspread.authorize(creds)
セルの3個目 カウントと転記
"""
NOTICE:
もし、SLACK_USER_OAUTH_TOKEN が設定されていません。が表示された場合は、左の鍵アイコンをクリック
Slack APIのxoxp-やxoxb-から始まるトークンをコピーしてください。
https://api.slack.com/
1. 上記URLにアクセス
2. Your Appsをクリック
3. 左サイドバーのOAuth & Permissions
emoji:read
chat:write
channels:history
reactions:read
search:read
などを追加してください
"""
import os
import re
from slack_sdk import WebClient
from slack_sdk.errors import SlackApiError
from datetime import datetime
from google.colab import userdata #Google Colab上で設定したAPI_KEYなどを読み込む
def convert_timestamp(timestamp):
# UNIXタイムスタンプをdatetimeオブジェクトに変換
dt_object = datetime.fromtimestamp(float(timestamp))
# datetimeオブジェクトを指定の形式に変換
formatted_time = dt_object.strftime('%Y/%m/%d %H:%M:%S')
return formatted_time
def get_slack_messages(custom_emoji):
"""
Slack APIからメッセージを取得し、絵文字に関連するメッセージ情報をリストとして返す
"""
# Slack Bot User OAuth Tokenを設定します (Slackアプリから取得)
slack_token = userdata.get("SLACK_USER_OAUTH_TOKEN")
if not slack_token:
raise ValueError("SLACK_USER_OAUTH_TOKEN が設定されていません。")
# WebClientインスタンスを作成します
client = WebClient(token=slack_token)
new_values = []
try:
# search.messages APIメソッドを呼び出します
response = client.search_messages(
query = f"has:{custom_emoji}" # カスタム絵文字を含むメッセージを検索します
)
# 検索結果を表示します
messages = response["messages"]["matches"]
if not messages:
print("一致するメッセージが見つかりませんでした。")
else:
for message in messages:
# Botのメッセージを除外
if message.get('user') is None:
continue # user が None の場合は次のループへ
# 絵文字のリアクションの数を取得
emoji_count = get_reaction_count(client, message['channel']['id'], message['ts'], custom_emoji)
new_values.append([
message['ts'],
convert_timestamp(message['ts']),
message['channel']['id'],
message['channel']['name'],
message['user'],
message['username'],
message['text'],
custom_emoji,
emoji_count
])
except SlackApiError as e:
# エラーが発生した場合は詳細を表示します
print(f"Error: {e.response}") # エラーレスポンス全体を表示
return new_values
def get_reaction_count(client, channel_id, timestamp, emoji):
"""
メッセージに付けられた特定の絵文字のリアクションの数を取得します
"""
try:
response = client.reactions_get(
channel=channel_id,
timestamp=timestamp
)
print(f"response.status_code: {response.status_code}")
print(f"{response}")
# 全てのスタンプが一覧で取得出来る
reactions = response['message'].get('reactions', [])
print(f"reactions: {reactions}\n")
# 絵文字の : を削除
emoji = re.sub(":", "", emoji)
for reaction in reactions:
if reaction['name'] == emoji:
return reaction['count']
except SlackApiError as e:
print(f"Error: {e.response}") # エラーレスポンス全体を表示
return 0
def set_new_values_to_sheet(new_values):
"""
Google SpreadheetにSlack APIから取得した全てのメッセージを転記する
"""
# URLからSpreadsheetを開く
url = "https://doc.google.com/spreadsheets/d/*********************"
spreadsheet = gc.open_by_url(url)
print(f"\nspreadsheet_name: {spreadsheet.title}\n")
# 転記先のシートを指定
sheet = spreadsheet.worksheet("シート1")
# 最終行を取得
last_row = len(sheet.get_all_values()) + 1
print(f"last_row: {last_row}\n")
# 新しい値を貼り付け
sheet.update(new_values, f"A{last_row}")
print("転記完了")
def main():
# カスタム絵文字
custom_emoji = ":snake:"
# Slack APIからメッセージを取得
new_values = get_slack_messages(custom_emoji)
# Google Spreadheetに転記
set_new_values_to_sheet(new_values)
if __name__ == "__main__":
main()
この記事が気に入ったらサポートをしてみませんか?