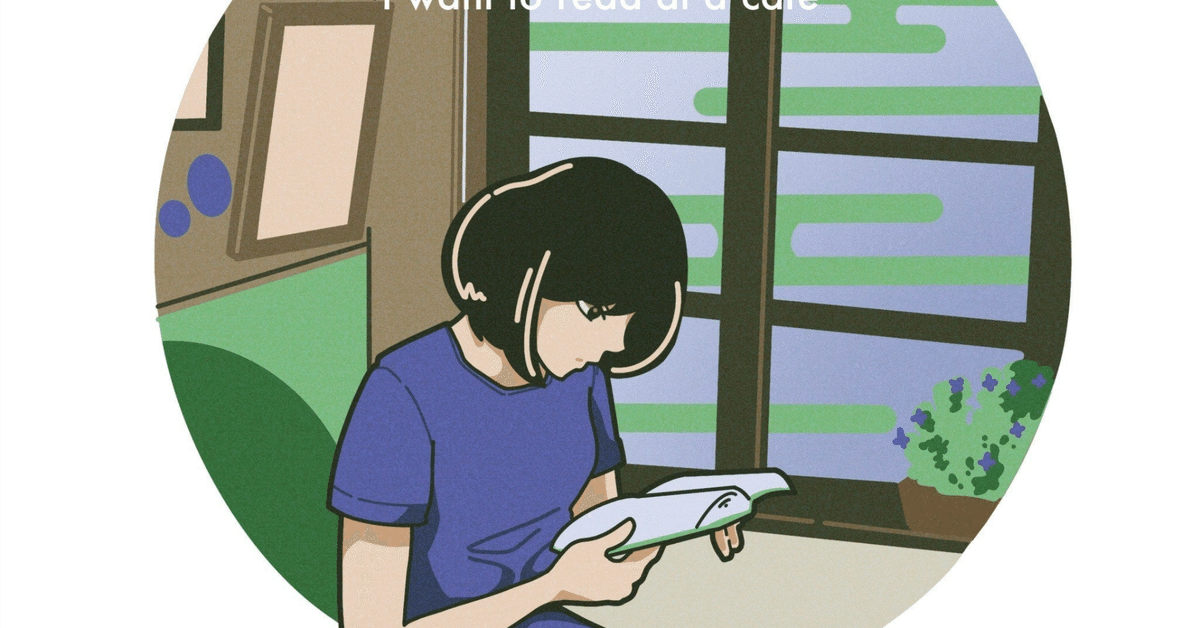
Photo by
msy03
1分ごとにBinanceの全銘柄の終値を記録する
どうも
愚者の戦略です
今回はバイナンスの全銘柄について、1分おきに終値をCSVファイルへ記録するプログラムを作ったので公開します。
import ccxt
import os
import time
import datetime
import pandas as pd
binance = ccxt.binance()
df = "NaN"
cycle = 0
def read_crypt_pricedata():
try:
# CSV読み込み
df = pd.read_csv('BinanceAllCryptPriceData.csv', index_col=0)
except:
print("BinanceAllCryptPriceData.csvが見つかりませんでした")
return df
def get_all_crypt_pricedata():
try:
AllCryptPriceData = binance.fetchTickers()
current_price_df = pd.DataFrame(AllCryptPriceData)
# closeのみ抽出
current_price_df = pd.DataFrame(current_price_df.loc["close"])
# 行列反転
current_price_df = current_price_df.T
except:
print("Binanceから価格情報を取得できませんでした")
return current_price_df
def add_current_price(df, current_price_df):
# 今日の日付で行追加
try:
# 日時取得
d_today = datetime.datetime.now()
df.loc[d_today] = "NaN"
except:
print("日時情報を取得できませんでした")
symbolList = current_price_df.columns
for symbol in symbolList:
if symbol in df.columns:
# csvに該当シンボルがある
df.loc[d_today, symbol] = current_price_df.loc["close", symbol]
else:
# csvに該当シンボルが無い(新規上場)
df[symbol] = "NaN"
df.loc[d_today, symbol] = current_price_df.loc["close", symbol]
return df
def write_crypt_pricedata(df):
try:
# CSV読み込み
df.to_csv("BinanceAllCryptPriceData.csv")
except:
print("BinanceAllCryptPriceData.csvに書き込めませんでした")
while True:
cycle += 1
start_time = time.perf_counter()
if os.path.exists('BinanceAllCryptPriceData.csv'):
df = read_crypt_pricedata()
current_price_df = get_all_crypt_pricedata()
df = add_current_price(df, current_price_df)
write_crypt_pricedata(df)
else:
# 初回起動時用
df = get_all_crypt_pricedata()
df.drop('close', axis=0)
df.set_axis([datetime.datetime.now()], axis=0, inplace=True)
write_crypt_pricedata(df)
end_time = time.perf_counter()
elapsed_time = end_time - start_time
print(datetime.datetime.now(), "の価格を取得しました")
if elapsed_time >= 60:
elapsed_time = 50
time.sleep(60 - elapsed_time)
チップありがとうございます!超嬉しいです。