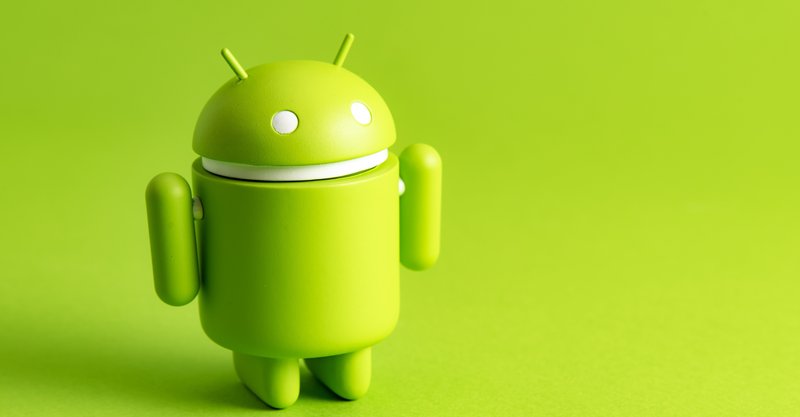
Photo by
ibaraki_nakai
Androidアプリ【Java】5.Spinner作成
↓
今回は上記のような住所の登録ページで都道府県の右側にある矢印を押下すると、リストがダイアログとして表示されるものを作成。
1.レイアウトファイル作成
サンプルのようなリストを作成するためにはSpinnerというViewを作成する
res/layout/activity_main.xml
<Spinner
android:paddingTop="40dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View2"
android:spinnerMode="dialog"
android:entries="@array/list"
android:paddingLeft="25dp"
android:id="@+id/View3"
style="@style/Widget.AppCompat.Spinner.Underlined"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
/>
android:spinnerMode="dialog"
sipnnerModeを設定することでdialogやpulldownといった表示形式を指定することが出来る。
android:entries="@array/list"
ここで指定している"@array/list"はres/values/stylesに記載した文字列(今回でいうと都道府県の一覧)を指す。
2.stylesに文字列を追加
res/values/stylesに文字列を追加。
↓
res/values/styles
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
<string-array name="list">
<item>都道府県</item>
<item>北海道</item>
<item>青森県</item>
<item>岩手県</item>
<item>宮城県</item>
<item>秋田県</item>
<item>山形県</item>
<item>福島県</item>
</string-array>
</resources>
追加した部分は<string-array>タグの部分。
これで先ほどのリストが完成する。
コード全文
res/layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
android:elevation="20dp"
android:background="#f5f5f5">
<TextView
android:textColor="#000000"
android:layout_height="match_parent"
android:layout_width="match_parent"
android:text="住所の登録"/>
</androidx.appcompat.widget.Toolbar>
<TextView
android:textSize="17dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="住所"
android:id="@+id/View1"
app:layout_constraintLeft_toLeftOf="parent"
android:layout_margin="20dp"
app:layout_constraintTop_toBottomOf="@id/toolbar"
android:textColor="@color/colorPrimary"/>
<EditText
android:paddingTop="40dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View1"
android:id="@+id/View2"
android:hint="郵便番号(数字)"
android:paddingLeft="30dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
/>
<Spinner
android:paddingTop="40dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View2"
android:spinnerMode="dialog"
android:entries="@array/list"
android:paddingLeft="25dp"
android:id="@+id/View3"
style="@style/Widget.AppCompat.Spinner.Underlined"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View3"
android:id="@+id/View4"
android:paddingTop="40dp"
android:hint="市区町村"
android:paddingLeft="30dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View4"
android:id="@+id/View5"
android:paddingTop="40dp"
android:hint="番地"
android:paddingLeft="30dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View5"
android:id="@+id/View6"
android:paddingTop="40dp"
android:hint="建物名(任意)"
android:paddingLeft="30dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View6"
android:id="@+id/View7"
android:paddingTop="40dp"
android:hint="電話番号"
android:paddingLeft="30dp"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@+id/View7"
android:id="@+id/Button1"
android:layout_marginTop="40dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:text="登録する"
android:textColor="#ffffff"
android:background="@drawable/shape_rounded"
/>
</androidx.constraintlayout.widget.ConstraintLayout>
Java/android.example.mongoose/MainActivity
package android.example.mongoose_app;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import androidx.appcompat.widget.Toolbar;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final Toolbar toolbar = findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
}
}
私も皆さんと少しづつ成長していきたくこういう形でアウトプットさせていただいております。一つのリアクションでかなりの励みになりますのでどうかよろしくお願いします。