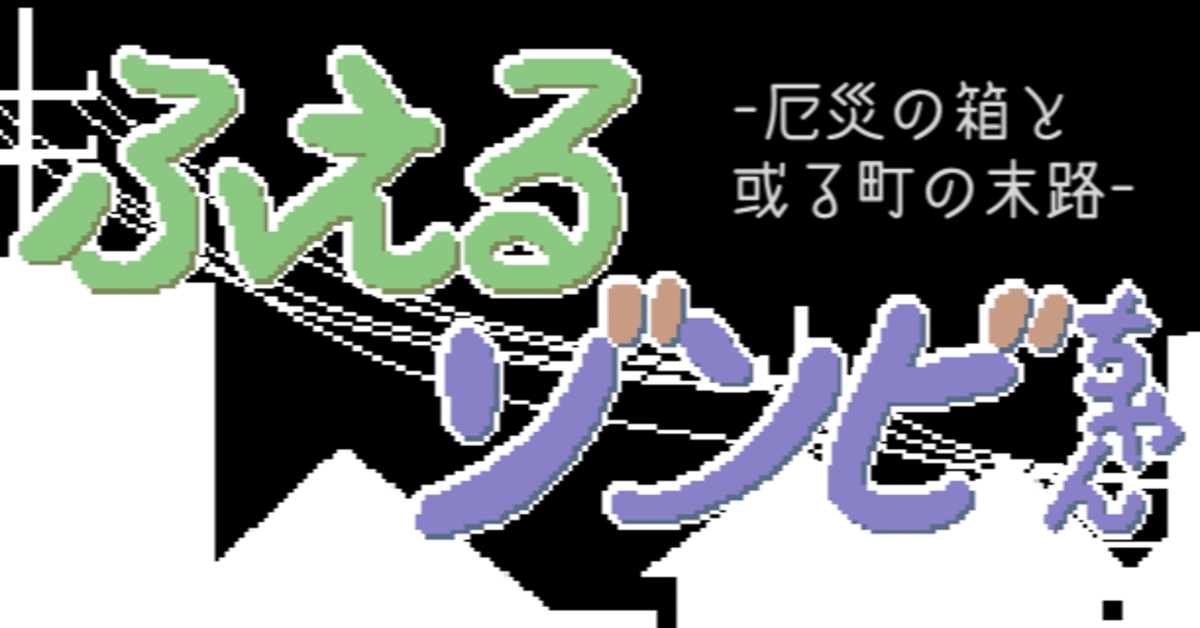
[Unity]ふえるゾンビちゃんの中身ちょっとだけ見せる[スクリプト公開]
https://unityroom.com/games/thevoodoobox
2020年8月10日〜8月16日のunity1week お題「ふえる」に投稿したゲーム「ふえるゾンビちゃん」です。
今回のunity1weekではスクリプト見せ合いっこの流れが来ているので、便乗して「ゾンビの移動クラス」「ゾンビ達のまとめクラス」を見せてみようと思います。
(ゲーム管理クラス・UI管理クラスは中身がごちゃっとしすぎているので今回は勘弁させていただく……)
AlgoEnemy(それぞれのゾンビの移動を担当)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using DG.Tweening;
using UnityEngine.Tilemaps;
public class AlgoEnemy : MonoBehaviour
{
public int x;
public int y;
public bool canMove;
private GameManager gameM;
public Tilemap map;
[SerializeField]
public Vector3Int enePos;
// Start is called before the first frame update
void Start(){
//位置をセット
this.gameObject.transform.position = new Vector3(enePos.x, enePos.y,enePos.y);
}
// 実行用
public void Execute(Vector3Int shuPos){
Move(shuPos);
}
// 移動処理
public void Move( Vector3Int shuPos) {
Vector3Int newpos = new Vector3Int(enePos.x + x, enePos.y + y, (enePos.y + y));
Vector3Int xyOnly = new Vector3Int(enePos.x + x, enePos.y + y, 0);
// 壁判定
Tile.ColliderType type = map.GetColliderType(xyOnly);
// 壁判定で引き返す
if (type != Tile.ColliderType.None)
{
x = x * -1;
y = y * -1;
newpos = new Vector3Int(enePos.x + x, enePos.y + y, (enePos.y + y));
xyOnly = new Vector3Int(enePos.x + x, enePos.y + y, 0);
}
// 両側を壁に挟まれてないか?
type = map.GetColliderType(xyOnly);
if (type == Tile.ColliderType.None)
{
canMove = true;
}
else
{
canMove = false;
}
// 移動先に主人公がいないか?
if (canMove) {
if (!(shuPos.x == newpos.x && shuPos.y == newpos.y))
{
canMove = true;
}
else
{
canMove = false;
}
}
if (canMove) {
// 本体の移動
this.gameObject.transform.DOMove(newpos, 0.1f).SetEase(Ease.Flash);
enePos = newpos;
}
}
}
見直し感想:
publicにしなくて良いもの多すぎ。あともう要らないデバッグ文残しすぎ。
ここに載せるために要らないコメントとか消し込んでたら全く使ってないメソッド出てきましたし……
EnemyManager(ゾンビをまとめて制御)
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Tilemaps;
public class EnemyManager : MonoBehaviour
{
private GameManager gameM;
private Tilemap map;
public List<AlgoEnemy> enemyList;
public GameObject prefabZombi_A;
public GameObject prefabZombi_B;
private int addZombiNum;
// Start is called before the first frame update
void Start()
{
gameM = GameObject.FindWithTag("GameManager").GetComponent<GameManager>();
map = gameM.map;
}
// 実行用
public void Execute()
{
for (int i = 0; i< (enemyList.Count - addZombiNum); i++) {
enemyList[i].Execute(gameM.newpos);
}
addZombiNum = 0;
}
//ゾンビを増やす
public void AddZombi() {
addZombiNum = 0;
int temp = enemyList.Count;
for (int i = 0; i < temp ; i++)
{
GameObject prefab = null;
if (enemyList[i].x == 0){
prefab = prefabZombi_A;
}
else {
prefab = prefabZombi_B;
}
Vector3Int addPos = enemyList[i].enePos;
prefab.GetComponent<AlgoEnemy>().enePos = enemyList[i].enePos;
GameObject obj = Instantiate(prefab, new Vector3(-10,-10,0), Quaternion.identity);
obj.GetComponent<AlgoEnemy>().map = map;
obj.transform.position = new Vector3(-10, -10, 0);
if (enemyList[i].x == 0)
{
obj.GetComponent<AlgoEnemy>().x = 1;
obj.GetComponent<AlgoEnemy>().y = 0;
}
else {
obj.GetComponent<AlgoEnemy>().x = 0;
obj.GetComponent<AlgoEnemy>().y = 1;
}
enemyList.Add(obj.GetComponent<AlgoEnemy>());
addZombiNum++;
}
}
}
見直し感想:
大体全部publicにしなくて良さそう。[SerializeField]に置き換えよう。せっかくゾンビの数を増えるごとに控えてたのに結果画面ではenemyListの長さをわざわざ集計してお知らせしているという残念な扱い。getter作りましょう。
スクリプト以外の余談
AlgoEnemy内の処理ですが、2-1以降ゾンビのデザインが縦長になったのでタイルマップ上で群れるゾンビ達を「上の方ほど奥」「下の方ほど手前」の概念で表示する必要にかられ、Y軸(上下)の値をそのままZ軸(奥行)にも当てはめるということをしています。主人公も同じです。
マップはタイルマップとレイヤーを3層使って
(奥)床⇒障害物⇒(ここにキャラ)⇒常に手前にあるもの(手前)
という構成で作っています。
(今回はこれで何とかなったものの「常にキャラの足元にかぶるように表示されるもの(水・草むら)」とかどう作ればいいんだろう……)
この記事が気に入ったらサポートをしてみませんか?