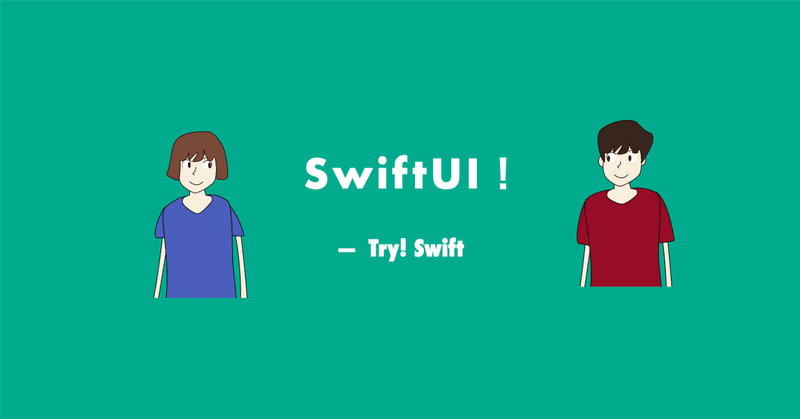
SwiftUIでじゃんけん!
じゃんけんゲームをSwiftUIで組み上げてみましょう!GoogleのGeminiを使って考えてもらい少し修正して使えるように調整します。
iPad,MacのSwiftPlaygroudsで実行できます。
Geminiに「swiftuiでじゃんけんを作って」とお願いして出してきたコードの回答案3を使うと
struct ContentView: View {
@State private var selectedHand: String = ""
@State private var computerHand: String = ""
@State private var result: String = ""
var body: some View {
VStack {
Text("じゃんけん")
.font(.largeTitle)
HStack {
Button(action: {
selectHand("グー")
}) {
Image(systemName: "hand.fingers.closed")
}
Button(action: {
selectHand("チョキ")
}) {
Image(systemName: "hand.scissors")
}
Button(action: {
selectHand("パー")
}) {
Image(systemName: "hand")
}
}
Text(result)
.padding()
Button(action: {
playJanken()
}) {
Text("勝負!")
}
}
}
func selectHand(_ hand: String) {
selectedHand = hand
}
func playJanken() {
let computerChoices = ["グー", "チョキ", "パー"]
let computerHand = computerChoices.randomElement()!
self.computerHand = computerHand
if selectedHand == computerHand {
result = "あいこ!"
} else if selectedHand == "グー" && computerHand == "チョキ" {
result = "勝ち!"
} else if selectedHand == "グー" && computerHand == "パー" {
result = "負け!"
} else if selectedHand == "チョキ" && computerHand == "グー" {
result = "負け!"
} else if selectedHand == "チョキ" && computerHand == "パー" {
result = "勝ち!"
} else if selectedHand == "パー" && computerHand == "グー" {
result = "勝ち!"
} else if selectedHand == "パー" && computerHand == "チョキ" {
result = "負け!"
}
}
}
実行画面は
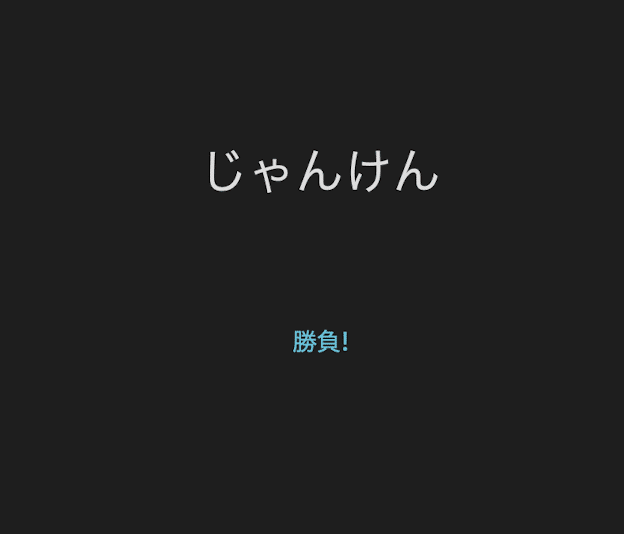
となって動きません。まず
HStack {
Button(action: {
selectHand("グー")
}) {
Image(systemName: "hand.fingers.closed")
}
Button(action: {
selectHand("チョキ")
}) {
Image(systemName: "hand.scissors")
}
Button(action: {
selectHand("パー")
}) {
Image(systemName: "hand")
}
}
の部分でうまく表示できていないので絵文字を表示するように変更します。
HStack {
Button(action: {
selectHand("グー")
}) {
Text("✊")
.font(.title)
}
Button(action: {
selectHand("チョキ")
}) {
Text("✌️")
.font(.title)
}
Button(action: {
selectHand("パー")
}) {
Text("🖐️")
.font(.title)
}
}
Image()を消してText()で置き換えます。
次にじゃんけんを実行するボタン"勝負"をもう少しボタンらしくします。
最初がこちら
Button(action: {
playJanken()
}) {
Text("勝負!")
}
修正後
Button(action: {
playJanken()
}) {
Text("勝負!")
.frame(width: 100, height: 50)
.foregroundColor(Color.white)
.frame(width: 100, height: 50)
.foregroundColor(Color.white)
.background(Color.blue)
.cornerRadius(25)
}
ここまでの画面表を見てみると
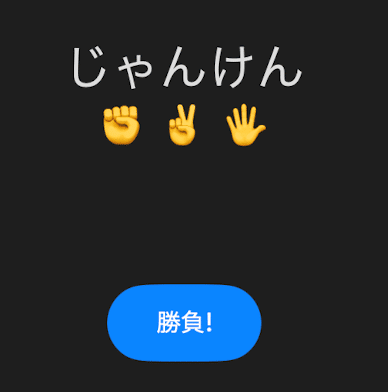
それっぽくなりました。使い方はまず自分の手を絵文字から選び押します。その後”勝負”ボタンを押すと真ん中に結果がでます。
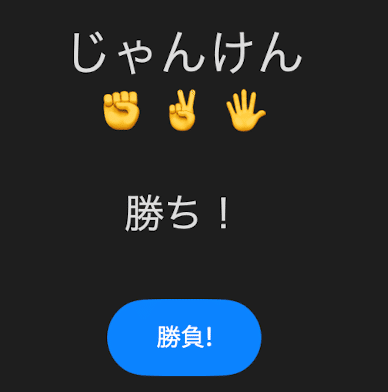
ここで勝敗の結果はわかりますが、自分の手と相手の手を表示させてみます。ちゃんとじゃんけんとして成り立っているかを確認します。
Text("自分の出した手:\(selectedHand)")
Text("相手の出した手:\(computerHand)")
を”勝負”ボタンに配置して表示させます。
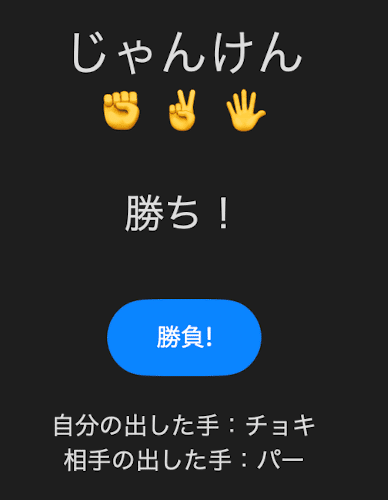
といい感じに勝敗の手を見ることができるようになりました。
全体のコードです。
import SwiftUI
struct ContentView: View {
@State private var selectedHand: String = ""
@State private var computerHand: String = ""
@State private var result: String = ""
var body: some View {
VStack {
Text("じゃんけん")
.font(.largeTitle)
HStack {
Button(action: {
selectHand("グー")
}) {
Text("✊")
.font(.title)
}
Button(action: {
selectHand("チョキ")
}) {
Text("✌️")
.font(.title)
}
Button(action: {
selectHand("パー")
}) {
Text("🖐️")
.font(.title)
}
}
Text(result)
.font(.title)
.padding()
Button(action: {
playJanken()
}) {
Text("勝負!")
.frame(width: 100, height: 50)
.foregroundColor(Color.white)
.frame(width: 100, height: 50)
.foregroundColor(Color.white)
.background(Color.blue)
.cornerRadius(25)
}
.padding()
Text("自分の出した手:\(selectedHand)")
Text("相手の出した手:\(computerHand)")
}
}
func selectHand(_ hand: String) {
selectedHand = hand
}
func playJanken() {
let computerChoices = ["グー", "チョキ", "パー"]
let computerHand = computerChoices.randomElement()!
self.computerHand = computerHand
print(computerHand)
if selectedHand == computerHand {
result = "あいこ!"
} else if selectedHand == "グー" && computerHand == "チョキ" {
result = "勝ち!"
} else if selectedHand == "グー" && computerHand == "パー" {
result = "負け!"
} else if selectedHand == "チョキ" && computerHand == "グー" {
result = "負け!"
} else if selectedHand == "チョキ" && computerHand == "パー" {
result = "勝ち!"
} else if selectedHand == "パー" && computerHand == "グー" {
result = "勝ち!"
} else if selectedHand == "パー" && computerHand == "チョキ" {
result = "負け!"
}
}
}
この記事が気に入ったらサポートをしてみませんか?