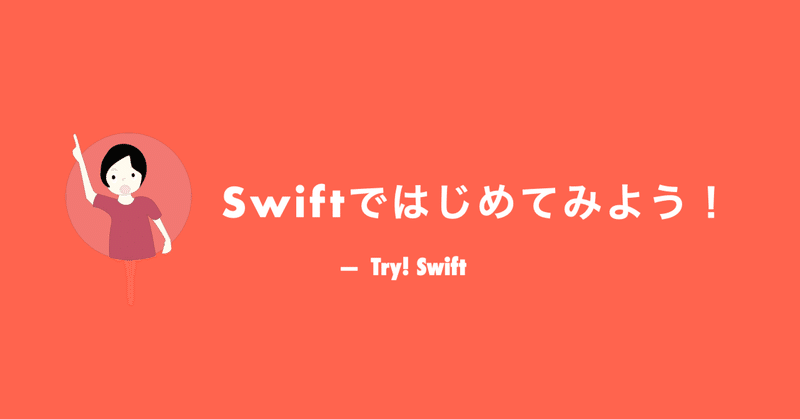
Swiftではじめてみよう! - "switch" ・・・の場合は。
"swich"を使ってみましょう。対象の変数にたいして複数のcaseを作ってその条件に合致する場合の処理をさせることができます。
基本的な構文は
switch 変数{
case 値: 1
処理1
case 値: 2
処理2
defalt:
処理(デフォルト)
{
となります。
let someCharacter: Character = "z"
switch someCharacter {
case "a":
print("The first letter of the alphabet")
case "z":
print("The last letter of the alphabet")
default:
print("Some other character")
}
これを実行すると、
"The last letter of the alphabet"
と出力されます。
単純にcaseでいろんな場合について処理を分けています。
実行すると"switch"に続く変数に該当する"case"定義された処理をすることができます。
上記の例では変数 "someCharacter"は"z"ということで宣言されています。
そしてswitch に続き変数someCharacterが指定されて、それ以下でcaseで宣言された各処理が書かれています。
case "a":
print("The first letter of the alphabet")
case "z":
print("The last letter of the alphabet")
default:
print("Some other character")
"someCharacter"は"z"と宣言されているので該当する"case"は"z"と書かれたものを探します。
2番目の"case"に"z"があるのでその宣言しある処理
print("The last letter of the alphabet")
が実行されるわけです。
case で使える条件ですが単純な値一つだけではないので他のものもあげていきたいと思います。
単純に2つ以上のものも指定できます。
let anotherCharacter: Character = "a"
switch anotherCharacter {
case "a", "A":
print("The letter A")
default:
print("Not the letter A")
}
"The letter A"
と出力されます。それから数字の範囲指定もできます。
let approximateCount = 62
let countedThings = "moons orbiting Saturn"
let naturalCount: String
switch approximateCount {
case 0:
naturalCount = "no"
case 1..<5:
naturalCount = "a few"
case 5..<12:
naturalCount = "several"
case 12..<100:
naturalCount = "dozens of"
case 100..<1000:
naturalCount = "hundreds of"
default:
naturalCount = "many"
}
print("There are \(naturalCount) \(countedThings).")
let approximateCount = 62
なので
case 12..<100:
naturalCount = "dozens of"
が選択されて
There are dozens of moons orbiting Saturn.
と出力されます。次タプル。
let somePoint = (1, 1)
switch somePoint {
case (0, 0):
print("\(somePoint) is at the origin")
case (_, 0):
print("\(somePoint) is on the x-axis")
case (0, _):
print("\(somePoint) is on the y-axis")
case (-2...2, -2...2):
print("\(somePoint) is inside the box")
default:
print("\(somePoint) is outside of the box")
}
case (-2...2, -2...2):
に該当するので
(1, 1) is inside the box
と出力されます。ここで、 (_, 0)となっているのは"_"はどんな数字でも良いという意味で、(1,0)、(2,0)、(3,0)でも良いということです。
Value Bindingsということでcaseの中に変数を使って処理内容の反映することもできます。
let anotherPoint = (2, 0)
switch anotherPoint {
case (let x, 0):
print("on the x-axis with an x value of \(x)")
case (0, let y):
print("on the y-axis with a y value of \(y)")
case let (x, y):
print("somewhere else at (\(x), \(y))")
}
実行すると
case (let x, 0):
print("on the x-axis with an x value of \(x)")
に該当して変数を定義し、その処理の中で変数を使って出力しています。
on the x-axis with an x value of 2
caseの中で条件を"where"で絞ることもできます。
let yetAnotherPoint = (1, -1)
switch yetAnotherPoint {
case let (x, y) where x == y:
print("(\(x), \(y)) is on the line x == y")
case let (x, y) where x == -y:
print("(\(x), \(y)) is on the line x == -y")
case let (x, y):
print("(\(x), \(y)) is just some arbitrary point")
}
case let (x, y) where x == -y:
print("(\(x), \(y)) is on the line x == -y")
に該当するので
(1, -1) is on the line x == -y
と出力されます。
この記事が気に入ったらサポートをしてみませんか?