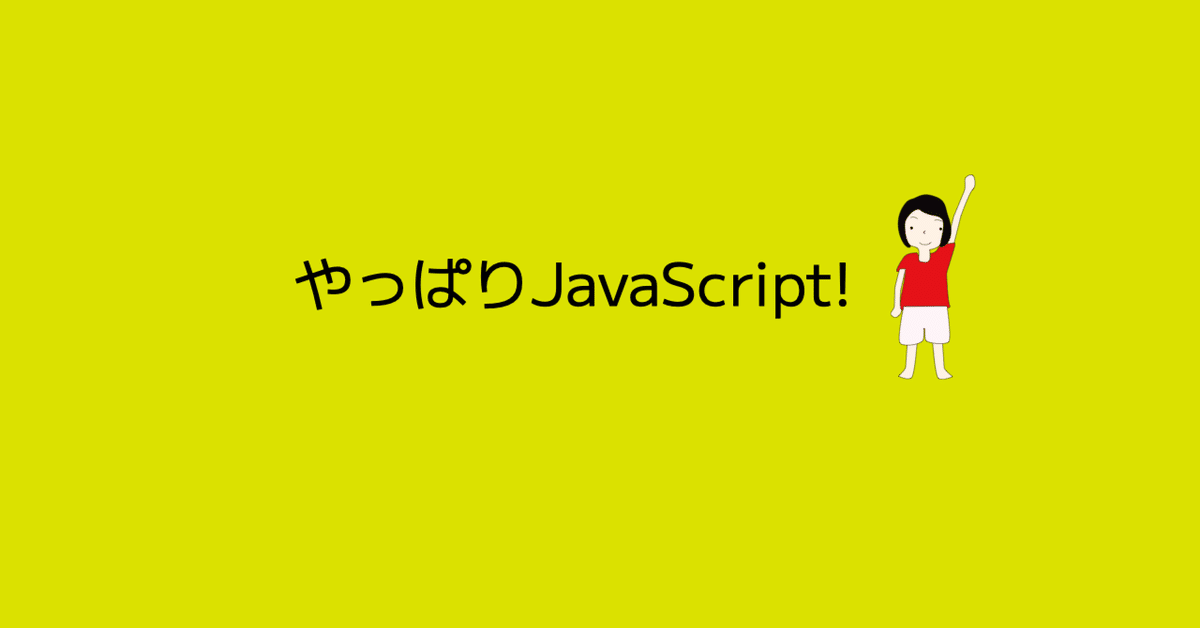
やっぱり。JavaScript!- ToDoリスト - 5
とにかにく入力できて、保存ができるようになりました。ToDoリストなので完了したという目印が欲しいところなのでその仕組みをつくります。
そこでオブジェクトという考え方が必要です。
一つの変数に2つ以上の情報(プロパティ)をもたすことになります。いわゆるプロパティというものになります。今回のものだと、
const todos = [];
として配列をつくていますがこの配列の中にオブジェクトと呼ばれる仕組みで保存のプロパティを作ります。
単純にテキスト(text)、文字を保存するものと、完了したこと(completed)を保存するものを作ります。
{ text: li.innerText,
completed: li.classList.contains("text-decoration-line-through") }
配列として定義してあるので、追加するにはpush()を使います。
todos.push({
text: li.innerText,
completed: li.classList.contains("text-decoration-line-through"),
});
全体としては
function saveData() {
const lists = document.querySelectorAll("li");
const todos = [];
lists.forEach((li) => {
todos.push({
text: li.innerText,
completed: li.classList.contains("text-decoration-line-through"),
});
});
localStorage.setItem("todos", JSON.stringify(todos));
}
となります。
このように保存しておくと"todo.completed"の状態によりリストに取り消し線("text-decoration-line-through")をつける(.add)ことができるようになります。
if (todo && todo.completed) {
li.classList.add("text-decoration-line-through");
}
そしてクリックすることでつけたり、外したり(toggle)できるようにします。
li.addEventListener("click", function () {
li.classList.toggle("text-decoration-line-through");
saveData();
});
全体としては以下となります。
const form = document.getElementById("form");
const input = document.getElementById("input");
const ul = document.getElementById("ul");
const todos = JSON.parse(localStorage.getItem("todos"));
if (todos) {
todos.forEach((todo) => {
add(todo);
});
}
form.addEventListener("submit", function (event) {
event.preventDefault();
add();
});
function add(todo){
let todoText = input.value;
if (todo) {
todoText = todo.text;
}
if (todoText) {
const li = document.createElement("li");
li.innerText = todoText;
li.classList.add('list-group-item')
if (todo && todo.completed) {
li.classList.add("text-decoration-line-through");
}
li.addEventListener("click", function () {
li.classList.toggle("text-decoration-line-through");
saveData();
});
li.addEventListener("contextmenu", function (event) {
event.preventDefault();
li.remove();
saveData();
});
ul.appendChild(li);
input.value = "";
saveData()
}
}
function saveData() {
const lists = document.querySelectorAll("li");
const todos = [];
lists.forEach((li) => {
todos.push({
text: li.innerText,
completed: li.classList.contains("text-decoration-line-through"),
});
});
localStorage.setItem("todos", JSON.stringify(todos));
}
この記事が気に入ったらサポートをしてみませんか?