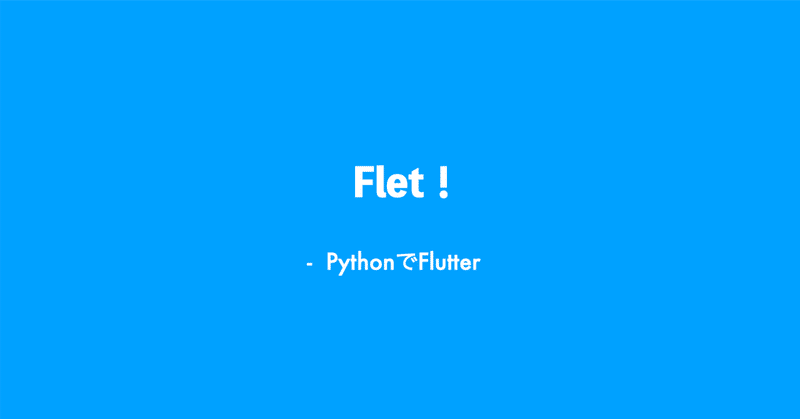
Fletを使ってみよう!- いろんなView
ListView
import flet as ft
def main(page: ft.Page):
for i in range(5000):
page.controls.append(ft.Text(f"Line {i}"))
page.scroll = "always"
page.update()
ft.app(target=main, view=ft.AppView.WEB_BROWSER)
と同じことが、ListView()を使ってスクロールするリストが
import flet as ft
def main(page: ft.Page):
lv = ft.ListView(expand=True, spacing=10)
for i in range(5000):
lv.controls.append(ft.Text(f"Line {i}"))
page.add(lv)
ft.app(target=main, view=ft.AppView.WEB_BROWSER)
とすればできます。リストの機能を簡単に実装できます。
lv = ft.ListView(expand=True, spacing=10)
変数"lv"にリストの機能を持たせ、
Text(f"Line {i}"
でリスト形式で表示させます。この場合for-inループで指定のrange(5000)から"i"として取り出して順番に表示しています。
オートスクロールの機能
lv = ft.ListView(expand=1, spacing=10, padding=20, auto_scroll=True)
とすれば自動でスクロールしてくれます。
GridView
import flet as ft
def main(page: ft.Page):
page.title = "GridView Example"
page.theme_mode = ft.ThemeMode.DARK
page.padding = 50
page.update()
images = ft.GridView(
expand=1,
runs_count=5,
max_extent=150,
child_aspect_ratio=1.0,
spacing=5,
run_spacing=5,
)
page.add(images)
for i in range(0, 60):
images.controls.append(
ft.Image(
src=f"https://picsum.photos/150/150?{i}",
fit=ft.ImageFit.NONE,
repeat=ft.ImageRepeat.NO_REPEAT,
border_radius=ft.border_radius.all(10),
)
)
page.update()
ft.app(target=main, view=ft.AppView.WEB_BROWSER)
GridView()
を使って画像を綺麗に並べることができます。
これを実行してやればグリッドで画像が並べられます。
images = ft.GridView(
expand=1,
runs_count=5,
max_extent=150,
child_aspect_ratio=1.0,
spacing=5,
run_spacing=5,
)
部分で表示設定します。
そして画像を取り出す命令です。
for i in range(0, 60):
images.controls.append(
ft.Image(
src=f"https://picsum.photos/150/150?{i}",
fit=ft.ImageFit.NONE,
repeat=ft.ImageRepeat.NO_REPEAT,
border_radius=ft.border_radius.all(10),
)
)
Webサイトから画像を一つずつ取り出します。
https://picsum.photos ・・・ ダミー画像を提供しているサイト
画像処理はここでは、"src","fit","repeat","border_radius"というプロパティを設定しています。
そして
page.update()
で実際の画面に表示されます。
実行する時の注意です。
ft.app(target=main, view=ft.AppView.WEB_BROWSER)
サンプルではAppView.WEB_BROWSERとしてしてありブラウザが立ち上がり表示されます。これをVSCodeで実行するとその後実行できなくなるので注意が必要です。
ft.app(target=main)
に変更して使うこともできるのでそちらの方がいいかも。
Table
シンプルなテーブルです。 ft.DataTable()の中にcolumn,rowの中身を指定してします。
import flet as ft
def main(page: ft.Page):
page.add(
ft.DataTable(
columns=[
ft.DataColumn(ft.Text("First name")),
ft.DataColumn(ft.Text("Last name")),
ft.DataColumn(ft.Text("Age"), numeric=True),
],
rows=[
ft.DataRow(
cells=[
ft.DataCell(ft.Text("John")),
ft.DataCell(ft.Text("Smith")),
ft.DataCell(ft.Text("43")),
],
),
ft.DataRow(
cells=[
ft.DataCell(ft.Text("Jack")),
ft.DataCell(ft.Text("Brown")),
ft.DataCell(ft.Text("19")),
],
),
ft.DataRow(
cells=[
ft.DataCell(ft.Text("Alice")),
ft.DataCell(ft.Text("Wong")),
ft.DataCell(ft.Text("25")),
],
),
],
),
)
ft.app(target=main)
表の部分は簡単にすると
ft.DataTable(
columns=[
],
rows=[
],
),
column縦とrows横を[ ]で囲ってデータを入れていきます
Tabs
ft.Tabs()で定義していきます。
import flet as ft
def main(page: ft.Page):
t = ft.Tabs(
selected_index=1,
animation_duration=300,
tabs=[
ft.Tab(
text="Tab 1",
content=ft.Container(
content=ft.Text("This is Tab 1"), alignment=ft.alignment.center
),
),
ft.Tab(
tab_content=ft.Icon(ft.icons.SEARCH),
content=ft.Text("This is Tab 2"),
),
ft.Tab(
text="Tab 3",
icon=ft.icons.SETTINGS,
content=ft.Text("This is Tab 3"),
),
],
expand=1,
)
page.add(t)
ft.app(target=main)
全体の設定。
selected_index=1, ・・・ tab1が初期に表示するページ
animation_duration=300, ・・・押した時のアニメーションの時間
tabの中身、内容は
tabs=[
ft.Tab()
]
で作っていきます。あとは 内容については
"Tab properties" ・・・ content、tab_content、icon
で設定していきます。
Card
ft.Card()の中に処理を書いていきます。
import flet as ft
def main(page):
page.title = "Card Example"
page.add(
ft.Card(
content=ft.Container(
content=ft.Column(
[
ft.ListTile(
leading=ft.Icon(ft.icons.ALBUM),
title=ft.Text("The Enchanted Nightingale"),
subtitle=ft.Text(
"Music by Julie Gable. Lyrics by Sidney Stein."
),
),
ft.Row(
[ft.TextButton("Buy tickets"), ft.TextButton("Listen")],
alignment=ft.MainAxisAlignment.END,
),
]
),
width=400,
padding=10,
)
)
)
ft.app(target=main)
この場合は
content=ft.Column
で縦並びで "ft.ListTile"と "ft.Row()"を配置しています。
ft.Row()は横並びでボタンを配置しています。
この記事が気に入ったらサポートをしてみませんか?