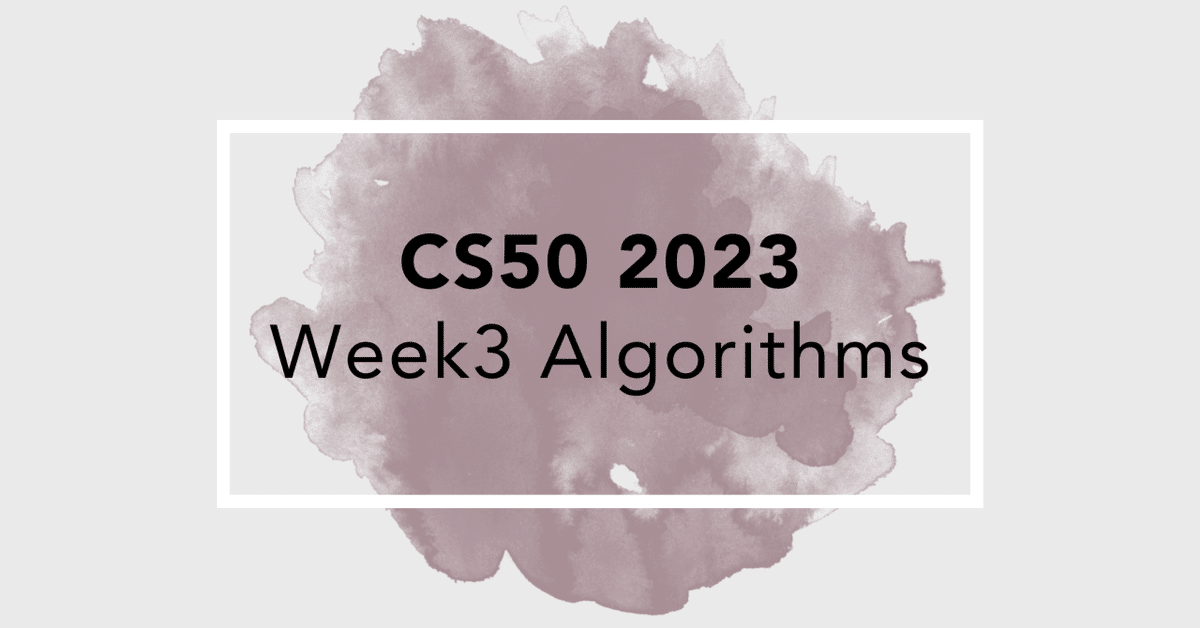
CS50 2023 - Week3 Algorithms
概要
Week3では、アルゴリズムを学びます。
講義の主な内容は、リニアサーチ(線形探索)、バイナリーサーチ(二分探索)、バブルソート、セレクションソート(選択ソート)、マージソート、漸近記法、再帰です。
Lab 3
Sort
sort1、sort2、sort3という3つのバイナリファイルが用意されています。これに加え、様々な順序や行数の数字リストも提供されています。
sortファイルのソースコードを直接確認することはできませんが、数字リストをソートする速度を基に、それぞれのファイルがどのソートアルゴリズムを採用しているのか推測します。
Walkthroughの動画で触れられているように、各ソートアルゴリズムの特徴をしっかりと理解することが鍵となります。
以下は、私が提出したanswers.txtの内容です。
sort1 uses:
Bubble
How do you know?:Faster on sorted lists, slower on reversed and random lists, significant time increase with larger list size.
sort2 uses:
Merge
How do you know?:
Consistent times across all inputs, moderate time increase with larger list size.
sort3 uses:
Selection
How do you know?:
Slower on reversed and random lists, moderately faster on sorted ones, significant time increase with larger list size.wget
Problem Set 3
Week3のProblem Setは、Pluralityと以下の課題からひとつ選び、計2つの課題を提出します。
Runoff (less comfortable)
Tideman (more comfortable)
本ページでは、Runoffを取り上げます。
Plurality
多数決式の選挙をシミュレートするプログラムを作成します。
仕様は下記です。
最大9人の候補者が選挙に参加できる
候補者は、名前と得票数の2つのフィールドを持つ
vote関数:指定された候補者の得票数を1増やす。ただし、名前が候補者リストになければ、無効票として扱い、得票数は変動しない。
print_winner関数: 最多得票の候補者の名前を出力。複数の候補者が最多得票の場合、それぞれの名前を別の行に表示。
vote関数とprint_winner関数の実装を完成させることが目的です。
以下は私が提出したコードです。
#include <cs50.h>
#include <stdio.h>
#include <string.h>
// Max number of candidates
#define MAX 9
// Candidates have name and vote count
typedef struct
{
string name;
int votes;
} candidate;
// Array of candidates
candidate candidates[MAX];
// Number of candidates
int candidate_count;
// Function prototypes
bool vote(string name);
void print_winner(void);
int main(int argc, string argv[])
{
// Check for invalid usage
if (argc < 2)
{
printf("Usage: plurality [candidate ...]\n");
return 1;
}
// Populate array of candidates
candidate_count = argc - 1;
if (candidate_count > MAX)
{
printf("Maximum number of candidates is %i\n", MAX);
return 2;
}
for (int i = 0; i < candidate_count; i++)
{
candidates[i].name = argv[i + 1];
candidates[i].votes = 0;
}
int voter_count = get_int("Number of voters: ");
// Loop over all voters
for (int i = 0; i < voter_count; i++)
{
string name = get_string("Vote: ");
// Check for invalid vote
if (!vote(name))
{
printf("Invalid vote.\n");
}
}
// Display winner of election
print_winner();
}
// Update vote totals given a new vote
bool vote(string name)
{
// TODO
for (int i = 0; i < candidate_count; i++)
{
if (strcmp(name, candidates[i].name) == 0)
{
candidates[i].votes++;
return true;
}
}
return false;
}
// Print the winner (or winners) of the election
void print_winner(void)
{
// TODO
int max_votes = 0;
for (int i = 0; i < candidate_count; i++)
{
if (candidates[i].votes > max_votes)
{
max_votes = candidates[i].votes;
}
}
for (int i = 0; i < candidate_count; i++)
{
if (candidates[i].votes == max_votes)
{
printf("%s\n", candidates[i].name);
}
}
return;
}
Runoff
選挙方式をシミュレートするプログラムを作成します。
この方式では、最多得票を取得した候補者が過半数を獲得していない場合、最も少ない得票を得た候補者を順に除外していきます。
主要な処理の流れは下記の通りです。
すべての投票者が投票を行う
最も得票数が多い候補者が過半数を獲得しているか確認する
過半数を獲得していない場合、最少得票の候補者を除外する
1人の勝者が決まるか、全員が同じ得票数になるまで2と3のステップを繰り返す
この課題で実装が求められる関数は下記の通りです。
vote:指定された投票者の優先度に基づき、得票を記録する
tabulate:各段階での候補者の得票数を更新する
print_winner:候補者が過半数の票を有している場合、その名前を出力する
find_min:現行の選挙で最少得票数を返す
is_tie:全ての残っている候補者が同じ得票数を持っているか確認する
eliminate:最少得票数を持つ候補者を選挙から除外する
以下は私が提出したコードです。
#include <cs50.h>
#include <stdio.h>
#include <string.h>
// Max voters and candidates
#define MAX_VOTERS 100
#define MAX_CANDIDATES 9
// preferences[i][j] is jth preference for voter i
int preferences[MAX_VOTERS][MAX_CANDIDATES];
// Candidates have name, vote count, eliminated status
typedef struct
{
string name;
int votes;
bool eliminated;
} candidate;
// Array of candidates
candidate candidates[MAX_CANDIDATES];
// Numbers of voters and candidates
int voter_count;
int candidate_count;
// Function prototypes
bool vote(int voter, int rank, string name);
void tabulate(void);
bool print_winner(void);
int find_min(void);
bool is_tie(int min);
void eliminate(int min);
int main(int argc, string argv[])
{
// Check for invalid usage
if (argc < 2)
{
printf("Usage: runoff [candidate ...]\n");
return 1;
}
// Populate array of candidates
candidate_count = argc - 1;
if (candidate_count > MAX_CANDIDATES)
{
printf("Maximum number of candidates is %i\n", MAX_CANDIDATES);
return 2;
}
for (int i = 0; i < candidate_count; i++)
{
candidates[i].name = argv[i + 1];
candidates[i].votes = 0;
candidates[i].eliminated = false;
}
voter_count = get_int("Number of voters: ");
if (voter_count > MAX_VOTERS)
{
printf("Maximum number of voters is %i\n", MAX_VOTERS);
return 3;
}
// Keep querying for votes
for (int i = 0; i < voter_count; i++)
{
// Query for each rank
for (int j = 0; j < candidate_count; j++)
{
string name = get_string("Rank %i: ", j + 1);
// Record vote, unless it's invalid
if (!vote(i, j, name))
{
printf("Invalid vote.\n");
return 4;
}
}
printf("\n");
}
// Keep holding runoffs until winner exists
while (true)
{
// Calculate votes given remaining candidates
tabulate();
// Check if election has been won
bool won = print_winner();
if (won)
{
break;
}
// Eliminate last-place candidates
int min = find_min();
bool tie = is_tie(min);
// If tie, everyone wins
if (tie)
{
for (int i = 0; i < candidate_count; i++)
{
if (!candidates[i].eliminated)
{
printf("%s\n", candidates[i].name);
}
}
break;
}
// Eliminate anyone with minimum number of votes
eliminate(min);
// Reset vote counts back to zero
for (int i = 0; i < candidate_count; i++)
{
candidates[i].votes = 0;
}
}
return 0;
}
// Record preference if vote is valid
bool vote(int voter, int rank, string name)
{
// TODO
for (int i = 0; i < candidate_count; i++)
{
if (strcmp(candidates[i].name, name) == 0)
{
preferences[voter][rank] = i;
return true;
}
}
return false;
}
// Tabulate votes for non-eliminated candidates
void tabulate(void)
{
// TODO
for (int i = 0; i < candidate_count; i++)
{
candidates[i].votes = 0;
}
for (int i = 0; i < voter_count; i++)
{
for (int j = 0; j < candidate_count; j++)
{
if (!candidates[preferences[i][j]].eliminated)
{
candidates[preferences[i][j]].votes++;
break;
}
}
}
}
// Print the winner of the election, if there is one
bool print_winner(void)
{
// TODO
for (int i = 0; i < candidate_count; i++)
{
if (candidates[i].votes > voter_count / 2)
{
printf("%s\n", candidates[i].name);
return true;
}
}
return false;
}
// Return the minimum number of votes any remaining candidate has
int find_min(void)
{
// TODO
int min_votes = voter_count;
for (int i = 0; i < candidate_count; i++)
{
if (!candidates[i].eliminated && candidates[i].votes < min_votes)
{
min_votes = candidates[i].votes;
}
}
return min_votes;
}
// Return true if the election is tied between all candidates, false otherwise
bool is_tie(int min)
{
// TODO
for (int i = 0; i < candidate_count; i++)
{
if (!candidates[i].eliminated && candidates[i].votes != min)
{
return false;
}
}
return true;
}
// Eliminate the candidate (or candidates) in last place
void eliminate(int min)
{
// TODO
for (int i = 0; i < candidate_count; i++)
{
if (candidates[i].votes == min)
{
candidates[i].eliminated = true;
}
}
return;
}
さいごに
C言語に関する学習も中盤を迎えました。
アルゴリズムの説明は煩雑になりやすいものですが、CS50の講義は非常に理解しやすく構成されていましたね。
このWeekからLabとProblem Setが更に魅力的になっていきます。
講義だけではなく、これらの課題もCS50の真骨頂です。楽しみながら学んでいきましょう。
この記事が気に入ったらサポートをしてみませんか?