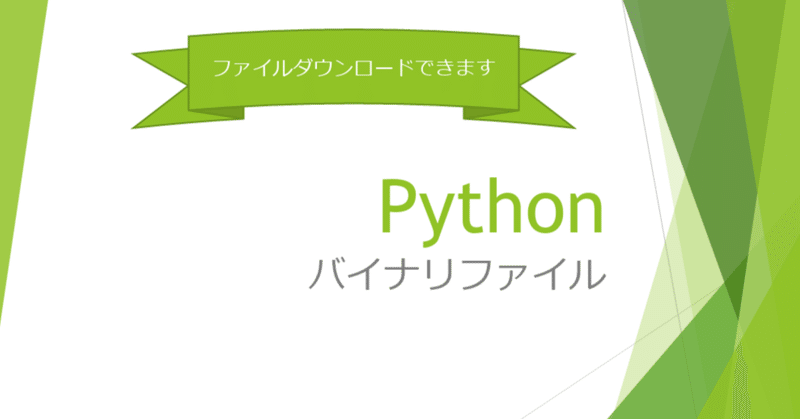
【Python】バイナリファイルのアクセス(ダウンロードファイル付き)
Pythonでバイナリファイルをアクセスする関数を作ってみました。
エラー処理も何もないスケルトン的な関数です。
ファイルをバイナリでダンプしたかったのが目的。
Pythonなら、もう少しタイトなコードにできるのかもしれません。
doctestを入れたので解説は省きました。
コード
def write_file_bin(file_name, bin):
''' ファイルライト(バイナリ)
file_name:ファイル名
bin:バイナリデータ
>>> bin = bytearray([0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f, 0x010, 0x011, 0x012, 0x013])
>>> write_file_bin('test.bin', bin)
'''
# ファイルオープン(wb=書き込みバイナリ)
with open(file_name, 'wb') as fo:
fo.write(bin)
def dump_file_bin(file_name, n=8):
''' ファイルダンプ(バイナリ)
file_name:ファイル名
nbyte毎に改行する
>>> bin = bytearray([0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f, 0x010, 0x011, 0x012, 0x013])
>>> write_file_bin('test.bin', bin)
>>> dump_file_bin('test.bin')
00000000 0a 0b 0c 0d 0e 0f 10 11
00000008 12 13
'''
# ファイルオープン(rb=読み込みバイナリ)
with open(file_name, 'rb') as fo:
# 1byteずつ処理する(fo.read()の戻り値は「bytes」)
for i, data in enumerate(fo.read(), start=1):
# 行の先頭にオフセットを表示する
if ((i % n) == 1):
print('%08x' % (i-1), end=' ')
# 16進数2桁で1byteを改行せずに表示する
print('%02x' % data, end=' ')
# nbyte毎に改行する
if ((i % n) == 0):
print('')
if __name__ == '__main__':
import doctest
doctest.testmod()
実行結果
~/.../shared/qpython $ python binary_file.py -v
Trying:
bin = bytearray([0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f, 0x010, 0x011, 0x012, 0x013])
Expecting nothing
ok
Trying:
write_file_bin('test.bin', bin)
Expecting nothing
ok
Trying:
dump_file_bin('test.bin')
Expecting:
00000000 0a 0b 0c 0d 0e 0f 10 11
00000008 12 13
ok
Trying:
bin = bytearray([0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f, 0x010, 0x011, 0x012, 0x013])
Expecting nothing
ok
Trying:
write_file_bin('test.bin', bin)
Expecting nothing
ok
1 items had no tests:
__main__
2 items passed all tests:
3 tests in __main__.dump_file_bin
2 tests in __main__.write_file_bin
5 tests in 3 items.
5 passed and 0 failed.
Test passed.
~/.../shared/qpython $
ダウンロード
※ご利用は自己責任でお願いします。
この記事が気に入ったらサポートをしてみませんか?