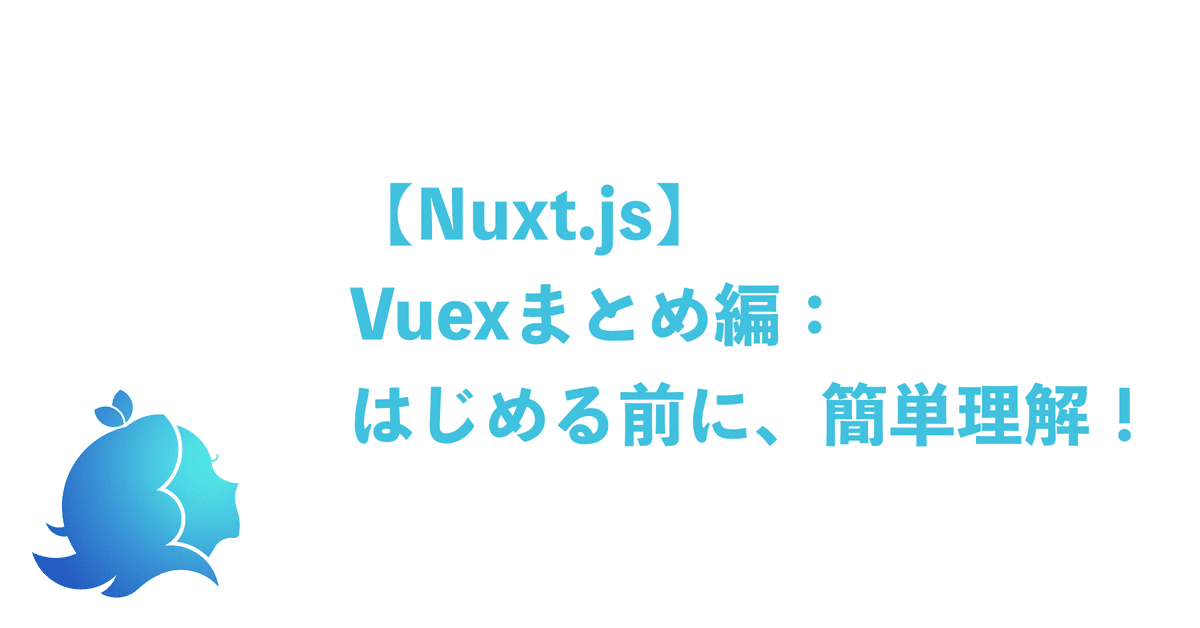
【Nuxt.js】Vuexまとめ編:はじめる前に、簡単理解!
🎈 この記事はWPへ移行しました
【Nuxt.js】Vuexまとめ編:はじめる前に、簡単理解!
# 前置き
ログイン情報の保持や
APIのデータ保存など
とにかく便利なVuex🌟
さぁ、やってみよう!💪
と思っても1から理解するのは大変ですよね?
「忙しいのに
また新しいことやるのか…😔💦」
そんな方へ向けて超簡単に
最低限ここさえ分かればOK!
な部分をまとめました。
※本日はVuex & Firebaseの予定でしたが、
Vuexについては簡単な部分しか
触れていなかったので
まとまった内容にしました✍️
導入の仕方や
ディレクトリ 構成は
基礎編でどうぞ!
# メリット
どこからでもアクセスできること
これが最大のメリットです👍
複数のコンポーネントで
同じデータを保有したい場合、
propsや$emitでもできますが
ネスト(階層, 入れ子)が深いと
受け渡しの関係が分かりにくく
複雑になってしまいます🌪
それを1箇所にまとめてくれるのがVuex🌟
コードもシンプルで分かりやすくなります!
# 状態管理パターン
データの状態管理の仕方です。
4つあり、一方通行(単一フロー)で操作します。
順番に
・state: data保管庫
・getters: stateの情報を取得
別gettersの呼び出しも可能
・actions: storeの上書き以外の処理や非同期通信
別actionsの呼び出しも可能
・mutations: stateの上書き(代入)
となります!
actionsとmutationsが
methodsのような役割をしています。
【例】
buttonクリックでcounterの数字を1増やしたい
storeでは
・state: counter
・getters: counterを取得
・actions: なし
・mutations: state.counter++
これをpagesで呼び出していきます🌟
【基本の呼び出し方】
・state: 直接呼び出すことはない
・getters: (return) this.$store.getters['{関数名}']
・actions: this.$store.dispatch('{関数名}')
・mutations: this.$store.commit('{関数名}')
関数名の部分はディレクトリ に応じて
書き方が変わります。
・store/index.jsのgetters hogeを指定
this.$store.getters['hoge']
・store/sample/index.jsのgetters hogeを指定
this.$store.getters['sample/hoge']
# state
【store/index.js】
export const state = () => ({
hoge: [],
})
【pages/index.vue】
一応直接呼び出しもできます。
<template>
<div class="page">
<p>{{ $store.state.hoge }}</p>
</div>
</template>
<script>
export default {
}
</script>
が、gettersを通して呼びましょう。
それぞれ役割が決まっているためです。
アーキテクチャやMVVMを見てみると
良いかもしれません👀
# getters
gettersの時だけ関数名を[ ]で囲みます!
【index.js】
export const getters = {
hoge: state => {
return state.hoge
},
}
【pages/index.vue】
<template>
<div class="page">
<p>{{ hoge }}</p>
</div>
</template>
<script>
export default {
computed: {
hoge () {
return this.$store.getters['hoge']
}
},
}
</script>
# actions
非同期通信やAPI通信など。
stateの上書きはmutationsに任せるので
mutationsの呼び出し(commit)をします。
サンプルコードは特にactionsを通さず
mutationsで事足りるのですが
あえて分かりやすく書くとこうなります。
【store/index.js】
export const state = () => ({
counter: 1,
})
export const getters = {
counter (state) {
return state.counter
},
}
export const actions = {
countAction ({ commit }) {
commit('countUp')
}
}
export const mutations = {
countUp (state) {
state.counter++
},
}
【pages/index.vue】
<template>
<div class="page">
<button
type="button"
@click="count"
>
{{ counter }}
</button>
</div>
</template>
<script>
export default {
computed: {
counter () {
return this.$store.getters['counter']
},
},
methods: {
count () {
return this.$store.dispatch('countAction')
},
},
}
</script>
# mutations
【store/index.js】
export const state = () => ({
counter: 1,
})
export const getters = {
counter (state) {
return state.counter
},
}
export const mutations = {
countUp (state) {
state.counter++
},
}
【pages/index.vue】
<template>
<div class="page">
<button
type="button"
@click="count"
>
{{ counter }}
</button>
</div>
</template>
<script>
export default {
computed: {
counter () {
return this.$store.getters['counter']
},
},
methods: {
count () {
return this.$store.commit('countUp')
},
},
}
</script>
# map系
値がたくさんあって楽をしたい時に使います!
ただあまり使うことはありません。
基本的に書き方が少し変わるだけで
やっていること自体に変化はありません。
【store/index.js】
export const state = () => ({
hoge: 'mapGetters'
})
export const getters = {
hoge (state) {
return state.hoge
},
}
【pages/index.vue】
<template>
<div class="page">
{{ hoge }}
</div>
</template>
<script>
import { mapGetters } from 'vuex'
export default {
computed: {
...mapGetters(['hoge'])
},
}
</script>
次回はVuex & Firebase!
公開予定日は4/24(金)です。
記事が公開したときにわかる様に、
フォローをお願いします😀
🎈 この記事はWPへ移行しました
【Nuxt.js】Vuexまとめ編:はじめる前に、簡単理解!
この記事が気に入ったらサポートをしてみませんか?