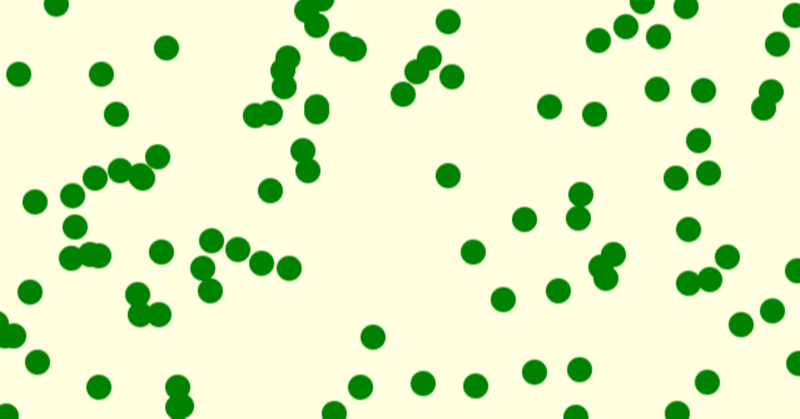
Canvasで大量の円を上下左右で跳ね返す
Canvas(JavaScript)を勉強しています。
前回、円が上下左右に跳ね回るものを作りました。
今回は、その円が大量(100個)に増やしてみました。
Classを使ったから、このような簡単に円の数が増やせました。
function initialize() {
canvas = document.body.querySelector('canvas');
ctx = canvas.getContext('2d');
for (let i = 0; i < NUM; i++) {
moveRect[i] = new Mover();
}
}
function render() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
// background
ctx.fillStyle = 'lightyellow';
ctx.fillRect(0, 0, canvas.width, canvas.height);
for (let i = 0; i < NUM; i++) {
moveRect[i].drawRect();
}
window.requestAnimationFrame(render);
}
class Mover {
constructor() {
this.x = Math.floor(Math.random() * canvas.width);
this.y = Math.floor(Math.random() * canvas.height);
this.dx = getRandomInt(-5, 5);
this.dy = getRandomInt(-5, 5);
}
drawRect() {
ctx.beginPath();
ctx.fillStyle = 'green';
ctx.arc(this.x, this.y, 10, 0, Math.PI * 2, false);
ctx.fill();
if (this.x < 0 || this.x > canvas.width) {
this.dx = this.dx * -1;
}
if (this.y < 0 || this.y > canvas.height) {
this.dy = this.dy * -1;
}
this.x = this.x + this.dx;
this.y = this.y + this.dy;
}
}
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min)) + min; //The maximum is exclusive and the minimum is inclusive
}
前回との違いは、
for (let i = 0; i < NUM; i++) {
moveRect[i] = new Mover();
}
initializeの中のこの部分と、
for (let i = 0; i < NUM; i++) {
moveRect[i].drawRect();
}
renderの中のこの部分です。
配列にして100回繰り返しています。
参考にしたもの
参考にしているのは、前回に引き続き、田所淳さんの『Processing クリエイティブ・コーディング入門−コードが生み出す創造表現』です。
こちらは、CanvasではなくProcessingの本なのですが、考え方は同じなのですごく参考になります。
ブラウザで確認
ブラウザで見たい方は下のサイトにあります。私の個人サイト(素材置場)です。
この記事が気に入ったらサポートをしてみませんか?