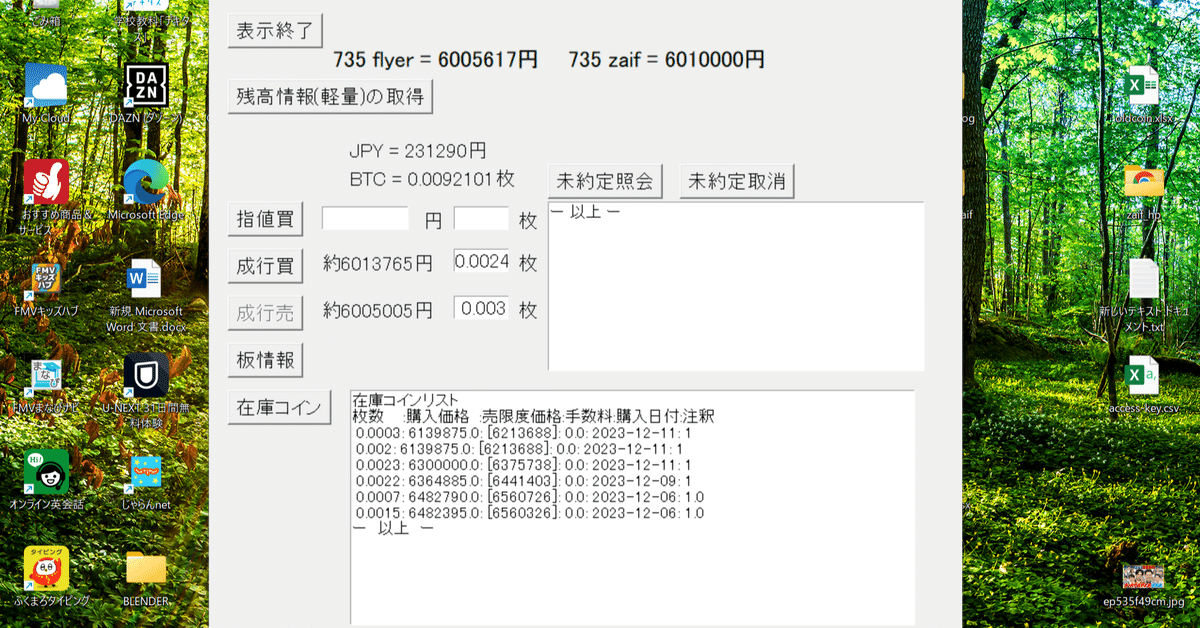
暗号資産・販売所ザイフ(zaif) pythonによるzaifAPIを用いたコイン在庫管理
zaifAPIのユーザー自身の取引履歴から現在の在庫コインの購入価格、枚数、を抽出し、利益の出る最低売り価格を計算します。
ただし、コインの売買には条件が付きます。その条件を満たした場合に限ります。
条件1)買い値は自由ですが、commentに希望利益率(%)を設定すること。指値または成行買いとします。
条件2)売りは買い値に買い手数料と売り手数料を考慮した上希望利益率以上で成行売りのみとします。指値売りはできません。
条件3)APIを用いない直接注文は禁止です。行った場合は動作は保証できません。また、既に取引を行っている場合は、一旦、全BTCコインを売却する必要が有ります。
以下にサンプル・プログラムを公開しています。
from zaifapi import ZaifPublicApi,ZaifTradeApi
zaif_public = ZaifPublicApi()
#from zaifapi import ZaifTradeApi
KEY = "Zaifで取得したkeyをコピペ" # Zaifで取得したkeyをコピペ
SECRET = "Zaifで取得したsecretをコピペ" # Zaifで取得したsecretをコピペ
zaif_trade = ZaifTradeApi(KEY,SECRET)
import time
import threading
import tkinter
import tkinter.messagebox
import requests
import json
import math
from datetime import datetime, timedelta
#import datetime
loop = 1260
hei = 680
count = -1
root = tkinter.Tk()
root.title("暗号資産・販売所ザイフ")
root.geometry("770x600")
#subw = tkinter.Toplevel()
#subw.title("単純移動平均")
#subw.geometry("1280x710")
#ca = tkinter.Canvas(subw,width=loop, height=hei, bg="white")
#ca.pack()
#me = tkinter.Message(subw, text="銭の生る樹", font=("Times New ZRoman", 12), width=500)
#me.place(x=20, y=100)
me_flyer = tkinter.Message(root, text="銭の生る樹", font=("Times New ZRoman", 16), width=500)
me_flyer.place(x=130, y=10)
me_zaif = tkinter.Message(root, text="銭の生る樹", font=("Times New ZRoman", 31), width=500)
me_zaif.place(x=300, y=40)
#me2 = tkinter.Message(root, text="countがloopに成るとコイン価格推移グラフが表示されます", font=("Times New ZRoman", 12), width=550)
#me2.place(x=20, y=130)
mefunds_all = tkinter.Message(root, text="時価総額", font=("Times New ZRoman", 12), width=500)
mefunds_all.place(x=40, y=70)
mefunds_jpy = tkinter.Message(root, text="現金", font=("Times New ZRoman", 12), width=500)
mefunds_jpy.place(x=40, y=90)
mefunds_btc = tkinter.Message(root, text="コイン", font=("Times New ZRoman", 12), width=500)
mefunds_btc.place(x=40, y=110)
flag = "off"
histry1s = []
market = "bitflyer"
cnt = 0
# 現在の終値(bitflyer)を取得
def bit_flyer(cnt):
cnt = cnt + 1
res = requests.get('https://api.bitflyer.jp/v1/getboard?product_code=BTC_JPY')
if res.status_code != 200:
if cnt < 10:
time.sleep(1)
res = bit_flyer(cnt)
return res
else:
res = {'mid_price':0.0}
return res
pass
pass #print("現在の終値(bitflyer)を取得完了")
return res
# 現在の終値を取得
def last_price(cnt):
cnt = cnt + 1
try:
res = zaif_public.last_price('btc_jpy')
except:
if cnt < 10:
time.sleep(0.5)
res = last_price(cnt)
return res
else:
print("retry error")
return -1
pass
finally:
pass #print("現在の終値を取得完了")
return res
# 残高情報(軽量)の取得
def get_info2(cnt):
cnt = cnt + 1
try:
res = zaif_trade.get_info2()
except:
if cnt < 10:
time.sleep(0.5)
res = get_info2(cnt)
return res
else:
print("retry error")
return "retry error"
pass
finally:
print("残高情報(軽量)の取得完了")
return res
# 板情報の取得
def depth(cnt,pair):
cnt = cnt + 1
try:
res = zaif_public.depth(pair)
except: #catch
if cnt < 10:
time.sleep(0.5)
res = depth(cnt,pair)
return res
else:
print("retry error")
return -1
pass
finally:
print("板情報の取得完了")
return res
# 未約定注文一覧の取得
def active_orders(cnt,pair):
#print("未約定注文一覧の取得")
cnt = cnt + 1
try:
res = zaif_trade.active_orders(currency_pair=pair)
except: #catch
if cnt < 10:
time.sleep(0.5)
res = active_orders(cnt,pair)
return res
else:
print("retry error")
return -1
pass
finally:
print("未約定注文一覧の取得完了")
return res
# ユーザー自身の取引履歴を取得
def trade_history(cnt,pair):
#print("ユーザー自身の取引履歴を取得開始")
cnt = cnt + 1
try:
res = zaif_trade.trade_history(currency_pair=pair)
except: #catch
if cnt < 10:
time.sleep(0.5)
res = trade_history(cnt,pair)
return res
else:
print("retry error")
return -1
finally:
print("ユーザー自身の取引履歴を取得終了")
return res
def trade(cnt,pair,action,price,amount,comment):
print("*** trade start ***")
cnt = cnt + 1
try:
res = zaif_trade.trade(currency_pair=pair, action=action, price=price, amount=amount,comment=comment)
except: #catch
if cnt < 10:
time.sleep(0.5)
res = trade(cnt,pair,action,price,amount,comment)
return res
finally:
print("注文完了")
pass
print("*** trade end ***")
return res
def cancel_order(cnt,pair,order_id):
cnt = cnt + 1
try:
res = zaif_trade.cancel_order(currency_pair=pair,order_id=order_id)
except: #catch
if cnt < 10:
time.sleep(0.5)
res = cancel_order(cnt,pair,order_id)
return res
finally:
print("注文の取消し完了")
return res
def key_func(n):
return n['limit']
def func1():
global flag
global loop
global ca
global count
global loop
global histry1s
while True:
time.sleep(1)
count = count + 1
# bitflyer
#res = requests.get('https://api.zaif.jp/api/1/last_price/btc_jpy')
#print("現在の終値(bitflyer)を取得開始")
#res = bit_flyer(0)
#print("現在の終値(bitflyer)を取得完了")
#price = json.loads(res.text)
#graph_y = int(price["mid_price"])
#me_flyer["text"] = "{} bitflyer = {:,}円".format(count,graph_y)
# zaif
#print("現在の終値を取得開始")
res = last_price(0)
#print("現在の終値を取得完了")
graph_y = int(res["last_price"])
me_zaif["text"] = "{} zaif = {:,}円".format(count,graph_y)
pass
def btn_on1():
global count
count = count + 1
# bitflyer
#res = requests.get('https://api.zaif.jp/api/1/last_price/btc_jpy')
res = requests.get('https://api.bitflyer.jp/v1/getboard?product_code=BTC_JPY')
if res.status_code != 200:
raise Exception('return status code is {}'.format(res.status_code))
price = json.loads(res.text)
graph_y = int(price["mid_price"])
me_flyer["text"] = "{} bitflyer = {:,}円".format(count,graph_y)
# zaif
#print("現在の終値を取得開始")
res = last_price(0)
#print("現在の終値を取得完了")
graph_y = int(res["last_price"])
me_zaif["text"] = "{} zaif = {:,}円".format(count,graph_y)
btn_bu_sign()
def btn_on2():
global flag
global bu2
bu2["text"] = "現在価格"
#flag = "on"
#time.sleep(1)
#if flag == "off":
thread_2 = threading.Thread(target=btn_on1)
thread_2.start()
pass
def btn_on3():
global bu3
#_残高情報(軽量)の取得
print("残高情報(軽量)の取得")
res = get_info2(0)
print("残高情報(軽量)の取得完了")
funds_jpy = res["funds"]["jpy"]
print("jpy:{:,}円".format(int(funds_jpy)))
mefunds_jpy["text"] = "JPY = {:,}円".format(int(funds_jpy))
funds_btc = res["funds"]["btc"]
print("btc:" + str(funds_btc))
mefunds_btc["text"] = "BTC = {:.4f}枚".format(float(funds_btc))
print("現在の終値を取得")
res = last_price(0)
print("現在の終値を取得完了 {} {}".format(res,type(res)))
act_price = float(res["last_price"])
funds_all = funds_jpy + funds_btc * act_price
mefunds_all["text"] = "時価総額 : {:,}円".format(int(funds_all))
def btn_bu_buy():
global bu_buy
buy_price = en_price.get()
if buy_price == "":
tkinter.messagebox.showinfo("エラー","買値を指定して下さい")
return
else:
int_price = int(buy_price)
pass
buy_amount = en_amount.get()
if buy_amount == "":
tkinter.messagebox.showinfo("エラー","枚数を指定して下さい")
return
else:
float_amount = float(buy_amount)
pass
get_ritu = en_get_ritu.get()
if get_ritu == "":
tkinter.messagebox.showinfo("エラー","利益率を指定して下さい")
return
pass
res = tkinter.messagebox.askokcancel("確認","買いを実行しますか?")
if res:
# 買い注文開始
res = trade(0,"btc_jpy","bid",int_price,float_amount,get_ritu)
# 買い注文完了
print("買い注文ID:"+str(res["order_id"]))
tkinter.messagebox.showinfo("確認","買いを発注しました。")
btn_bu_contract()
else:
tkinter.messagebox.showinfo("確認","キャンセルしました。")
pass
def btn_bu_bid():
#_現在値の取得
print("現在の終値を取得")
res = last_price(0)
print("現在の終値を取得完了 {} {}".format(res,type(res)))
act_price = float(res["last_price"])
#_残高情報(軽量)の取得
print("残高情報(軽量)の取得")
res = get_info2(0)
print("残高情報(軽量)の取得完了")
funds_jpy = float(res["funds"]["jpy"])
print("jpy:{:,}円".format(funds_jpy))
funds_btc = float(res["funds"]["btc"])
print("btc:{:,}円".format(funds_btc))
buy_money = (funds_jpy + funds_btc * act_price) // 20
print("板情報の取得開始")
res = depth(0,"btc_jpy")
print("板情報の取得完了")
bid_price = int(res["asks"][0][0])
bid_amount = round(float(buy_money / bid_price),4)
if bid_amount < 0.001:
tkinter.messagebox.showinfo("エラー","資金が足りません。")
return
pass
get_ritu = en_get_ritu.get()
if get_ritu == "" or float(get_ritu) < 0.0:
tkinter.messagebox.showinfo("エラー","利益率を指定して下さい")
return
pass
answer = tkinter.messagebox.askokcancel("確認","買値={}円 枚数={}枚 利益率={}% で買いますか?".format(bid_price,bid_amount,get_ritu))
if answer:
# 買い注文開始
res = trade(0,"btc_jpy","bid",bid_price,bid_amount,get_ritu)
# 買い注文完了
tkinter.messagebox.showinfo("通知","買いを発注しました。")
print("買い注文ID:"+str(res["order_id"]))
else:
tkinter.messagebox.showinfo("通知","買いを取消しました。")
pass
def coin_check(stock_table,ask_table,stock): ########
bid_coins = 0 # 買気配枚数累計
for i in range(len(ask_table)): # 買い気配テーブル
bid_coins = bid_coins + ask_table[i][1]
print("買い気配 = {} 枚数 = {}".format(ask_table[i][0],ask_table[i][1]))
sell_ok_coins = 0 # 売却可能コイン 累計
for j in range(len(stock_table)):
if stock_table[j]['limit'] <= ask_table[i][0]:
sell_ok_coins = sell_ok_coins + stock_table[j]['amount'] - stock_table[j]['fee_amount']
pass
pass
if sell_ok_coins <= bid_coins or sell_ok_coins == 0:
break # 売却可能コイン数より買気配累計が多い
pass
pass
print("sell_ok_coins = {}".format(sell_ok_coins))
sells = sell_ok_coins
sell_price = ask_table[i][0]
print("sells = {}".format(sells))
list = {'coins':sells,'price':sell_price}
print(list)
return list
def btn_sell_quick():
own_histry = []
#_未約定注文一覧の取得
print("未約定注文一覧の取得")
res = active_orders(0,"btc_jpy")
print("未約定注文一覧の取得完了")
if len(res) > 0:
answer = tkinter.messagebox.askokcancel("確認","未約定注文があります。\n注文を取消し、成行売り注文を続行しますか?")
if answer:
for id in res:
print(id)
key = str(id)
print('currency_pair = '+ res[key]['currency_pair'])
print('action = ' + res[key]['action'])
print('amount = ' +str(res[key]['amount']))
print('price = ' +str(res[key]['price']))
print('timestamp = ' +str(res[key]['timestamp']))
print('comment = ' +str(res[key]['comment']))
#_注文の取消し
print("注文の取消し")
cancel_id = int(id)
# 注文の取消し開始
res = cancel_order(0,"btc_jpy",cancel_id)
# 注文の取消し完了
print("取消ID:"+str(res["order_id"]))
pass
tkinter.messagebox.showinfo("確認","取り消しました。")
else:
tkinter.messagebox.showinfo("通知","売りを取消しました。")
return
print("板情報の取得開始")
res = depth(0,"btc_jpy")
print("板情報の取得完了")
ask_table = res["bids"]
#_残高情報(軽量)の取得
print("残高情報(軽量)の取得")
res = get_info2(0)
print("残高情報(軽量)の取得完了")
funds_jpy = res["funds"]["jpy"]
mefunds_jpy["text"] = "JPY = {}円".format(str(int(funds_jpy)))
funds_btc = res["funds"]["btc"]
mefunds_btc["text"] = "BTC = {:.4f}枚".format(float(funds_btc))
print("btc:" + str(funds_btc))
if funds_btc < 0.001:
print("在庫コインが有りません")
return
pass
res = coin_valid(funds_btc) # valid
own_histry = res['table']
stock_coin = coin_stock(own_histry,funds_btc)
print("len(stock_coin) = {}".format(len(stock_coin)))
res = coin_check(stock_coin,ask_table,funds_btc)
print(res)
if res['coins'] == 0:
tkinter.messagebox.showinfo("通知","利益の出るコインがありません。")
print("*** 利益の出るコインがありません。 ***")
return
else:
sell_coin = round(res["coins"],4)
sell_price = res["price"]
pass
answer = tkinter.messagebox.askokcancel("確認","売値={}円 枚数={}枚 で売りますか?".format(sell_price,sell_coin))
if answer:
# 売り注文開始
res = trade(0,"btc_jpy","ask",sell_price,sell_coin,"")
# 売り注文終了
tkinter.messagebox.showinfo("通知","売りを発注しました。")
print("売り注文ID:"+str(res["order_id"]))
else:
tkinter.messagebox.showinfo("通知","売りを取消しました。")
pass
def btn_bu_cancel():
global bu_cancel
res = tkinter.messagebox.askyesno("確認","取消しを実行しますか?")
if res:
#_未約定注文一覧の取得
print("未約定注文一覧の取得")
res = active_orders(0,"btc_jpy")
print("未約定注文一覧の取得完了")
for id in res:
print(id)
key = str(id)
print('currency_pair = '+res[key]['currency_pair'])
print('action = '+res[key]['action'])
print('amount = '+str(res[key]['amount']))
print('price = '+str(res[key]['price']))
print('timestamp = '+str(res[key]['timestamp']))
print('comment = '+str(res[key]['comment']))
#_注文の取消し
print("注文の取消し")
cancel_id = int(id)
# 注文の取消し開始
res = cancel_order(0,"btc_jpy",cancel_id)
# 注文の取消し完了
print("取消ID:"+str(res["order_id"]))
tkinter.messagebox.showinfo("確認","取り消しました。")
btn_bu_contract()
def btn_bu_contract():
global bu_contract
#_未約定注文一覧の取得
print("未約定注文一覧の取得")
res = active_orders(0,"btc_jpy")
print("未約定注文一覧の取得完了")
te.delete("1.0",tkinter.END)
for id in res:
print(id)
key = str(id)
#te.insert(tkinter.END,id+" ")
print('currency_pair = {} '.format(res[key]['currency_pair']))
te.insert(tkinter.END,res[key]['currency_pair']+" : ")
print('action = {} '.format(res[key]['action']))
te.insert(tkinter.END,res[key]['action']+" : ")
print('amount = {} '.format(str(res[key]['amount'])))
te.insert(tkinter.END,str(res[key]['amount'])+" : ")
print('price = {} '.format(str(res[key]['price'])))
te.insert(tkinter.END,str(res[key]['price'])+" : ")
print('timestamp = {} '.format(str(res[key]['timestamp'])))
#te.insert(tkinter.END,str(res[key]['timestamp'])+" : ")
print('comment = {} '.format(str(res[key]['comment'])))
te.insert(tkinter.END,str(res[key]['comment'])+"\n")
#te.insert(tkinter.END,'\n')
te.insert(tkinter.END,"ー 以上 ー")
def coin_valid(hold_coins ):
print('coin_valid({:.4f}) start'.format(hold_coins))
#_ユーザー自身の取引履歴を取得
print("ユーザー自身の取引履歴を取得")
res = trade_history(0,"btc_jpy")
print("ユーザー自身の取引履歴の取得完了")
print('len(trade_history) = {} '. format(len(res)))
after = []
for id in res:
key = str(id)
#if res[key]['comment'] != "": # BOTでの取引のみを対象とする場合有効にする。
#after.append(res[key])
after.append(res[key])
#pass
if res[key]['your_action'] == "ask":
hold_coins = hold_coins + res[key]['amount']
pass
if res[key]['your_action'] == "bid":
hold_coins = hold_coins - (res[key]['amount'] - res[key]['fee_amount'])
pass
if hold_coins < 0.001 and hold_coins != 0.0: # hold_coins == 0.0 全売却後の時0start
break
pass
pass
print('len(after) = {} '. format(len(after)))
bot_coins = 0
for i in range(len(after)-1,-1,-1):
if after[i]['your_action'] == "bid":
bot_coins = bot_coins + (after[i]['amount'] - after[i]['fee_amount'])
pass
if after[i]['your_action'] == "ask":
bot_coins = bot_coins - after[i]['amount']
pass
pass
print('bot_coins = {} hold_coins = {}'. format(bot_coins,hold_coins))
if bot_coins < 0.0:
bot_coins = 0.0
pass
last_order_price = after[0]["price"]
last_order_action = after[0]["your_action"]
list = {'table':after,'bot_coins':bot_coins,'last_order_price':last_order_price,'last_order_action':last_order_action}
print('coin_valid() end')
return list
def coin_stock(after,bot_coins):
print('coin_stock() start')
dt=datetime.now()
#dt=datetime.date.today()
today_s = dt.strftime("%Y-%m-%d")
print("today_s ",today_s)
flag = "undo"
picup = []
picup = after
#ask_priceL = 0 # 上限売価格 売ってはいけないコイン
# 売っても良いコイン
ask_priceS = 0 # 下限売価格 既に売られたコイン
coins = 0
list = []
for i in range(len(picup)):
if picup[i]['your_action'] == "ask":
ask_flag = "ask"
if picup[i]['price'] >= ask_priceS:
ask_priceS = picup[i]['price']
pass
elif picup[i]['your_action'] == "bid":
limit_line = picup[i]['price'] * picup[i]['amount'] / (picup[i]['amount'] - picup[i]['fee_amount']) * 1.001
if picup[i]['comment'] == "":
profit = 0.0 # coin_valid で抽出しない場合は此処は実行されない
else:
profit = float(picup[i]['comment'])
pass
limit_line = limit_line * (1.0 + profit /100) # 限界価格 売買手数料や利益率を考慮した販売設定価格
if limit_line > ask_priceS: # 未だ売られていない注文(コイン)
coins = coins + picup[i]['amount'] - picup[i]['fee_amount'] # 保有コイン枚数を算出する
if coins >= bot_coins: # BOTでの購入コイン数を超えたか?
flag = "break"
pass
int_limit_line = int(limit_line)
dt = datetime.fromtimestamp(int(picup[i]['timestamp']))
check_str_day = dt.strftime("%Y-%m-%d")
part = {'amount' : picup[i]['amount'] # 購入枚数
,'price' : picup[i]['price'] # 購入価格
,'limit' : int_limit_line # 最低売却価格
,'fee_amount': picup[i]['fee_amount'] # 購入手数料
,'date' : check_str_day # 購入日付
,'comment' : picup[i]['comment'] # 利益率
}
print(part)
list.append(part)
pass
pass
if flag == "break":
break
pass
return list
def btn_coin_list():
print("残高情報(軽量)の取得")
#_残高情報(軽量)の取得
print("残高情報(軽量)の取得")
res = get_info2(0)
print("残高情報(軽量)の取得完了")
funds_jpy = res["funds"]["jpy"]
mefunds_jpy["text"] = "JPY = {}円".format(str(int(funds_jpy)))
funds_btc = res["funds"]["btc"]
mefunds_btc["text"] = "BTC = {:.4f}枚".format(float(funds_btc))
if funds_btc >= 0.001:
print("funds_btc = {:.4f}".format(funds_btc))
res = coin_valid(funds_btc) # valid
after = res['table']
bot_coins = res['bot_coins']
print("bot_coins = {} funds_btc = {}".format(bot_coins,funds_btc))
list = coin_stock(after,bot_coins) # coin_stock
print("len(list) = {}".format(len(list)))
print(min(list, key=key_func))
te_message.delete("1.0",tkinter.END)
te_message.insert(tkinter.END," 在庫コインリスト\n")
te_message.insert(tkinter.END, " 枚数 : 購入価格 : 売限度額 : 手数料 : 購入日付 : 利益率\n")
for i in range(len(list)):
te_message.insert(tkinter.END, " {:.4f} : ".format(list[i]['amount']))
te_message.insert(tkinter.END, " {} : ".format(list[i]['price']))
te_message.insert(tkinter.END, " [{}] : ".format(list[i]['limit']))
te_message.insert(tkinter.END, " {:f} : ".format(list[i]['fee_amount']))
te_message.insert(tkinter.END, " {} : ".format(list[i]['date']))
te_message.insert(tkinter.END, " {}\n".format(list[i]['comment']))
pass
te_message.insert(tkinter.END," \n最小販売可能価格\n")
te_message.insert(tkinter.END, " 枚数 : 購入価格 : 売限度額 : 手数料 : 購入日付 : 利益率\n")
te_message.insert(tkinter.END, " {:.4f} : ".format(min(list, key=key_func)['amount']))
te_message.insert(tkinter.END, " {} : ".format(min(list, key=key_func)['price']))
te_message.insert(tkinter.END, " [{}] : ".format(min(list, key=key_func)['limit']))
te_message.insert(tkinter.END, " {:f} : ".format(min(list, key=key_func)['fee_amount']))
te_message.insert(tkinter.END, " {} : ".format(min(list, key=key_func)['date']))
te_message.insert(tkinter.END, " {}\n".format(min(list, key=key_func)['comment']))
else:
te_message.delete("1.0",tkinter.END)
te_message.insert(tkinter.END, "コインはありません。\n\n")
pass
te_message.insert(tkinter.END, "ー 以上 ー\n")
def trade_check(table,bot_coins):
print("*** trade_check(tablle,{:.4f}) start ***".format(bot_coins))
coin_count = 0
ask_moneys = 0
bid_moneys = 0
fee_moneys = 0
flag = "none"
ask_priceL = 0 # 上限売価格 売ってはいけないコイン
# 売っても良いコイン
ask_priceS = 0 # 下限売価格 既に売られたコイン
hand_coin = 0
table2 = []
#dt=datetime.date.today()
#base_date = dt.strftime("%Y-%m-%d")
yesterday = datetime.now() - timedelta(1)
base_date = yesterday.strftime('%Y-%m-%d')
print("base_date = {}".format(base_date))
if len(table) > 0:
print("報告日の売却コイン枚数を算出する")
print("len(table) = {} 有効注文データ数".format(len(table)))
for i in range(len(table)): # 報告日の売却コイン枚数を算出する。
dt = datetime.fromtimestamp(int(table[i]['timestamp']))
check_date = dt.strftime("%Y-%m-%d")
if time.strptime(check_date,"%Y-%m-%d") <= time.strptime(base_date,"%Y-%m-%d"):
if table[i]['your_action'] == "ask" or flag == "start": # 直近ask と それ以後の取引
if flag == "none":
ask_priceL = table[i]['price'] # 直近のaskを上限売価格とする
ask_priceS = 0
flag = "start" # 抽出開始
pass
if table[i]['your_action'] == "ask":
if time.strptime(check_date,"%Y-%m-%d") == time.strptime(base_date,"%Y-%m-%d"):
coin_count = coin_count + table[i]['amount'] # 売却コイン枚数 累計
ask_moneys = ask_moneys + table[i]['amount'] * table[i]['price'] # 収益金 累計
fee_moneys = fee_moneys + table[i]['fee_amount'] # askはfee_amountに手数料金が設定されている。
pass
pass
table2.append(table[i]) # bid と 報告日以外のask を抽出する
else:
pass # 直近ask以前の取引は全て破棄
else:
print("対象外 check_date {} <= base_date {}".format(check_date,base_date))
pass
pass
print("coin_count = {} ask_moneys = {:.1f} fee_moneys = {:.1f}".format(coin_count,ask_moneys,fee_moneys))
else:
print("データがありません")
pass
counter2 = 0
coins = 0
info = []
ask_priceL = 0 # 上限売価格 売ってはいけないコイン
# 売っても良いコイン
ask_priceS = 0 # 下限売価格 既に売られたコイン
print("len(table2) = {}".format(len(table2)))
print("売却コイン枚数 = {:.4f}枚".format(coin_count)) # 売却コイン枚数 累計
print("収益金 累計 = {:.1f}円".format(ask_moneys)) # 収益金 累計
print("手数料 累計 = {:.1f}円".format(fee_moneys)) # 手数料 累計
# debug start
#for i in range(len(table2)):
#p_1 = table2[i]['currency_pair']
#p_2 = table2[i]['action']
#p_3 = table2[i]['amount']
#p_4 = table2[i]['price']
#p_5 = table2[i]['fee']
#p_6 = table2[i]['fee_amount']
#p_7 = table2[i]['your_action']
#p_8 = table2[i]['bonus']
#dt = datetime.fromtimestamp(int(table2[i]['timestamp']))
#display_date = dt.strftime("%Y-%m-%d %H:%M:%S")
#p_9 = display_date
#p_0 = table2[i]['comment']
#print("{} {} {} {:.4f} {} {:.1f} {:.6f} {} {} {} {}".format(i,p_1,p_2,p_3,p_4,p_5,p_6,p_7,p_8,p_9,p_0))
#pass
# debug end
flag = "start"
table3 = []
if len(table2) > 0 and coin_count != 0:
print("売却コインの仕入れ価格を抽出する")
for i in range(len(table2)):
dt = datetime.fromtimestamp(int(table2[i]['timestamp']))
display_date = dt.strftime("%Y-%m-%d %H:%M:%S")
if table2[i]['your_action'] == "ask":
if flag == "start": # 最初のask (必ず最初はask)
ask_priceL = table2[i]['price']
flag = "undo"
elif table2[i]['price'] > ask_priceL:
dt = datetime.fromtimestamp(int(table2[i]['timestamp']))
check_date = dt.strftime("%Y-%m-%d")
if time.strptime(check_date,"%Y-%m-%d") == time.strptime(base_date,"%Y-%m-%d"):
ask_priceL = table2[i]['price'] # 報告日ならask_priceLを更新して継続
else:
if table2[i]['price'] > ask_priceS: # 既に売却済みの価格を設定
ask_priceS = table2[i]['price']
pass
if ask_priceS >= ask_priceL:
flag = "break"
pass
pass
else:
pass
pass
pass
if table2[i]['your_action'] == "bid":
limit_line = table2[i]['price'] * table2[i]['amount'] / (table2[i]['amount'] - table2[i]['fee_amount']) * 1.001
profit = float(table2[i]['comment'])
limit_line = int(limit_line * (1.0 + (profit /100))) # 最低売却価格を算出
if ask_priceS < limit_line and limit_line < ask_priceL: # 仕入れ買い注文 ?
add_coin = table2[i]['amount'] - table2[i]['fee_amount'] # 購入コイン枚数を算出
coins = coins + add_coin # 購入コイン枚数を加算
if coins >= coin_count:
flag = "break"
if coins == coin_count:
plus = add_coin
bid_moneys = bid_moneys + plus * table2[i]['price'] # 総仕入れ額
fee_moneys = fee_moneys + table2[i]['fee_amount'] * table2[i]['price']
table3.append(table2[i])
else: # coins > coin_count
plus = add_coin - (coins - coin_count)
bid_moneys = bid_moneys + plus * table2[i]['price']
fee_moneys = fee_moneys + table2[i]['fee_amount'] * table2[i]['price']
else:
bid_moneys = bid_moneys + table2[i]['amount'] * table2[i]['price']
fee_moneys = fee_moneys + table2[i]['fee_amount'] * table2[i]['price']
table3.append(table2[i])
else:
print("対象外bid")
pass # 対象外bid
pass
else:
pass
if flag == "break":
break
pass
else:
print("収支はありません")
pass
print("**** table3 **** {} ".format(len(table3)))
for i in range(len(table3)):
disp_your_action = table3[i]['your_action']
disp_price = table3[i]['price']
disp_amount = table3[i]['amount']
disp_fee_amount = table3[i]['fee_amount']
dt = datetime.fromtimestamp(int(table3[i]['timestamp']))
display_date = dt.strftime("%Y-%m-%d %H:%M:%S")
print("{} {} {:.1f} {:.4f} {:.6f} date = {}".format(i,disp_your_action,disp_price,disp_amount,disp_fee_amount,display_date))
pass
list = {"date" :base_date
,"ask_moneys":ask_moneys
,"bid_moneys":bid_moneys
,"fee_moneys":fee_moneys
,"coins" :coins
,"coin_count":coin_count
}
print(list)
return list
def btn_gain_report():
print("*** 昨日の収支報告 ***")
#_残高情報(軽量)の取得
print("残高情報(軽量)の取得")
res = get_info2(0)
print("残高情報(軽量)の取得完了")
funds_jpy = res["funds"]["jpy"]
mefunds_jpy["text"] = "JPY = {}円".format(str(int(funds_jpy)))
funds_btc = res["funds"]["btc"]
mefunds_btc["text"] = "BTC = {:.4f}枚".format(float(funds_btc))
if funds_btc > 0.001:
res = coin_valid(funds_btc) # valid
else:
res = coin_valid(0.0) # valid 全コイン売却時
pass
after = res['table']
bot_coins = res['bot_coins']
list = trade_check(after,bot_coins)
print("len(list) = {}".format(len(list)))
te_message.delete("1.0",tkinter.END)
te_message.insert(tkinter.END,"収支報告\n")
#te_message.insert(tkinter.END, "枚数 :購入価格 :売限度額 :手数料:購入日付:利益率\n")
te_message.insert(tkinter.END, "報告日付 = {}\n".format(list["date"]))
te_message.insert(tkinter.END, "売却金額 = {:.1f}\n".format(list["ask_moneys"]))
te_message.insert(tkinter.END, "仕入れ金額 = {:.1f}\n".format(list["bid_moneys"]))
te_message.insert(tkinter.END, "取引手数料 = {:.2f}\n".format(list["fee_moneys"]))
te_message.insert(tkinter.END, "仕入れ枚数 = {:.4f}\n".format(list["coins"]))
te_message.insert(tkinter.END, "売却枚数 = {:.4f}\n\n".format(list["coin_count"]))
total = list["ask_moneys"] - list["bid_moneys"]
te_message.insert(tkinter.END, "昨日の収支は{:,.0f}円の利益がありました。\n\n".format(total))
te_message.insert(tkinter.END, "ー 以上 ー\n")
def btn_bu_sign():
global bu_sign
#_板情報の取得
print("板情報の取得開始")
res = depth(0,"btc_jpy")
print("板情報の取得完了")
btc_ask_price = int(res["asks"][0][0])
btc_ask_amount = round(float(res["asks"][0][1]), 4)
me_bid_price["text"] = "売{:,}円".format( btc_ask_price)
en_bid_amount.delete(0,tkinter.END)
en_bid_amount.insert(tkinter.END,btc_ask_amount)
print("売気配値:" + str(btc_ask_price) + " " + str(btc_ask_amount))
btc_bid_price = int(res["bids"][0][0])
btc_bid_amount = round(float(res["bids"][0][1]), 4)
me_ask_price["text"] = "買{:,}円".format(btc_bid_price)
en_ask_amount.delete(0,tkinter.END)
en_ask_amount.insert(tkinter.END,btc_bid_amount)
print("買気配値:" + str(btc_bid_price) + " " + str(btc_bid_amount))
bu2 = tkinter.Button(root, text="現在価格", font=("Times New ZRoman", 12), command=btn_on2)
bu2.place(x=20, y=10)
bu3 = tkinter.Button(root, text="残高情報(軽量)の取得", font=("Times New ZRoman", 12), command=btn_on3)
bu3.place(x=20, y=40)
bu_buy = tkinter.Button(root, text="指値買", font=("Times New ZRoman", 12), command=btn_bu_buy)
bu_buy.place(x=20, y=145)
en_price = tkinter.Entry(root, width=8, font=("Times New ZRoman",12), justify=tkinter.RIGHT)
en_price.place(x=120, y=145)
me_price = tkinter.Message(root, text="円", font=("Times New ZRoman", 12), width=500)
me_price.place(x=210, y=145)
en_amount = tkinter.Entry(root, width=6, font=("Times New ZRoman",12), justify=tkinter.RIGHT)
en_amount.place(x=250, y=145)
me_amount = tkinter.Message(root, text="枚", font=("Times New ZRoman", 12), width=500)
me_amount.place(x=318, y=145)
bu_bid = tkinter.Button(root, text="成行買", font=("Times New ZRoman", 12), command=btn_bu_bid)
bu_bid.place(x=20, y=180)
me_bid_price = tkinter.Message(root, text="売り気配値", font=("Times New ZRoman", 12), width=500)
me_bid_price.place(x=110, y=180)
en_bid_amount = tkinter.Entry(root, width=6, font=("Times New ZRoman",12), justify=tkinter.RIGHT)
en_bid_amount.place(x=250, y=180)
me_bid_amount = tkinter.Message(root, text="枚", font=("Times New ZRoman", 12), width=500)
me_bid_amount.place(x=318, y=180)
bu_ask = tkinter.Button(root, text="成行売", font=("Times New ZRoman", 12), command=btn_sell_quick)
bu_ask.place(x=20, y=215)
#bu_ask.configure(state="disabled")
me_ask_price = tkinter.Message(root, text="買い気配値", font=("Times New ZRoman", 12), width=500)
me_ask_price.place(x=110, y=215)
en_ask_amount = tkinter.Entry(root, width=6, font=("Times New ZRoman",12), justify=tkinter.RIGHT)
en_ask_amount.place(x=250, y=215)
me_ask_amount = tkinter.Message(root, text="枚", font=("Times New ZRoman", 12), width=500)
me_ask_amount.place(x=318, y=215)
bu_sign = tkinter.Button(root, text="板情報", font=("Times New ZRoman", 12), command=btn_bu_sign)
bu_sign.place(x=20, y=250)
me_msg_gain = tkinter.Message(root, text="利益率", font=("Times New ZRoman", 12), width=500)
me_msg_gain.place(x=110, y=250)
en_get_ritu = tkinter.Entry(root, width=5, font=("Times New ZRoman",12), justify=tkinter.RIGHT)
en_get_ritu.place(x=260, y=250)
en_get_ritu.insert(0,"1.0")
me_msg_amount = tkinter.Message(root, text="%", font=("Times New ZRoman", 12), width=500)
me_msg_amount.place(x=318, y=250)
bu_contract = tkinter.Button(root, text="未約定照会", font=("Times New ZRoman", 12), command=btn_bu_contract)
bu_contract.place(x=360, y=110)
bu_cancel = tkinter.Button(root, text="未約定取消", font=("Times New ZRoman", 12), command=btn_bu_cancel)
bu_cancel.place(x=500, y=110)
te = tkinter.Text(font=("Times New ZRoman", 13))
te.place(x=360,y=145,width=390,height=180)
#te.configure(state="disabled")
bu_valid = tkinter.Button(root, text="在庫コイン", font=("Times New ZRoman", 12), command=btn_coin_list)
bu_valid.place(x=20, y=340)
bu_gain = tkinter.Button(root, text="収支報告", font=("Times New ZRoman", 12), command=btn_gain_report)
bu_gain.place(x=20, y=375)
te_message = tkinter.Text(font=("Times New ZRoman", 13))
te_message.place(x=150,y=340,width=600,height=250)
#action = tkinter.Message(subw, text="状態", font=("Times New ZRoman", 12), width=150)
#action.place(x=150, y=50)
thread_1 = threading.Thread(target=func1)
thread_1.start()
#thread_2 = threading.Thread(target=func2)
#thread_2.start()
root.mainloop()
この記事が気に入ったらサポートをしてみませんか?