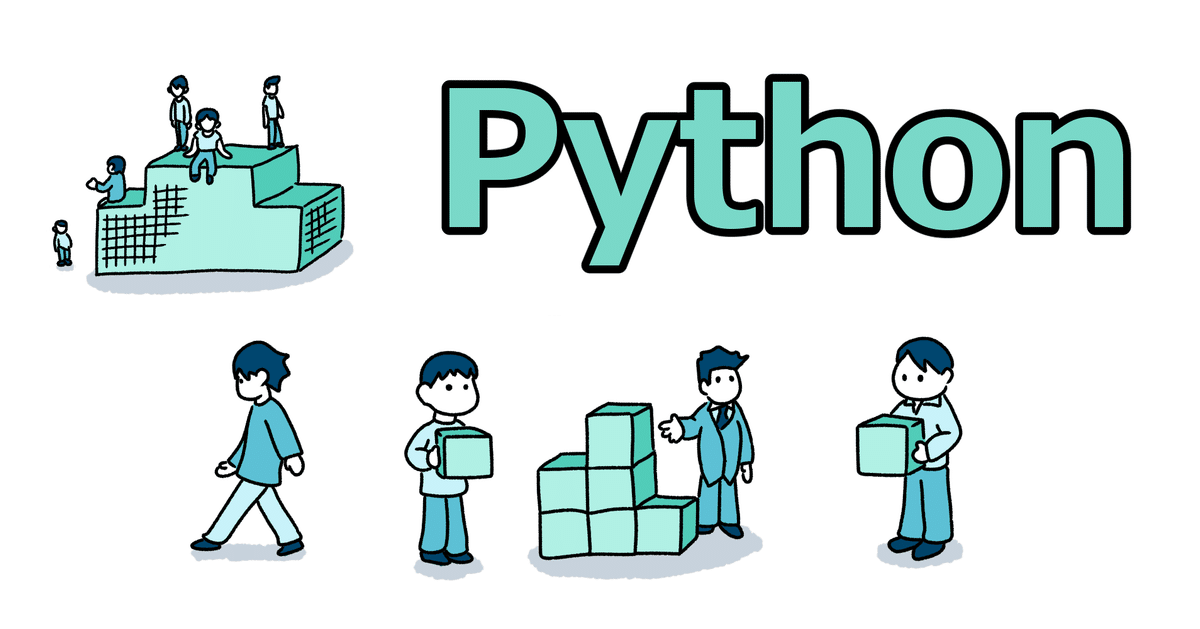
Python資格取得への道のり 5日目
・集合型
>>> a = {1, 2, 2, 3, 4, 4, 4, 5, 6}
>>> a
{1, 2, 3, 4, 5, 6}
>>> type(a)
<class 'set'>
>>> b = {2, 3, 3, 6, 7}
>>> b
{2, 3, 6, 7}
#a中でbにある値を引く
>>> a - b
{1, 4, 5}
>>> a
{1, 2, 3, 4, 5, 6}
>>> b
{2, 3, 6, 7}
#b中でaにある値を引く
>>> b - a
{7}
#aにもbにもある値
>>> a & b
{2, 3, 6}
>>>
#aかbのどちらかにある値
>>> a | b
{1, 2, 3, 4, 5, 6, 7}
>>>
#aかbにある値(かぶっているものは除く)
>>> a ^ b
{1, 4, 5, 7}
・集合のメソッド
集合には場所の概念がないため「s[0]」についてはエラーを返す。
ただ、値の追加・削除・全値の削除は可能である。
>>> s = { 1, 2, 3, 4, 5}
>>> s
{1, 2, 3, 4, 5}
>>>
>>> s[0]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'set' object is not subscriptable
>>> s.add(6)
>>> s
{1, 2, 3, 4, 5, 6}
>>> s.add(6)
>>> s
{1, 2, 3, 4, 5, 6}
>>> s.remove(6)
>>> s
{1, 2, 3, 4, 5}
>>> s.clear()
>>> s
set()
>>>
・集合型の使い所
「何かの共通点を見つけるときに使う」や「重複しているものを省く場合」
my_friend = {'A', 'C', 'D'}
A_friend = {'B', 'D', 'E', 'F'}
print(my_friend & A_friend)
f = ['apple', 'banana', 'apple', 'banana']
kind = set(f)
#set(f)にてfをリスト型から集合型へと変換し、kindという変数に代入した
print(kind)
セクション5 制御フローとコード構造
・if文
初めに条件に引っかかったときに値を返す
注意点としては、インテンドが重要。
1マスでもズレるとエラーを返すので、必ず確認する。
x = 0
if x < 0:
print('negative')
elif x ==0:
print('zero')
else:
print('positive')
# →zero
a = 5
b = 10
if a > 0:
print('a is positive')
if b > 0:
print('b is positive')
# →a is positive
# →b is positive
・InとNotの使い所
Notは「==」を否定し「not ==」のような使い方は好ましくない。
その場合、「!=」と表現した方が他者が読みやすいからである
では、どのようなときに使うか。それはboon型。
「is_ok」を否定するときは、notを使う方が良い。
y = [1, 2, 3]
x = 1
if x in y:
print('in')
# → in
if 100 not in y:
print('not in')
# → not in
#a = 1
#b = 2
#
#if not a == b:
# print('Not equal')
#
#if a != b:
# print('Not equal')
is_ok = True
if is_ok:
print('hello')
# → hello
if not is_ok:
print('hello')
・値が入っているか、入っていないかを判定する
変数に何か値が入っている場合は、「True」
変数に何も入っていない、もしくは0の場合は「False」を返す。
Falseの条件としては、0, 0.0, ' ', [ ], ( ), { }, set( )
is_ok = 1
if is_ok:
print('OK!')
else:
print('No!')
# → OK!
is_ok = 'abcdefg'
if is_ok:
print('OK!')
else:
print('No!')
# → OK!
is_ok = 0
if is_ok:
print('OK!')
else:
print('No!')
# → No!
is_ok = ''
if is_ok:
print('OK!')
else:
print('No!')
# → No!
・Noneを判定する場合
変数に何も入れたくない場合、「変数 = None」と定義する。
・「==」と「is」の違い
「==」は、値を比較し、「is」はオブジェクト単位まで確認を行う。
そのため変数が同じ値かどうか確認する場合は、「==」を使い、
「is」を使用する場合は、変数がNoneになるかを確認するために使用されることが多い。
is_empty = None
if is_empty == None:
print('None!')
# → None!
#あまり好ましくない表現
if is_empty is None:
print('None!')
# → None!
#適した表現
print(1 == True)
print(1 is True)
print(True is True)
# → True
# → False
# → True
・while文とcontinue文とbreak文
while文は条件を繰り返す際に使用
break文はwhile文から抜け出す際に使用
continue文はwhile文の条件下でスキップさせる条件を指定して例外対応ができる。
count = 0
while count < 5:
print(count)
count += 1
# → 0
# → 1
# → 2
# → 3
# → 4
・continue文の使い方
number = 1
while True:
if number > 5:
break
if number == 2:
number += 1
continue
print(number)
number += 1
# → 1
# → 3
# → 4
# → 5
# 2はcontinueでスキップされ、出力はされていない
・while else文とbreak文
else文とbreak文については、breakが優先となり、breakの条件になった際にはwhile、elseの両方から抜け出すこととなる。
count = 0
while count < 5:
print(count)
count += 1
else:
print('done')
# → 0
# → 1
# → 2
# → 3
# → 4
# → done
break文が途中にある場合の出力結果
count = 0
while count < 5:
if count == 1:
break
print(count)
count += 1
else:
print('done')
# → 0
・input関数
入力した値が決まったものではない場合、while文を繰り返す。
下記例は「OK」(大文字)が入力されない場合、くり返す例となる。
while True:
word = input('Enter:')
if word == "OK":
break
print('Next')
# → Enter:ok
# → Next
# → Enter:gp
# → Next
# → Enter:go
# → Next
# → Enter:OK
・wordのタイプを変え、数値にすることも可能である。
while True:
word = input('Enter:')
num = int(word)
if num == 3:
break
print('Next')
# → Enter:1
# → Next
# → Enter:2
# → Next
# → Enter:3
・for文とbreak文とcontinue文
forとwhileの違いとしては、forはインデックスを指定する必要がない。
具体例は下記。まずはwhile文
some_list = [1, 2, 3, 4, 5]
i = 0
while i < len(some_list):
print(some_list[i])
i += 1
# → 1
# → 2
# → 3
# → 4
# → 5
・for文の場合
some_list = [1, 2, 3, 4, 5]
for i in some_list:
print(i)
# → 1
# → 2
# → 3
# → 4
# → 5
for s in 'abcde':
print(s)
# → a
# → b
# → c
# → d
# → e
・for文とbreak文
for word in ['My' , 'name', 'is', 'Mike']:
if word == 'is':
continue
print(word)
# → My
# → name
・for文とcontinue文
for word in ['My' , 'name', 'is', 'Mike']:
if word == 'is':
continue
print(word)
# → My
# → name
# → Mike
・for else文
for fruits in ['apple' , 'banana', 'orange']:
print(fruits)
else:
print('I ate all.')
# → apple
# → banana
# → orange
# → I ate all.
・for else文とbreak文
for fruits in ['apple' , 'banana', 'orange']:
if fruits == 'orange':
print('stop eating')
break
print(fruits)
else:
print('I ate all.')
# → apple
# → banana
# → stop eating
今日はここまで!
制御フローにいよいよ入り、少しずつプログラミングになってきました。
まだまだ理解できるところですが、必ずつまづきがくると思いますので、それまで反復して下地をしっかりとつけていきます。
土日は時間が取れる。
平日もここまで時間が取れれば,,,,,,。
最初から読みたい方はどうぞ
この記事が気に入ったらサポートをしてみませんか?