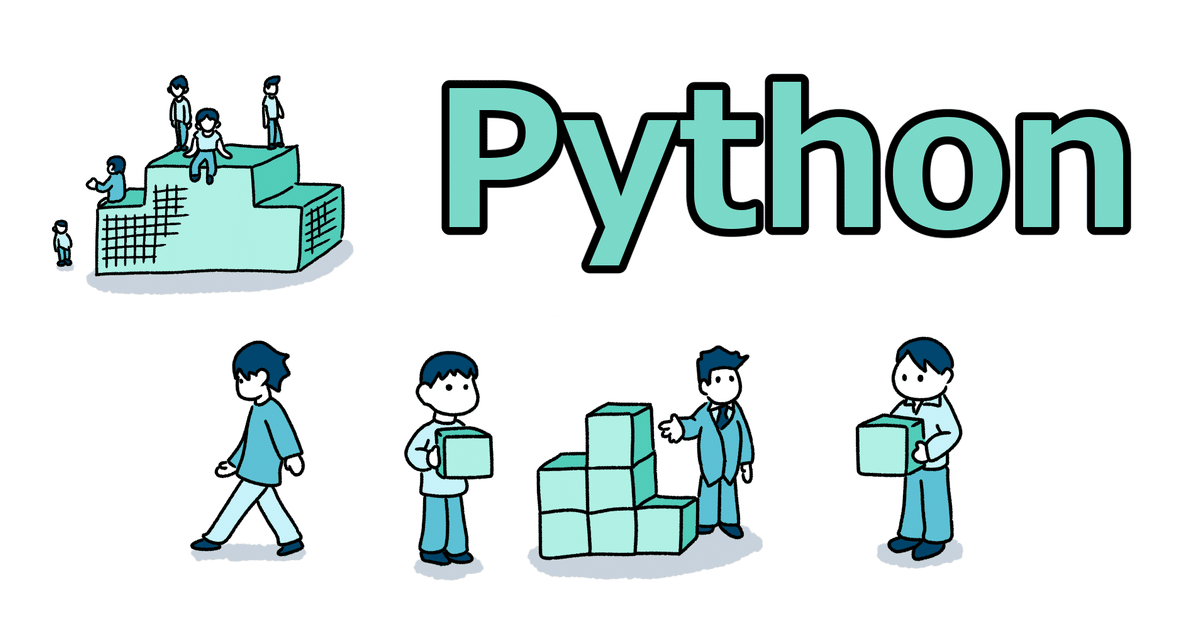
Photo by
golchiki
Python資格取得への道のり 3日目
・リストのメソッド
r = [1, 2, 3, 4, 5, 1, 2, 3]
print(r.index(3)]
# → 2
# 3はどのインデックスにいるのか教えてくれる
print(r.index(3, 3)]
# → 7
# print(r.index(値, 場所)]となり、2つの目の3はリストの7番目にあるため7を返す
print(r.count(3)]
# → 2
if 5 in r:
print('exist')
# → exist
r.sort()
print(r)
# → [1, 1, 2, 2, 3, 3, 4, 5]
r.sort(reverse=True)
print(r)
# → [5, 4, 3, 3, 2, 2, 1, 1]
r.reverse()
print(r)
# → [1, 1, 2, 2, 3, 3, 4, 5]
s = 'My name is Mike.'
to_split = s.split(' ')
print(to_split)
# → ['My', 'name', 'is', 'Mike.']
# スペースで分けて、リスト化してくれる
#to_split = s.split('!!!')
#print(to_split)
## → ['My name is Mike.']
## リスト化するが、分けれられない
x = ' '.join(to_split)
print(x)
# → My name is Mike.
x = ' ######### '.join(to_split)
print(x)
# → My ######### name ######### is ######### Mike.
print(help(list))
# → メソッドが確認できる
・リストのコピー(参照渡しと値渡し)
i = [1, 2, 3, 4, 5]
j = i
j[0] = 100
print('j = ', j)
print('i = ', i)
# j = [100, 2, 3, 4, 5]
# i = [100, 2, 3, 4, 5]
#参照渡しの例
#回避策
x = [1, 2, 3, 4, 5]
y = x.copy()
# y = x[:]
# 上記でも「y = x.copy()」と同様ですが、「y = x.copy()」が一般的
y[0] = 100
print('y = ', y)
print('x = ', x)
# y = [100, 2, 3, 4, 5]
# x = [1, 2, 3, 4, 5]
X = 20
Y = X
Y = 5
print(Y)
print(x)
#5
#20
#値渡しの例
#リストはコピーする際に参照渡しになるので、目的に応じて回避策を講じる必要あり
・リストの使い所
例えばタクシーの乗客をカウントするケース
対話型のshell
>>> seat = []
>>> min = 0
>>> max = 5
>>> min <= len(seat) < max
True
#乗れる場合は「True」を返す
>>> seat.append('p')
#apeendメソッドで乗客を増やす
>>> min <= len(seat) < max
True
>>> seat.append('p')
>>> min <= len(seat) < max
True
>>> seat.append('p')
>>> min <= len(seat) < max
True
>>> len(seat)
3
>>> seat.append('p')
>>> min <= len(seat) < max
True
>>> seat.append('p')
>>> min <= len(seat) < max
False
>>> len(seat)
5
>>>
>>> seat.pop(0)
'p'
#popメソッドで乗客を減らす
>>> min <= len(seat) < max
True
・タプル型
値を入れたら、読み込み際に使うことが多い
データを変更されたくない時に使用
>>> t = (1, 2, 3, 4, 1, 2)
>>> t
(1, 2, 3, 4, 1, 2)
>>> type(t)
<class 'tuple'>
>>> t[0] = 100
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
#値を変更しようとしても、不可
>>> t[0]
1
>>> t[-1]
2
>>> t[2:5]
(3, 4, 1)
>>> t[:]
(1, 2, 3, 4, 1, 2)
>>>
>>> t.index(2)
1
>>> t.index(2, 2)
5
>>> t.count(2)
2
>>> t = ([1, 2, 3], [4, 5, 6])
>>> t
([1, 2, 3], [4, 5, 6])
#タプルの中にリストを入れることは可能
#タプルの中のリストの値を変更することも可能
>>> t[0]
[1, 2, 3]
>>> t[0] = [1]
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
>>> t[0][0] = 100
>>> t[0]
[100, 2, 3]
>>>
>>> t= 1, 2, 3
>>> type(t)
<class 'tuple'>
>>> t
(1, 2, 3)
>>> t= 1,
>>> type(t)
<class 'tuple'>
>>> t
(1,)
>>> t= ()
>>> type(t)
<class 'tuple'>
>>> t= (1)
>>> type(t)
<class 'int'>
>>> t= ('test')
>>> type(t)
<class 'str'>
>>> t= ('test', )
>>> type(t)
<class 'tuple'>
#タプルは()がなくても作成可能。
#しかし、「,」がない場合タプル型にならないケースが多い
#タプル型で値の変更を行うことができないが、新しい変数を定義することで可能。
#またタプル同士の加算は可能
#しかし、タプルと数値や文字列の加算は不可能
>>> new_tuple = (1, 2, 3) + (4, 5, 6)
>>> new_tuple
(1, 2, 3, 4, 5, 6)
>>> new_tuple = (1) + (4, 5, 6)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: unsupported operand type(s) for +: 'int' and 'tuple'
>>>
>>> new_tuple = (1,) + (4, 5, 6)
>>> new_tuple
(1, 4, 5, 6)
>>>
・タプルのアンパッキングと数値の入れ替え
num_tuple = (10, 20)
print(num_tuple)
x, y = num_tuple
print(x, y)
x, y =10, 20
print(x, y)
min, max = 0, 100
print(min, max)
#a, b, c, d, e, f = 'Mike', '1', '1', '1', 'e', 'f'
#タプルは文字の宣言が多いことに対しては見にくくなってしまうため、多くなる場合は下記のように行う
a = 'Mike'
b = '1'
#タプルのアンパッキングを利用して、数値の入れ替えが簡単になる
i = 10
j = 20
#iとjの値を交換する際は?
#①
tmp = i
i= j
j = tmp
print(i, j)
#→ 20 10
a = 100
b = 200
#②
print(a, b)
#→ 100 200
a, b = b, a
print(a, b)
#→ 200 100
・タプルの使い所
#例)3つの選択肢から2つを選んだ際に選択したものを表示する場合
chose_from_two = ('A', 'B', 'C')
answer = []
answer.append('A')
answer.append('C')
print(chose_from_two)
print(answer)
#リスト型とタプル型を併用した問題であるが、
#answerをタプル型にはできない。
#なぜならタプル型には値の追加ができないため、リスト型を使用する
今日はここまでリスト型とタプル型が終わりました。
明日は辞書型を終え、セクション5の「制御フローとコード構造」に入っていきます。
まだまだ基礎中の基礎感がありますが、試験で問われるところを意識して対応していきます。
最初から読みたい方は下記からどうぞ
この記事が気に入ったらサポートをしてみませんか?