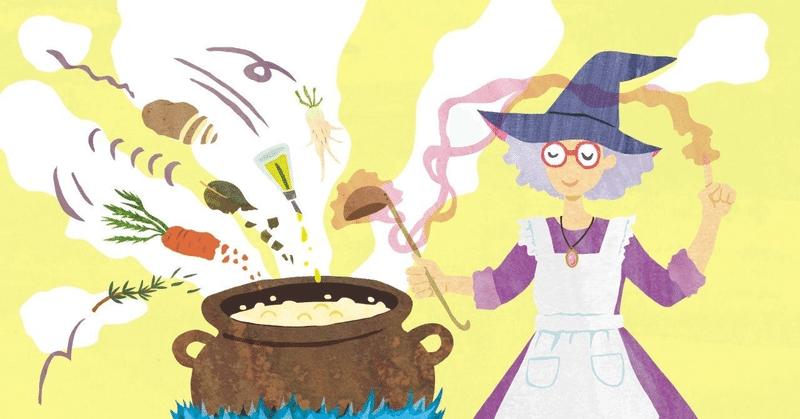
Tips
TensorFlow
tf.Dataset <- 解説(本家)
tf.TensorShape() <- Represents the shape of a Tensor.
A TensorShape represents a possibly-partial shape specification for a Tensor. It may be one of the following:
Fully-known shape: has a known number of dimensions and a known size for each dimension.
e.g. TensorShape([16, 256])
動的shape:動的shapeはshapeの明確な値を指定したくない場合に使用。
入力のshapeが動的に変動する可能性がある場合は明確に指定せずNoneとして記述することが求めらる。
Partially-known shape: has a known number of dimensions, and an unknown size for one or more dimension.
e.g. TensorShape([None, 256])
Unknown shape: has an unknown number of dimensions, and an unknown size in all dimensions.
e.g. TensorShape(None)
tf.ensure_shape <- Updates the shape of a tensor and checks at runtime that the shape holds.
tf.squeeze <- Removes dimensions of size 1 from the shape of a tensor.
tf.image.resize <- Resize images to size using the specified method.(検証)
tf.map_fn() <- Transforms elems by applying fn to each element unstacked on axis 0. (deprecated arguments)
tf.py_function <- Wraps a python function into a TensorFlow op that executes it eagerly. 一部分だけEager Modeで実行することができる機能。(参考:TF2.0応用編】TFの例の強いデータセット機能で汎用的なDataAugmentationを並列化しハイスピードで実現した件 )
tf.function <- Python の構文のサブセットを移植可能でハイパフォーマンスな TensorFlow のグラフに変換
tf.numpy_function <- MapDataset で numpy データ型を使う場合。(Tips)
TensorFlow の NumPy API <- TensorFlow により高速化された NumPy コードを実行し、TensorFlow のすべて API にもアクセスできる。
tf.experimental.numpy <- NumPy API on TensorFlow
Tensorflowでnumpyの動作
# If you are looking for numpy-related methods, please run the following:
from tensorflow.python.ops.numpy_ops import np_config
np_config.enable_numpy_behavior()
[TensorFlow 2] グラフモードでTensorの中身を確認する方法 <- tf.print など
ど
tf.random.normal() <- Outputs random values from a normal distribution.
tf.zeros_like() <- Creates a tensor with all elements set to zero.
Keras
正則化<- 最適化中にレイヤーパラメータあるいはレイヤーの出力に制約を課す
・keras.regularizers.l1(0.)
・keras.regularizers.l2(0.)
・keras.regularizers.l1_l2(l1=0.01, l2=0.01)
モデル
ゲート付き回帰型ユニット
GRUのアイデアは次の式で表される(実際の演算とは異なる)。
$${\displaystyle h_{t}=(1-z)\cdot h_{t-1}+z\cdot f(x_{t}+r\cdot h_{t-1})}$$
Python
set型: 集合演算(重複しない要素(同じ値ではない要素、ユニークな要素)のコレクションで、和集合、積集合、差集合などの集合演算を行う)
inplace=True : 変数の中身を変更。つけないと、変更せずに、欠損値を削除した新しいデータを作成
re.sub メソッド : 正規表現で文字列を置換
decimal --- 十進固定及び浮動小数点数の算術演算
Python ライブラリ
libsora
librosa is a python package for music and audio analysis. It provides the building blocks necessary to create music information retrieval systems.
STFT 事例
wavelet 事例
定Q変換(CQT: Constant-Q transform) 低周波数で良い分解能を示す。 (事例)
melspectrogram 周波数が人間の知覚に近いメル尺度(低周波数の音を良く知覚する)に変換されたSTFT(事例)
resampry
オーディオ信号向けの効率的なリサンプリング処理。
xfeat
: 特徴量エンジニアリングのライブラリ.By using cuDF and CuPy, xfeat can generate features 10 ~ 30 times faster than a naive pandas operation.(使用例1、使用例2)
lightbgm
: LightGBM is a gradient boosting framework that uses tree based learning algorithms
・FAQ
・Missing Value Handle
・[LightGBM] [Warning] Met negative value in categorical features, will convert it to NaN <- The column you’re trying to pass via categorical_feature likely contains very large values. Categorical features in LightGBM are limited by int32 range, so you cannot pass values that are greater than Int32.MaxValue (2147483647) as categorical features (see Microsoft/LightGBM#1359). You should convert them to integers ranging from zero to the number of categories first.
・LightGBMのパラメータチューニングまとめ
・GPU高速化 <- lightGBMはツリー系のなかでもあまりGPU処理の恩恵が得られないライブラリ。コア数の少ないCPUを利用している場合は、GPU利用の恩恵があるかもしれない。
optuna
timeout設定:Optuna で決められた時間内で最適化する場合
optuna.integration.lightgbm.LightGBMTuner : Hyperparameter tuner for LightGBM.(使用例1、使用例2、使用例3、リリースノート)
It optimizes the following 7 hyperparameters in a stepwise manner:
・"lambda_l1", "lambda_l2", "num_leaves", "feature_fraction", ・"bagging_fraction", "bagging_freq" and "min_child_samples".
Optuna の拡張機能。ハイパーパラメータ自動最適化。「重要なハイパーパラメータから順に最適なハイパーパラメータをチューニングする」
sklearn
・model_selection
KFold : 機械学習のモデル評価で行うクロスバリデーション
pandas
mapメソッド: pandas.Seriesのmap()は、引数に関数を渡すことでpandas.Seriesの各要素に関数を適用するメソッド。
applyメソッド:Invoke function on values of Series.
pandasのapplyに複数の引数を持つ関数を与える
Numpy
numpy.matmul() <- Matrix product of two arrays. (行列積の計算、挙動)
numpy.dot() <- Dot product of two arrays. (numpy.dotの挙動)
numpy.argsort() <- ソートされた値ではなくインデックスのndarrayを返す関数(本家)
random(),uniform(), randrange(), randint() <- 乱数(ランダムな浮動小数点数floatや整数int)を生成
numpy.apply_along_axis <- 指定した軸に沿って1次元配列を引数にとる関数を適用。
numpy.absolute() <- Calculate the absolute value element-wise.
numpy.corrcoef() <- Return Pearson product-moment correlation coefficients.
numpy.argsort() <- Returns the indices that would sort an array. 例1、例2NumPy配列ndarrayの結合
・numpy.concatenate()
・結合する配列ndarrayのリストを指定
・結合する軸(次元)を指定: 引数axis
・numpy.stack() <- 新たな軸(次元)に沿って結合
・numpy.block() <- 配置を指定して結合
・numpy.vstack() <- 縦に結合
・numpy.hstack() <- 横に結合
・numpy.dstack() <- 深さ方向に結合
Matplotlib
本家 事例
ヒストグラム
カラーマップ
ログスケール表示とグリッド表示
legend(凡例) の 位置を調整
実線、破線、破線&点線、点線 < linestyle= solid, dashed, dashdot, dotted
文字列から空白文字を削除
文字列のstrip系のメソッド、replaceメソッド、maketrans/translateメソッド、splitメソッドを使って文字列から空白文字を削除
# 文字列前後の空白文字を削除
s = ' sample string '
print(s, end='*\n') # sample string *
result = s.strip()
print(result, end='*\n') # sample string*
# 文字列先頭の空白文字を削除
s = ' sample string '
print(s, end='*\n') # sample string *
result = s.lstrip()
print(result, end='*\n') # sample string *
# 文字列末尾の空白文字を削除
s = ' sample string '
print(s, end='*\n') # sample string *
result = s.rstrip()
print(result, end='*\n') # sample string*
# 上記は全角の空白文字や改行文字も削除する
s = ' \tサンプル 文字列\n '
print(s, end='*\n')
# 出力結果
# サンプル 文字列
# *
result = s.strip()
print(result, end='*\n') # サンプル 文字列*
# replaceメソッドで文字列に含まれている空白文字を削除
s = '+ foo bar baz '
print(s, end='*\n') # + foo bar baz *
result = s.replace(' ', '')
print(result) # +foobarbaz
# 複数の空白文字をreplaceメソッドで削除
s = ' \tサンプル 文字列\n '
result = s.replace(' ', '').replace('\t', '').replace('\n', '')
print(result) # サンプル文字列
# 文字列のmaketrans/translateメソッドを使用する
d = { k: '' for k in [' ', ' ', '\t', '\n']}
tbl = str.maketrans(d)
s = ' \tサンプル 文字列\n '
result = s.translate(tbl)
print(result) # サンプル文字列
# 文字列に含まれている空白文字を削除
s = ' \tサンプル \t 文字列\n '
print(s, end='*\n')
# 出力結果:
# サンプル 文字列
# *
result = ''.join(s.split())
print(result) # サンプル文字列
# 文字列中の連続する空白文字を単独の空白文字にする
s = ' \tサンプル \t 文字列\n '
print(s, end='*\n')
# 出力結果:
# サンプル 文字列
# *
result = ' '.join(s.split())
print(result) # サンプル 文字列
進捗バー
tqdm:Instantly make your loops show a smart progress meter - just wrap any iterable with tqdm(iterable), and you're done!(事例)
統計関係
Note
表の埋め込み方 <- gist を利用して表を埋め込む
この記事が気に入ったらサポートをしてみませんか?