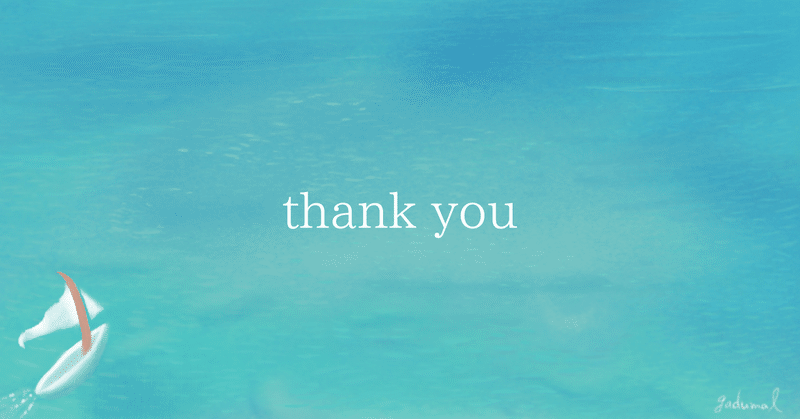
Photo by
kabakaja
AURAサンプル(お問い合わせ画面)
作成者:SUMKING@李
この投稿は私個人の練習メモです。 ご利用は自己責任でお願いいたします。
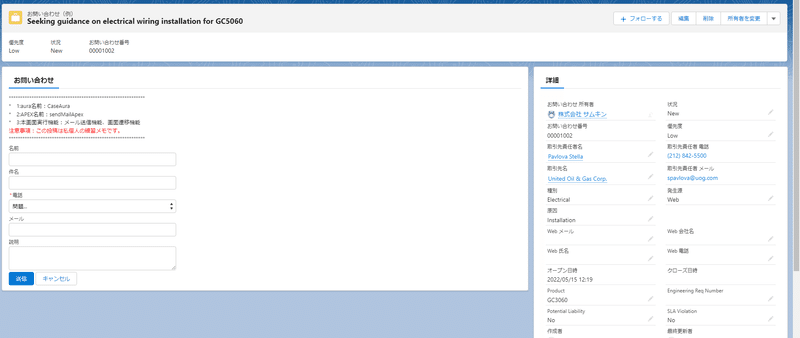
aura
<!--
@description : お問い合わせAURA画面
@author : SUMKING@李
@last modified on : 05-24-2022
-->
<aura:component implements="force:appHostable,flexipage:availableForAllPageTypes,flexipage:availableForRecordHome,force:hasRecordId"
access="global"
controller="sendMailApex">
<aura:attribute name="errorflg" type="Boolean" default="true" />
<aura:attribute name="errorMassage" type="String" />
<aura:attribute name="SuppliedName" type="String" />
<aura:attribute name="Subject" type="String" />
<aura:attribute name="SuppliedPhone" type="String" />
<aura:attribute name="SuppliedEmail" type="String" />
<aura:attribute name="Description" type="String" />
<aura:if isTrue="{!v.errorflg}">
<div class="row" style="width: 400px;" >
<lightning:input name="SuppliedName" label="名前" style="width: 400px;" aura:id="SuppliedNameId" value="{!v.SuppliedName}"/>
<lightning:input name="Subject" label="件名" style="width: 400px;" aura:id="SubjectId" value="{!v.Subject}"/>
<lightning:input name="SuppliedPhone" label="件名" style="width: 400px;" aura:id="SuppliedPhoneId" value="{!v.SuppliedPhone}"/>
<lightning:select name="SuppliedPhone" label="電話" required="true" aura:id="SuppliedPhoneId" value="{!v.SuppliedPhone}">
<option value="">問題...</option>
<option value="問題1">問題1</option>
<option value="問題2">問題2</option>
<option value="問題3">問題3</option>
</lightning:select>
<lightning:input name="SuppliedEmail" label="メール" style="width: 400px;" aura:id="SuppliedEmailId" value="{!v.SuppliedEmail}"/>
<lightning:textarea name="Description" label="説明" style="width: 400px;" aura:id="DescriptionId" value="{!v.Description}"/>
<lightning:button variant="brand" label="送信" title="Brand action" onclick="{! c.sendMail }" value=""/>
<lightning:button label="キャンセル" title="cancel action" onclick="{! c.handleClickB }" value=""/>
</div>
<aura:set attribute="else">
{!v.errorMassage}
</aura:set>
</aura:if>
</aura:component>
({
//メール送信メゾンド
sendMail : function(component, event, helper) {
//helperメール送信呼び出し
helper.sendMailHelper(component);
}
})
({
//メール送信メゾンド
sendMailHelper : function(component) {
//画面側に入力した内容を取得します(方法1:通过aura:id来取)
// var SuppliedName = component.find("SuppliedNameId").get("v.value");
// var Subject = component.find("SubjectId").get("v.value");
// var SuppliedPhone = component.find("SuppliedPhoneId").get("v.value");
// var SuppliedEmail = component.find("SuppliedEmailId").get("v.value");
// var Description = component.find("DescriptionId").get("v.value");
//画面側に入力した内容を取得します(方法2:通过前端变量来取,这种方法更常用)
var SuppliedNameV = component.get("v.SuppliedName");
var SubjectV = component.get("v.Subject");
var SuppliedPhoneV = component.get("v.SuppliedPhone");
var SuppliedEmailV = component.get("v.SuppliedEmail");
var DescriptionV = component.get("v.Description");
//logで取得した値を確認します
console.log("SuppliedName=="+SuppliedNameV);
console.log("Subject=="+SubjectV);
console.log("SuppliedPhone=="+SuppliedPhoneV);
console.log("SuppliedEmail=="+SuppliedEmailV);
console.log("Description=="+DescriptionV);
//sendMailApexのsendMailメゾンドを呼び出し
var action = component.get("c.sendMailMethod2");
//画面側取得した値はsendMailメゾンドのパラメータに設定します
action.setParams({
SuppliedName:SuppliedNameV,
Subject:SubjectV,
SuppliedPhone:SuppliedPhoneV,
SuppliedEmail:SuppliedEmailV,
Description:DescriptionV
});
action.setCallback(this, function(response){
var state = response.getState();
console.log("state=="+state);
//メッセージ表示フラグを設定します
component.set("v.errorflg", false);
if(state === "SUCCESS"){
//取得apex后台回调的值 true:メール送信成功 false:メール送信失敗
var errorReturnValue=response.getReturnValue();
if(errorReturnValue){
//成功メッセージは画面に設定します
component.set("v.errorMassage", "メール送信成功しました。");
}else{
//失敗メッセージは画面に設定します
component.set("v.errorMassage", "メール送信失敗しました。");
}
}else{
//失敗メッセージは画面に設定します
component.set("v.errorMassage", "メール送信失敗しました。");
}
});
$A.enqueueAction(action);
}
})
Apex
/***********************************************
* @description : メール送信APEX
* @author : sumking@李
* @last modified on : 05-24-2022
************************************************/
public with sharing class sendMailApex {
/**
* @description メール送信メゾンド *方法1:メールテンプレートの使用しない場合
* @author sumking@李
* @param SuppliedName :名前
* @param Subject :件名
* @param SuppliedPhone :電話
* @param SuppliedEmail :メール
* @param Description :説明
* @return errorflg : true:メール送信成功 false:メール送信失敗
**/
@AuraEnabled
public static boolean sendMailMethod(String SuppliedName,String Subject, String SuppliedPhone, String SuppliedEmail,String Description){
//logで引数確認します
System.debug('SuppliedName=='+SuppliedName);
System.debug('Subject=='+Subject);
System.debug('SuppliedPhone=='+SuppliedPhone);
System.debug('SuppliedEmail=='+SuppliedEmail);
System.debug('Description=='+Description);
boolean errorflg;
try {
Messaging.SingleEmailMessage mail = new Messaging.SingleEmailMessage();
List<String> mailList = new List<String>();
// 送信先のメールアドレスはListに追加 这里把邮箱写死了,这里一般不写死,使用方法1或者方法2
//mailList.add('xu951124@gmail.com');
//方法1:【表示ラベル】からメールを取得します。
// String mail = System.Label.mail;
//送信先のメールアドレスはListに追加
// mailList.add(mail);
// 送信先(String[])設定します。
// mail.setToAddresses(mailList);
//方法2:【組織のアドレス】からメールを取得します。
OrgWideEmailAddress org = [SELECT
Id,
Address,
DisplayName
FROM
OrgWideEmailAddress
WHERE
DisplayName = 'お問い合わせメール'
LIMIT 1
];
// 送信元になるようセット
mail.setOrgWideEmailAddressId(org.id);
// 送信先(String[])
List<String> AddressList=new List<String>();
AddressList.add(org.Address);
mail.setToAddresses(AddressList);
// 件名(String)
mail.setSubject(Subject);
// 本文(String)
mail.setPlainTextBody(Description);
// 返信先メールアドレス(String)
mail.setReplyTo(SuppliedEmail);
//メール送信します
List<Messaging.SendEmailResult> results = Messaging.sendEmail(new List<Messaging.Email> {mail}, false);
//メール送信成功した場合
if (results[0].isSuccess()) {
errorflg=false;
// お問い合わせ内容を登録します
Case caseIns = new Case();
caseIns.SuppliedName = SuppliedName;
caseIns.Subject = Subject;
caseIns.SuppliedPhone = SuppliedPhone;
caseIns.SuppliedEmail = SuppliedEmail;
caseIns.Description = Description;
insert caseIns;
} else {
//メール送信失敗場合
errorflg=true;
}
} catch (Exception e) {
errorflg=true;
}
return errorflg;
}
/**
* @description メール送信メゾンド *方法2:メールテンプレート使用の場合
* @author XXXXXXX@李
* @param SuppliedName :名前
* @param Subject :件名
* @param SuppliedPhone :電話
* @param SuppliedEmail :メール
* @param Description :説明
* @return errorflg : true:メール送信成功 false:メール送信失敗
**/
@AuraEnabled
public static boolean sendMailMethod2(String SuppliedName,String Subject, String SuppliedPhone, String SuppliedEmail,String Description){
boolean errorflg;
try {
Messaging.SingleEmailMessage mail = new Messaging.SingleEmailMessage();
// 「メール:組織のアドレス」に登録したメールアドレスを取得
ID orgWideEmailAddressId = [SELECT
Id,
Address
FROM
OrgWideEmailAddress
WHERE
DisplayName = 'お問い合わせメール'
LIMIT 1
].Id;
// 送信元設定
mail.setOrgWideEmailAddressId(orgWideEmailAddressId);
// 送信先設定(取引先責任者から取得する)
// Contact contactInfo = [SELECT Id, Name, Account.Name FROM Contact WHERE Id=:contactId];
// mail.setTargetObjectId(contactInfo.Id);
// メールの添付ファイルを設定
// ContentVersion cVer = [SELECT Title, FileExtension, FileType, VersionData FROM ContentVersion WHERE ContentDocumentId = :contentDocumentId AND IsLatest = true];
// List<Messaging.Emailfileattachment> fas = new List<Messaging.Emailfileattachment>();
// Messaging.Emailfileattachment fa = new Messaging.Emailfileattachment();
// fa.setFileName(cVer.Title + '.' + cVer.FileExtension);
// fa.setBody(cVer.VersionData);
// fas.add(fa);
// mail.setFileAttachments(fas);
// メールテンプレートからメール送信内容を設定する
EmailTemplate et = [SELECT
Id,
Subject,
Body,
HtmlValue
FROM EmailTemplate
WHERE name = 'お問い合わせ2'
];
et.Subject=et.Subject.replace('#Subject#', Subject);
et.HtmlValue=et.HtmlValue.replace('#SuppliedName#', SuppliedName);
et.HtmlValue=et.HtmlValue.replace('#SuppliedPhone#', SuppliedPhone);
et.HtmlValue=et.HtmlValue.replace('#SuppliedEmail#', SuppliedEmail);
et.HtmlValue=et.HtmlValue.replace('#Description#', Description);
mail.setSubject(et.Subject);
mail.setHtmlBody(et.HtmlValue);
//メール送信します
List<Messaging.SendEmailResult> results = Messaging.sendEmail(new List<Messaging.Email> {mail}, false);
//メール送信成功した場合
if (results[0].isSuccess()) {
errorflg=false;
// お問い合わせ内容を登録します
Case caseIns = new Case();
caseIns.SuppliedName = SuppliedName;
caseIns.Subject = Subject;
caseIns.SuppliedPhone = SuppliedPhone;
caseIns.SuppliedEmail = SuppliedEmail;
caseIns.Description = Description;
insert caseIns;
} else {
//メール送信失敗場合
errorflg=true;
}
} catch (Exception e) {
errorflg=true;
}
return errorflg;
}
}
株式会社SUMKING(サムキン)
〒113-0034東京都文京区湯島2丁目4-1 TOURYUお茶の水ビル 201
ホーム:https://sumking.co.jp/
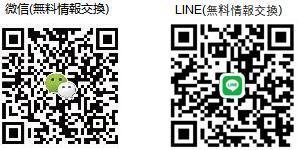
この記事が気に入ったらサポートをしてみませんか?