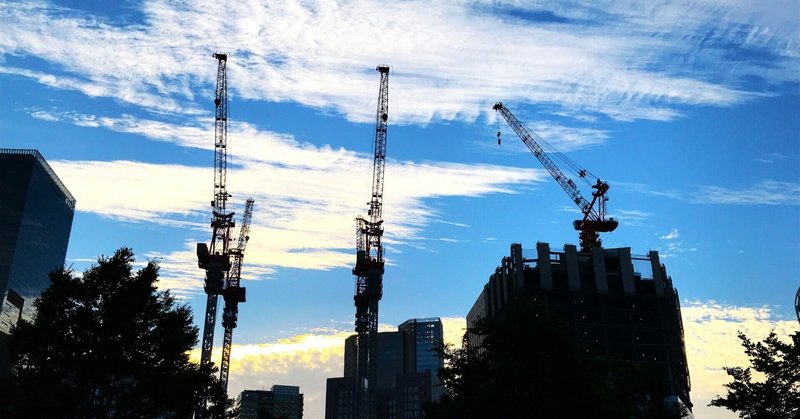
プログラミング学習の記録 #008(C)
今日は、宇宙物理学教室の後輩からリンゴをもらった。せっかくなので、ウサギリンゴにしてみた。1回生の冬に、当時の4回生の先輩につくってもらったウサギリンゴの方が上手だった。あれから3年が経って、僕ももうすぐ卒業かと思うと、少し寂しい気持ちになった。
前回の復習
両替計算のコード
前回の学習のときに、教科書を参考にして、EURとUSD、JPYの両替を行うプログラムコードを書いたが、もっと短く書けると思ったので、工夫して書き直してみた。
#include <stdio.h>
#include <math.h>
int main()
{
double r_euro, r_yen ;
double e, d, y ;
double price ;
char unit ;
r_euro = 0.9467 ; // euro/dollar
r_yen = 136.0960 ; // yen/dollar
printf("EUR:E, USD:D, JPY:Y\n");
printf("Input the money unit (E, D or Y): ");
scanf("%c", &unit);
if(unit != 'E' && unit != 'D' && unit != 'Y')
{
printf("Error in unit.\n");
}else
{
printf("Input the price: ");
scanf("%lf", &price);
switch(unit)
{
case 'D':
d = price ;
e = d * r_euro ;
y = d * r_yen ;
break;
case 'E':
e = price ;
d = e / r_euro ;
y = d * r_yen ;
break;
case 'Y':
y = price ;
d = y / r_yen ;
e = d * r_euro ;
break;
}
printf("%6.3f EUR =%6.3f USD =%6.3f JPY.\n", e,d,y );
}
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
結局、短くはならなかったが、一部の修正はできた。単位エラーがあるにもかかわらず、金額入力を求めていたが、単位エラーが判明した時点でエラーだという旨を表示し、プログラムが終了されるようになった。
練習問題 5.6
血液型性格診断プログラムを作成してみよう。男女の性別と血液型(0,A,B,AB)を入力すると、性格に関する診断結果が出力されるような簡単なものでよい。性別や血液型は数で指定するようにしてもよい(例えば0型は1、A型は2など)。性格に関する診断結果は各自本を参考にするなど工夫して調べて作ってみよ。
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
int main()
{
char q;
int type, gender ;
printf("I can do blood type fortune telling.\n");
// I ask your gender.
// male: 1, female: 2, others: 3.
printf("Are you male? y/n: ");
scanf("%s", &q);
if(q != 'y' && q != 'n')
{
printf("Error in your answer.\n");
exit(1);
}else if( q == 'y')
{
gender = 1 ;
}else
{
printf("Are you female? y/n: ");
scanf("%s", &q);
if(q != 'y' && q != 'n')
{
printf("Error in your answer.\n");
exit(1);
}else if( q == 'y')
{
gender = 2 ;
}else
{
gender = 3 ;
}
}
// I ask whether you know your blood type.
printf("Do you know your blood type? y/n: ");
scanf("%s", &q);
if(q != 'y' && q != 'n')
{
printf("Error in your answer.\n");
exit(1);
}else if( q == 'y')
{
printf("OK.\n") ;
}else
{
printf("OK. Please imagine your blood type.\n");
}
// I ask your blood type.
// A: 1, B: 2, AB: 3, O: 4.
printf("Is your blood type AB? y/n: ");
scanf("%s", &q);
if(q != 'y' && q != 'n')
{
printf("Error in your answer.\n");
exit(1);
}else if( q == 'y')
{
type = 3 ;
}else
{
printf("What is your blood type? A/B/O: ");
scanf("%s", &q);
switch(q)
{
case 'A':
type = 1;
break;
case 'B':
type = 2;
break;
case 'O':
type = 4;
break;
default:
printf("Error in your answer.\n");
exit(1);
break;
}
}
// I tell you results.
printf("I tell you results.\n");
if(gender == 1) //Male
{
switch(type)
{
case 1: //Type A
printf("Type A men are characterized by their tendency to \"walk across a bridge after tapping it.\"\n");
printf("They tend to plan things out carefully and take action cautiously. \n");
printf("They have a strict and critical view of people and society, and are also strict with themselves.\n");
printf("However, they understand the position they are in and take responsibility for their actions.\n");
printf("They also make an effort to follow rules and customs properly. \n");
printf("By sticking to these principles, they become strict with themselves, and without realizing it, they may demand the same of others and impose their strict principles on them.\n");
break;
case 2: //Type B
printf("Type B men are easygoing and have bright personalities.\n");
printf("They easily become influenced by others and act according to their mood, and once they make a decision, they charge straight towards their goal.\n");
printf("They often appear very reliable to others and become a leader-like presence.\n");
printf("However, they believe that only they are right and act without considering objectivity.\n");
printf("They tend to be selfish and can cause others to have a negative impression of them, which can lead to them becoming isolated.\n");
printf("However, they are very skilled at switching their moods and make various plans to convince themselves, and in the next moment they return to their usual selves.\n");
break;
case 3: //Type AB
printf("Type AB men are efficient and capable of handling things skillfully without waste in every aspect.\n");
printf("They also have a rich sensibility and have a sentimental and poetic side, making them quite idealistic.\n");
printf("While they are efficient at their work, they also seem to be able to think about unrealistic things.\n");
printf("Because their expressions can sometimes be different, they may be perceived as having a split personality or be treated as oddballs.\n");
break;
default: //Type O
printf("Type O men have clear goals and always strive to move towards them.\n");
printf("They are able to overcome difficulties with their own mental strength without relying on others.\n");
printf("They may lack planning skills, but they do not pay much attention to small details.\n");
printf("They sometimes take care of things on the spot while moving towards their goals.\n");
printf("They have confidence in their abilities, and may unconsciously look down on others.\n");
printf("Therefore, they may sometimes be seen as isolated by those around them.\n");
break;
}
}else if(gender == 2) //Female
{
switch(type)
{
case 1: //Type A
printf("Type A women are often thought of as having a pure and upright personality, and are often modest and reserved.\n");
printf("They are also single-minded and introspective, but on the other hand, they have a strong-willed and competitive side.\n");
printf("They always pay attention to those around them and are able to show meticulous consideration for others, and are always seen as a leader in any situation.\n");
break;
case 2: //Type B
printf("Type B women are always independent and go about things at their own pace.\n");
printf("They are also moody, so they act according to their mood at the time, and to others it may seem that their words and actions lack consistency.\n");
printf("In reality, Type B women want to help others who are in trouble with all their heart and support them like they would their own selves, to the point where they cry together.\n");
printf("However, their emotions change quickly and do not last.\n");
printf("When their emotions change, they approach others with a cold attitude, so they may be seen as only pretending to be cold.\n");
printf("They are also prone to misunderstandings with others of the same sex, who may question whether they really care and whether they have empathy for others.\n");
break;
case 3: //Type AB
printf("Type AB women are interested in a wide range of hobbies and have a wealth of knowledge.\n");
printf("Their pure and innocent atmosphere is a mystic charm to men.\n");
printf("They are seen as cute younger sisters by other women, and are loved by those around them.\n");
printf("They are good at mediating between people and arbitrating fights, and have a mediator-like quality.\n");
printf("On the other hand, they can be introspective and timid, and it may take time for them to show their true selves.\n");
break;
default: //Type O
printf("Type O women are pure and seek dreams and ideals.\n");
printf("They have a compassionate personality and show love for living things such as flowers and animals, and prefer a life with style.\n");
printf("They are also rich in sensibility and easily moved or impressed.\n");
printf("They are proactive and do not pay much attention to appearance or form, and are straightforward with their feelings and can act single-mindedly.\n");
break;
}
}else //Others
{
switch(type)
{
case 1: //Type A
printf("Type A people are weak-willed and shy.\n");
printf("They may not be able to clearly express their feelings when they feel that something is troublesome.\n");
printf("In such a situation, their attitude may be to reluctantly act with a sigh.\n");
printf("When the other person becomes angry at this indecisive attitude, they may finally express their feelings in anger.\n");
printf("However, it takes a lot for Type A people to consider something troublesome, so when they start to show their attitude, it is a sign that they have a lot of stress.\n");
printf("If their dissatisfaction explodes, they may give up on everything.\n");
break;
case 2: //Type B
printf("Type B people are active and kind.\n");
printf("Even if they feel that something is troublesome, they will gladly take on requests.\n");
printf("However, they are very easily bored and lack caution, so if you ask them to do a delicate job or request something that takes a long time, they may give up in the middle.\n");
printf("Their attitude when they are bothered is very casual and they should clearly refuse.\n");
printf("However, it is awkward for anyone to refuse.\n");
printf("To avoid unpleasant feelings, you should only make requests that are suitable for Type B personalities.\n");
break;
case 3: //Type AB
printf("Type AB people are prone to moodiness and complaints.\n");
printf("They are short-tempered but unable to express their feelings honestly.\n");
printf("As a result, even if they feel that something is troublesome, they may just be irritable or sulky on their own without letting the other person know.\n");
printf("Furthermore, even though Type AB people are strong in requesting things, they dislike being asked for favors, so it may be best to leave them alone unless it is something very important.\n");
printf("In fact, they may have more friendships with people who listen to their requests rather than people who ask for favors.\n");
break;
default: //Type O
printf("Type O people are self-centered and do not like to be told what to do.\n");
printf("If they are asked in a way that bothers them even slightly, they will quickly become annoyed.\n");
printf("Their attitude at that time is very easy to understand and they will clearly say no in words.\n");
printf("Even if they are pushed to accept, they are quite stubborn, so they will not listen.\n");
printf("However, they are weak if they are flattered, so if you can skillfully compliment them with flattery and praise, they will probably take it on even if they think it is a bit of a bother.\n");
break;
}
}
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
このサイトとこのサイトに記載されていた診断内容をChatGPTを用いて英語に翻訳したものを診断結果とした。コードを書いているとき、「scanf("%c", &q);」を複数回使用して回答を入力させようとしたが、うまく実行されなかった。このサイトによると、このような「scanf("%c", &q);」の連続使用はよくないということであったので、「scanf("%s", &q);」としたところ、うまく実行されるようになった。また、「exit(1);」については、このサイトを参考にした。
for
繰り返し(ループ)を行うための代表的な方法として、forとwhileを用いた方法がある。まず、forから学習した。forによる繰り返し文では、ループ回数を記録するためのカウンタ変数を用意することが多い。教科書を参考にして、カウンタ変数iとして、1から10までの整数の和
$$
\mathrm{sum}
= \sum_{i=1}^{10}i
$$
を求めるコードを書いた。
#include <stdio.h>
int main()
{
int i, sum;
sum = 0 ;
for(i = 1; i <= 10; i = i + 1 )
{
sum = sum + i ;
printf("i = %2d, sum = %2d\n", i,sum);
}
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
これをTerminalで実行すると、以下のように表示された。
% ./a.out
i = 1, sum = 1
i = 2, sum = 3
i = 3, sum = 6
i = 4, sum = 10
i = 5, sum = 15
i = 6, sum = 21
i = 7, sum = 28
i = 8, sum = 36
i = 9, sum = 45
i = 10, sum = 55
HAPPY SMILE (^_^)v
また、教科書を参考にして、Leibnizの公式
$$
\dfrac{\pi}{4}
= \sum_{n=0}^{\infty} \dfrac{(-1)^{n}}{2n+1}
$$
によって円周率$${\pi}$$の値を近似的に求めるプログラムコードを書いた。
#include <stdio.h>
#include <math.h>
int main()
{
double y, yp;
int i, max, count;
printf("I can calculate π approximately.\n");
printf("I will use Leibniz formula for π.\n");
printf("Input the number of terms (>=10): ");
scanf("%d", &max);
y = 0 ;
yp = 0 ;
count = max / 10;
for(i = 0; i <= max; i = i+1 )
{
y = y + pow(-1, i) / (2 * i + 1) ;
yp = y * 4 ;
if(i == floor(i / count) * count) //Display calculation results about 10 times.
{
printf("π ≈ %13.10f ( i = %7d)\n", yp,i );
}
}
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
これをTerminalで実行すると、以下のように表示された。項数は、200,000とした。
% ./a.out
I can calculate π approximately.
I will use Leibniz formula for π.
Input the number of terms (>=10): 200000
π ≈ 4.0000000000 ( i = 0)
π ≈ 3.1416426511 ( i = 20000)
π ≈ 3.1416176530 ( i = 40000)
π ≈ 3.1416093200 ( i = 60000)
π ≈ 3.1416051534 ( i = 80000)
π ≈ 3.1416026535 ( i = 100000)
π ≈ 3.1416009869 ( i = 120000)
π ≈ 3.1415997964 ( i = 140000)
π ≈ 3.1415989036 ( i = 160000)
π ≈ 3.1415982091 ( i = 180000)
π ≈ 3.1415976536 ( i = 200000)
HAPPY SMILE (^_^)v
while
次に、whileをを学習した。whileは、forと異なって、カウンタ変数を用いた回数の指定をしなくてよいので、希望する計算精度を実現するために必要な試行回数がわからないときに便利らしい。もちろん、たいていの計算は、forとwhileのどちらを用いても可能だが、目的に合わせて使い分けるとよいということであった。教科書を参考にして、2つの整数の最小公倍数を求めるプログラムコードを書いた。
#include <stdio.h>
int main()
{
int num1, num2, i ;
printf("Input integer 1: ");
scanf("%d", &num1);
printf("Input integer 2: ");
scanf("%d", &num2);
i = num1 ;
while(i % num2 != 0)
{
i = i + num1 ;
}
printf("LCM(%d,%d) = %d\n",num1,num2,i);
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
桁数が大きくなると、正しく計算されなかった。num1とnum2がともに5桁以上になると怪しくなってくるように思う。
インクリメント演算子・デクリメント演算子
うまく用いると便利らしいが、用いないようにしている。こういったプログラミング独特の表現がプログラミングに対する苦手意識を加速させる一因となっているからである。一応、記録だけしておく。
インクリメント演算子 ++ (例:x++ → x = x + 1 )
デクリメント演算子 -- (例:x-- → x = x - 1 )
-----
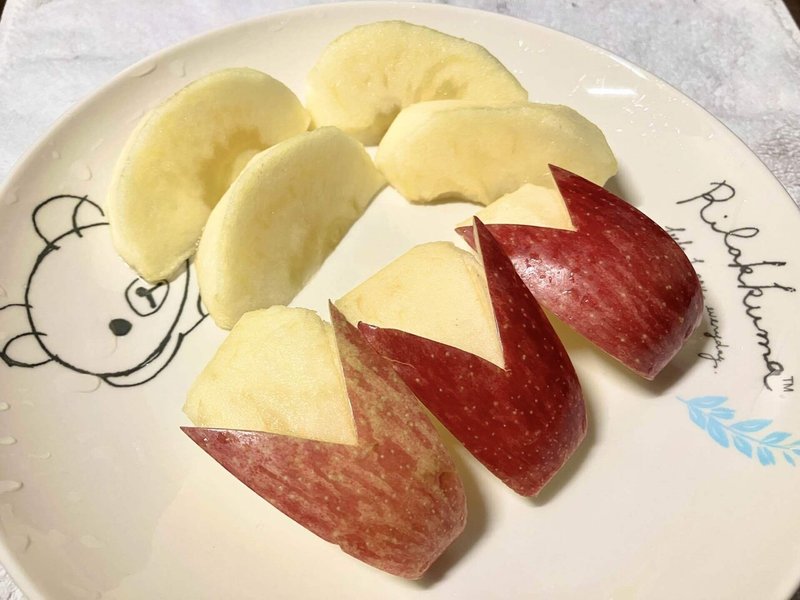
動け!タイムライン
動物園か水族館にいきたいですね。