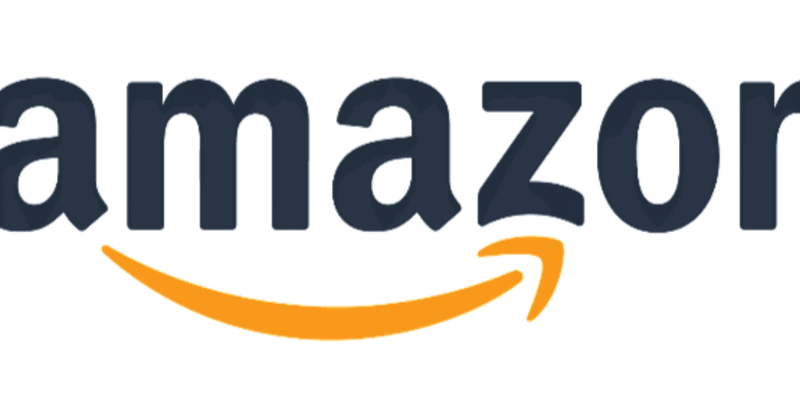
Pythonを使ってAmazonで品切れ商品が入荷されたときに自動注文をする
まず、Amazon などの外部 Web サイトとやり取りする自動化されたプログラムを作成するには、Web スクレイピングに関する高度な知識が必要であり、Amazon の利用規約に違反する可能性があることに注意してください。そのため、Amazon のポリシーを確認し、必要に応じて専門家の助けを求めることをお勧めします。
そうは言っても、Amazon に在庫がない製品が利用可能になったときに自動的に注文するプログラムの基本的なロジックは次のとおりです。
1.
Requests、BeautifulSoup、Selenium などの必要なライブラリをインポートすることから始めます。
2.
購入したい商品の在庫状況を確認する関数を定義します。この関数は、Web スクレイピング技術を使用して、Amazon の Web サイトでの製品の在庫状況に関する情報を抽出する必要があります。
3.
製品が利用できない場合は、時間モジュールを使用して一定期間 (たとえば 6 時間) のタイマーを設定してから、再度確認してください。
4.
製品が入手可能になったら、製品の URL を抽出し、Selenium 自動化を使用してショッピング カートに追加します。
5.
別の関数を使用して、チェックアウト プロセスを完了します。この機能は、Amazon アカウントへのログイン、配送および支払い情報の入力、購入の確認のプロセスを自動化する必要があります。
開始に役立つサンプル Python コードを次に示します。
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
import time
# Define the product URL
product_url = "https://www.amazon.com/dp/B07VLK4WZN"
# Define a function to check the product availability
def check_availability():
# Send a GET request to the product URL
response = requests.get(product_url)
# Parse the HTML content using BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
# Find the product's availability status
availability = soup.find('div', {'id': 'availability'}).get_text().strip()
if availability == 'In Stock.':
return True
else:
return False
# Define a function to complete the checkout process
def checkout():
# Start a new Chrome browser instance
driver = webdriver.Chrome()
# Navigate to the Amazon sign-in page
driver.get('https://www.amazon.com/gp/sign-in.html')
# Automate the sign-in process using your email and password
# ...
# Add the product to your cart using the product URL
driver.get(product_url)
driver.find_element_by_id('add-to-cart-button').click()
# Navigate to your cart and start the checkout process
driver.get('https://www.amazon.com/gp/cart/view.html')
driver.find_element_by_name('proceedToCheckout').click()
# Enter your shipping and payment information and complete the purchase
# ...
# Loop until the product becomes available
while not check_availability():
print("Product not available. Checking again in 6 hours...")
time.sleep(21600) # Wait for 6 hours
# Once the product becomes available, complete the checkout process
checkout()
繰り返しますが、Amazon の Web サイトとの自動対話はポリシーに違反する可能性があることに注意してください。このコードはデモンストレーションのみを目的としています。ご自身の責任で使用してください。
この記事が気に入ったらサポートをしてみませんか?