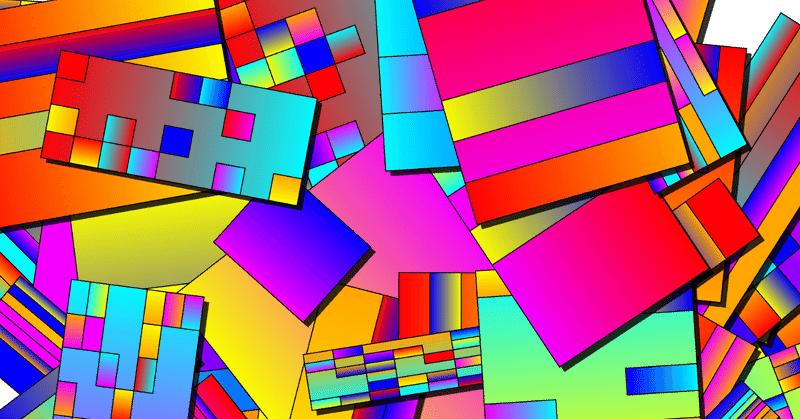
ProcessingでGenerative art #88
Code
int count = 100;
Tile[] tiles = new Tile[count];
int actRandomSeed = 0;
void setup() {
size(800, 800, P2D);
pixelDensity(2);
colorMode(HSB, 360, 100, 100, 100);
newTile();
}
void draw() {
randomSeed(actRandomSeed);
background(360);
for (int i = 0; i < count; i ++) {
tiles[i].display();
}
}
void newTile() {
for (int i = 0; i < count; i ++) {
tiles [i] = new Tile();
}
}
void mousePressed() {
newTile();
actRandomSeed = (int)random(1000000);
}
void keyPressed() {
if (key == 's')saveFrame("####.png");
}
//------------------------------------------------------------------------------------------------
class Tile {
float x, y, w, h;
float angle;
int countW, countH;
color[] col = {#FF0004, #0300FC, #F8FC00, #03F9FF, #F200FF, #FFA600};
int colNum;
float randomDirection;
Tile() {
x = random(width);
y = random(height);
w = random(50, 350);
h = random(50, 350);
angle = random(TWO_PI);
countW = int(random(1, 10));
countH = int(random(1, 10));
colNum = (int)random(6);
randomDirection = random(1);
}
void display() {
rectMode(CENTER);
pushMatrix();
translate(x, y);
translate(5, 5);
rotate(angle);
noStroke();
fill(0, 80);
rect(0, 0, w, h);
popMatrix();
pushMatrix();
translate(x, y);
rotate(angle);
fill(randomCol());
gradationRect(0, 0, w, h);
rects();
popMatrix();
}
void rects() {
rectMode(CORNER);
for (float j = - (h/2); j < h/2-1; j += h / countH) {
for (float i = - w/2; i < w/2-1; i += w / countW) {
noFill();
colNum = (int)random(6);
rect(i, j, w/countW, h/countH);
if (random(1) > 0.5) {
fill(randomCol());
gradationRect(i + w/countW/2, j + h/countH/2, w/countW, h/countH);
}
}
}
}
void gradationRect(float x_, float y_, float w_, float h_) {
float cw = w_/2;
float ch = h_/2;
colNum = (int)random(6);
pushStyle();
rectMode(CENTER);
stroke(0);
if (random(1) > 3.0/4.0) {
beginShape();
vertex(x_-cw, y_-ch);
vertex(x_+cw, y_ -ch);
fill(randomCol());
vertex(x_+cw, y_+ch);
vertex(x_-cw, y_+ch);
endShape(CLOSE);
} else if (random(1) > 2.0/4.0) {
beginShape();
vertex(x_-cw, y_+ch);
vertex(x_-cw, y_-ch);
fill(randomCol());
vertex(x_+cw, y_-ch);
vertex(x_+cw, y_+ch);
endShape(CLOSE);
} else if (random(1) > 1.0/4.0) {
beginShape();
vertex(x_+cw, y_-ch);
vertex(x_+cw, y_+ch);
fill(randomCol());
vertex(x_-cw, y_+ch);
vertex(x_-cw, y_-ch);
endShape(CLOSE);
} else {
beginShape();
vertex(x_+cw, y_+ch);
vertex(x_-cw, y_+ch);
fill(randomCol());
vertex(x_-cw, y_-ch);
vertex(x_+cw, y_-ch);
endShape(CLOSE);
}
popStyle();
}
color randomCol() {
return col[colNum];
}
}
Happy coding!!
応援してくださる方!いつでもサポート受け付けてます!