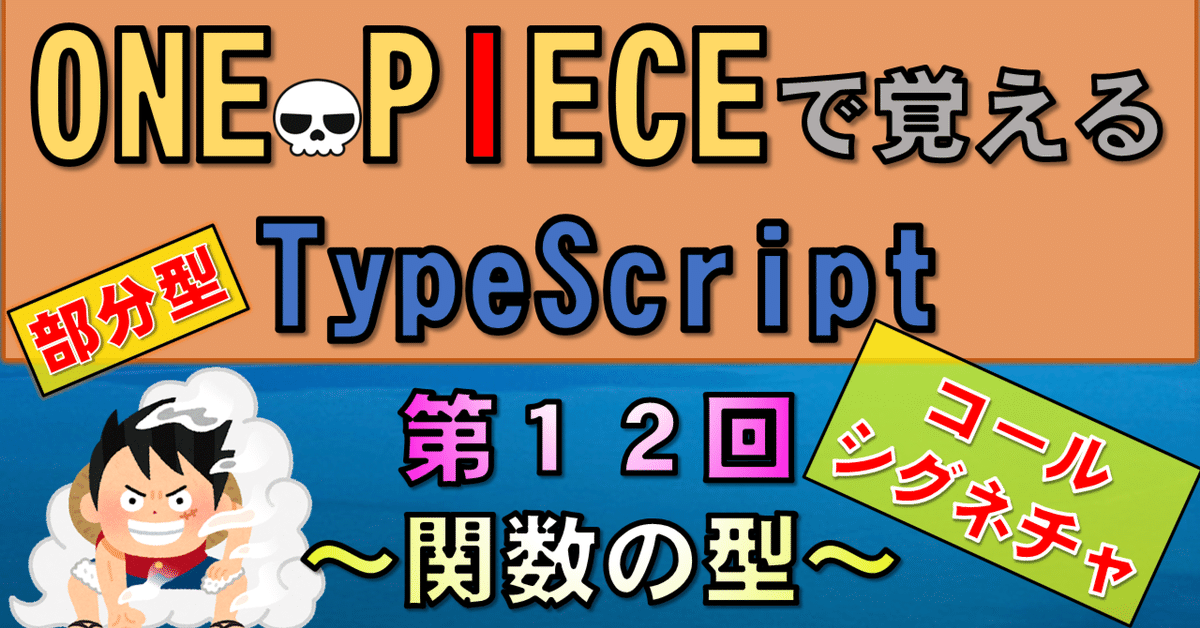
【ワンピースで覚えるTypeScript】第12回 関数の型(JavaScript学習者向け)
今回はTypeScriptの関数の型について説明します。
アロー関数式の記法に似ています。
部分型との関係も含めて理解していきましょう!
type S = (material: string) => string;
const createDevilFruits: S = (str: string): string => str.repeat(2)+ "の実";
console.log(createDevilFruits("ゴム"));
//引数名によって、ドキュメント化を支援
console.log(createDevilFruits("ヒト"));
//コールシグネチャ
type AbilityPerson = {
age?: number;
(str: string): void;
}
//string型を受け取る関数
const luffy: AbilityPerson = (str: string) => {
console.log(`${str}の能力者`);
}
luffy.age = 19;
console.log(luffy.age);
luffy("ゴムゴムの実");
//部分型(返り値)
type Character = {
name: string;
}
type WantedCharacter = {
name: string;
bounty: number;
}
//WantedCharacterはCharacterの部分型
//(bounty: number) => WantedCharacter は
//(bounty: number) => Character の部分型
//オブジェクトを返す関数chopper //返り値
const chopper = (bounty: number): WantedCharacter => ({
name: "チョッパー",
bounty,
});
console.log(chopper);
console.log(chopper(1000));
//「(bounty: number) => Character型」の変数characterに
//「(bounty: number) => WantedCharacter型」のchopperを代入
const character: (bounty: number) => Character = chopper;
console.log(character(1000));
//部分型(引数)
type Character1 = {
name: string;
}
type WantedCharacter1 = {
name: string;
bounty: number;
}
//WantedCharacter1はCharacter1の部分型
const getName = (obj: Character1) => {
console.log(obj.name);
}
//(obj: WantedCharacter1) => void は
//(obj: Character1) => void の部分型
const character1: (obj: WantedCharacter1) => void = getName;
character1({
name: "チョッパー", bounty: 1000
});
//bountyプロパティを持っているが、本来はCharacter1型なので影響はない
//部分型(引数の数の違い)
type One = (bounty: number) => number;
type Two = (bounty1: number, bounty2: number) => number;
const doubleBounty: One = bounty => bounty * 2;
const addBounty: Two = (bounty1, bounty2) => bounty1 + bounty2;
const calcuBounty: Two = doubleBounty;
console.log(calcuBounty(1000, 50));
//Two型を使用しているので、2つの引数を渡している
//しかし、代入されているのはOne型の関数
//=> 引数は1つしか受け取らない
この記事が気に入ったらサポートをしてみませんか?