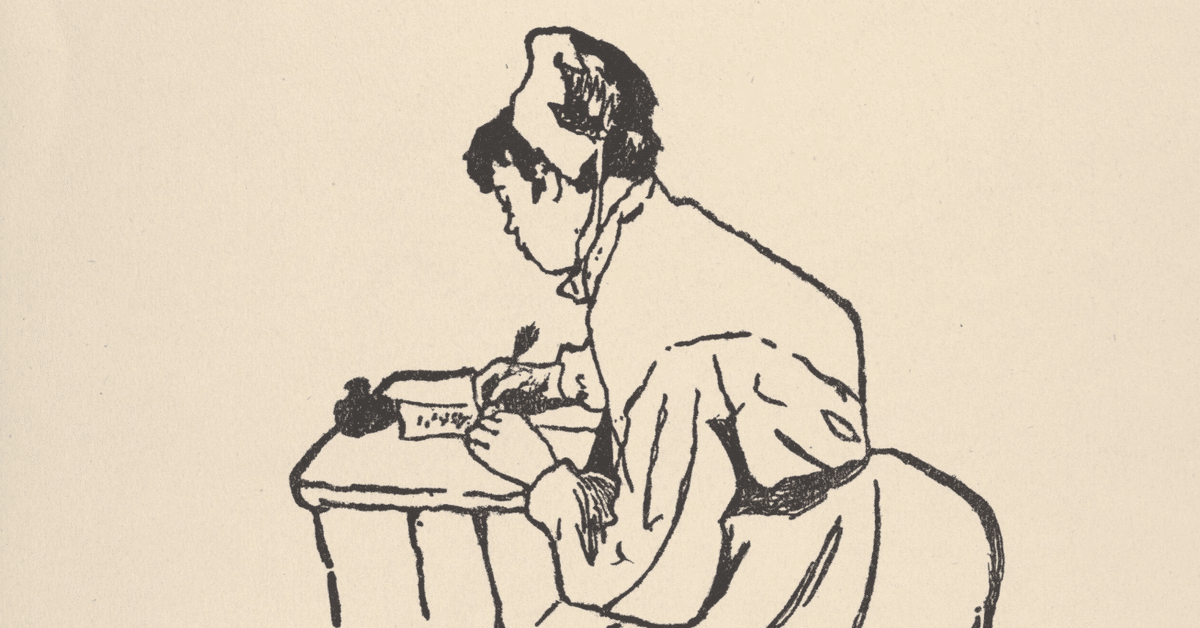
Photo by
アンリ・ド・トゥールーズ=ロートレック / メトロポリタン美術館
note 記事のバックアップ用スクリプト
下書きを含めて note 記事をバックアップする python スクリプト。記事情報の json をだいたいそのまま Gmail で自分宛てに送る。条件によっては不具合ありそうなのでご利用の際は自己責任で。
import requests
import os
import json
import datetime
from io import BytesIO
import smtplib
from email.mime.text import MIMEText
from email.utils import formatdate
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
note_login = NOTE_LOGIN
note_password = NOTE_PASSWORD
gmail_address = GMAIL_ADDRESS
gmail_password = GMAIL_PASSWORD
login_url = "https://note.com/api/v1/sessions/sign_in"
contents_api_url = "https://note.com/api/v2/note_list/contents"
def sendmail(json_text, count):
subject = 'Note Backup ' + datetime.datetime.now().strftime('%Y/%m/%d')
body_text = str(count) + " notes"
msg = MIMEMultipart()
msg['Subject'] = subject
msg['From'] = gmail_address
msg['To'] = gmail_address
msg['Date'] = formatdate()
msg.attach(MIMEText(body_text, "plain", "utf-8"))
with BytesIO() as bs:
bs.write(json_text.encode(encoding='utf-8'))
bs.seek(0)
mb = MIMEApplication(bs.read())
mb.add_header("Content-Disposition", "attachment", filename="notes.json")
msg.attach(mb)
smtpobj = smtplib.SMTP('smtp.gmail.com', 587)
smtpobj.starttls()
smtpobj.login(gmail_address, gmail_password)
smtpobj.sendmail(gmail_address, gmail_address, msg.as_string())
smtpobj.quit()
def get_contents():
payload = {
"login": note_login,
"password": note_password,
"redirect_path": "https://note.com/"
}
session = requests.Session()
response = session.post(login_url, params=payload)
response = session.get(contents_api_url)
notes = []
is_last_page = False
page = 1
while not is_last_page:
response = session.get(contents_api_url + "?page=" + str(page))
json_contents = json.loads(response.text)
new_results = json_contents["data"]["notes"]
is_last_page = json_contents["data"]["isLastPage"]
notes.extend(new_results)
page += 1
return json.dumps(notes, ensure_ascii=False), len(notes)
contents = get_contents()
sendmail(contents[0], contents[1])
※ GMAIL_PASSWORD は通常のパスワードではなくアプリパスワード。
この記事が気に入ったらサポートをしてみませんか?