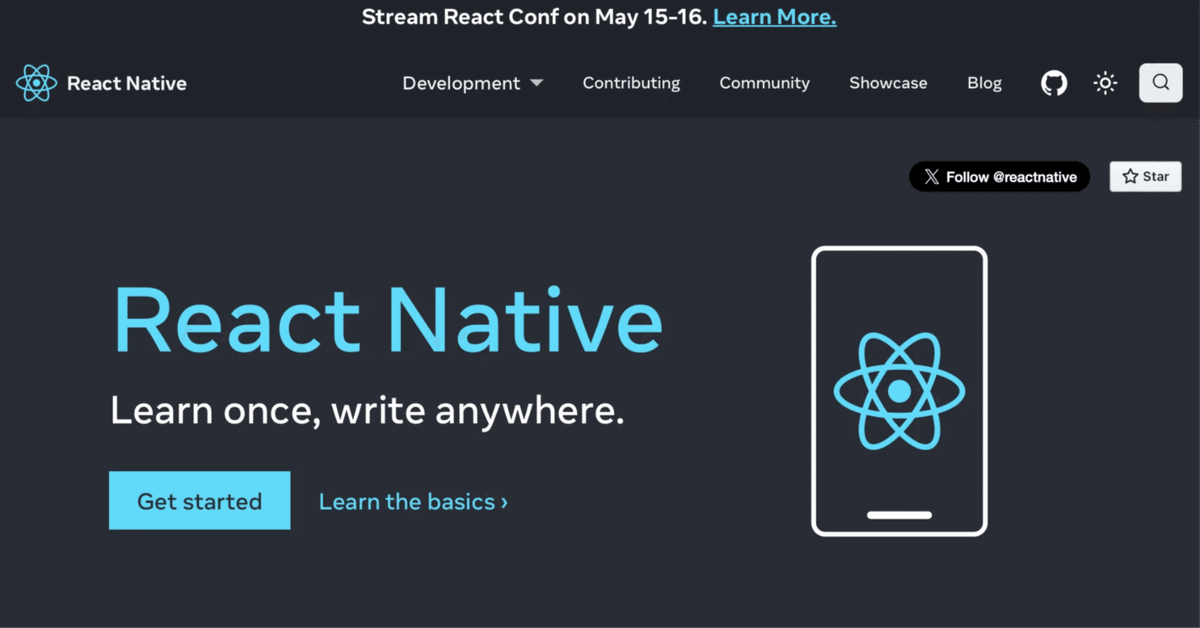
React Native の expo-av の使い方
「React Native」の 「expo-av」の使い方をまとめました。
・Expo SDK 51
前回
1. expo-av
「expo-av」は、「Expo」を利用した「React Native」アプリでオーディオおよびビデオの再生をサポートするライブラリです。「Expo」は「React Native」アプリの開発を簡単にするツールキットになります。
・オーディオの再生・録音
・ビデオの再生
・再生コントロール (再生、一時停止、停止、シークなど)
2. オーディオの再生
オーディオの再生の手順は、次のとおりです。
2-1. React Nativeプロジェクトの作成
(1) React Nativeプロジェクトの作成。
npx react-native init my_app
cd my_app
(2) パッケージのインストール。
npx install-expo-modules
npm install expo-av
2-2. アセットの配置
(1) プロジェクト下にassetsフォルダを作成し、オーディオ(sample.mp3)を配置。
・my_app
・assets
・sample.mp3
2-3. iOSのセットアップ
(1) podのインストール。
cd ios
pod install
cd ..
(2) Xcodeで署名し、iPhoneにインストールできることを確認。
xcworkspaceを開いた時に以下のエラーがでた場合、「Build Settings → Swift Compiler - Language」でバージョン「Swift 4.2」を指定することで解決しました。
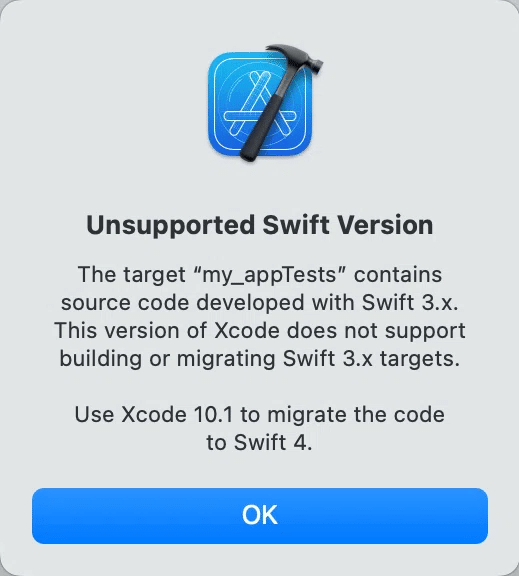
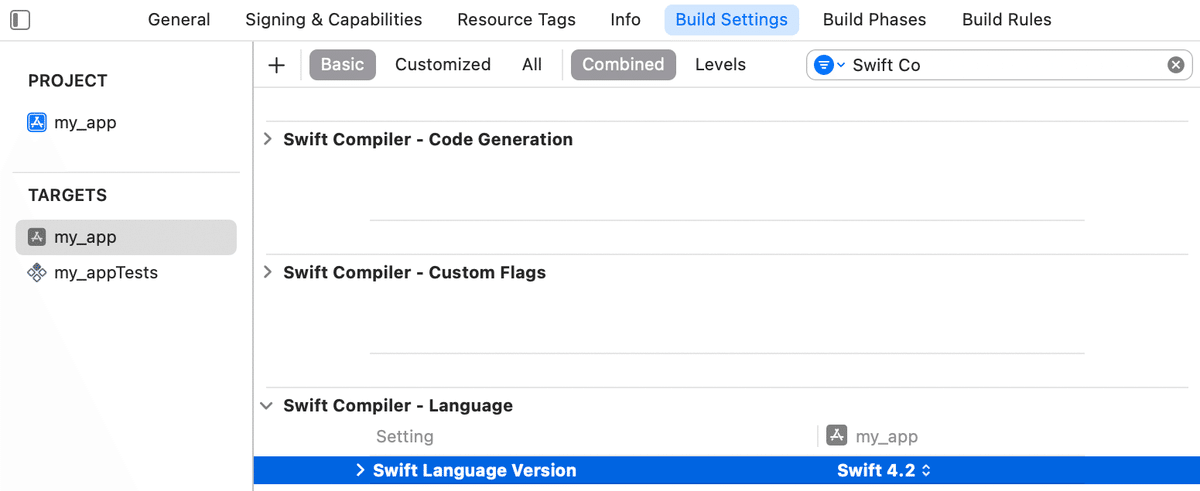
2-4. オーディオの再生
(1) コードの編集。
・App.tsx
import React, { useEffect, useState } from 'react';
import { Button, StyleSheet, View, Text } from 'react-native';
import { Audio } from 'expo-av';
// アプリ
const App = () => {
const [sound, setSound] = useState<Audio.Sound | null>(null);
// 音声ファイルの読み込み
const loadSound = async () => {
const { sound } = await Audio.Sound.createAsync(
require('./assets/sample.mp3')
);
setSound(sound);
};
// 音声ファイルの再生
const playSound = async () => {
if (sound) {
await sound.replayAsync();
}
};
// 初期化
useEffect(() => {
loadSound();
return () => {
if (sound) {
sound.unloadAsync();
}
};
}, []);
// UI
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Button title="オーディオの再生" onPress={playSound} />
</View>
);
}
export default App;
(2) コードの実行。
npm start
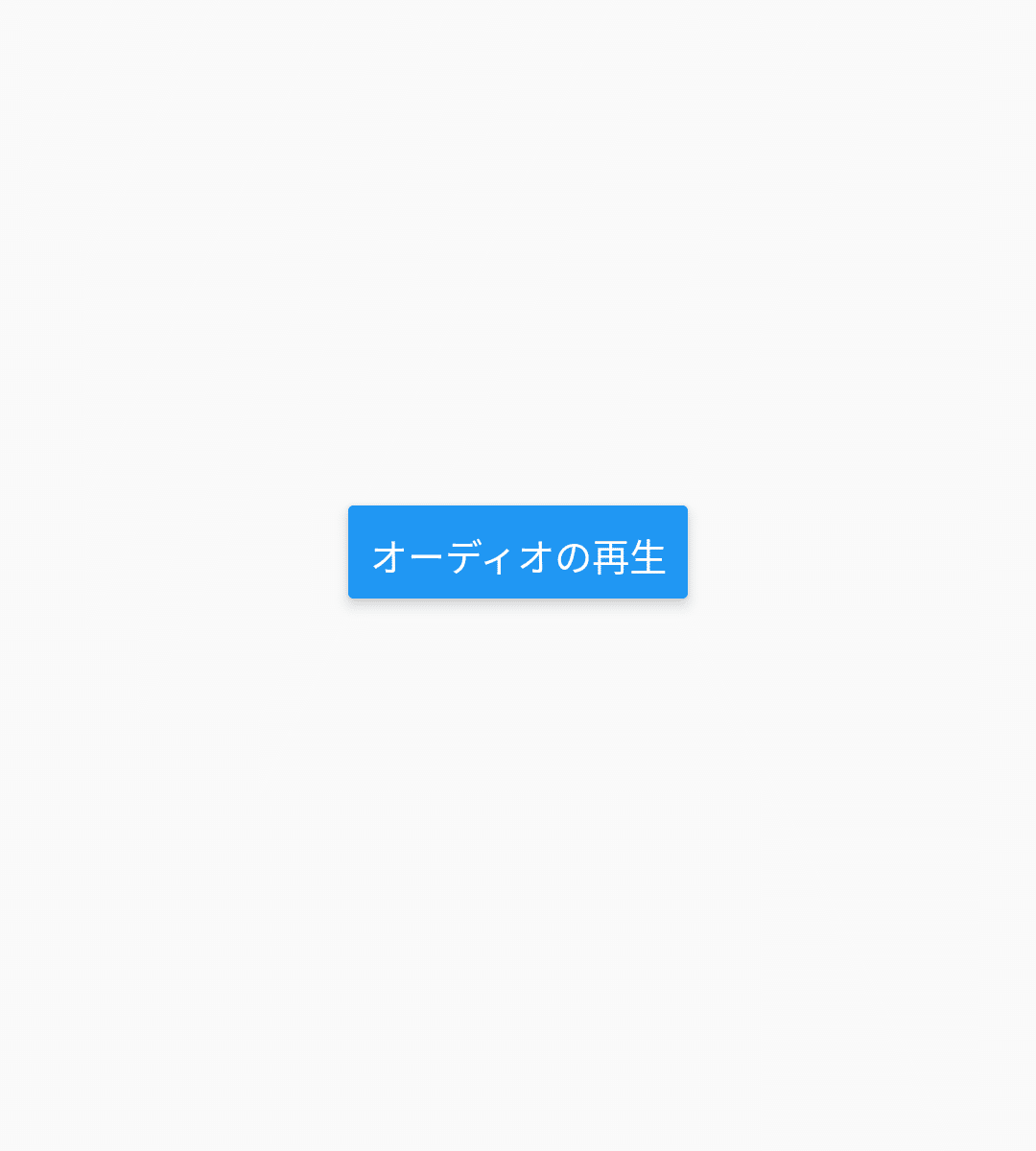
3. ビデオの再生
3-1. アセットの配置
(1) プロジェクト下にassetsフォルダを作成し、ビデオ(sample.mp4)を配置。
・my_app
・assets
・sample.mp4
3-2. ビデオの再生
(1) コードの編集。
・App.tsx
import React from 'react';
import { View, Text } from 'react-native';
import { Video, ResizeMode } from 'expo-av';
// アプリ
const App = () => {
const videoRef = React.useRef<Video>(null);
// UI
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Video
ref={videoRef}
style={{ width: '100%', height: '100%'}}
source={require('./assets/sample.mp4')}
resizeMode={ResizeMode.CONTAIN}
isLooping
isMuted
shouldPlay
/>
</View>
);
}
export default App;
(2) コードの実行。
npm start
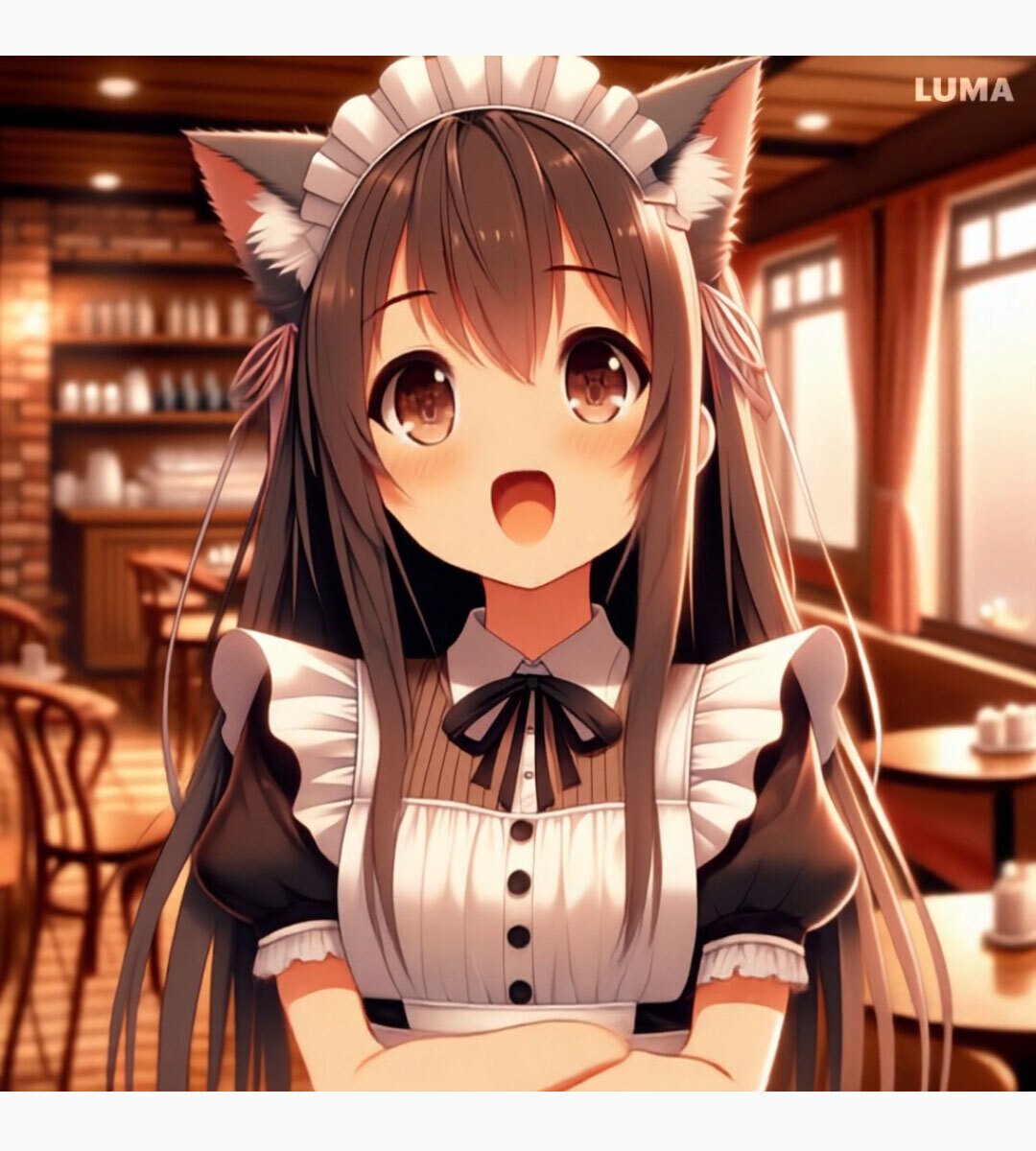
次回
この記事が気に入ったらサポートをしてみませんか?