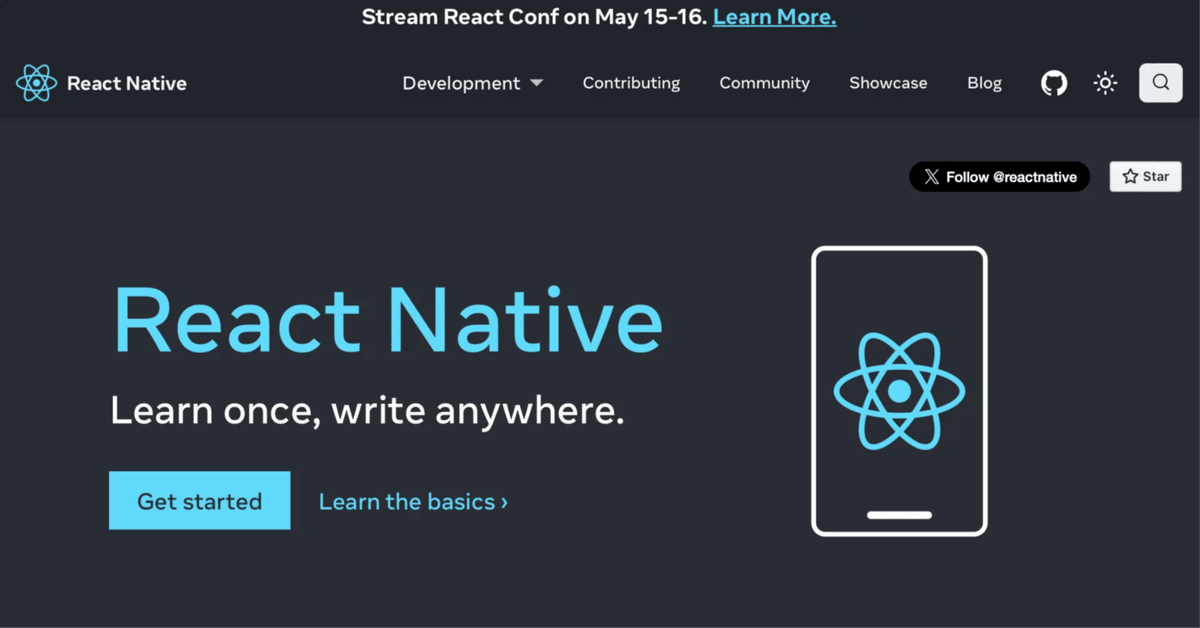
react-native-voice の使い方
「react-native-voice」の使い方をまとめました。
前回
1. react-native-voice
「react-native-video」は、「React Native」で音声認識を行うためのライブラリです。
2. react-native-voiceの使い方
「react-native-voice」の使い方は、次のとおりです。
2-1. React Nativeプロジェクトの作成
(1) React Nativeプロジェクトの作成。
npx react-native init my_app
cd my_app
(2) パッケージのインストール。
npm i @react-native-voice/voice --save
2-2. iOSのセットアップ
(1) podのインストール。
cd ios
pod install
cd ..
(2) Xcodeで署名し、iPhoneにインストールできることを確認。
(3) 「Info.plist」に以下の項目を設定。
<key>NSSpeechRecognitionUsageDescription</key>
<string>音声認識を行うために必要です</string>
<key>NSMicrophoneUsageDescription</key>
<string>マイクへのアクセスが必要です</string>
2-3. Androidのセットアップ
(1) AndroidManifext.xmlに以下のパーミッションを追加。
<uses-permission android:name="android.permission.RECORD_AUDIO" />
2-4. 音声認識の実装
(1) コードの編集。
・App.tsx
import React, { useState, useEffect } from 'react';
import { View, Text, Button, PermissionsAndroid, Platform, StyleSheet } from 'react-native';
import Voice, { SpeechResultsEvent, SpeechErrorEvent } from '@react-native-voice/voice';
// アプリ
const App: React.FC = () => {
const [state, setState] = useState<string>('停止');
const [result, setResult] = useState<string>('');
// 音声認識の開始
const startRecognizing = async () => {
// パーミッションダイアログの表示
if (Platform.OS === 'android') {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.RECORD_AUDIO,
{
title: 'Voice Recognition Permission',
message: 'Voice Recognition needs access to your microphone',
buttonNeutral: 'Ask Me Later',
buttonNegative: 'Cancel',
buttonPositive: 'OK',
},
);
if (granted !== PermissionsAndroid.RESULTS.GRANTED) {
return;
}
}
// 音声認識の開始
try {
await Voice.start('ja-JP');
setState('開始');
setResult('');
} catch (e) {
console.error(e);
}
};
// 音声認識の停止
const stopRecognizing = async () => {
setState('停止');
try {
await Voice.stop();
} catch (e) {
console.error(e);
}
};
// 初期化
useEffect(() => {
// 認識完了時に呼ばれる
Voice.onSpeechResults = (e: SpeechResultsEvent) => {
if (e.value) setResult(e.value[0]);
}
// エラー時に呼ばれる
Voice.onSpeechError = (e: SpeechErrorEvent) => {
console.error(e.error);
stopRecognizing();
};
// UI
return () => {
Voice.destroy().then(Voice.removeAllListeners);
};
}, []);
// UI
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>{state}</Text>
<Text>{result}</Text>
<Button title="音声認識の開始" onPress={startRecognizing} />
<Button title="音声認識の停止" onPress={stopRecognizing} />
</View>
);
};
export default App;
(2) コードの実行。
npm start
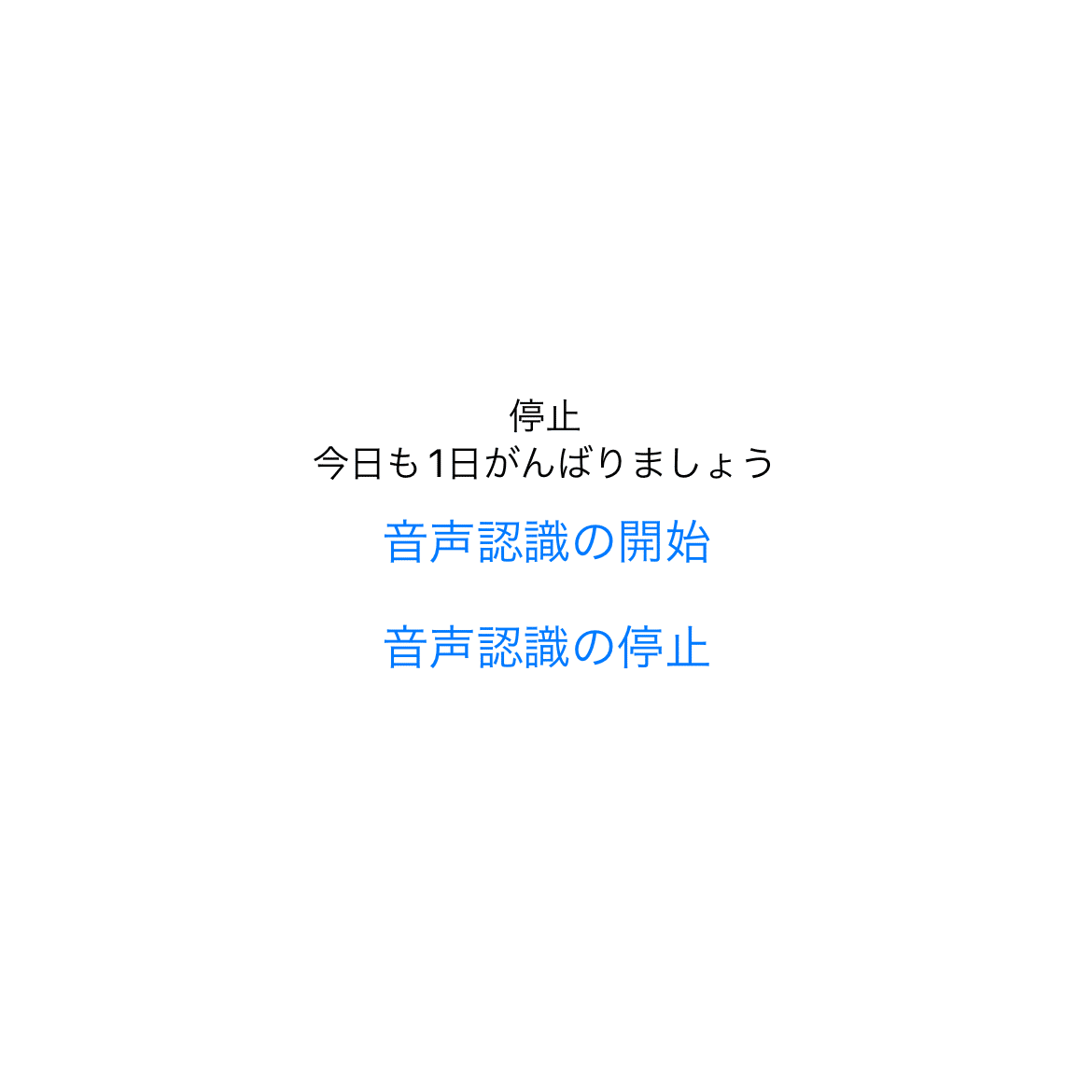
iOSでは正しく動作しましたが、Androidではエラーで該当IssueはOpenのままでした。
・Error: {"message":"5/Client side error"} #248
・fix: new NativeEventEmitter() was called with a non-null argument console warning #460
次回
この記事が気に入ったらサポートをしてみませんか?