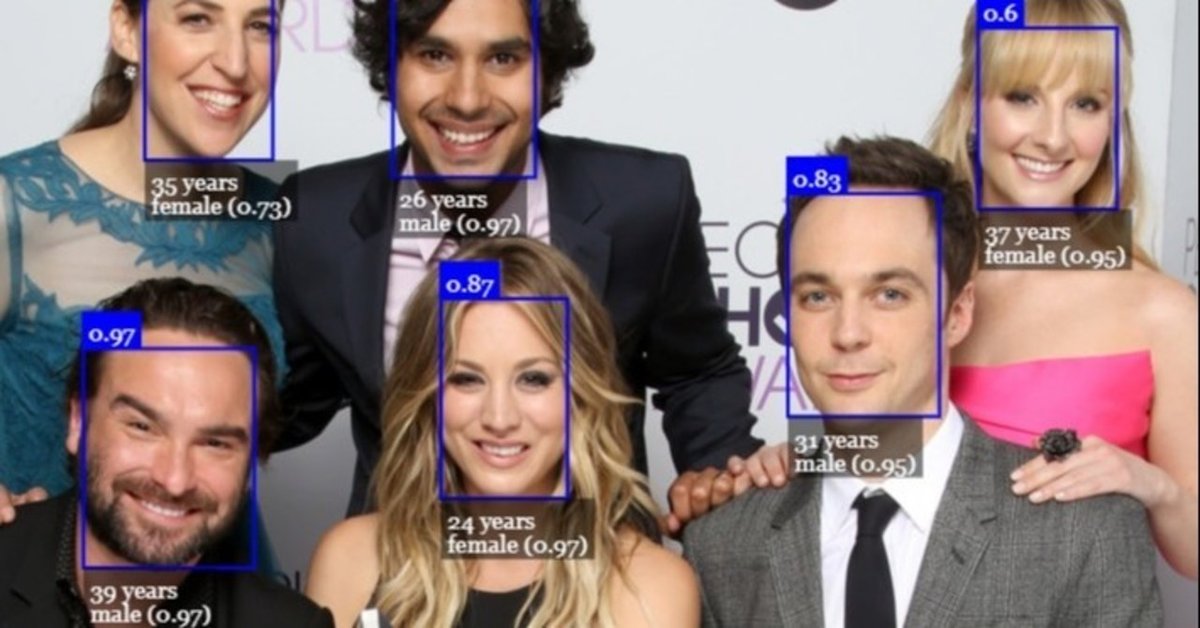
face-api.js - ブラウザでの顔認識を行うJavaScript API
1. face-api.js
「face-api.js」は、顔検出・顔認識のための「JavaScript API」です。「tensorflow.js」(tensorflow/tfjs-core)上に実装されています。
利用可能な顔検出・顔認識モデルは次のとおり。
・ ssdMobilenetv1
精度が高い顔検出。バウンディングボックスと精度を取得。
・ tinyFaceDetector
推論が高速な顔検出。バウンディングボックスと精度を取得。
・ faceLandmark68Net
顔ランドマークを検出。68個の顔のポイントを取得。
・ faceRecognitionNet
2つの顔の類似性を判断。
・ faceExpressionNet
顔の表情の分類。neutral, happy, sad, angry, fearful, disgusted, surprised。
・ ageGenderNet
年齢と性別を推定。
2. 顔検出
顔検出のコードは、次のとおりです。
<!-- face-api.js -->
<script src="js/face-api.min.js"></script>
<!-- テストに使用する画像 -->
<img id="img" src="face.jpg"></img>
<script>
// 画像の取得
const img = document.getElementById('img')
const app = async () => {
// モデルの読み込み
await faceapi.nets.tinyFaceDetector.load("models/");
// 顔検出の実行
const detections = await faceapi.detectAllFaces(img, new faceapi.TinyFaceDetectorOptions())
// 結果の出力
console.log(detections);
}
app()
</script>
◎ face-apiのライブラリとモデルの準備
face-api.jsのリポジトリをクローンします。
git clone https://github.com/justadudewhohacks/face-api.js.git
そして、リポジトリのdistをjs、weightをmodelsに名前変更して、コードと同じフォルダに配置してください。
・index.html
・js ... distを名前変更
・models ... weightを名前変更
◎ モデルの読み込み
顔検出のモデルの読み込みは、以下のメソッドを使います。
・ssdMobilenetv1: faceapi.nets.ssdMobilenetv1.load()
・tinyFaceDetector: faceapi.nets.tinyFaceDetector.load()
await faceapi.nets.tinyFaceDetector.load("models/");
◎ 顔検出の実行
顔検出の実行は、以下の引数でfaceapi.detectAllFaces()を使います。
・ssdMobilenetv1: faceapi.SsdMobilenetv1Options()
・tinyFaceDetector: faceapi.TinyFaceDetectorOptions()
const detections = await faceapi.detectAllFaces(img, new faceapi.TinyFaceDetectorOptions())
◎ 結果の出力
結果の出力は、次のとおりです。
0: dd {_imageDims: Vp, _score: 0.9959318041801453, _classScore: 0.9959318041801453, _className: "", _box: ad}
1: dd {_imageDims: Vp, _score: 0.982027530670166, _classScore: 0.982027530670166, _className: "", _box: ad}
2: dd {_imageDims: Vp, _score: 0.9756534695625305, _classScore: 0.9756534695625305, _className: "", _box: ad}
3: dd {_imageDims: Vp, _score: 0.9497638940811157, _classScore: 0.9497638940811157, _className: "", _box: ad}
_boxにはバウンディングボックスの位置とサイズが含まれています。
_box: ad {_x: 545.497253537178, _y: 198.83009835072627, _width: 134.53352451324463, _height: 148.01298348435208}
3. 顔ランドマーク検出
顔ランドマーク検出のコードは、次のとおりです。
顔検出の結果に対して、顔ランドマーク検出を行っています。
<!-- face-api.js -->
<script src="js/face-api.min.js"></script>
<!-- テストに使用する画像 -->
<img id="img" src="face.jpg"></img>
<script>
// 画像の取得
const img = document.getElementById('img')
const app = async () => {
// モデルの読み込み
await faceapi.nets.tinyFaceDetector.load("models/");
await faceapi.nets.faceLandmark68Net.load("models/");
// 顔ランドマーク検出の実行
const detectionsWithLandmarks = await faceapi.detectAllFaces(img,
new faceapi.TinyFaceDetectorOptions()).withFaceLandmarks()
// 結果の出力
console.log(detectionsWithLandmarks);
}
app()
</script>
◎ モデルの読み込み
顔ランドマーク検出のモデルの読み込みは、以下のメソッドを使います。
・faceLandmark68Net: faceapi.nets.faceLandmark68Net.load()
◎ 顔ランドマーク検出の実行
顔検出のメソッドの後にwithFaceLandmarks()を指定します。
◎ 結果の出力
結果の出力は、次のとおりです。
0: {detection: dd, landmarks: Td, unshiftedLandmarks: Td, alignedRect: dd}
1: {detection: dd, landmarks: Td, unshiftedLandmarks: Td, alignedRect: dd}
2: {detection: dd, landmarks: Td, unshiftedLandmarks: Td, alignedRect: dd}
3: {detection: dd, landmarks: Td, unshiftedLandmarks: Td, alignedRect: dd}
landmarksには顔ランドマークの68ポイントの情報が含まれています。
landmarks: Td
_positions: Array(68)
0: od {_x: 76.95784227922559, _y: 196.56321563416563}
1: od {_x: 74.85605263710022, _y: 217.74829753333174}
:
66: od {_x: 149.0186665058136, _y: 296.99664123946275}
67: od {_x: 141.08693608641624, _y: 294.7601939051732}
4. 顔の表情の分類
顔の表情の分類のコードは、次のとおりです。
顔検出の結果に対して、顔の表情の分類を行っています。
<!-- face-api.js -->
<script src="js/face-api.min.js"></script>
<!-- テストに使用する画像 -->
<img id="img" src="face.jpg"></img>
<script>
// 画像の取得
const img = document.getElementById('img')
const app = async () => {
// モデルの読み込み
await faceapi.nets.tinyFaceDetector.load("models/");
await faceapi.nets.faceExpressionNet.load("models/");
// 顔の表情の分類
const detectionsWithExpressions = await faceapi.detectAllFaces(img,
new faceapi.TinyFaceDetectorOptions()).withFaceExpressions()
// 結果の出力
console.log(detectionsWithExpressions);
}
app()
</script>
◎ モデルの読み込み
顔の表情の分類のモデルの読み込みは、以下のメソッドを使います。
・faceExpressionNet: nets.faceExpressionNet.load()
◎ 顔の表情の分類の実行
顔検出のメソッドの後にwithFaceExpressions()を指定します。
◎ 結果の出力
結果の出力は、次のとおりです。
0: {detection: dd, expressions: tm}
1: {detection: dd, expressions: tm}
2: {detection: dd, expressions: tm}
3: {detection: dd, expressions: tm}
expressionsには顔の表情の分類が含まれています。
・neutral: ニュートラル
・happy: 喜び
・sad: 悲しみ
・angry: 怒り
・fearful: 恐れ
・disgusted: うんざり
・surprised: 驚き
expressions: tm
angry: 0.00033354622428305447
disgusted: 0.025459259748458862
fearful: 0.00001721922126307618
happy: 0.23955923318862915
neutral: 0.46917790174484253
sad: 0.2652508020401001
surprised: 0.00020209986541885883
5. 利用可能なタスク構成
利用可能なタスク構成は、次のとおりです。
// 複数の顔検出
await faceapi.detectAllFaces(input)
await faceapi.detectAllFaces(input).withFaceExpressions()
await faceapi.detectAllFaces(input).withFaceLandmarks()
await faceapi.detectAllFaces(input).withFaceLandmarks().withFaceExpressions()
await faceapi.detectAllFaces(input).withFaceLandmarks().withFaceExpressions().withFaceDescriptors()
await faceapi.detectAllFaces(input).withFaceLandmarks().withAgeAndGender().withFaceDescriptors()
await faceapi.detectAllFaces(input).withFaceLandmarks().withFaceExpressions().withAgeAndGender().withFaceDescriptors()
// 単一の顔検出
await faceapi.detectSingleFace(input)
await faceapi.detectSingleFace(input).withFaceExpressions()
await faceapi.detectSingleFace(input).withFaceLandmarks()
await faceapi.detectSingleFace(input).withFaceLandmarks().withFaceExpressions()
await faceapi.detectSingleFace(input).withFaceLandmarks().withFaceExpressions().withFaceDescriptor()
await faceapi.detectSingleFace(input).withFaceLandmarks().withAgeAndGender().withFaceDescriptor()
await faceapi.detectSingleFace(input).withFaceLandmarks().withFaceExpressions().withAgeAndGender().withFaceDescriptor()
この記事が気に入ったらサポートをしてみませんか?