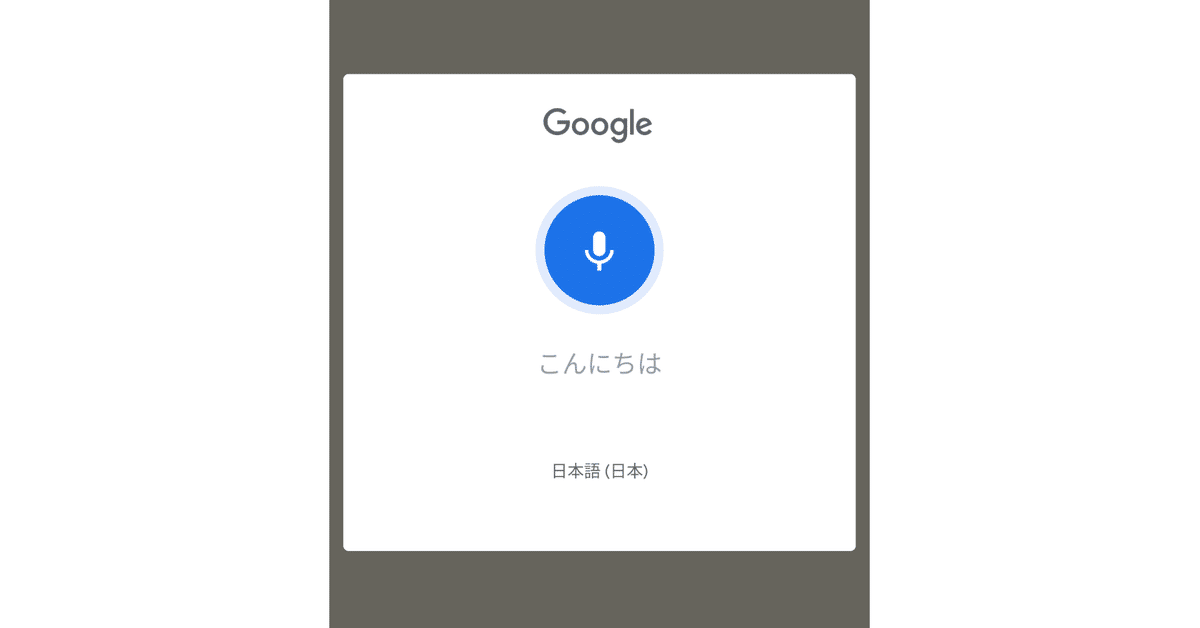
Android の 音声認識 と 音声合成 を試す
「Android」の「音声認識」と「音声合成」を試したので、まとめました。
・API 34 (Android 14)
1. Androidの音声認識
(1) コードの編集。
・MainActivity.kt
package net.npaka.helloworld
import android.content.ActivityNotFoundException
import android.content.Intent
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.activity.enableEdgeToEdge
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import net.npaka.helloworld.ui.theme.HelloWorldTheme
import androidx.activity.result.contract.ActivityResultContracts.StartActivityForResult
import android.speech.RecognizerIntent
// メイン
class MainActivity : ComponentActivity() {
private val _recognizedText = mutableStateOf("")
// コンストラクタ
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge()
// UI
setContent {
HelloWorldTheme {
Scaffold(modifier = Modifier.fillMaxSize()) { innerPadding ->
Column(modifier = Modifier.padding(innerPadding).padding(16.dp)) {
Button(onClick = { startVoiceRecognition() }) {
Text(text = "音声認識")
}
Text(text = _recognizedText.value)
}
}
}
}
}
// 音声認識の開始
private fun startVoiceRecognition() {
val intent = Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH)
intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM)
intent.putExtra(RecognizerIntent.EXTRA_PROMPT, "Speak now")
try {
speechResultLauncher.launch(intent)
} catch (e: ActivityNotFoundException) {
e.printStackTrace()
}
}
// 音声認識結果リスナー
private val speechResultLauncher = registerForActivityResult(StartActivityForResult()) { result ->
if (result.resultCode == RESULT_OK && result.data != null) {
val matches = result.data!!.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS)
val recognizedText = matches?.get(0) ?: ""
_recognizedText.value = recognizedText
}
}
}
(2) 音声認識ボタンを押す。
音声認識が開始し、文字起こしします。
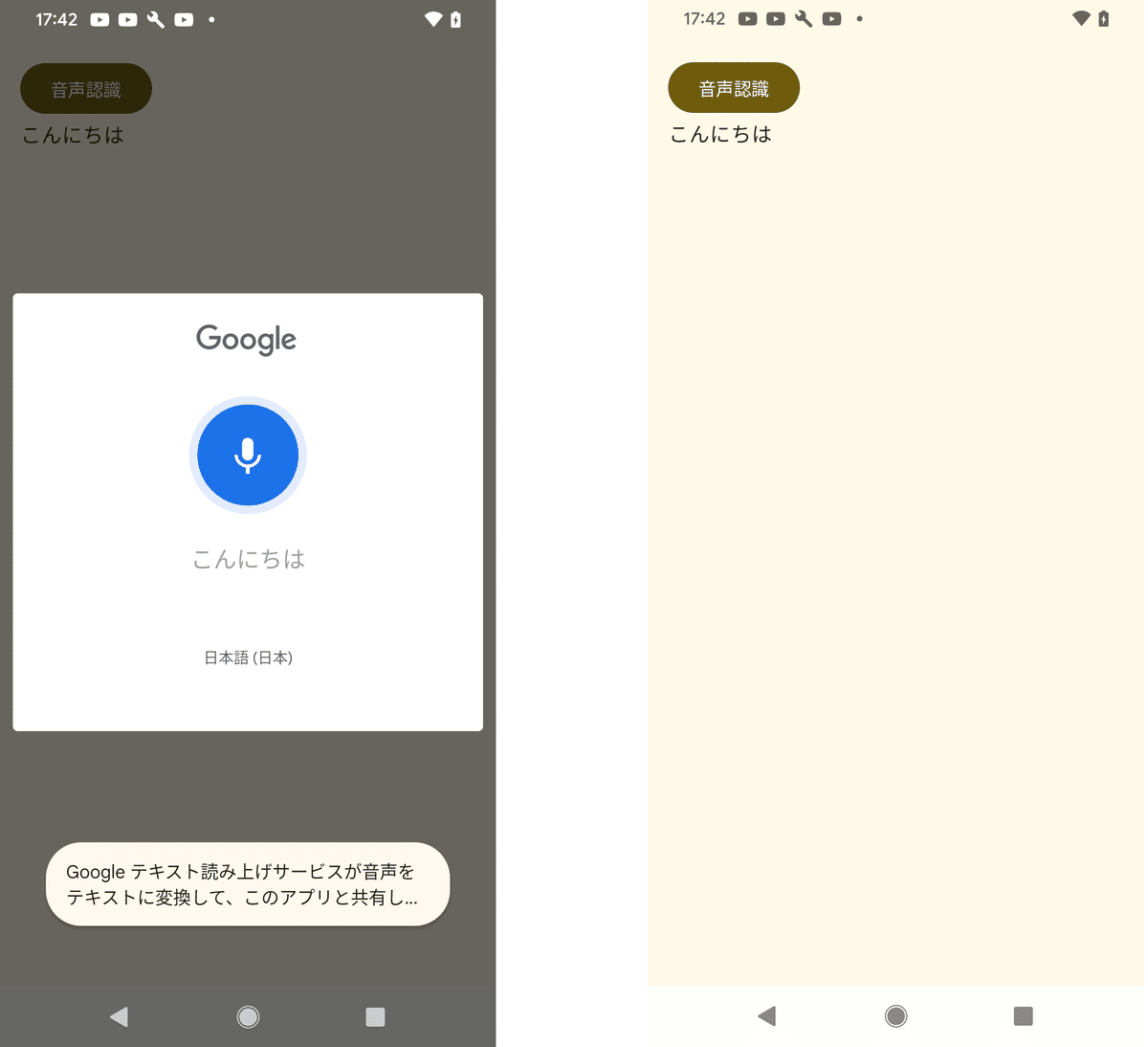
2. Androidの音声合成
(1) コードの記述。
・MainActivity.kt
package net.npaka.helloworld
import android.os.Bundle
import android.speech.tts.TextToSpeech
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.activity.enableEdgeToEdge
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.Column
import androidx.compose.material3.Button
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.rememberUpdatedState
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import net.npaka.helloworld.ui.theme.HelloWorldTheme
import java.util.*
// メイン
class MainActivity : ComponentActivity(), TextToSpeech.OnInitListener {
private lateinit var tts: TextToSpeech
// コンストラクタ
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge()
// TTSの準備
tts = TextToSpeech(this, this)
// UI
setContent {
HelloWorldTheme {
Scaffold(modifier = Modifier.fillMaxSize()) { innerPadding ->
Column(modifier = Modifier.padding(innerPadding).padding(16.dp)) {
Button(onClick = { speak("こんにちは") }) {
Text("音声合成")
}
}
}
}
}
}
// TTSの初期化完了時に呼ばれる
override fun onInit(status: Int) {
if (status == TextToSpeech.SUCCESS) {
tts.language = Locale.JAPAN
}
}
// 音声合成
private fun speak(text: String) {
tts.speak(text, TextToSpeech.QUEUE_FLUSH, null, null)
}
// デストラクタ
override fun onDestroy() {
if (this::tts.isInitialized) {
tts.stop()
tts.shutdown()
}
super.onDestroy()
}
}
(2) 音声合成ボタンを押す。
「こんにちは」と発話します。
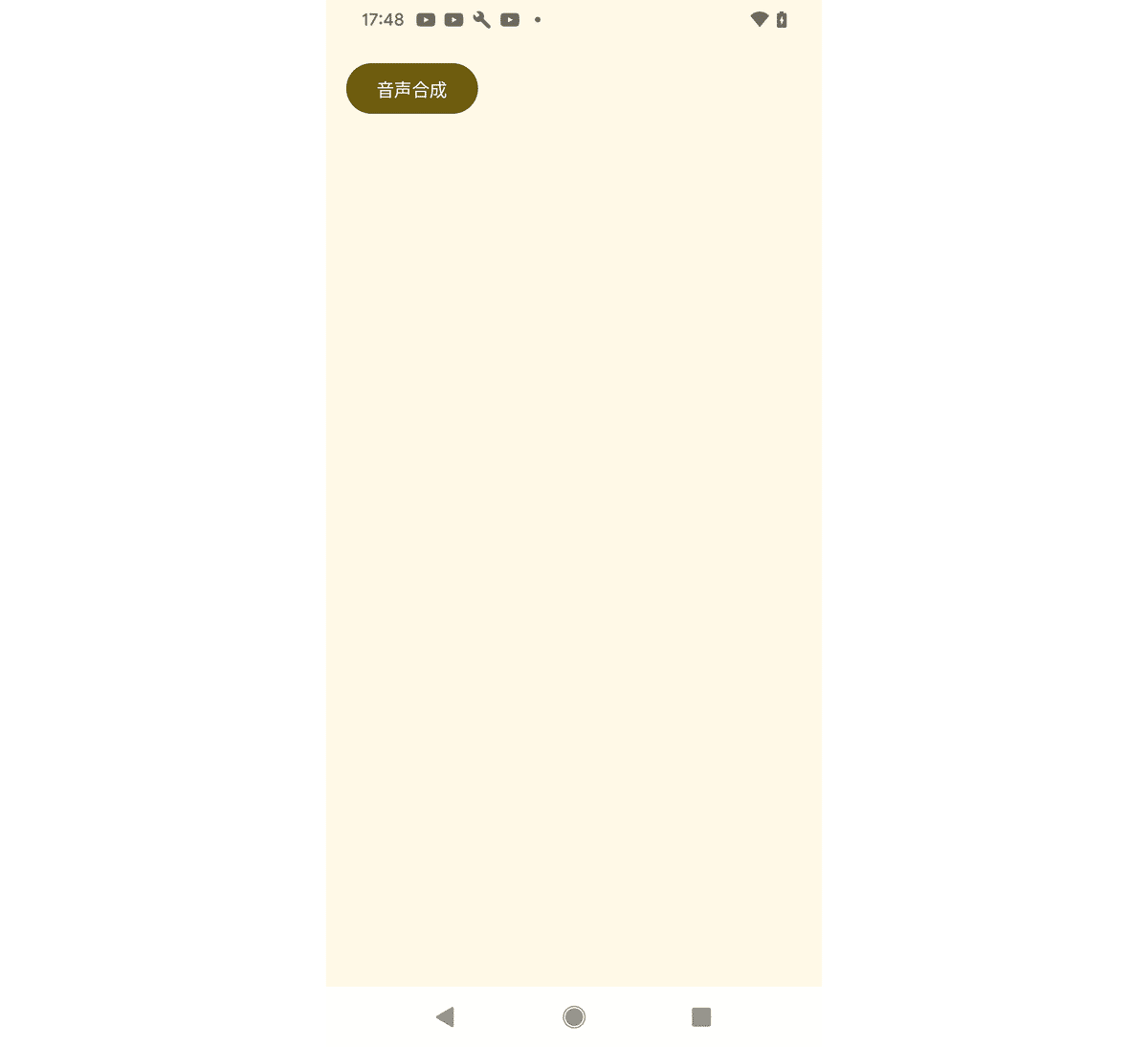
この記事が気に入ったらサポートをしてみませんか?